Environmental Science
Win10 Python3.6.6 Django2.1.3
Middleware role
- Middleware is used to globally modify the input or output of Django.
Common uses of Middleware
- cache
- Session authentication
- Log record
- abnormal
Middleware execution process
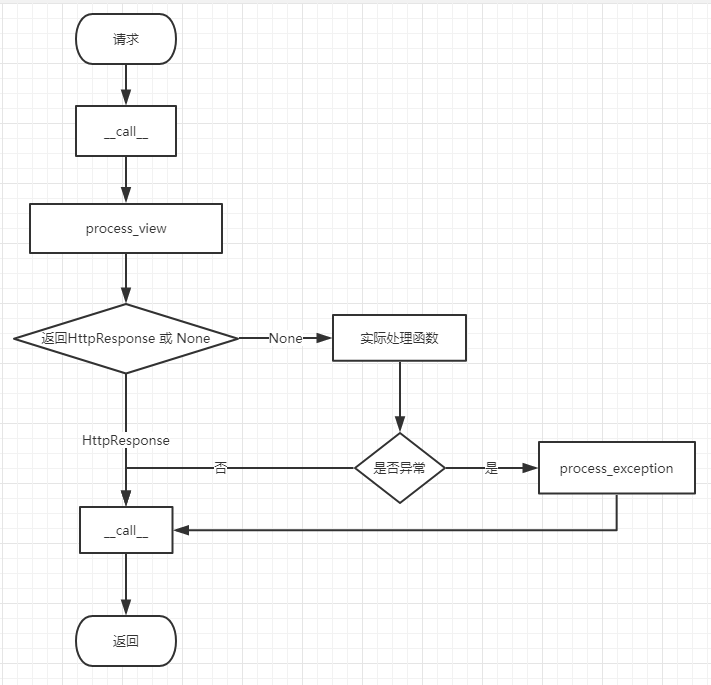
image
Global exception capture implementation
Create django project & add app
django-admin startproject middleware cd middleware django-admin startapp app
Add app to project
# middleware/settings.py # Installed? Applast add app INSTALLED_APPS = [ 'app', ]
Edit middleware and add to project
- Note: middleware registration access has certain relevance, and the location cannot be placed at will
# Create app/middleware.py and edit from django.http import JsonResponse class CustomMiddleware: def __init__(self, get_response): print("Execute on program start, Only once") self.get_response = get_response def __call__(self, request): print("Middleware start") response = self.get_response(request) print("End of Middleware") return response def process_view(self, request, view_func, view_args, view_kwargs): print("Execute before requesting actual function") def process_exception(self, request, exception): print("Execute when the program is abnormal") return JsonResponse({"msg": exception.args[0], "code": -1}) //Edit middleware.settings.py MIDDLEWARE = [ ... 'app.middleware.CustomMiddleware' ]
Write an exception
# app/views.py from django.http import JsonResponse def json_response(request): print('json_response') err = 3 / 0 return JsonResponse({"msg": "ok", "code": 0})
Add to route
# middleware/urls.py from app.views import json_response, view_response urlpatterns = [ ... path("view", view_response) ]
- Operation test
- Visit: http://127.0.0.1:8000/json/
-
Result
image
Another sleep use log
# Add in the middleware function process view print("path: {}; method: {}; data: {}".format(request.get_full_path(), request.method, request.body or ''))