DOM, BOM Operational Set
I. definition
Document Object Model (DOM) is a programming interface for HTML and XML documents.
Two, node
Node type
Node type |
describe |
1 |
Element |
Representative elements |
2 |
Attr |
Representative attribute |
3 |
Text |
Represents text content in elements or attributes |
4 |
CDATASection |
Represents the CDATA part of the document (text that will not be parsed by the parser) |
5 |
EntityReference |
Representational entity citation |
6 |
Entity |
Representative entity |
7 |
ProcessingInstruction |
Representation Processing Instruction |
8 |
Comment |
Representative notes |
9 |
Document |
Represents the entire document (the root node of the DOM tree) |
10 |
DocumentType |
Provide interfaces to entities defined for documents |
11 |
DocumentFragment |
Represents a lightweight Document object that can accommodate a part of a document |
12 |
Notation |
Symbols representing declarations in DTD |
Node relationship
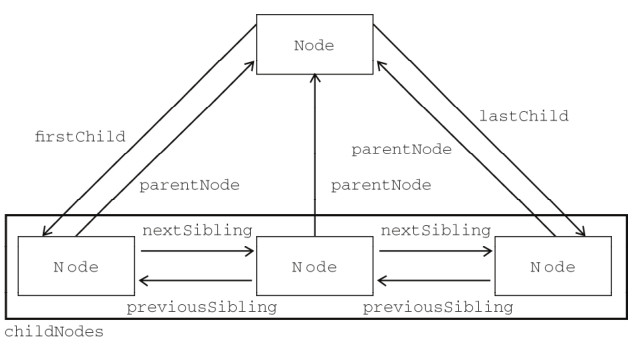
nodeType |
Returns the numeric value of the node type (1-12) |
nodeName |
Element Node: Label Name (Uppercase), Attribute Node: Attribute Name, Text Node: #text, Document Node: #document |
nodeValue |
Text Node: Contains Text, Attribute Node: Contains Attribute, Element Node and Document Node: null |
parentNode |
Parent node |
parentElement |
Parent Node Label Element |
childNodes |
All child nodes |
children |
Layer 1 sub-node |
firstChild |
First byte point, Node object form |
firstElementChild |
The first sublabel element |
lastChild |
Last child node |
lastElementChild |
Last sub-label element |
previousSibling |
Last sibling node |
previousElementSibling |
Last Brother Label Element |
nextSibling |
Next sibling node |
nextElementCount |
Next Brother Label Element |
childElementCount |
Number of elements at the first level (excluding text nodes and comments) |
ownerDocument |
Text nodes pointing to the entire document |
Code example
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<div id="t">
<span></span>
<span id="s">
<a></a>
<h1>nick</h1>
</span>
<p></p>
</div>
<script type="text/javascript">
var tT = document.getElementById("t");
console.log(tT.nodeType,tT.nodeName,tT.nodeValue);
console.log(tT.parentNode);
console.log(tT.childNodes);
console.log(tT.children);
var sT = document.getElementById("s");
console.log(sT.previousSibling);
console.log(sT.previousElementSibling);
console.log(sT.nextSibling);
console.log(sT.nextElementSibling);
console.log(sT.firstChild);
console.log(sT.firstElementChild);
console.log(sT.lastChild);
console.log(sT.lastElementChild);
console.log(tT.childElementCount);
console.log(tT.ownerDocument);
</script>
</body>
</html>
Node Relation Method:
- hasChildNodes() returns true when it contains one or more nodes
- contains() returns true if it is a descendant node
- isSaneNode(), isEqualNode() Input node and reference node return true for the same object
- compareDocumentPostion() Determines the relationships between nodes
numerical value |
relationship |
1 |
The given node is not in the current document |
2 |
The given node precedes the reference node |
4 |
The given node is behind the reference node |
8 |
A given node contains a reference node |
16 |
The given node is contained by the reference node |
Code example
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<div id="t">
<span></span>
<span id="s">
<a></a>
<h1>Nick</h1>
</span>
<p></p>
</div>
<script type="text/javascript">
var tT = document.getElementById("t");
var sT = document.getElementById("s");
console.log(tT.hasChildNodes());
console.log(tT.contains(document.getElementById('s')));
console.log(tT.compareDocumentPosition(document.getElementById('s')));
console.log(tT.isSameNode(document.getElementById('t')));
console.log(tT.isEqualNode(document.getElementById('t')));
console.log(tT.isSameNode(document.getElementById('s')));
</script>
</body>
</html>
3. Selector
selector
getElementById() |
One parameter: the ID of the element tag |
getElementByTagName() |
One parameter: element tag name |
getElementByName() |
One parameter: name attribute name |
getElementsByClassName() |
One parameter: a string containing one or more class names |
classList |
Returns an array of all class names
- Add (add)
- Contains (there is a return true, otherwise false)
- Remove (delete)
- Toggle (delete if it exists, otherwise add)
|
querySelector() |
Accept the CSS selector, return the first element that matches, and null if not |
querySelectorAll() |
Receives the CSS selector, returns an array, and returns [] |
Code example
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<div id="t"></div>
<script>
var tT = document.getElementById('t');
console.log(tT);
</script>
<div id="t">
<div></div>
<div></div>
<div></div>
</div>
<script>
var tT = document.getElementsByTagName('div');
console.log(tT);
console.log(tT[0]);
console.log(tT.length);
</script>
<div name="nick"></div>
<script>
var tT = document.getElementsByName("nick");
console.log(tT);
</script>
<div class="t">
<div></div>
<div></div>
<div></div>
</div>
<script>
var tT = document.getElementsByClassName('t');
console.log(tT);
console.log(tT[0]);
console.log(tT.length);
</script>
<div class="t t2 t3"></div>
<script>
var tT = document.getElementsByTagName('div')[0];
tTList = tT.classList;
console.log(tT);
console.log(tTList);
tTList.add("t5");
console.log(tTList.contains("t5"));
tTList.remove("t5");
console.log(tTList.contains("t5"));
tTList.toggle("t5");
console.log(tTList.contains("t5"));
</script>
<div class="t t2 t3"></div>
<div class="t" id="t"></div>
<div name="nick"></div>
<script>
var tT = document.querySelector("div");
console.log(tT);
var tI = document.querySelector("#t");
console.log(tI);
var tC = document.querySelector(".t");
console.log(tC);
var tN = document.querySelector("[name]");
console.log(tN);
</script>
<div class="t t2 t3"></div>
<div class="t" id="t"></div>
<div name="nick"></div>
<script>
var tT = document.querySelectorAll("div");
console.log(tT);
var tI = document.querySelectorAll("#t");
console.log(tI);
var tC = document.querySelectorAll(".t");
console.log(tC);
var tN = document.querySelectorAll("[name]");
console.log(tN);
</script>
</body>
</html>
Four, style
Style operation method style
style.cssText |
You can read and write code in style |
style.item() |
Returns the name of the CSS attribute for a given location |
style.length |
Number of parameters in style block |
style.getPropertyValue() |
Returns the string value of a given attribute |
style.getPropertyPriority() |
Check whether a given property is set! Important, set to return "important"; otherwise return an empty string |
style.removeProperty() |
Delete specified properties |
style.setProperty() |
Setting attributes can take three parameters: setting attribute name, setting attribute value, and setting "important". |
Code example
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<div id="t" style="background-color: yellow; width: 100px; height: 100px">8</div>
<script>
var tT = document.getElementById("t");
console.log(tT.style.cssText);
tT.style.cssText = "background-color: yellow; width: 200px; height: 200px";
console.log(tT.style.cssText);
console.log(tT.style.item("0"));
console.log(tT.style.length);
console.log(tT.style.getPropertyValue("background-color"));
console.log(tT.style.getPropertyPriority("background-color"));
console.log(tT.style.removeProperty("width"));
tT.style.setProperty("width","200px","");
</script>
</body>
</html>
V. Table Operation
Form operation method
createTHead() |
Create thead elements and return references |
deleteTHead() |
Delete the thead element |
createTBody() |
Create the tbody element and return the reference |
inseRow(0) |
Insert the tr element, starting at 0 |
deleteRow(pos) |
Delete rows at specified locations |
insertCell(0) |
Insert the td element, starting at 0 |
deleteCell(pos) |
Delete cells at specified locations |
Code example
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<script>
var table = document.createElement("table")
table.border = "1px"
table.width = "150px"
var theadt = table.createTHead()
var tbody = table.createTBody()
var trH0 = theadt.insertRow(0)
trH0.insertCell(0).appendChild(document.createTextNode("Full name"))
trH0.insertCell(1).appendChild(document.createTextNode("Age"))
var trB0 = tbody.insertRow(0)
var trB1 = tbody.insertRow(1)
trB0.insertCell(0).appendChild(document.createTextNode("nick"))
trB0.insertCell(1).appendChild(document.createTextNode("18"))
trB1.insertCell(0).appendChild(document.createTextNode("jenny"))
trB1.insertCell(1).appendChild(document.createTextNode("21"))
trB0.deleteCell(1)
console.log(table)
document.body.appendChild(table)
</script>
</body>
</html>
Form operation
Form operation mode
document.forms |
Get all forms |
.submit() |
Submit Form |
Code example
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="https://www.baidu.com/s" method="get">
<input type="text" name="wd" />
<input type="button" value="Use Baidu Search" onclick="this.disable=true;BaiDu(this);" />
</form>
<script>
var form = document.forms;
var formOne = form[0];
console.log(form);
console.log(formOne);
function BaiDu(ths) {
var inputBaiDu = ths;
inputBaiDu.parentNode.submit();
}
</script>
</body>
</html>
Element Node ELEMENT
nodeType:1
nodeName |
Label name of access element |
tagName |
Label name of access element |
createElement() |
Create node |
appendChild() |
Add the node at the end and return the new node |
insertBefore() |
Insert a node before the reference node, with two parameters: the node to be inserted and the participating node |
insertAfter() |
The reference node is inserted after the reference node, with two parameters: the node to be inserted and the reference node. |
replaceChild() |
Replacement node, two parameters: the node to be inserted and the node to be replaced (removed) |
removeChild() |
Remove node |
cloneNode() |
Cloning, a Boolean parameter, true for deep copy, false for shallow copy |
importNode() |
Copy a node from the document with two parameters: the node to be copied and the Boolean value (whether or not to copy the child node) |
insertAdjacentHTML() |
Insert text, two parameters: the location of insertion and the text to be inserted
- "Before begin", insert before this element
- "afterbegin" is inserted before the first child element of the element
- "beforeend" is inserted after the last child element of the element
- "afterend" is inserted after the element
|
Code example:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<div id="t">
<span id="one"></span>
<span id="s">
<a></a>
<h1>Nick</h1>
</span>
<p></p>
</div>
<script>
var tT = document.getElementById("t");
var a1New = document.createElement('a');
tT.appendChild(a1New);
console.log(tT.lastElementChild);
var a2New = document.createElement('a');
a2New.className = 't';
a2New.id = 'oneNew';
a2New.innerText = 'jenny';
tT.insertBefore(a2New,document.getElementById('one'));
console.log(tT.firstElementChild);
var a3New = document.createElement('h3');
tT.replaceChild(a3New,document.getElementById('oneNew'));
console.log(tT.firstElementChild);
tT.removeChild(tT.firstElementChild);
console.log(tT.firstElementChild);
var clNo = tT.cloneNode(true);
console.log(clNo);
var imNoT = document.importNode(tT,true);
console.log(imNoT.firstChild);
var imNoF = document.importNode(tT,false);
console.log(imNoF.firstChild);
tT.insertAdjacentText("beforebegin","beforebegin");
tT.insertAdjacentText("afterbegin","afterbegin");
tT.insertAdjacentText("beforeend","beforeend");
tT.insertAdjacentText("afterend","afterend");
</script>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<div id="t" class="tClass" title="tTitle" lang="en" dir="ltr"></div>
<script>
var tN = document.getElementById("t");
console.log(tN.nodeType);
console.log(tN.tagName);
console.log(tN.nodeName);
console.log(tN.id);
console.log(tN.title);
console.log(tN.lang);
console.log(tN.dir);
console.log(tN.className);
var dirNew = document.createElement("div");
dirNew.className = "tC";
dirNew.innerText = "Nick";
console.log(dirNew);
</script>
</body>
</html>
attributes of attribute nodes
nodeType:2
attributes |
Get all tag attributes |
getAttribute() |
Gets the specified label attribute |
setAttribute() |
Setting the specified label properties |
removeAttribute() |
Remove specified label attributes |
var s=document.createAttribute("age")
s.nodeValue="18" |
Create age attributes
Set the property value to 18 |
Code example
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<div id="t" class="s1 s2" name="nick"></div>
<script>
var tT = document.getElementById("t");
console.log(tT.attributes);
console.log(tT.attributes.id);
console.log(tT.attributes.class);
console.log(tT.attributes.getNamedItem("name"));
console.log(tT.attributes.removeNamedItem("class"));
console.log(tT.attributes.getNamedItem("class"));
var s = document.createAttribute("age");
s.nodeValue = "18";
console.log(tT.attributes.setNamedItem(s));
console.log(tT.attributes);
console.log(tT.attributes.item("1"));
</script>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<div id="t" class="s1 s2" name="nick"></div>
<script>
var tT = document.getElementById("t");
console.log(tT.attributes);
console.log(tT.attributes.id);
console.log(tT.attributes.class);
console.log(tT.getAttribute("name"));
tT.setAttribute("age",18);
console.log(tT.getAttribute("age"));
tT.removeAttribute("age");
console.log(tT.getAttribute("age"));
</script>
</body>
</html>
Text Node
nodeType:3
innerText |
All plain text content, including text in subtags |
outerText |
Similar to innerText |
innerHTML |
All child nodes (including elements, annotations, and text nodes) |
outerHTML |
Returns its own node and all its children |
textContent |
Similar to innerText, the returned content is styled |
data |
Text content |
length |
Text length |
createTextNode() |
Create text |
normalize() |
Delete blanks between text fields |
splitText() |
Division |
appendData() |
Append |
deleteData(offset,count) |
Delete count characters from the location specified by offset |
insertData(offset,text) |
Insert text at the location specified by offset |
replaceData(offset,count,text) |
Replacement. Text replaces text from offset to count |
substringData(offset,count) |
Extract text from offset to count |
Code example:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<div id="t">Nick</div>
<script>
var tT = document.getElementById("t");
var tTOne = tT.firstChild;
console.log(tTOne.nodeType);
console.log(tTOne.data);
console.log(tTOne.length);
var tTNew = document.createTextNode("18");
console.log(tTNew.nodeType,tTNew.data);
tTOne.appendData("18");
console.log(tTOne.data);
tTOne.deleteData(4,2);
console.log(tTOne.data);
tTOne.insertData(0,"jenny");
console.log(tTOne.data);
tTOne.replaceData(0,5,"18");
console.log(tTOne.data);
var tTSub = tTOne.substringData(2,6);
console.log(tTSub);
</script>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<div id="t">
<div>1</div>
<div>2</div>
<div>3</div>
</div>
<script>
var tT = document.getElementById("t");
console.log(tT.innerText);
console.log(tT.outerText);
console.log(tT.innerHTML);
console.log(tT.outerHTML);
console.log(tT.textContent);
</script>
</body>
</html>
Document Node
nodeType: 4
document.documentElement |
Represents html elements in a page |
document.body |
Represents the body element in the page |
document.doctype |
Representative! DOCTYPE tag |
document.head |
Represents the head element in the page |
document.title |
Text representing title elements, modifiable |
document.URL |
The URL address of the current page |
document.domain |
The domain name of the current page |
document.chartset |
Character set used in the current page |
document.defaultView |
Returns the window object associated with the current document object without returning null |
document.anchors |
All a elements with name attributes in the document |
document.links |
All a elements with href attributes in the document |
document.forms |
All form elements in the document |
document.images |
All img elements in the document |
document.readyState |
Two values: load (loading document), complete (already loaded document) |
document.compatMode |
Two values: BackCompat: Standard Compatibility Mode turned off, CSS1Compat: Standard Compatibility Mode turned on |
write()/writeln() |
write() text output to the screen, writeln() output followed by line breaks |
open()/close() |
open() empties the content and opens a new document, close() closes the current document, and next time writes a new document |
Position operation method
document.documentElement.offsetHeight |
Total Document Height |
document.documentElement.clientHeight |
Documents occupy the current screen height |
document.documentElement.clientWidth |
Documents occupy the current screen width |
offsetHeight |
Height + padding + border |
scrollHeight |
Document height (height+padding) |
offsetTop |
Magin |
clientTop |
border Height |
offsetParent |
Parent Location Labels, Elements |
scrollTop |
Rolling height |
Code example
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
* {
margin: 0;
padding: 0;
}
.zg {
height: 1000px;
position: relative;
border: 3px solid transparent;
}
.dg {
height: 500px;
padding-top: 10px;
margin-top: 20px;
border: 2px solid transparent;
}
</style>
</head>
<body onscroll="Scroll()">
<div id="zg" class="zg">
<div id="dg" class="dg">
</div>
</div>
<script>
var zg = document.documentElement.offsetHeight;
console.log(zg);
var dg = document.documentElement.clientHeight;
console.log(dg);
var dgBox = document.getElementById("dg");
console.log(dgBox.offsetHeight);
console.log(dgBox.scrollHeight);
console.log(dgBox.offsetTop);
console.log(dgBox.clientTop);
console.log(dgBox.offsetParent);
function Scroll() {
console.log(document.body.scrollTop);
}
</script>
</body>
</html>
12. Timer
setInterval |
Multiple Timers (Millisecond Timing)> |
clearInterval |
Clear multiple timers |
setTimeout |
One-shot Timer |
clearTimeout |
Clear single timer |
Code example
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<input type="button" value="Interval" onclick="Interval();" />
<input type="button" value="StopInterval" onclick="StopInterval();" />
<script>
function Interval() {
s1 = setInterval(function () {
console.log(123);
}, 1000);
s2 = setInterval(function () {
console.log(456);
}, 2000);
console.log(1);
}
function StopInterval() {
clearInterval(s1);
clearInterval(s2);
}
</script>
</body>
</html>
13. Pop-up Box
alert() |
Popup |
confirm() |
Confirmation box - Return value: true, false |
prompt() |
Input box - Two parameters: the text of the prompt and the default value of the input, and the return value: the input value, "", null |
prompt
var result = prompt("What is your name?" ,"Nick");
if(result != null){
alert("welcome,"+result);
}
console.log(result)
14. location
location.href |
Get URL |
location.href="URL" |
redirect |
location.assign("http://www.baidu.com") |
Redirect to URL |
location.search="wd=hundan" |
Modify the Query String (Baidu Search) |
location.hostname |
Service hostname, example: www.baidu.com |
location.pathname |
Path, example: baidu |
location.port |
Port number |
location.reload |
Reload |
XV. Others
navigator |
Contains information about browsers |
screen |
Contains information about the client display screen |
history |
Contains URL s accessed by users (in browser windows) |
window.print() |
Display Print Dialog Box |
Code example:
history.go(-1)
history.go(1);
history.go(2);
history.go()
history.back()
history.forward()
Event Operation
attribute |
When did this happen? . |
onabort |
The loading of the image was interrupted. |
onblur |
Elements lose focus |
onchange |
The content of the domain is changed |
onclick |
Event handle invoked when a user clicks on an object |
ondblclick |
Event handle invoked when a user double-clicks an object |
onerror |
Error loading document or image |
onfocus |
Elements get focus |
onkeydown |
A keyboard key is pressed |
onkeypress |
A keyboard key is pressed and released |
onkeyup |
A keyboard key is released |
onload |
Complete loading of a page or image |
onmousemove |
Mouse is moved |
onmousedown |
The mouse button is pressed |
onmouseout |
Mouse moves away from an element |
onmouseover |
Mouse over an element |
onmouseup |
The mouse button is released |
onreset |
Reset button clicked |
onresize |
The window or frame is resized |
onselect |
Text is selected |
onsubmit |
Confirm that the button is clicked |
onunload |
User Exit Page |