Simply generate the code and find the MDK driver file
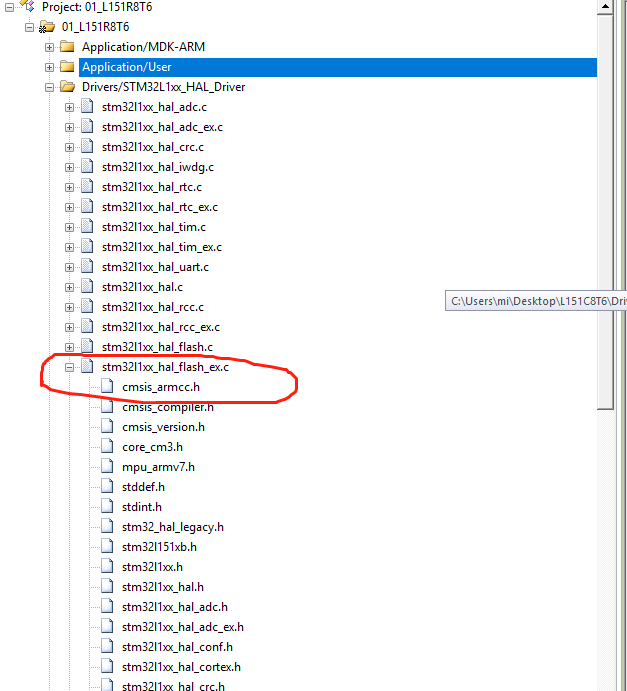
Official Recommended Steps
##### Data EEPROM Programming Function#####
===============================================================================
[.] Any operation of erasing or programming should follow the following steps: (#) Call the @ref HAL_FLASHEx_DATAEEPROM_Unlock() function to enable data EEPROM access Erase control register access with Flash program. Call the required functions to erase or program data. (#) Call @ref HAL_FLASHEx_DATAEEPROM_Lock() to disable data EEPROM access Erase control register access with Flash programs (recommended) Protect DATA_EEPROM from possible accidental operations.
It only takes three steps to summarize the experiment.
- Unlock
- Write data (erase and write data but write 0x00)
- Locking (it's recommended, but it's useless. It's not a big problem to use it.)
void Program_EEPROM(void) { uint32_t data = 0x12345678; HAL_FLASHEx_DATAEEPROM_Unlock(); HAL_FLASHEx_DATAEEPROM_Program( FLASH_TYPEPROGRAMDATA_WORD, 0x08080000, data); HAL_FLASHEx_DATAEEPROM_Program( FLASH_TYPEPROGRAMDATA_WORD, 0x08080000 + 4, data); HAL_FLASHEx_DATAEEPROM_Erase(FLASH_TYPEERASEDATA_WORD, 0x08080000); HAL_FLASHEx_DATAEEPROM_Lock(); }
- FLASH_TYPEPROGRAMDATA_WORD is a data type and can be Program word (32-bit) at a specified address OR Program word (16-bit) at a specified address OR Program word (8-bit) at a specified address.
- 0x08080000 is the address, which can be found in the official manual.
- Data is written data
Actual operation effect
Erase is also write
/** * @brief Erase a word in data memory. * @param Address specifies the address to be erased. * @param TypeErase Indicate the way to erase at a specified address. * This parameter can be a value of @ref FLASH_Type_Program * @note To correctly run this function, the @ref HAL_FLASHEx_DATAEEPROM_Unlock() function * must be called before. * Call the @ref HAL_FLASHEx_DATAEEPROM_Lock() to the data EEPROM access * and Flash program erase control register access(recommended to protect * the DATA_EEPROM against possible unwanted operation). * @retval HAL_StatusTypeDef HAL Status */ HAL_StatusTypeDef HAL_FLASHEx_DATAEEPROM_Erase(uint32_t TypeErase, uint32_t Address) { HAL_StatusTypeDef status = HAL_OK; /* Check the parameters */ assert_param(IS_TYPEPROGRAMDATA(TypeErase)); assert_param(IS_FLASH_DATA_ADDRESS(Address)); /* Wait for last operation to be completed */ status = FLASH_WaitForLastOperation(FLASH_TIMEOUT_VALUE); if(status == HAL_OK) { /* Clean the error context */ pFlash.ErrorCode = HAL_FLASH_ERROR_NONE; if(TypeErase == FLASH_TYPEERASEDATA_WORD) { /* Write 00000000h to valid address in the data memory */ *(__IO uint32_t *) Address = 0x00000000U; } if(TypeErase == FLASH_TYPEERASEDATA_HALFWORD) { /* Write 0000h to valid address in the data memory */ *(__IO uint16_t *) Address = (uint16_t)0x0000; } if(TypeErase == FLASH_TYPEERASEDATA_BYTE) { /* Write 00h to valid address in the data memory */ *(__IO uint8_t *) Address = (uint8_t)0x00; } status = FLASH_WaitForLastOperation(FLASH_TIMEOUT_VALUE); } /* Return the erase status */ return status; }
If you don't understand it, you can search for pointers to force type conversion.
I have a couple of questions that I'm confused about.
- Does a new download erase this subregion?
- Can flash use the same function?