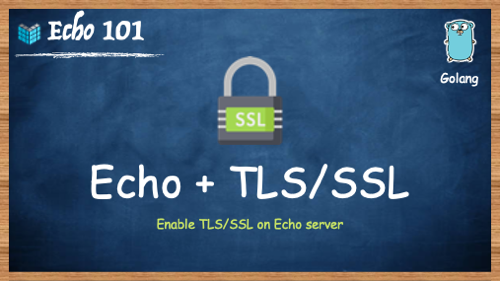
introduce
With a complete example, turning on TLS/SSL in the Echo framework is what we call https.
We will use rk-boot To start the microservice for the Echo framework.
Visit the following address for the complete tutorial:
Generate Self-Signed Certificate
Users can purchase certificates from major cloud vendors or use them cfssl Create a custom certificate.
We describe how to generate certificates locally.
1. Download cfssl & cfssljson command line
The rk command line is recommended for download.
$ go get -u github.com/rookie-ninja/rk/cmd/rk $ rk install cfssl $ rk install cfssljson
Official Download
$ go get github.com/cloudflare/cfssl/cmd/cfssl $ go get github.com/cloudflare/cfssl/cmd/cfssljson
2. Generate CA
$ cfssl print-defaults config > ca-config.json $ cfssl print-defaults csr > ca-csr.json
Modify ca-config.json and ca-csr.json as needed.
$ cfssl gencert -initca ca-csr.json | cfssljson -bare ca -
3. Generate service-side certificates
server.csr, server.pem, and server-key.pem will be generated.
$ cfssl gencert -config ca-config.json -ca ca.pem -ca-key ca-key.pem -profile www ca-csr.json | cfssljson -bare server
install
go get github.com/rookie-ninja/rk-boot
Quick Start
rk-boot Support for gRPC services to obtain certificates in the following ways.
- Local File System
- Remote File System
- Consul
- ETCD
Let's first see how to get a certificate locally and start it.
1. Create boot.yaml
In this example, we only start the server-side certificate. locale is used to distinguish certs in different environments.
Refer to previous articles for details:
--- cert: - name: "local-cert" # Required provider: "localFs" # Required, etcd, consul, localFs, remoteFs are supported options locale: "*::*::*::*" # Required, default: "" serverCertPath: "cert/server.pem" # Optional, default: "", path of certificate on local FS serverKeyPath: "cert/server-key.pem" # Optional, default: "", path of certificate on local FS echo: - name: greeter port: 8080 enabled: true enableReflection: true cert: ref: "local-cert" # Enable grpc TLS commonService: enabled: true
2. Create main.go
package main import ( "context" "github.com/rookie-ninja/rk-boot" ) // Application entrance. func main() { // Create a new boot instance. boot := rkboot.NewBoot() // Bootstrap boot.Bootstrap(context.Background()) // Wait for shutdown sig boot.WaitForShutdownSig(context.Background()) }
3. Folder structure
. ├── boot.yaml ├── cert │ ├── server-key.pem │ └── server.pem ├── go.mod ├── go.sum └── main.go 1 directory, 6 files
4. Start main.go
$ go run main.go
5. Validation
$ curl -X GET --insecure https://localhost:8080/rk/v1/healthy {"healthy":true}
Framework
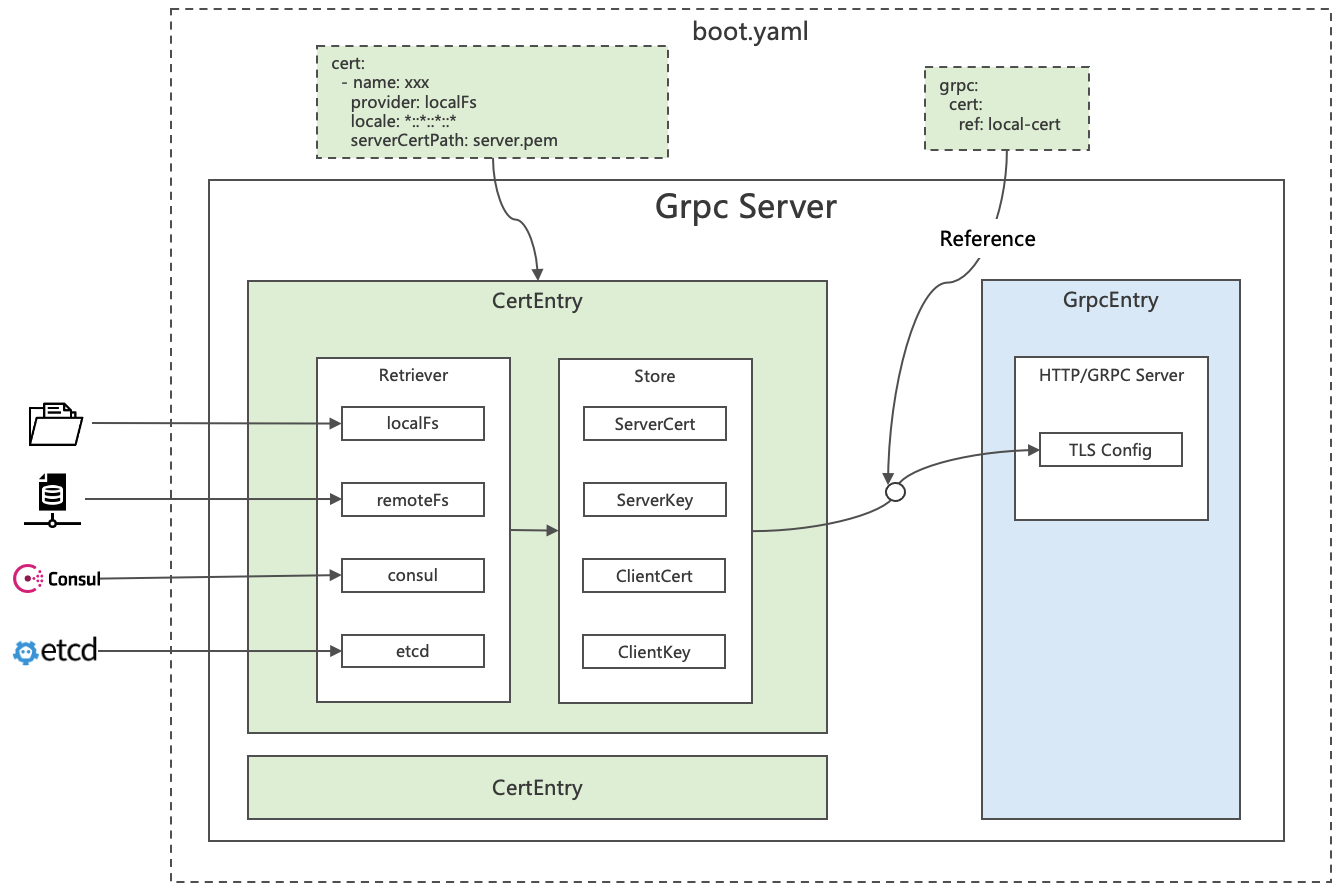
Parameter introduction
1. Read certificates locally
Configuration Items | details | Need | Default value |
---|---|---|---|
cert.localFs.name | Local File System Getter Name | yes | "" |
cert.localFs.locale | Compliance with locale: \<realm>:\<region>:\<az>:\<domain> | yes | "" |
cert.localFs.serverCertPath | Server Certificate Path | no | "" |
cert.localFs.serverKeyPath | Server Certificate Key Path | no | "" |
cert.localFs.clientCertPath | Client Certificate Path | no | "" |
cert.localFs.clientCertPath | Client Certificate Key Path | no | "" |
- Example
--- cert: - name: "local-cert" # Required description: "Description of entry" # Optional provider: "localFs" # Required, etcd, consul, localFs, remoteFs are supported options locale: "*::*::*::*" # Required, default: "" serverCertPath: "cert/server.pem" # Optional, default: "", path of certificate on local FS serverKeyPath: "cert/server-key.pem" # Optional, default: "", path of certificate on local FS echo: - name: greeter port: 8080 enabled: true enableReflection: true cert: ref: "local-cert" # Enable grpc TLS
2. Read certificates from remote file service
Configuration Items | details | Need | Default value |
---|---|---|---|
cert.remoteFs.name | Remote File Service Getter Name | yes | "" |
cert.remoteFs.locale | Obey locale:\<realm>:\<region>:\<region>:\<az>:\<domain> | yes | "" |
cert.remoteFs.endpoint | Remote address: http://x.x.x.x Or x.x.x.x | yes | N/A |
cert.remoteFs.basicAuth | Basic auth: <user:pass>. | no | "" |
cert.remoteFs.serverCertPath | Server Certificate Path | no | "" |
cert.remoteFs.serverKeyPath | Server Certificate Key Path | no | "" |
cert.remoteFs.clientCertPath | Client Certificate Path | no | "" |
cert.remoteFs.clientCertPath | Client Certificate Key Path | no | "" |
- Example
--- cert: - name: "remote-cert" # Required description: "Description of entry" # Optional provider: "remoteFs" # Required, etcd, consul, localFs, remoteFs are supported options endpoint: "localhost:8081" # Required, both http://x.x.x.x or x.x.x.x are acceptable locale: "*::*::*::*" # Required, default: "" serverCertPath: "cert/server.pem" # Optional, default: "", path of certificate on local FS serverKeyPath: "cert/server-key.pem" # Optional, default: "", path of certificate on local FS echo: - name: greeter port: 8080 enabled: true cert: ref: "remote-cert" # Enable grpc TLS
3. Read certificates from Consul
Configuration Items | details | Need | Default value |
---|---|---|---|
cert.consul.name | Consul Getter Name | yes | "" |
cert.consul.locale | Compliance with locale: \<realm>:\<region>:\<az>:\<domain> | yes | "" |
cert.consul.endpoint | Consul address: http://x.x.x.x Or x.x.x.x | yes | N/A |
cert.consul.datacenter | Consul Data Center | yes | "" |
cert.consul.token | Consul Access Key | no | "" |
cert.consul.basicAuth | Consul Basic auth, format: <user:pass>. | no | "" |
cert.consul.serverCertPath | Server Certificate Path | no | "" |
cert.consul.serverKeyPath | Server Certificate Key Path | no | "" |
cert.consul.clientCertPath | Server Certificate Key Path | no | "" |
cert.consul.clientCertPath | Server Certificate Key Path | no | "" |
- Example
--- cert: - name: "consul-cert" # Required provider: "consul" # Required, etcd, consul, localFS, remoteFs are supported options description: "Description of entry" # Optional locale: "*::*::*::*" # Required, "" endpoint: "localhost:8500" # Required, http://x.x.x.x or x.x.x.x both acceptable. datacenter: "dc1" # Optional, default: "", consul datacenter serverCertPath: "server.pem" # Optional, default: "", key of value in consul serverKeyPath: "server-key.pem" # Optional, default: "", key of value in consul echo: - name: greeter port: 8080 enabled: true cert: ref: "consul-cert" # Enable grpc TLS
4. Read certificates from ETCD
Configuration Items | details | Need | Default value |
---|---|---|---|
cert.etcd.name | ETCD Getter Name | yes | "" |
cert.etcd.locale | Compliance with locale: \<realm>:\<region>:\<az>:\<domain> | yes | "" |
cert.etcd.endpoint | ETCD address: http://x.x.x.x Or x.x.x.x | yes | N/A |
cert.etcd.basicAuth | ETCD basic auth, format: <user:pass>. | no | "" |
cert.etcd.serverCertPath | Server Certificate Path | no | "" |
cert.etcd.serverKeyPath | Server Certificate Path | no | "" |
cert.etcd.clientCertPath | Client Certificate Path | no | "" |
cert.etcd.clientCertPath | Client Certificate Key Path | no | "" |
- Example
--- cert: - name: "etcd-cert" # Required description: "Description of entry" # Optional provider: "etcd" # Required, etcd, consul, localFs, remoteFs are supported options locale: "*::*::*::*" # Required, default: "" endpoint: "localhost:2379" # Required, http://x.x.x.x or x.x.x.x both acceptable. serverCertPath: "server.pem" # Optional, default: "", key of value in etcd serverKeyPath: "server-key.pem" # Optional, default: "", key of value in etcd echo: - name: greeter port: 8080 enabled: true cert: ref: "etcd-cert" # Enable grpc TLS