Bridging mode
preface
The code implementation of the bridge mode is very simple, but it is a little difficult to understand, and the application scenarios are relatively limited. Therefore, equivalent to the agent mode, the bridge mode is not so commonly used in actual projects, so it is OK to distinguish it. There is no key learning here.
definition
Bridging pattern: separate the abstract part from its implementation part so that they can change independently.
After reading the definition, I still look confused 😳
Take chestnuts for example: use the mobile phone chestnuts in the big talk mode to analyze
We know that different brands of mobile phones and different versions of different brands of mobile phones have different requirements for mobile phone software. Maybe the software in brand M can not be used in brand N mobile phones. The software of generation 10 of M brand may not be installed and used in generation 1.
If we use code to implement this relationship:
1. Structure diagram implemented by brand classification
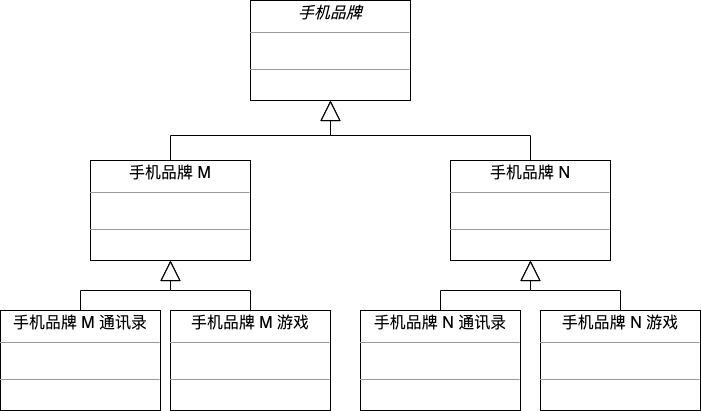
2. Structure diagram of software classification
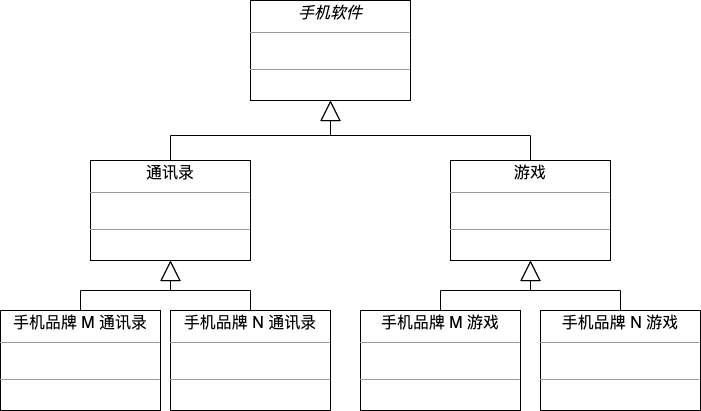
The first and second implementations above
If we need to add A,B and C for mobile phone brands, we need to add notes, input method and alarm clock for Software...
Then the originally written modules also need to be modified. Obviously, this modification is disaster level, and the addition of new functions requires many modules to be modified
3. Structure diagram of implementation using bridge mode
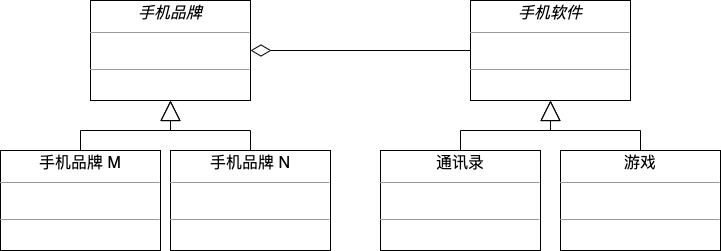
The third is the bridging mode we talk about. If there are mobile phone brands and software types, it only needs to be expanded, and the previously written modules do not need to be modified.
Because there are many ways of implementation, the core of bridge mode is to separate these implementations and let them change themselves. In this way, each change will not affect the realization of other changes, so as to achieve the purpose of coping with changes.
advantage
1. Separation of abstraction and implementation, strong scalability
2. Comply with the opening and closing principle
3. Comply with the principle of synthetic reuse
4. Its implementation details are transparent to customers
Generally speaking, our implementation system may have multi angle classification, and each type may change. What the bridge mode does is to separate these multi angles, so that they can change themselves without affecting other modules and reduce the coupling between them.
shortcoming
The introduction of bridging mode will increase the difficulty of system understanding and design. Because the aggregation association relationship is based on the abstraction layer, developers are required to design and program for the abstraction.
Application scenario
1. If a system needs to add more flexibility between the abstract role and concrete role of components to avoid establishing static inheritance relationship between the two levels, they can establish an association relationship at the abstract level through bridging mode.
2. The bridging mode is especially suitable for those systems that do not want to use inheritance or the number of system classes increases sharply due to multi-level inheritance.
3. A class has two independently changing dimensions, and both dimensions need to be extended.
code implementation
Or the chestnuts on the cell phone
// Mobile software type HandsetSoft interface { Run() string } // mobile game type HandsetGame struct { } func (hg *HandsetGame) Run() string { return "Running mobile games" } // Mobile phone address book type HandsetAddressList struct { } func (hg *HandsetAddressList) Run() string { return "Run phone book" } // Mobile phone brand type HandsetBrand interface { SetHandsetSoft(HandsetSoft) } // M brand mobile phone type HandsetBrandM struct { HandsetSoft } func NewHandsetBrandM() *HandsetBrandM { return &HandsetBrandM{} } func (hw *HandsetBrandM) SetHandsetSoft(soft HandsetSoft) { hw.HandsetSoft = soft } func (hw *HandsetBrandM) Run() string { return "M Brand mobile phone-" + hw.HandsetSoft.Run() } // Brand N mobile phone type HandsetBrandN struct { HandsetSoft } func NewHandsetBrandN() *HandsetBrandN { return &HandsetBrandN{} } func (ap *HandsetBrandN) SetHandsetSoft(soft HandsetSoft) { ap.HandsetSoft = soft } func (ap *HandsetBrandN) Run() string { return "N Brand mobile phone-" + ap.HandsetSoft.Run() }
reference resources
[code in text] https://github.com/boilingfrog/design-pattern-learning/tree/master/ Bridging mode
[big talk design mode] https://book.douban.com/subject/2334288/
[geek time] https://time.geekbang.org/column/intro/100039001
[bridging mode] https://boilingfrog.github.io/2021/11/11/ Using go to implement bridging mode/