@Tinbur Internet of things
Display function of digital tube with Arduino
1. Code to realize the function
1.1 digital tube display scrolling custom string
#include <SevenSegmentTM1637.h> SevenSegmentTM1637 display(5,4); void setup(){ display.begin(); } void loop(){ display.setBacklight(50); display.print("123456"); }
1.2 time of digital display customization
#include <SevenSegmentExtended.h> #include <SevenSegmentTM1637.h> SevenSegmentExtended display(5,4); void setup(){ display.begin(); display.setBacklight(20); } void loop(){ display.printTime(12,30,HIGH); }
1.3 digital tube display stopwatch
#include <EEPROM.h> #include <SevenSegmentTM1637.h> #include <avr/pgmspace.h> #include <TimerOne.h> SevenSegmentTM1637 display(5,4); int8_t TimeDisp[] = {0x00,0x00,0x00,0x00}; unsigned char ClockPoint = 1; unsigned char Update; unsigned char microsecond_10 = 0; unsigned char second; unsigned char _microsecond_10 = 0; unsigned char _second; unsigned int eepromaddr; boolean Flag_ReadTime; #define ON 1 #define OFF 0 void TimeUpdate2(void) { if(ClockPoint)tm1637.point(POINT_ON); else tm1637.point(POINT_OFF); TimeDisp[2] = _microsecond_10 / 10; TimeDisp[3] = _microsecond_10 % 10; TimeDisp[0] = _second / 10; TimeDisp[1]= _second % 10; Update = OFF; } void TimingISR2() { microsecond_10 ++; Update = ON; if(microsecond_10 == 100) { second ++; if(second == 60) { second = 0; } microsecond_10 = 0; } ClockPoint =(~ClockPoint) & 0x01; if(Flag_ReadTime == 0) {_microsecond_10 = microsecond_10; _second = second; } } void readTime(){ Flag_ReadTime = 1; if(eepromaddr == 0) { Serial.println("The time had been read"); _microsecond_10 = 0; _second = 0; } else{ _second = EEPROM.read(-- eepromaddr); _microsecond_10 = EEPROM.read(-- eepromaddr); Serial.println("List the time"); } Update = ON; } void saveTime() {EEPROM.write(eepromaddr ++,microsecond_10); EEPROM.write(eepromaddr ++,second); } void stopwatchPause() { TCCR1B &= ~(_BV(CS10) | _BV(CS11) | _BV(CS12)); } void stopwatchReset() { stopwatchPause(); Flag_ReadTime = 0; _microsecond_10 = 0; _second = 0; microsecond_10 = 0; second = 0; Update = ON; } void stopwatchStart() { Flag_ReadTime = 0; TCCR1B |=Timer1.clockSelectBits; } void setup(){ display.begin(); display.setBacklight(20); tm1637.set(); tm1637.init(); Timer1.initialize(10000); Timer1.attachInterrupt(TimingISR2); } void loop(){ stopwatchStart(); if(Update == ON) { TimeUpdate2(); tm1637.display(TimeDisp); } }
2. The following screenshot using visual programming
2.1 digital tube rolling string
2.2 digital tube display custom time
2.3 function of digital display stopwatch
3. Effect achieved
3.1 rolling character video
Digital tube realizes rolling string
3.2 time to implement customization
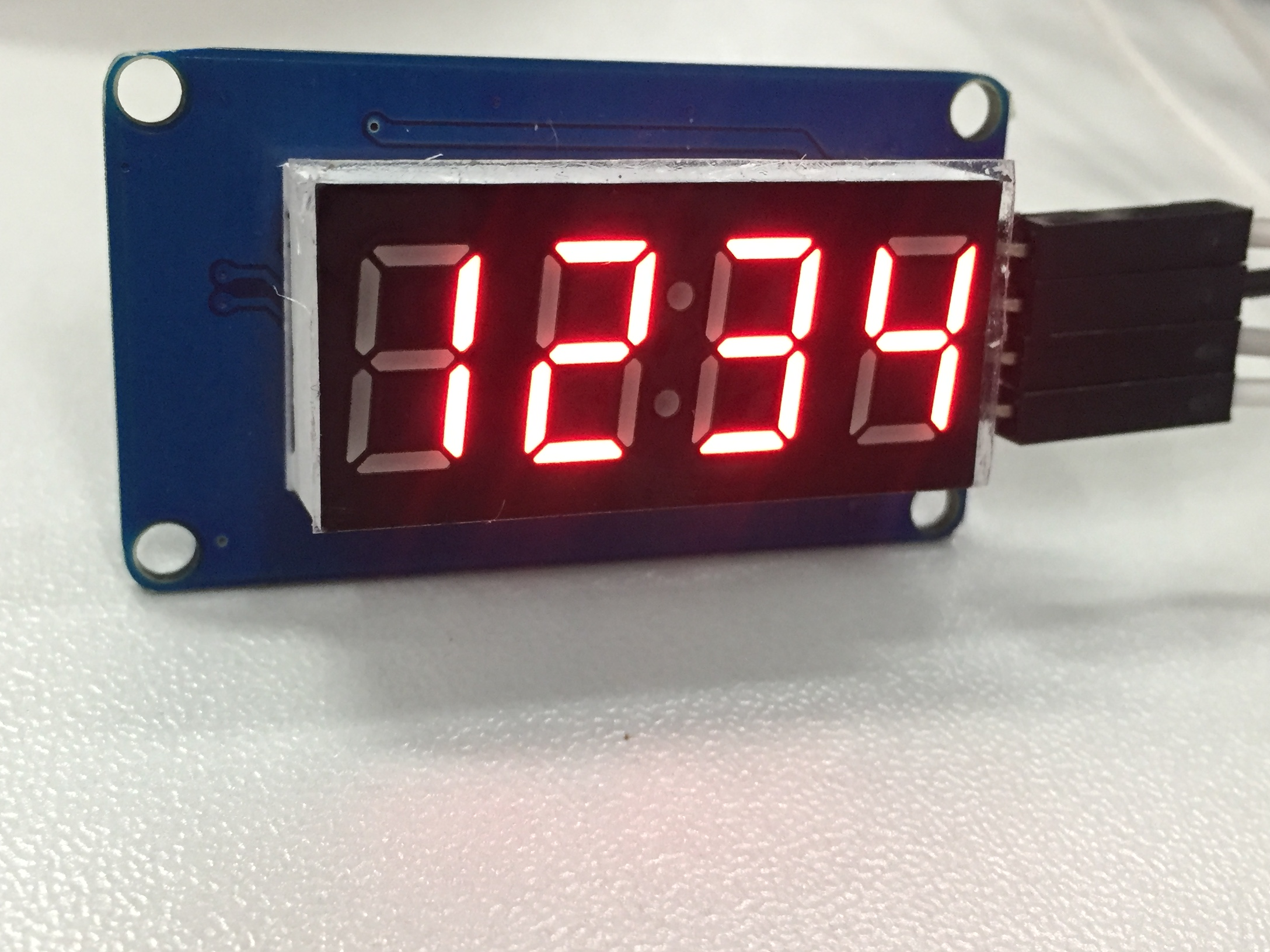
3.3 realization of stopwatch video
Realize stopwatch of digital tube