Ways of Verification of Interface Structures
Checking in Pure Coding Form
Generally, the conventional interface return value verification requires assertion judgment of the fields that need to be checked one by one, which has a great coding workload and is obviously not suitable for the interface testing platform. Let's look at an example:
import requests #Query Conference Interface url = "http://127.0.0.1:8000/api/get_event_list/" r = requests.get(url, params={'eid':'1'}) result = r.json() print(result) assert result['status'] == 200 assert result['message'] == "success" assert result['data']['name'] == "Hammer Mobile Press Conference" assert result['data']['address'] == "Chengdu" assert result['data']['start_time'] == "2017-11-21T15:25:19"
If there are five key values in the return value of an interface, five assertions need to be written.
The way JSON schema works
So this time we intend to use JSON schema to verify the interface structure.
JSON schema describes your JSON data format; JSON schema (application / schema + JSON) has many uses, one of which is instance validation. The validation process can be interactive or non-interactive. For example, applications can use JSON patterns to build user interfaces to generate interactive content in addition to user input checking or validating data obtained from various sources.
Json Schema Quick Start https://blog.csdn.net/silence_xiao/article/details/81303935
Please refer to relevant documents for specific rules.
JsonSchema is equivalent to a contract test that stipulates a constraint that passes if it meets the requirements and fails if it does not.
Contract test https://www.jianshu.com/p/ec40734c872a
Specific advantages, please feel for yourself, after all, who asserts who knows.
Automatic Generation of JsonSchema
Although our requirement should be that people using the platform pass in a self-written Json Schema, and then we compare the results. However, such a high cost of learning is not very realistic. Therefore, the achievement of this contract needs some assistance.
The idea of the design is that the user passes in a return value of the interface that he thinks is correct, the platform makes a preliminary classification judgment and asks if it is necessary to increase the constraint of each key value. For example, if an object whose type is number needs to be checked to increase the maximum value and the minimum value.
Existing JSON schema converters: https://jsonschema.net/#/
However, without finding the source code, we can only implement one by ourselves.
The first is format checking.
def to_jsonschema(self, json_data, result): ''' //Recursive generation of JSON schema ''' if isinstance(json_data, dict): is_null = True result.append('{') result.append("'type': 'object',") result.append("'additionalProperties': 'false',") # No other attributes are allowed to be added. result.append("'required':[],") # Necessary attributes, leave blank first result.append("'properties': {") for k, v in json_data.items(): is_null = False result.append("'%s':" % k) self.to_jsonschema(v, result) result.append(',') if not is_null: result.pop() result.append('}') result.append('}') elif isinstance(json_data, list): result.append('{') result.append("'type': 'array',") result.append("'items': ") self.to_jsonschema(json_data[0], result) result.append('}') elif isinstance(json_data, float): result.append("{") result.append("'type': 'number'") result.append('}') elif isinstance(json_data, int): result.append("{") result.append("'type': 'integer'") result.append('}') elif isinstance(json_data, str): result.append("{") if json_data.upper() in ("TRUE", "FALSE"): result.append("'type': 'boolean'") else: result.append("'type': 'string'") result.append('}') return "".join(result)
Covered: object,array,number,boolean,string
Complete required and add restrictions below
def complement_required(self, jsonschema_dict): """ //Complete required attributes """ if isinstance(jsonschema_dict, dict): for item, value in jsonschema_dict.items(): if value == 'object': properties = jsonschema_dict.get("properties") if isinstance(properties, dict): for i, j in properties.items(): if j.get("type") in ("integer", "number", "string"): self.complement_limit(i, j) jsonschema_dict['required'].append(i) if isinstance(j, dict) and j.get('type') == "object": self.complement_required(j) elif isinstance(jsonschema_dict, list): for i in jsonschema_dict: self.complement_required(i) def complement_limit(self, name, limit_dict): for i in self.limit: if name == i[0]: limit_type = i[1] if limit_type == 'integer' or limit_type == 'number': if i[2]: limit_dict["maximum"] = i[2] if i[3]: limit_dict["minimum"] = i[3] if i[4]: limit_dict["enum"] = i[4] if i[5]: limit_dict["multipleOf"] = i[5] elif limit_type == 'string': if i[2]: limit_dict["maxLength"] = i[2] if i[3]: limit_dict["minLength"] = i[3] if i[4]: limit_dict["enum"] = i[4] if i[5]: limit_dict["pattern"] = i[5]
Conduct simple tests:
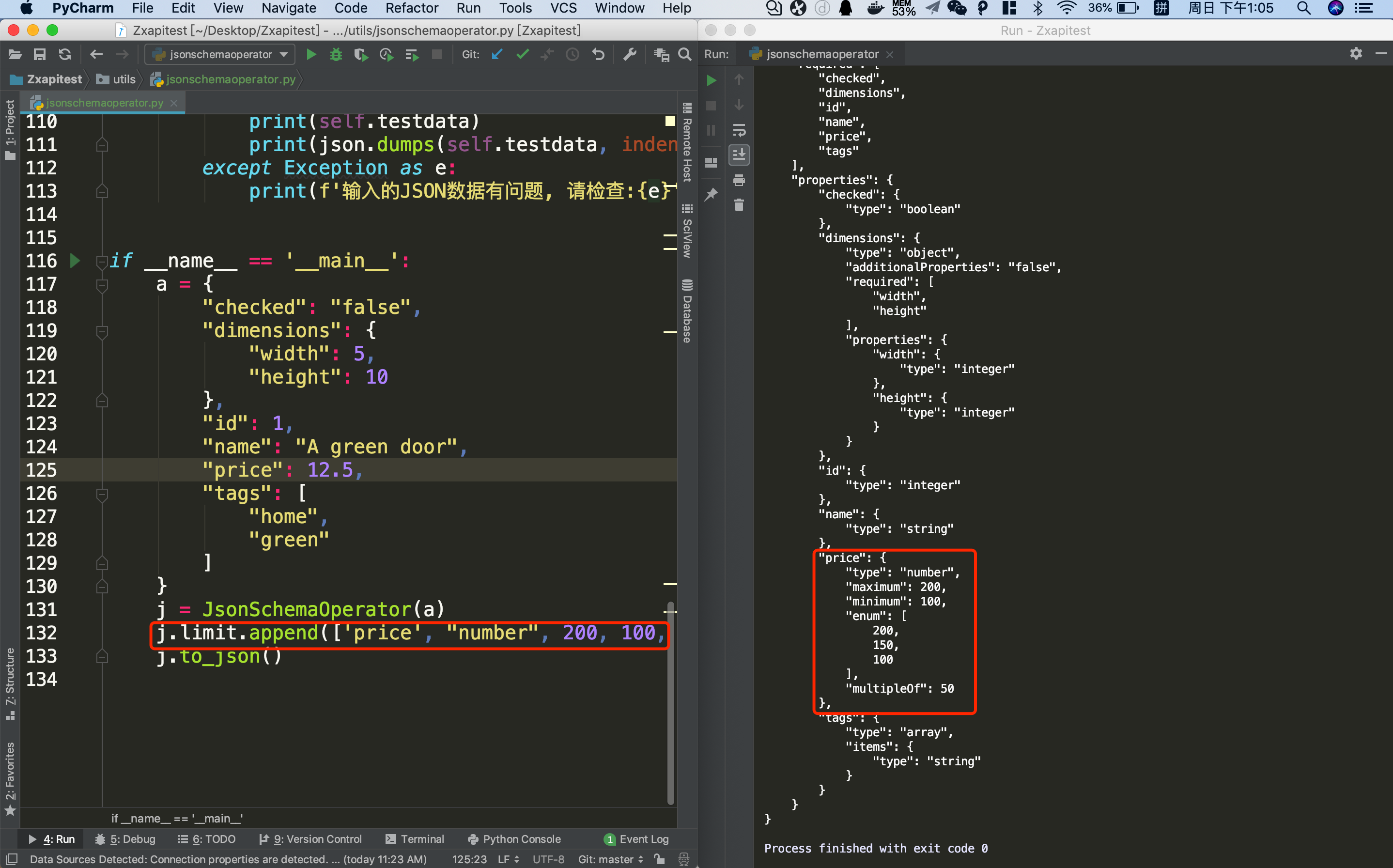
You can see that it basically meets the requirements, and the following pages/interfaces can be automatically generated by passing in the correct things.
There are still some shortcomings in the restrictions on array, which will be supplemented later.
See the code:
https://github.com/zx490336534/Zxapitest/blob/master/utils/jsonschemaoperator.py
PS: Welcome to my public number:
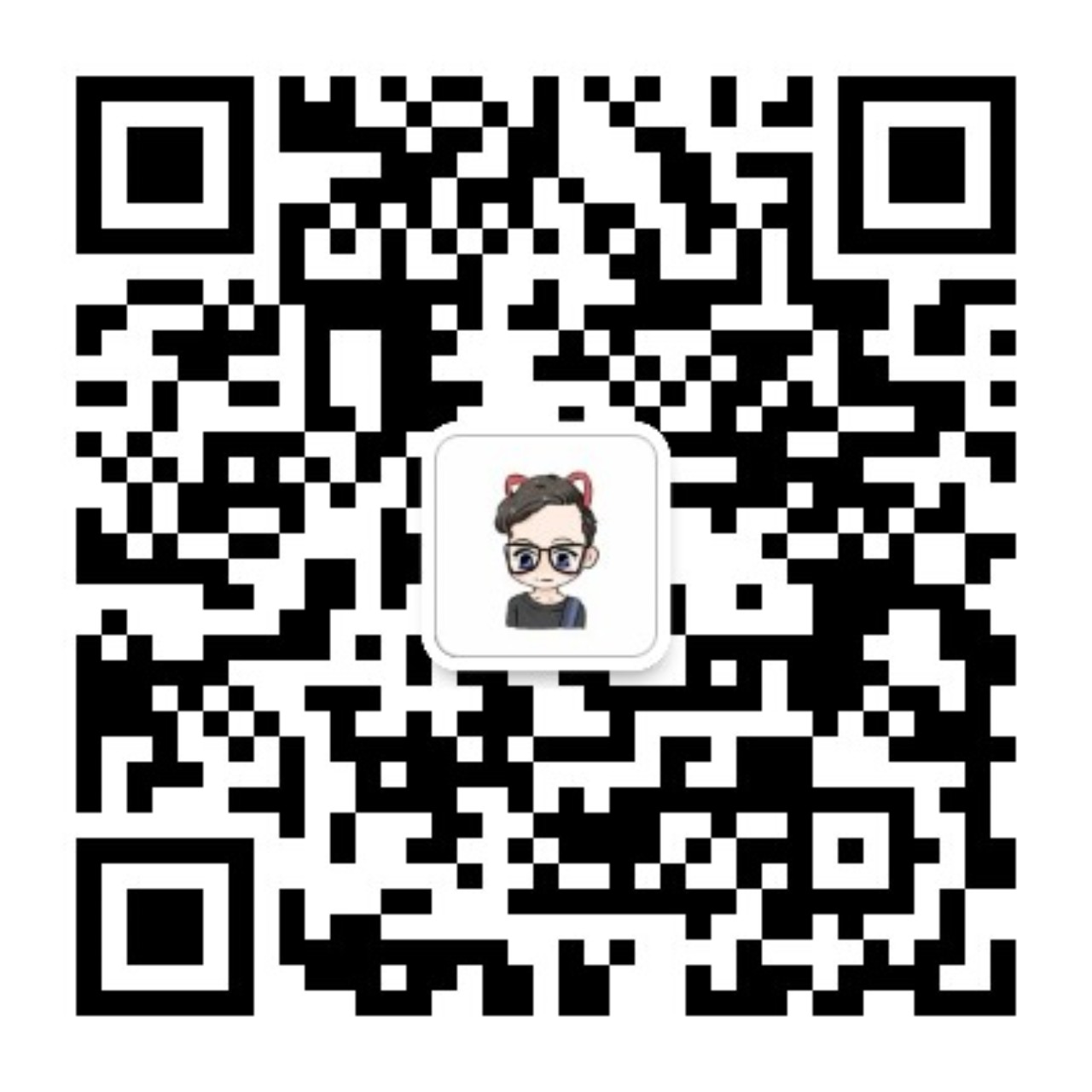