Preface:
Android Device Manager It's Google that helps Android owners provide superior features in the following ways:
- Track the location of your Android device with a Google account to manage your device.
- Sound your Android phone, regardless of its location.
- Reset the lock screen password.
- Eliminate all data on your Android phone
Device Manager creation or more cumbersome, then we look at the api to create a simple function case.
First of all, go to the official document and see the Demo and its instructions given in the document:
Creating the manifest
To use the Device Administration API, the application's manifest must include the following:
A subclass of DeviceAdminReceiver
that includes the following:The BIND_DEVICE_ADMIN
permission.
The ability to respond to the ACTION_DEVICE_ADMIN_ENABLED
intent, expressed in the manifest as an intent filter.
A declaration of security policies used in metadata.
Here is an excerpt from the Device Administration sample manifest:
android:label="@string/activity_sample_device_admin"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.SAMPLE_CODE" /> </intent-filter> </activity> <receiver android:name=".app.DeviceAdminSample$DeviceAdminSampleReceiver" android:label="@string/sample_device_admin" android:description="@string/sample_device_admin_description" android:permission="android.permission.BIND_DEVICE_ADMIN"> <meta-data android:name="android.app.device_admin" android:resource="@xml/device_admin_sample" /> <intent-filter> <action android:name="android.app.action.DEVICE_ADMIN_ENABLED" /> </intent-filter> </receiver>
Since the activity interface system will help us, we just need to register for broadcasting here.
Step 1: Find the official document, copy and paste the receiver on it into the manifest file (copy to the manifest file, you will find many errors, don't be afraid to deal with the following little bit)
Step 2: Looking down at the document at the receiver node name, you will find that you need a DeviceAdminReceiver, so create a class inheriting DeviceAdminReceiver and register at the name as you did before when you built a broadcast receiver.
Step 3: Processing down and seeing @string, you will find that this requires strings, so you can write several strings yourself.
Step 4: "@xml/device_admin_sample processing, we created the XML folder according to his request and then created the XML file. The document gives the following demo:
<device-admin xmlns:android="http://schemas.android.com/apk/res/android"> <uses-policies> <limit-password /> <watch-login /> <reset-password /> <force-lock /> <wipe-data /> <expire-password /> <encrypted-storage /> <disable-camera /> </uses-policies> </device-admin>
Just paste this into your xml file.
Then you can write a simple layout button (my demo):
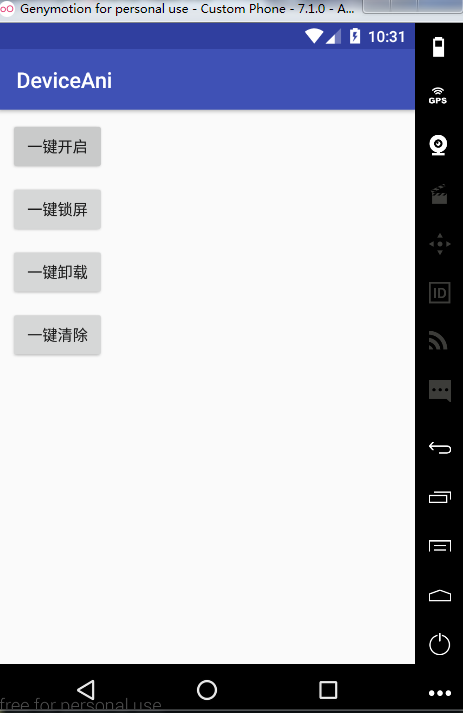
Step 5: Open the function by one key and then look down at the document and you will find that:
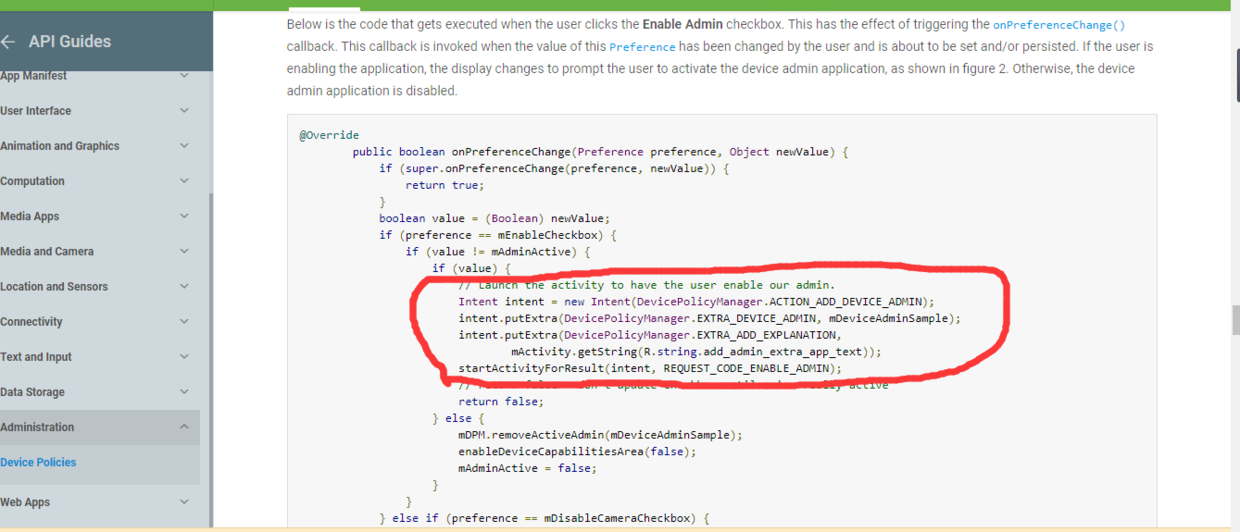
Document here shows how to open it or do you go to your open button as usual?
Error handling here. The following string is simple and you can write it yourself, but mDeviceAdminSample will find that you need a ComponentName object here.
The parameter context, the bytecode file corresponding to the class (broadcast receiver), does not need to return the result here, so the start activity is opened directly. My demo map is as follows
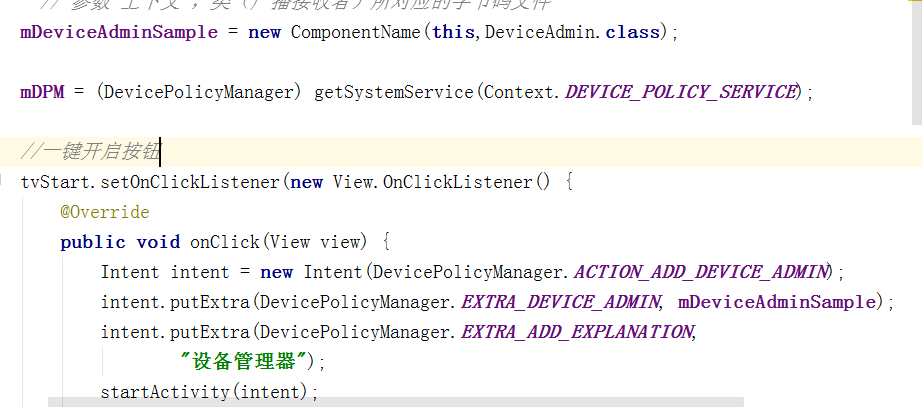
Step: 6 lock screen function
Documentation gives two ways to create it, and you'll find the second simpler.
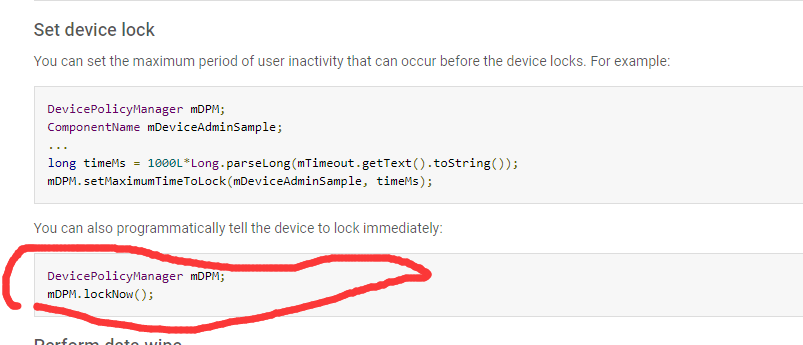
It's easy to use this example. (Learn to analyze the code DevicePolicy Manager is obtained by system services, mDPM is a member variable
so
mDPM = (DevicePolicyManager) getSystemService(Context.DEVICE_POLICY_SERVICE); // If the device manager is not activated, clicking on the lock screen will collapse. You can judge first. // Component objects can be used as judgement markers for activation or inactivation if (mDPM.isAdminActive(mDeviceAdminSample)){ mDPM.lockNow(); // Lock screen to set password at the same time mDPM.resetPassword("1996",0); }else{ Toast.makeText(MainActivity.this, "Please go to:Setting up Security Device Manager Click Activation", Toast.LENGTH_LONG).show(); }
Note: It's impossible to unload the application without shutting down the activation device manager.
Appendix (my code)
List file:
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.administrator.deviceani"> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <receiver android:name=".DeviceAdmin" android:label="@string/sample_device_admin" android:description="@string/sample_device_admin_description" android:permission="android.permission.BIND_DEVICE_ADMIN"> <meta-data android:name="android.app.device_admin" android:resource="@xml/device_admin_sample" /> <intent-filter> <action android:name="android.app.action.DEVICE_ADMIN_ENABLED" /> </intent-filter> </receiver> </application> </manifest>
java code
package com.example.administrator.deviceani; import android.app.admin.DevicePolicyManager; import android.content.ComponentName; import android.content.Context; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.Toast; public class MainActivity extends AppCompatActivity { private ComponentName mDeviceAdminSample; private DevicePolicyManager mDPM; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button tvLock = (Button) findViewById(R.id.tv_lock); Button tvRemove = (Button) findViewById(R.id.tv_remove); Button tvUninstall = (Button) findViewById(R.id.tv_uninstall); Button tvStart = (Button) findViewById(R.id.tv_start); // Parametric context, the bytecode file corresponding to the class (broadcast receiver) mDeviceAdminSample = new ComponentName(this,DeviceAdmin.class); mDPM = (DevicePolicyManager) getSystemService(Context.DEVICE_POLICY_SERVICE); //One-click Open Button tvStart.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Intent intent = new Intent(DevicePolicyManager.ACTION_ADD_DEVICE_ADMIN); intent.putExtra(DevicePolicyManager.EXTRA_DEVICE_ADMIN, mDeviceAdminSample); intent.putExtra(DevicePolicyManager.EXTRA_ADD_EXPLANATION, "Equipment Manager"); startActivity(intent); if (mDPM.isAdminActive(mDeviceAdminSample)){ Toast.makeText(MainActivity.this, "Kindly you have started", Toast.LENGTH_SHORT).show(); } } }); //One-click Lock Screen Button tvLock.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { //Lock screen function to view the set device lock below the document (code case) // DevicePolicyManager mDPM; //mDPM.lockNow(); //It's easy to use this example (learn to analyze the code DevicePolicy Manager is obtained by system services, mDPM is a member variable) // If the device manager is not activated, clicking on the lock screen will collapse. You can judge first. // Component objects can be used as judgement markers for activation or inactivation if (mDPM.isAdminActive(mDeviceAdminSample)){ mDPM.lockNow(); // Lock screen to set password at the same time mDPM.resetPassword("1996",0); }else{ Toast.makeText(MainActivity.this, "Please go to:Setting up Security Device Manager Click Activation", Toast.LENGTH_LONG).show(); } } }); } }
The simpler layout is no longer given.
Summary:
After the above steps to achieve the opening of the lock screen two functions below the function of looking at the document I believe you can also create a simple. As a beginner, I recently found that this function has a lot of uses in mobile phone anti-theft when I was working on a project. Because this step is more cumbersome, write down a stroke here, of course, it is also convenient for you to check it later.
Hope to be helpful to the needs, if there is insufficiency, welcome to give advice Hey Hey!!!