This article will mainly share with you how to quickly create node plug-ins and publish them to npm.
npm is a tool for JavaScript programmers to share and reuse code. We can not only install other people's plug-ins, but also publish our own code.
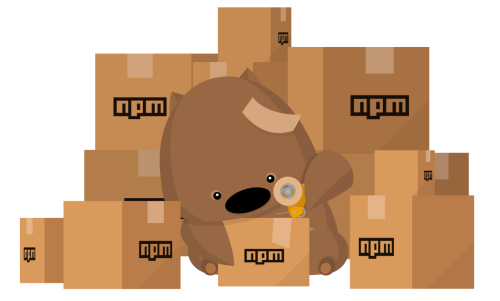
1. Initialize a node project
Let me name this node plugin my-plugin, create a directory my-plugin, enter the directory, and initialize the node project using NPM init-yes default mode. The command window command is as follows:
$ mkdir my-plugin $ cd my-plugin $ npm init --yes
At this time, package.json is generated in the project directory. The following is the basic configuration of the file.
"name": "my-plugin", "version": "1.0.0", "description": "A simple plug-in", "main": "myPlugin.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "repository": { "type": "git", "url": "https://github.com/xxx/my-plugin.git" }, "keywords": [ "my", "plugin" ], "author": "Name", "license": "MIT", "bugs": { "url": "https://github.com/xxx/my-plugin/issues" } }
The package.json related fields are described below.
-
Name of the name plug-in
-
Version plug-in version number
-
Description plug-in description
-
author's Name
-
main entry file path, request (name) will be introduced based on this path
-
keywords, using array form to facilitate npm official search
-
scripts command line, executed through npm run
-
License license, generally open source is MIT
-
repository github warehouse project address
Here I will create myPlugin.js as the main code file in the project directory, so I need to set the main field to myPlugin.js, so that when someone install s your plug-in, using require('my-plugin') is equivalent to require('node_modules/my-plugin/myPlugin.js').
Create the following files under my-plugin directory:
my-plugin ├── myPlugin.js #Main code ├── LICENSE #License ├── package.json #npm configuration file ├── README.md #Plug-in instructions
LICENSE can be generated directly on the github repository, step: create new file
-> File name input LICENSE - > Select the type on the right side, where MIT is generally selected.
MIT License Copyright (c) 2017 myName Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
2. Create code
Next, I implement a simple function in myPlugin.js, create an object, and implement a delay function sleep.
// myPlugin.js function myPlugin() { return { sleep: sleep } } function sleep(long) { var start = Date.now(); while((Date.now() - start) < long){ } console.log('finish!'); } module.exports = myPlugin();
The above code uses module.exports to export the object. In the node environment, we call it in the following way:
var myPlugin = require('my-plugin'); // or import myPlugin from 'my-plugin' myPlugin.sleep(2000);
In the end, if the plug-in developed by require is only a module running in the node environment, just need it, such as the code above. If we want to run the plug-in on the browser side, we need to be compatible with all kinds of build environments. The following code refers to the well-known q module (polyfill, which implements promise in the early stage) and passes code fragments into the compatible as parameters.
// myPlugin.js (function (definition) { "use strict"; // CommonJS if (typeof exports === "object" && typeof module === "object") { module.exports = definition(); // RequireJS } else if (typeof define === "function" && define.amd) { define(definition); // <script> } else if (typeof window !== "undefined" || typeof self !== "undefined") { // Prefer window over self for add-on scripts. Use self for // non-windowed contexts. var global = typeof window !== "undefined" ? window : self; // initialize myPlugin as a global. global.myPlugin = definition(); } else { throw new Error("This environment was not anticipated by myPlugin,Please file a bug."); } })(function () { function myPlugin() { return { sleep: sleep } } function sleep(long) { var start = Date.now(); while((Date.now() - start) < long){} console.log('finish!'); } return myPlugin(); })
Among them, according to the common JS grammar specification, compatible with node environment and web pack construction tools, we use module.exports; use define(myPlugin) amd grammar compatible with requireJS; and finally, if only in the way of script on the html page, assign the object to window.myPlugin.
// html <script src='my-plugin/myPlugin.js'></script> // js if (window.myPlugin) console.log('this is my plugin!');
3. Release
A simple node plug-in has been developed, and now we want to publish it to the npm official website for you to install cai (keng). For the first time, you need to register your account on the npm official website and log in directly next time.
$ npm adduser //Registered Account Username: YOUR_USER_NAME Password: YOUR_PASSWORD Email: YOUR_EMAIL@domain.com $ npm publish . //Release
Now, you can search your published package by typing my-plugin on the npm official website. You can install the newly released plugin into your own project directly through npm install my-plugin or yarn add my-plugin.
Finally, if a bug is found in the plug-in and you want to redistribute it after modification, you will report an error by directly executing npm push. Since npm checks that the version you released already exists, you need to update your version number to redistribute it. At this time, you need the following commands:
$ npm version patch
For your npm package version number, you will find that the version field of package.json has been upgraded from 0.0.0 to 0.0.1. Now execute publish, and you can see that your package has been updated on the npm official website.
Other
The above is a simple process of node plug-in release. In fact, the development of node plug-in includes many scenarios. A large project also needs to consider unit testing, code compression, integration testing and so on.
A complete node plug-in directory structure is generally as follows:
Directory structure of large node plug-ins
. ├── bin #Running Directory ├── lib #Home Code Directory ├── example #Example catalogue ├── test #Test directory, providing unit tests ├── .travis.yml #Integrated Automatic Test Configuration ├── .npmignore #Files ignored when npm is published ├── CHANGELOG.md #Version update instructions ├── LICENSE #License ├── package.json #npm configuration ├── README.md #README
Reference material:
Nguyen Yifeng npm module management