01 knapsack problem is a classical dynamic programming problem. The scenario is described as follows:
Image description: thief, entering a mansion at night, can steal a lot of things, but the ability to bear the weight is limited. Which can steal more?
Further abstractions are:
givenItems, each with its own weight
And value
, at the limited total weight / total capacity
Select several of them (i.e. 0 or 1 item can be selected for each item), and design options make the total value of items the highest
The top-level abstract description is the mathematical language, which is described as follows:
Conditions:
1: Pairs of numbers
2: positive integer
To solve the 0-1 programming problem:
0-1 knapsack problem substructure: select a given item, you need to compare and select
The optimal solution and no choice of the subproblem formed by
The optimal solution of the subproblem. It is divided into two subproblems to choose the best one.
Recursive process of 0-1 knapsack problem: with n items, the weight of knapsack is,
Is the optimal solution. That is to say, it only needs a formula to judge three conditions to get the optimal solution:
With this formula to judge the three conditions, the code is easier to implement. The Java implementation source code is as follows:
public class CB { public static void main(String[] args) { int totalWeight = 10; //Number of items, Backpack Capacity Bean[] data = new Bean[]{new Bean(0, 0), new Bean(2, 6), new Bean(2, 3), new Bean(6, 5), new Bean(5, 4), new Bean(4, 6)}; System.out.println(getMaxValue(data, totalWeight)); } public static int getMaxValue(Bean[] data, int totalWeight) { int n = data.length; int[][] table = new int[n][totalWeight + 1]; for (int i = 1; i < n; i++) { //Goods for (int w = 1; w <= totalWeight; w++) { //Backpack size if (data[i].weight > w) { //The weight of the current item i is larger than the capacity of the backpack j. if you can't fit it, you must not table[i][w] = table[i - 1][w]; } else { //Hold it, Max {hold item i, no item i} table[i][w] = Math.max(table[i - 1][w], table[i - 1][w - data[i].weight] + data[i].value); } } } for (int f = 0; f < table.length; f++) { System.out.println(Arrays.toString(table[f])); } return table[n - 1][totalWeight]; } static class Bean { int weight = 0; int value = 0; Bean(int w, int v) { weight = w; value = v; } @Override public String toString() { return weight + " " + value; } } }
We initialize five pairsTotal weight
The final output of the algorithm is as follows:
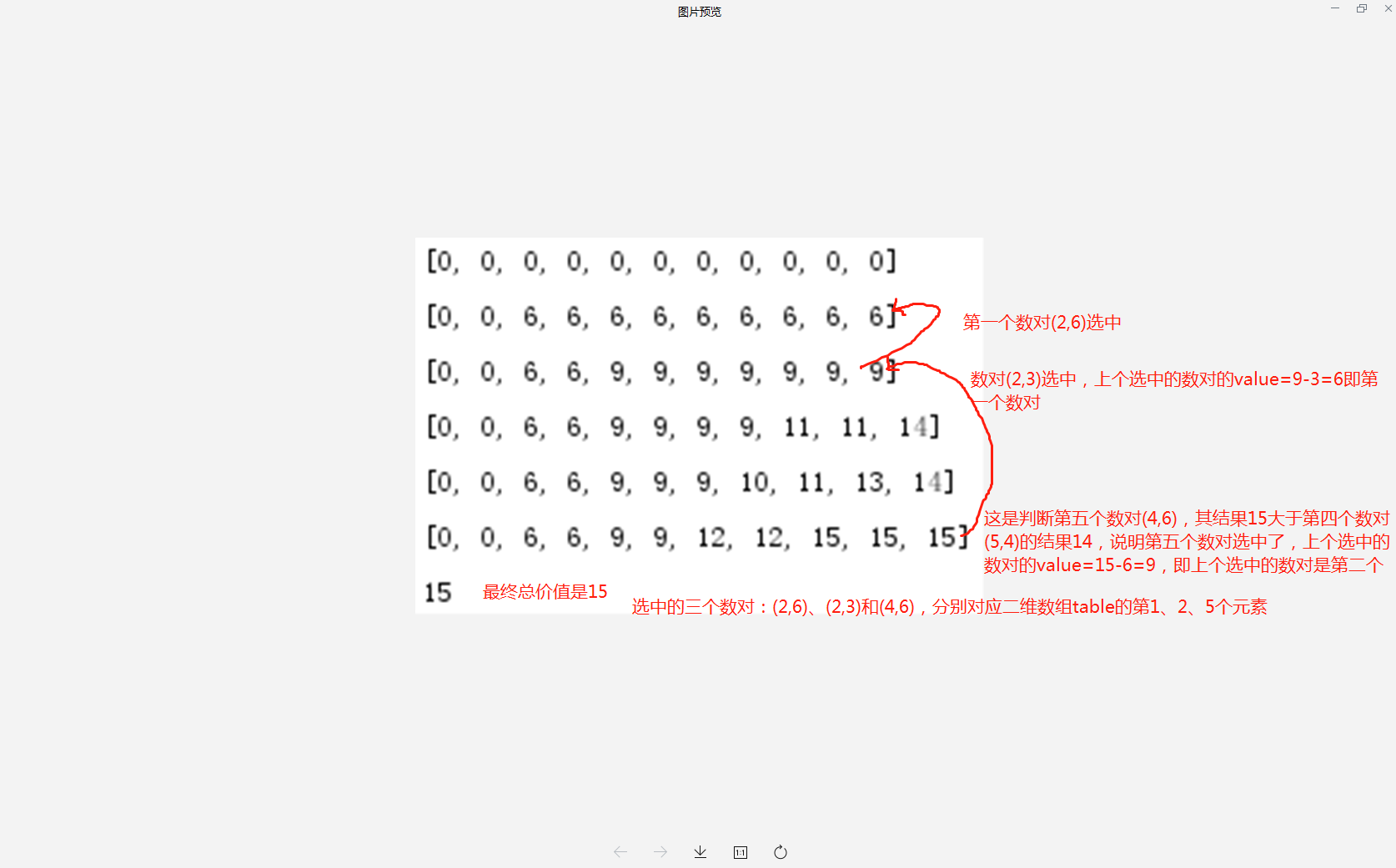