1. Configparser installation
pip3 install configparser
2. Introduction to configparser
- The python package used to read the configuration file;
- Generally, this module is used to encapsulate some constants when doing automated testing. For example, database, email, user name and password, project constant, etc;
- The usage is determined according to personal preferences and projects. You don't have to use this module. You can also use other methods for configuration, such as py file, xml, excel, yaml, json, etc.
- The source code of configparser is about 1360 lines. Reading through the source code can effectively understand the use of this module. This article only briefly introduces the common methods.
3 representation
- Create a new file named conf.py;
- Write the following data in the following format:
[mysqldb] sql_host = 127.0.0.1 sql_port = 3699 sql_user = root sql_pass = 123456 [mailinfo] name = NoamaNelson passwd = 123456 address = 123456@qq.com
4. Detailed use of configparser
4.1 object initialization
- Write the following code to initialize the object.
# -*- coding:utf-8 -*- # By noama Nelson # Date: November 19, 2021 # File name: conf.py # Function: use of configparser module # Contact: VX (noama Nelson) # Blog: https://blog.csdn.net/NoamaNelson import configparser import os class Conf: def __init__(self): self.conf = configparser.ConfigParser() self.root_path = os.path.dirname(os.path.abspath(__file__)) self.f = os.path.join(self.root_path + "/config.conf") self.conf.read(self.f)
4.2 get all sections
def read_sections(self): print(f"1,Get all sections:{self.conf.sections()}") if __name__ == "__main__": aa = Conf() aa.read_sections()
- The result is:
D:\Python37\python.exe F:/python_study/conf.py 1,Get all sections:['mysqldb', 'mailinfo']
4.3 obtain the options corresponding to all sections
def read_options(self, s1, s2): print(f"2,obtain mysqldb be-all options:{self.conf.options(s1)}") print(f"3,obtain mailinfo be-all options:{self.conf.options(s2)}") if __name__ == "__main__": aa = Conf() aa.read_sections() aa.read_options("mysqldb", "mailinfo")
- The result is:
D:\Python37\python.exe F:/python_study/conf.py 1,Get all sections:['mysqldb', 'mailinfo'] 2,obtain mysqldb be-all options:['sql_host', 'sql_port', 'sql_user', 'sql_pass'] 3,obtain mailinfo be-all options:['name', 'passwd', 'address']
4.4 read method and get method to obtain the option value under the specified section
- Read is to read the configuration file. For example, self.conf.read(self.f) is to read the specified config.conf file;
- Get is to get a specific value;
def read_conf(self, m, n): name = self.conf.get(m, n) # Gets the option value of the specified section print(f"4,Get specified section:{m}Lower option: {n}The value of is{name}") if __name__ == "__main__": aa = Conf() aa.read_sections() aa.read_options("mysqldb", "mailinfo") aa.read_conf("mysqldb", "sql_host")
- The result is:
D:\Python37\python.exe F:/python_study/conf.py 1,Get all sections:['mysqldb', 'mailinfo'] 2,obtain mysqldb be-all options:['sql_host', 'sql_port', 'sql_user', 'sql_pass'] 3,obtain mailinfo be-all options:['name', 'passwd', 'address'] 4,Get specified section:mysqldb Lower option: sql_host The value of is 127.0.0.1
4.5 items method to obtain the configuration information used for pointing the section
def get_items(self, m, n): print(f"5,obtain sectoion:{m}The configuration information under is:{self.conf.items(m)}") print(f"6,obtain sectoion:{n}The configuration information under is:{self.conf.items(n)}") if __name__ == "__main__": aa = Conf() aa.read_sections() aa.read_options("mysqldb", "mailinfo") aa.read_conf("mysqldb", "sql_host") aa.get_items("mysqldb", "mailinfo")
- The result is:
D:\Python37\python.exe F:/python_study/conf.py 1,Get all sections:['mysqldb', 'mailinfo'] 2,obtain mysqldb be-all options:['sql_host', 'sql_port', 'sql_user', 'sql_pass'] 3,obtain mailinfo be-all options:['name', 'passwd', 'address'] 4,Get specified section:mysqldb Lower option: sql_host The value of is 127.0.0.1 5,obtain sectoion:mysqldb The configuration information under is:[('sql_host', '127.0.0.1'), ('sql_port', '3699'), ('sql_user', 'root'), ('sql_pass', '123456')] 6,obtain sectoion:mailinfo The configuration information under is:[('name', 'NoamaNelson'), ('passwd', '123456'), ('address', '123456@qq.com')]
4.6 set and write methods to modify the value of an option
- If option does not exist, create it;
def set_option(self, m, n, s): self.conf.set(m, n, s) self.conf.write(open(self.f, "w")) print(f"7,set up setion:{m}Lower option:{n}The value of is:{s}") if __name__ == "__main__": aa = Conf() aa.read_sections() aa.read_options("mysqldb", "mailinfo") aa.read_conf("mysqldb", "sql_host") aa.get_items("mysqldb", "mailinfo") aa.set_option("mysqldb", "sql_name", "tourist")
- The result is:
D:\Python37\python.exe F:/python_study/conf.py 1,Get all sections:['mysqldb', 'mailinfo'] 2,obtain mysqldb be-all options:['sql_host', 'sql_port', 'sql_user', 'sql_pass'] 3,obtain mailinfo be-all options:['name', 'passwd', 'address'] 4,Get specified section:mysqldb Lower option: sql_host The value of is 127.0.0.1 5,obtain sectoion:mysqldb The configuration information under is:[('sql_host', '127.0.0.1'), ('sql_port', '3699'), ('sql_user', 'root'), ('sql_pass', '123456')] 6,obtain sectoion:mailinfo The configuration information under is:[('name', 'NoamaNelson'), ('passwd', '123456'), ('address', '123456@qq.com')] 7,set up setion:mysqldb Lower option:sql_name The value of is: tourists
- Because there was no SQL in the previous configuration file_ Name, so it will be newly created. If the created content exists, directly modify the corresponding value;
4.7 has_section and has_option method
- has_ The section method checks whether the corresponding section exists;
- has_ The option method checks whether the corresponding option exists;
def has_s_o(self, s, o): print(f"8,inspect section: {s}Is there:{self.conf.has_section(s)}") print(f"9,inspect section: {s}Lower option: {o}Is there:{self.conf.has_option(s, o)}") if __name__ == "__main__": aa = Conf() aa.read_sections() aa.read_options("mysqldb", "mailinfo") aa.read_conf("mysqldb", "sql_host") aa.get_items("mysqldb", "mailinfo") aa.set_option("mysqldb", "sql_name", "tourist") aa.has_s_o("mysqldb", "sql_name")
- The result is:
D:\Python37\python.exe F:/python_study/conf.py 1,Get all sections:['mysqldb', 'mailinfo'] 2,obtain mysqldb be-all options:['sql_host', 'sql_port', 'sql_user', 'sql_pass', 'sql_name'] 3,obtain mailinfo be-all options:['name', 'passwd', 'address'] 4,Get specified section:mysqldb Lower option: sql_host The value of is 127.0.0.1 5,obtain sectoion:mysqldb The configuration information under is:[('sql_host', '127.0.0.1'), ('sql_port', '3699'), ('sql_user', 'root'), ('sql_pass', '123456'), ('sql_name', 'tourist')] 6,obtain sectoion:mailinfo The configuration information under is:[('name', 'NoamaNelson'), ('passwd', '123456'), ('address', '123456@qq.com')] 7,set up setion:mysqldb Lower option:sql_name The value of is: tourists 8,inspect section: mysqldb Is there: True 9,inspect section: mysqldb Lower option: sql_name Is there: True
4.8 add_section method, adding section and option
-
add_section: add a section;
-
Before adding:
def add_s_o(self, s, o, v): if not self.conf.has_section(s): self.conf.add_section(s) print(f"10,Add new section by{s}") else: print(f"10,Add new section by{s}Already exists, no need to add!") if not self.conf.has_option(s, o): self.conf.set(s, o, v) print(f"11,To add option by{o}, Value is{v}") else: print(f"11,To add option by{o}, Value is{v},Already exists, no need to add!") self.conf.write(open(self.f, "w")) if __name__ == "__main__": aa = Conf() aa.read_sections() aa.read_options("mysqldb", "mailinfo") aa.read_conf("mysqldb", "sql_host") aa.get_items("mysqldb", "mailinfo") aa.set_option("mysqldb", "sql_name", "tourist") aa.has_s_o("mysqldb", "sql_name") aa.add_s_o("login", "name", "root")
- After adding:
D:\Python37\python.exe F:/python_study/conf.py 1,Get all sections:['mysqldb', 'mailinfo'] 2,obtain mysqldb be-all options:['sql_host', 'sql_port', 'sql_user', 'sql_pass', 'sql_name'] 3,obtain mailinfo be-all options:['name', 'passwd', 'address'] 4,Get specified section:mysqldb Lower option: sql_host The value of is 127.0.0.1 5,obtain sectoion:mysqldb The configuration information under is:[('sql_host', '127.0.0.1'), ('sql_port', '3699'), ('sql_user', 'root'), ('sql_pass', '123456'), ('sql_name', 'tourist')] 6,obtain sectoion:mailinfo The configuration information under is:[('name', 'NoamaNelson'), ('passwd', '123456'), ('address', '123456@qq.com')] 7,set up setion:mysqldb Lower option:sql_name The value of is: tourists 8,inspect section: mysqldb Is there: True 9,inspect section: mysqldb Lower option: sql_name Is there: True 10,Add new section by login 11,To add option by name, Value is root
- When you run the code again, you will be prompted that it already exists:
10,Add new section by login Already exists, no need to add! 11,To add option by name, Value is root,Already exists, no need to add!
4.9 remove_section and remove_option method, delete section and option
def remove_s_o(self, s, o): if self.conf.has_section(s): self.conf.remove_section(s) print(f"12,delete section:{s}==OK!") else: print(f"12,To delete section:{s}Does not exist, do not delete!") if self.conf.has_option(s, o): self.conf.remove_option(s, o) print(f"13,delete section: {s}Lower option: {o}==OK!") else: print(f"13,To delete section: {s}Lower option: {o}Does not exist, do not delete!") if __name__ == "__main__": aa = Conf() aa.read_sections() aa.read_options("mysqldb", "mailinfo") aa.read_conf("mysqldb", "sql_host") aa.get_items("mysqldb", "mailinfo") aa.set_option("mysqldb", "sql_name", "tourist") aa.has_s_o("mysqldb", "sql_name") aa.add_s_o("login", "name", "root") aa.remove_s_o("login", "name")
- The result is:
D:\Python37\python.exe F:/python_study/conf.py 1,Get all sections:['mysqldb', 'mailinfo', 'login'] 2,obtain mysqldb be-all options:['sql_host', 'sql_port', 'sql_user', 'sql_pass', 'sql_name'] 3,obtain mailinfo be-all options:['name', 'passwd', 'address'] 4,Get specified section:mysqldb Lower option: sql_host The value of is 127.0.0.1 5,obtain sectoion:mysqldb The configuration information under is:[('sql_host', '127.0.0.1'), ('sql_port', '3699'), ('sql_user', 'root'), ('sql_pass', '123456'), ('sql_name', 'tourist')] 6,obtain sectoion:mailinfo The configuration information under is:[('name', 'NoamaNelson'), ('passwd', '123456'), ('address', '123456@qq.com')] 7,set up setion:mysqldb Lower option:sql_name The value of is: tourists 8,inspect section: mysqldb Is there: True 9,inspect section: mysqldb Lower option: sql_name Is there: True 10,Add new section by login Already exists, no need to add! 11,To add option by name, Value is root,Already exists, no need to add! 12,delete section:login==OK! 13,To delete section: login Lower option: name Does not exist, do not delete!
5. Common exceptions of configparser
abnormal | describe |
---|---|
ConfigParser.Error | Base class for all exceptions |
ConfigParser.NoSectionError | The specified section was not found |
ConfigParser.DuplicateSectionError | Call add_ The section name has already been used when section() |
ConfigParser.NoOptionError | The specified parameter was not found |
ConfigParser.InterpolationError | An unexpected base class occurs when there is a problem performing string interpolation |
ConfigParser.InterpolationDepthError | When the string interpolation cannot be completed, it cannot be completed because the number of iterations exceeds the maximum range. Subclass of InterpolationError |
InterpolationMissingOptionError | An exception occurs when the referenced option does not exist. Subclass of InterpolationError |
ConfigParser.InterpolationSyntaxError | An exception occurs when the resulting replacement source text does not conform to the required syntax. Subclass of InterpolationError. |
ConfigParser.MissingSectionHeaderError | An exception occurs when trying to parse a file without a segmented title. |
ConfigParser.ParsingError | An exception occurs when an error occurs while trying to parse a file |
ConfigParser.MAX_INTERPOLATION_DEPTH | When the raw parameter is false, the maximum depth of recursive interpolation of get(). This applies only to the ConfigParser class |
6 all source code of this article
# -*- coding:utf-8 -*- # By noama Nelson # Date: November 19, 2021 # File name: conf.py # Function: use of configparser module # Contact: VX (noama Nelson) # Blog: https://blog.csdn.net/NoamaNelson import configparser import os class Conf: def __init__(self): self.conf = configparser.ConfigParser() self.root_path = os.path.dirname(os.path.abspath(__file__)) self.f = os.path.join(self.root_path + "/config.conf") self.conf.read(self.f) def read_sections(self): print(f"1,Get all sections:{self.conf.sections()}") def read_options(self, s1, s2): print(f"2,obtain mysqldb be-all options:{self.conf.options(s1)}") print(f"3,obtain mailinfo be-all options:{self.conf.options(s2)}") def read_conf(self, m, n): name = self.conf.get(m, n) # Gets the option value of the specified section print(f"4,Get specified section:{m}Lower option: {n}The value of is{name}") def get_items(self, m, n): print(f"5,obtain sectoion:{m}The configuration information under is:{self.conf.items(m)}") print(f"6,obtain sectoion:{n}The configuration information under is:{self.conf.items(n)}") def set_option(self, m, n, s): self.conf.set(m, n, s) self.conf.write(open(self.f, "w")) print(f"7,set up setion:{m}Lower option:{n}The value of is:{s}") def has_s_o(self, s, o): print(f"8,inspect section: {s}Is there:{self.conf.has_section(s)}") print(f"9,inspect section: {s}Lower option: {o}Is there:{self.conf.has_option(s, o)}") def add_s_o(self, s, o, v): if not self.conf.has_section(s): self.conf.add_section(s) print(f"10,Add new section by{s}") else: print(f"10,Add new section by{s}Already exists, no need to add!") if not self.conf.has_option(s, o): self.conf.set(s, o, v) print(f"11,To add option by{o}, Value is{v}") else: print(f"11,To add option by{o}, Value is{v},Already exists, no need to add!") self.conf.write(open(self.f, "w")) def remove_s_o(self, s, o): if self.conf.has_section(s): self.conf.remove_section(s) print(f"12,delete section:{s}==OK!") else: print(f"12,To delete section:{s}Does not exist, do not delete!") if self.conf.has_option(s, o): self.conf.remove_option(s, o) print(f"13,delete section: {s}Lower option: {o}==OK!") else: print(f"13,To delete section: {s}Lower option: {o}Does not exist, do not delete!") if __name__ == "__main__": aa = Conf() aa.read_sections() aa.read_options("mysqldb", "mailinfo") aa.read_conf("mysqldb", "sql_host") aa.get_items("mysqldb", "mailinfo") aa.set_option("mysqldb", "sql_name", "tourist") aa.has_s_o("mysqldb", "sql_name") aa.add_s_o("login", "name", "root") aa.remove_s_o("login", "name")
"Full stack testing technology, sharing, mutual encouragement, progress and improvement"
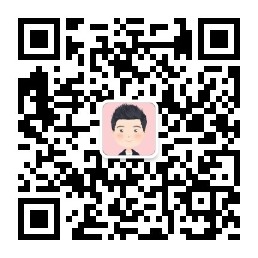