Python Advancement (II) - First Understanding of Python Data Elements: Dictionary & Time
3 dictionary
3.1 Dictionary Introduction
Dictionary is the most flexible built-in data structure type in python besides list. A list is an ordered combination of objects, and a dictionary is an unordered collection of objects. The difference between the two is that the elements in a dictionary are accessed by keys, not by offsets.
A dictionary consists of keys and corresponding values. Dictionaries are also called associative arrays or hash tables. The basic grammar is as follows:
dict = {'Alice': '2341', 'Beth': '9102', 'Cecil': '3258'};
_can also create dictionaries in this way:
dict1 = { 'abc': 456 };
dict2 = { 'abc': 123, 98.6: 37 };
Each key and value must be separated by a colon (:). Each pair is separated by a comma and placed in braces ({}). Keys must be unique, but values need not be; values can take any data type, but must be immutable, such as strings, numbers or tuples.
3.2 Access the values in the dictionary
dict = {'name': 'Zara', 'age': 7, 'class': 'First'};
print "dict['name']: ", dict['name'];
print "dict['age']: ", dict['age'];
3.3 Revising Dictionaries
_The way to add new content to dictionary is to add new key/value pairs and modify or delete existing key/value pairs.
The following examples are given:
dict = {'name': 'Zara', 'age': 7, 'class': 'First'};
dict["age"]=27; #Modify the value of an existing key
dict["school"]="wutong"; #Add new key/value pairs
print "dict['age']: ", dict['age'];
print "dict['school']: ", dict['school'];
3.4 Delete Dictionaries
del dict['name']; # Delete the entry whose key is'name'
dict.clear(); # Empty all entries in the dictionary
del dict ; # Delete dictionary
- Note: The dictionary does not exist. del causes an exception.
3.5 Dictionary Built-in Function-Method
cmp(dict1, dict2) #Compare two dictionary elements.
len(dict) #Calculate the number of dictionary elements, that is, the total number of keys.
str(dict) #Output dictionary printable string representation.
type(variable) #Returns the input variable type and the dictionary type if the variable is a dictionary.
clear() #Delete all elements in the dictionary
copy() #Returns a shallow copy of a dictionary
fromkeys() #Create a new dictionary with the elements in the sequence seq as the keys of the dictionary, and val as the initial values of all keys in the dictionary
get(key, default=None) #Returns the value of the specified key if it is not in the dictionary
has_key(key) #If the key returns true in dictionary dict, otherwise false
items() #Returns a traversable array of (keys, values) tuples in a list
keys() #Returns all keys of a dictionary in a list
setdefault(key, default=None) #Similar to get(), but if the key does not exist in the dictionary, the key is added and the value is set to default.
update(dict2) #Update dictionary dict2 key/value pairs to Dict
values() #Returns all values in the dictionary in a list
Date and time of 4 days
4.1 Get the current time
import time, datetime;
localtime = time.localtime(time.time())
print "Local current time :", localtime
_Description: time. struct_time (tm_year=2014, tm_mon=3, tm_mday=21, tm_hour=15, tm_min=13, tm_sec=56, tm_wday=4, tm_yday=80, tm_dsist=0) belongs to the struct_time tuple. The struct_time tuple has the following attributes:
4.2 Getting Formatting Time
_can choose various formats according to the requirements, but the simplest function to obtain readable time patterns is asctime():
4.2.1 Date Converted to String
_Preferred: print time.strftime('% Y-% m-% d% H:% M:% S');
Secondly, print datetime.datetime.strftime(datetime.datetime.now(),'%Y-%m-%d%H:%M:%S')
_Finally: print str (datetime. datetime. now ()[: 19]
4.2.2 String Converted to Date
expire_time = "2013-05-21 09:50:35"
d = datetime.datetime.strptime(expire_time,"%Y-%m-%d %H:%M:%S")
print d;
4.2.3 Acquisition Date Difference
oneday = datetime.timedelta(days=1)
#Today, 2014-03-21
today = datetime.date.today()
#Yesterday, 2014-03-20
yesterday = datetime.date.today() - oneday
#Tomorrow, 2014-03-22
tomorrow = datetime.date.today() + oneday
#Get the time for today's zero, 2014-03-21:00:00:00
today_zero_time=datetime.datetime.strftime(today, '%Y-%m-%d %H:%M:%S')
#0:00:00.001000
print datetime.timedelta(milliseconds=1), #1 milliseconds
#0:00:01
print datetime.timedelta(seconds=1), #One second
#0:01:00
print datetime.timedelta(minutes=1), #1 minutes
#1:00:00
print datetime.timedelta(hours=1), #1 hours
#1 day, 0:00:00
print datetime.timedelta(days=1), #1 days
#7 days, 0:00:00
print datetime.timedelta(weeks=1)
4.2.4 acquisition time difference
#1 day, 0:00:00
oneday = datetime.timedelta(days=1)
#Today, 2014-03-21 16:07:23.943000
today_time = datetime.datetime.now()
#Yesterday, 2014-03-20 16:07:23.943000
yesterday_time = datetime.datetime.now() - oneday
#Tomorrow, 2014-03-22 16:07:23.943000
tomorrow_time = datetime.datetime.now() + oneday
Note that the time is a floating point number with milliseconds.
To get the current time, you need to format it:
print datetime.datetime.strftime(today_time, '%Y-%m-%d %H:%M:%S')
print datetime.datetime.strftime(yesterday_time, '%Y-%m-%d %H:%M:%S')
print datetime.datetime.strftime(tomorrow_time, '%Y-%m-%d %H:%M:%S')
4.2.5 Get the last day of last month
last_month_last_day = datetime.date(datetime.date.today().year,datetime.date.today().month,1)-datetime.timedelta(1)
4.2.6 string date formatted into seconds
_Return to Floating Point Type
expire_time = "2013-05-21 09:50:35"
d = datetime.datetime.strptime(expire_time,"%Y-%m-%d %H:%M:%S")
time_sec_float = time.mktime(d.timetuple())
print time_sec_float
4.2.7 days formatted as seconds
_Return to Floating Point Type
d = datetime.date.today()
time_sec_float = time.mktime(d.timetuple())
print time_sec_float
4.2.8 seconds to string
time_sec = time.time()
print time.strftime("%Y-%m-%d %H:%M:%S", time.localtime(time_sec))
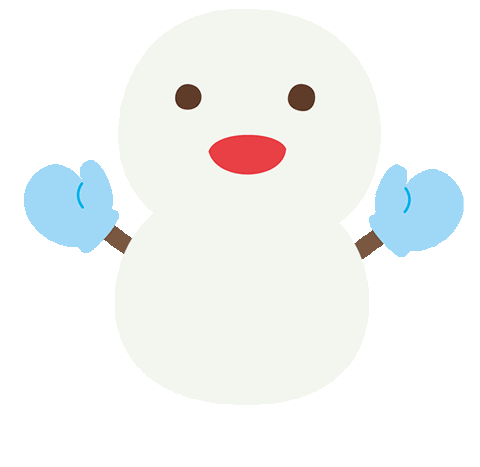
