IDEA is a powerful tool for java development, springboot is the most popular micro service framework in the Java ecosystem, and docker is the hottest container technology at present. What chemical reaction will they produce together?
1, Preparation before development
1.Docker installation
Refer to:
https://docs.docker.com/install/
2. Configure docker remote connection port
vi /usr/lib/systemd/system/docker.service
Find ExecStart and add - H on the last side tcp://0.0.0.0:2375 , as shown in the figure below
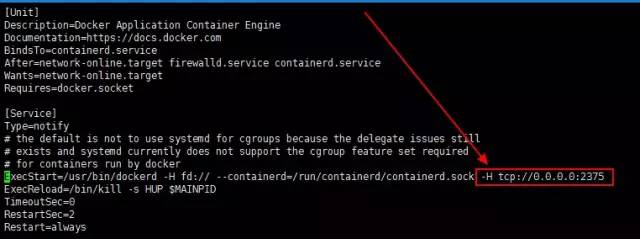
picture
3. Restart docker
systemctl daemon-reload systemctl start docker
4. Open port
firewall-cmd --zone=public --add-port=2375/tcp --permanent
5. Install the plug-in for idea and restart it

picture
6. Connect to remote docker
1. Edit configuration
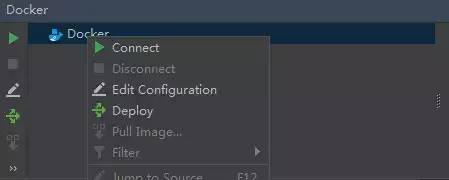
picture
2. Fill in the remote docker address

3. If the connection is successful, the remote docker container and image will be listed
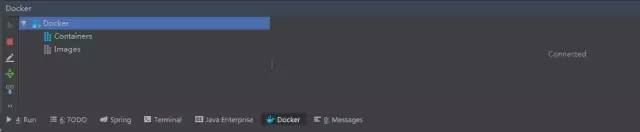
2, New project
1. Create a springboot project
Project structure diagram

picture
1. Configuring pom files
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>docker-demo</groupId> <artifactId>com.demo</artifactId> <version>1.0-SNAPSHOT</version> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.0.2.RELEASE</version> <relativePath /> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <docker.image.prefix>com.demo</docker.image.prefix> <java.version>1.8</java.version> </properties> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> <plugin> <groupId>com.spotify</groupId> <artifactId>docker-maven-plugin</artifactId> <version>1.0.0</version> <configuration> <dockerDirectory>src/main/docker</dockerDirectory> <resources> <resource> <targetPath>/</targetPath> <directory>${project.build.directory}</directory> <include>${project.build.finalName}.jar</include> </resource> </resources> </configuration> </plugin> <plugin> <artifactId>maven-antrun-plugin</artifactId> <executions> <execution> <phase>package</phase> <configuration> <tasks> <copy todir="src/main/docker" file="target/${project.artifactId}-${project.version}.${project.packaging}"></copy> </tasks> </configuration> <goals> <goal>run</goal> </goals> </execution> </executions> </plugin> </plugins> </build> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>log4j</groupId> <artifactId>log4j</artifactId> <version>1.2.17</version> </dependency> </dependencies> </project>
2. Create a docker directory under src/main directory and a Dockerfile file
FROM openjdk:8-jdk-alpine ADD *.jar app.jar ENTRYPOINT ["java","-Djava.security.egd=file:/dev/./urandom","-jar","/app.jar"]
3. Create the application.properties file in the resource directory
logging.config=classpath:logback.xml logging.path=/home/developer/app/logs/ server.port=8990
4. Create DockerApplication file
@SpringBootApplication public class DockerApplication { public static void main(String[] args) { SpringApplication.run(DockerApplication.class, args); } }
5. Create DockerController file
@RestController public class DockerController { static Log log = LogFactory.getLog(DockerController.class); @RequestMapping("/") public String index() { log.info("Hello Docker!"); return "Hello Docker!"; } }
6. Add configuration
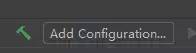


Command interpretation:
- Image tag: specify the image name and tag. The image name is docker demo and the tag is 1.1
- Bind ports: bind the host port to the container internal port. Format is [host port]: [container internal port]
- Bind mounts: hang the host directory into the internal directory of the container.
The format is [Host Directory]: [container internal directory]. The springboot project will print the log in the container / home/developer/app/logs / directory. After the host directory is mounted to the internal directory of the container, the log will be persisted in the host directory outside the container.
7. Maven pack

8. Run


First pull the basic image, then package the image, and deploy the image to the remote docker for operation

picture
Here we can see that the image name is docker Demo: 1.1 and the docker container is docker server
9. Run successfully

10. Browser access
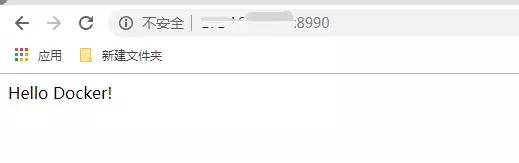
picture
11. Log view
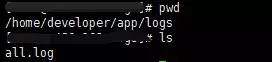
picture
Since then, the springboot project has been successfully deployed to docker through IDEA! It's hard to imagine how easy it is to deploy a Java Web project!
Thanks for reading, hope to help you:) Source: juejin.im/post/5d026212f265da1b8608828b
- EOF -