This article is excerpted from "design patterns should be learned this way"
For example, we often communicate with our colleagues through e-mail messages, SMS messages or messages in the system. Especially when we go through some approval processes, we need to record these processes for future reference. According to the type, messages can be divided into mail messages, SMS messages and messages in the system. However, according to the degree of urgency, messages can be divided into ordinary messages, urgent messages and urgent messages. Obviously, the whole message system can be divided into two dimensions, as shown in the figure below.
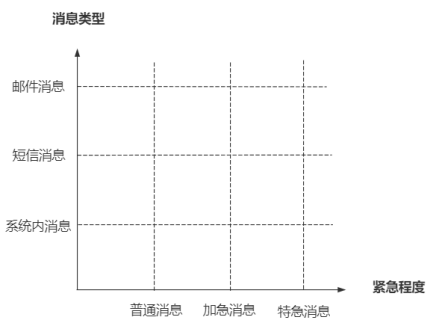
If we use inheritance, the situation is complicated and not conducive to expansion. Mail messages can be ordinary or urgent; SMS messages can be ordinary or urgent. Next, we use bridge mode to solve this problem.
First, create an IMessage interface to act as a bridge.
/** * Unified interface for message sending */ public interface IMessage { //The content and recipient of the message to be sent void send(String message, String toUser); }
Create a mail message to implement the EmailMessage class.
/** * Implementation class of mail message */ public class EmailMessage implements IMessage { public void send(String message, String toUser) { System.out.println("Send with mail message" + message + "to" + toUser); } }
Create SMS message and implement SmsMessage class.
/** * Implementation class of short message * SMS(Short IMessage Service)Short message service */ public class SmsMessage implements IMessage { public void send(String message, String toUser) { System.out.println("Send with SMS message" + message + "to" + toUser); } }
Then create the bridge Abstract role AbstractMessage class.
/** * Abstract message class */ public abstract class AbstractMessage { //An object that holds an implementation part IMessage message; //Construction method, passing in the object of the implementation part public AbstractMessage(IMessage message) { this.message = message; } //Send a message and delegate it to the method of the implementation part public void sendMessage(String message, String toUser) { this.message.send(message, toUser); } }
Create a NomalMessage class that implements ordinary messages.
/** * General message class */ public class NomalMessage extends AbstractMessage { //Construction method, passing in the object of the implementation part public NomalMessage(IMessage message) { super(message); } @Override public void sendMessage(String message, String toUser) { //For ordinary messages, you can directly call the parent class method to send the message super.sendMessage(message, toUser); } }
Create the UrgencyMessage class that implements the urgent message.
/** * Urgent message class */ public class UrgencyMessage extends AbstractMessage { //Construction method public UrgencyMessage(IMessage message) { super(message); } @Override public void sendMessage(String message, String toUser) { message = "Urgent:" + message; super.sendMessage(message, toUser); } //Expand its function to monitor the processing status of a message public Object watch(String messageId) { //Query the processing status of the message according to the given message code (messageId) //Organize into monitored processing status, and then return return null; } }
Finally, write the client test code.
public static void main(String[] args) { IMessage message = new SmsMessage(); AbstractMessage abstractMessage = new NomalMessage(message); abstractMessage.sendMessage("Quick approval of overtime application", "Wang Zong"); message = new EmailMessage(); abstractMessage = new UrgencyMessage(message); abstractMessage.sendMessage("Quick approval of overtime application", "Wang Zong"); }
The operation results are shown in the figure below.
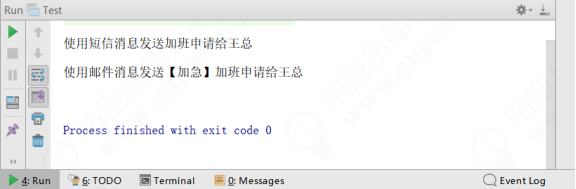
In the above case, we use the bridging mode to decouple the two independent dimensions of "message type" and "message urgency". If there are more message types in the future, such as wechat and nailing, you can directly create a new class to inherit IMessage; If the urgency needs to be added, just create a new class to implement the AbstractMessage class.
This article is the original of "Tom bomb architecture". Please indicate the source for reprint. Technology lies in sharing, I share my happiness!