It's time for the interview again to prepare some dry goods for the interview.
provider example
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:dubbo="http://code.alibabatech.com/schema/dubbo" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd "> <!-- use dubbo Protocol Exposure Service at Port 20880 --> <dubbo:protocol name="dubbo" port="20952"/> <!-- Declare service interfaces that need to be exposed --> <dubbo:service interface="com.***.workorder.facade.exports.ApplyTaskFacade" ref="applyTaskFacadeImpl" version="1.0.0" delay="-1"/> <dubbo:service interface="com.***.workorder.facade.exports.TaskFacade" ref="taskFacadeImpl" version="1.0.0" delay="-1"/> </beans>
These are the basic provider configurations
dubbo seamlessly plugged into spring. Let's see how it works.
From the configuration file above, you can see that dubbo uses custom tags, so you must have implemented Namespace Handler Support to parse your tags.
You can find the implementation of Namespace Handler SupportDubbo Namespace Handler
Register the current handler through META-INF/spring.handlers, which is the extension mechanism of spring
Not much to say here.

Here, Dubbo Namespace Handler delegates parsing to DubboBean Definition Parser, but there's not much to say here.
DubboBean Definition Parser can see that dubbo collates the classes used for each custom tag parsing
Label | Analytic class | Effect |
---|---|---|
application | ApplicationConfig | Application configuration is used to configure current application information, whether the application is a provider or a consumer. |
module | ModuleConfig | Module configuration, used to configure current module information, optional. |
registry | RegistryConfig | Registry configuration, which is used to configure information related to connecting registry. |
monitor | MonitorConfig | Monitoring center configuration, used to configure the connection monitoring center related information, optional. |
provider | ProviderConfig | The default value of the provider is optional when a property of ProtocolConfig and ServiceConfig is not configured. |
consumer | ConsumerConfig | The default configuration of the consumer is optional when an attribute of Reference Config is not configured. |
protocol | ProtocolConfig | Protocol configuration, which is used to configure the protocol information for providing services. The protocol is designated by the provider and passively accepted by the consumer. |
service | ServiceBean | Service configuration is used to expose a service and define its meta-information. A service can be exposed by multiple protocols, and a service can also be registered with multiple registries. |
reference | ReferenceBean | Reference configuration to create a remote service proxy that can point to multiple registries. |
annotation | AnnotationBean | Annotation Recognition Processor |
Here the specific tag attribute parsing process is not analyzed for the time being. If you are interested, you can view the original tag by yourself.
DubboBean Definition Parser completes the process of parsing the xml configuration and loading it into the spring container
I don't know much about spring's parsing mechanism, but through DubboBean Definition Parser, we can see that the spring container loads a Bean Definition, which can understand the abstract implementation of objects in spring.
When the provider object is parsed and loaded, the service is naturally generated according to configuration.
Let's look at the implementation of ServiceBean code
public class ServiceBean<T> extends ServiceConfig<T> implements InitializingBean, DisposableBean, ApplicationContextAware, ApplicationListener, BeanNameAware
Service exposure
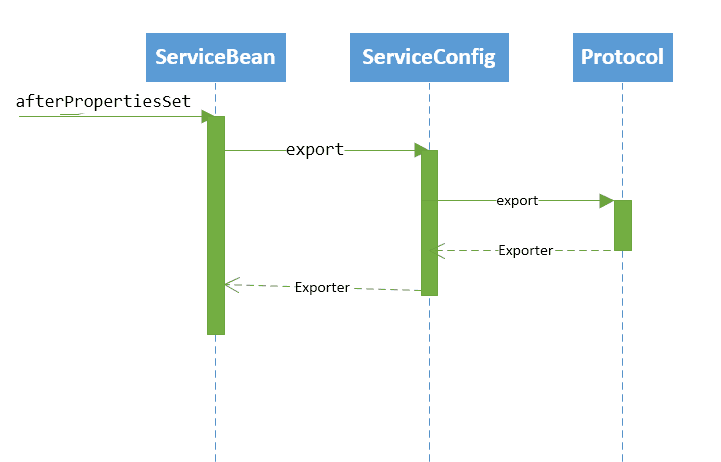
You can see that the export() method that eventually calls to the Protocol object exposes the service.
At this point, service exposures on the process are completed. The details of the exposures are implemented by the protocols themselves.
Service Citation
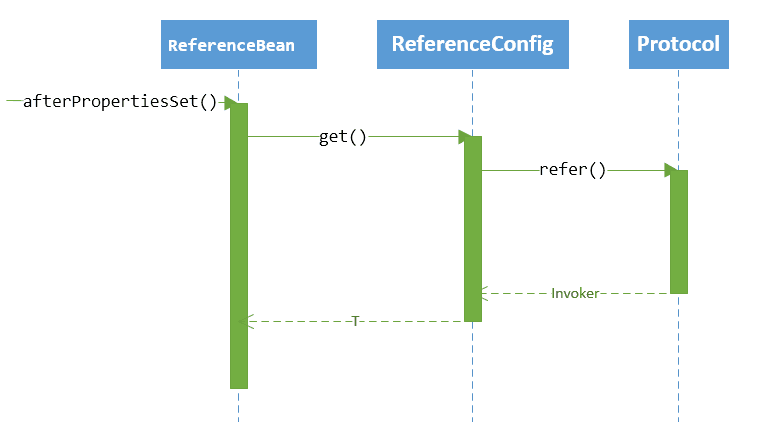
An Invoker object is obtained by refer() of Protocol, and then the proxy object of interface is generated by dynamic proxy to realize rpc communication.
Summary: exporter() and refer() interfaces of Protocol interface are used to complete service exposure and service reference in process.
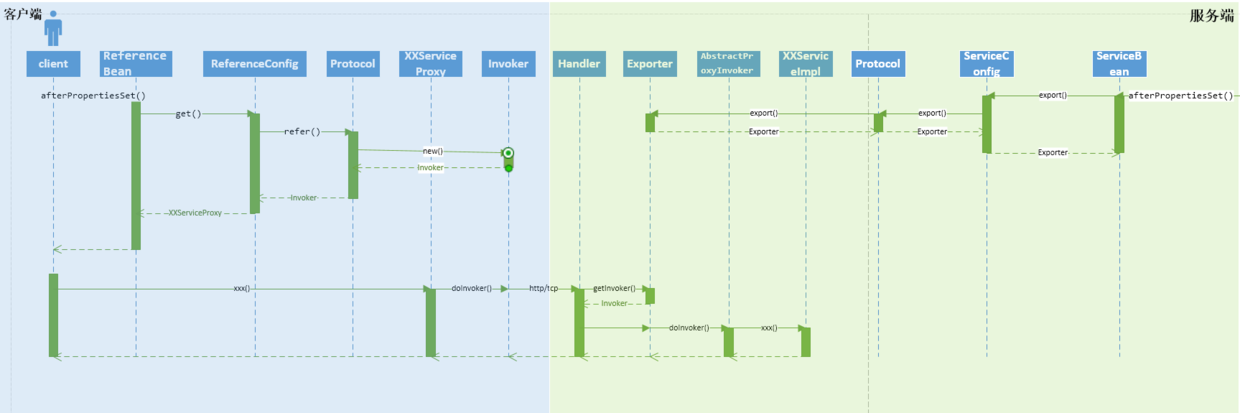
Introduction of dubbo-protocol layer
Protocol interface belongs to Protocol layer in dubbo. There are three main objects in Protocol layer: Exporter, Invoker and Protocol.
- Exporter: Exporter through which Invoker objects can be obtained
- Invoker:(Communication) Actuator to communicate with the remote end
- Protocol: An Abstract protocol that exposes and references services
Protocol layer is the core layer of dubbo. As long as there is Protocol + Invoker + Exporter, opaque RPC calls can be completed.
Look at Protocol,Exporter,Invoker interface definitions
@SPI("dubbo") public interface Protocol { /** * Gets the default port, which is used when the user does not configure the port. * * @return Default port */ int getDefaultPort(); /** * Exposure to remote services: <br> * 1. When receiving a request, the protocol should record the address information of the requester's source: RpcContext. getContext (). setRemoteAddress (); < br > * 2. export()It must be idempotent, that is, the Invoker that exposes the same URL twice is no different from the Invoker that exposes the same URL once. <br> * 3. export()The incoming Invoker is implemented by the framework and passed in. The protocol does not need to be concerned. <br> * * @param <T> Types of services * @param invoker Executives of services * @return exporter Exposure service references for eliminating exposure * @throws RpcException Thrown when exposing service errors, such as port occupancy */ @Adaptive <T> Exporter<T> export(Invoker<T> invoker) throws RpcException; /** * Reference to remote services: <br> * 1. When the user calls the invoke() method of the Invoker object returned by refer(), the protocol needs to execute the invoke() method of the Invoker object passed in with the remote export() of the URL. <br> * 2. refer()The returned Invoker is implemented by a protocol in which remote requests are usually sent. <br> * 3. When check=false is set in the url, the connection failure cannot throw an exception, and the internal recovery is automatic. <br> * * @param <T> Types of services * @param type Types of services * @param url URL address of remote service * @return invoker Local Agent for Services * @throws RpcException Thrown when the connection service provider fails */ @Adaptive <T> Invoker<T> refer(Class<T> type, URL url) throws RpcException; /** * Release agreement: <br> * 1. Cancel all exposed and referenced services of the protocol. <br> * 2. Release all resources occupied by the protocol, such as connections and ports. <br> * 3. After release, the protocol can still expose and refer to new services. <br> */ void destroy(); } public interface Exporter<T> { Invoker<T> getInvoker(); void unexport(); } ** * Invoker. (API/SPI, Prototype, ThreadSafe) * There are three kinds.: * 1.AbstractInvoker Calling remote services over the network (Client side) * 2.AbstractProxyInvoker Call local implementation (Server side) * 3.ClusterInvoker Providing Cluster Services (Client side) */ public interface Invoker<T> extends Node { Class<T> getInterface(); Result invoke(Invocation invocation) throws RpcException; }