catalogue
Why is there dynamic memory management
Introduction to dynamic memory functions
Why is there dynamic memory management
1. You can apply for large memory for large-scale applications
2. The application can be made during the running of the program, and the memory space can be used more flexibly.
(malloc() function is used to apply for space. It can only be called when the program is running. The compilation process has already passed.)
How to manage space?
Apply for space and use through malloc function, and release space with free function
Common details:
1. Overall application and release.
2. If the requested memory is not returned, it will cause memory leakage.
Memory leak: a serious problem that causes less and less available memory.
When the process exits, the memory leak problem is gone.
The program is divided into: 1. The program that runs immediately ends and the resident program (the program that is endless and will not end). The resident program is easy to cause memory leakage.
3. The application has a size. Free didn't tell us how many bytes should be released? So how does free know how much to release? At present, the free parameter can only know where to release.
When applying, the space actually applied for will be larger than the space you want, and the larger part will be used to save the "original information" of this application
Original information: attribute information (corresponding to the size of heap space, etc.)
4. Heap space is suitable for the application of large space (because more application space is needed to save attribute information)
5. What exactly did free do?
Cancel the relationship between the address saved inside the pointer and heap space, but the value of the pointer remains unchanged. Therefore, it is recommended to set the pointer to NULL after free ing space.
Introduction to dynamic memory functions
malloc and free
Dynamic memory development function provided by C language
void* malloc(size_t size);
void free (void* ptr);
calloc
void* calloc(size_t num,size_t size);
realloc
The realloc function can adjust the size of the requested memory.
void* realloc (void* ptr, size_t size);
ptr is the memory address to be adjusted; New size after size adjustment; The return value is the adjusted memory starting position.
Common dynamic memory errors
1, Dereference operation on NULL pointer
void test() { int *p = (int *)malloc(INT_MAX/4); *p = 20;//If the value of p is NULL, there is a problem free(p); }
void test() { int i = 0; int *p = (int *)malloc(10*sizeof(int)); if(NULL == p) { exit(EXIT_FAILURE); } for(i=0; i<=10; i++) { *(p+i) = i;//Cross border access when i is 10 } free(p); }
3, free the non dynamic space
void test() { int a = 10; int *p = &a; free(p);//ok? }
4, Use free to release part of the memory space (it must be applied and released as a whole)
void test() { int *p = (int *)malloc(100); p++; free(p);//p no longer points to the beginning of dynamic memory } than
5, Multiple releases of the same dynamic memory
void test() { int *p = (int *)malloc(100); free(p); free(p);//Repeated release }
6, Dynamic memory forget release (memory leak)
void test() { int *p = (int *)malloc(100); if(NULL != p) { *p = 20; } } int main() { test(); while(1); }
Written test questions
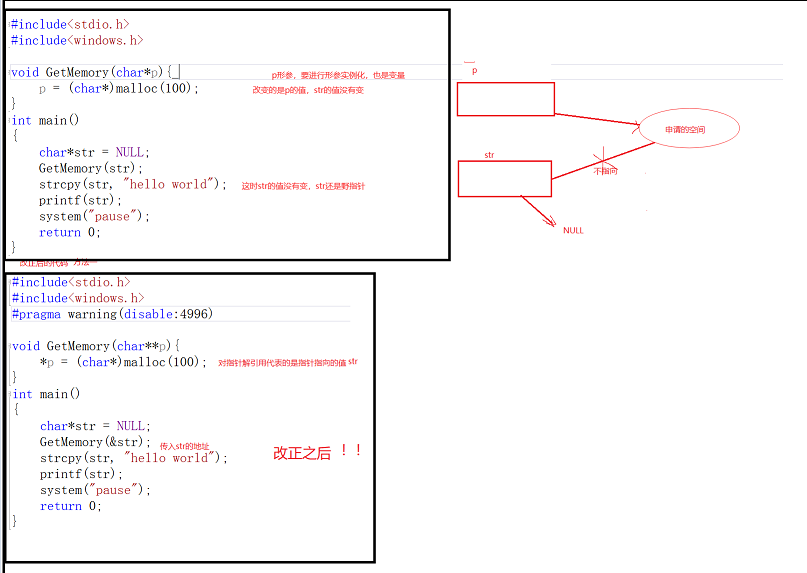
2. Topic 2
char *GetMemory(void) { char p[] = "hello world"; return p; } void Test(void) { char *str = NULL; str = GetMemory(); printf(str); }
The function is opened on the stack. After the function is called, it is released, so there is no return value.
Note: the free space in the computer will not empty the data (the setting data is invalid), so str still points to Hello world
It can be seen from the figure above that the function has been executed, but Hello world still exists, so why not output str?
That's because printf is also a function, and it needs to open up space on the stack to be called, so it will overwrite the space originally saved for Hello world data, so it can't be output.
3. Topic 3:
void GetMemory(char **p, int num) { *p = (char *)malloc(num); } void Test(void) { char *str = NULL; GetMemory(&str, 100); strcpy(str, "hello"); printf(str); }
The validity of the parameters is not judged, and the space requested by malloc is not released (memory leakage may occur!!).
4. Topic 4:
void Test(void) { char *str = (char *) malloc(100); strcpy(str, "hello"); free(str); if(str != NULL) { strcpy(str, "world"); printf(str); } }
After free releases the space, the str pointer is not equal to NULL, but the space can not be used. Therefore, it is generally recommended to set the pointer to NULL after space release.
Flexible array
typedef struct st_type { int i; int a[0];//If the flexible array member reports an error, change it to int a []; }type_a;
For example:
typedef struct st_type { int i; int a[0];//Flexible array member }type_a; printf("%d\n", sizeof(type_a));//The output is 4
Use of flexible arrays
//Code 1 int i = 0; type_a *p = (type_a*)malloc(sizeof(type_a)+100*sizeof(int)); //Business processing p->i = 100; for(i=0; i<100; i++) { p->a[i] = i; } free(p);
Advantages of flexible arrays
The above code can also be written as:
//Code 2 typedef struct st_type { int i; int *p_a; }type_a; type_a *p = malloc(sizeof(type_a)); p->i = 100; p->p_a = (int *)malloc(p->i*sizeof(int)); //Business processing for(i=0; i<100; i++) { p->p_a[i] = i; } //Free up space free(p->p_a); p->p_a = NULL; free(p); p = NULL;