1, Brief introduction to command mode
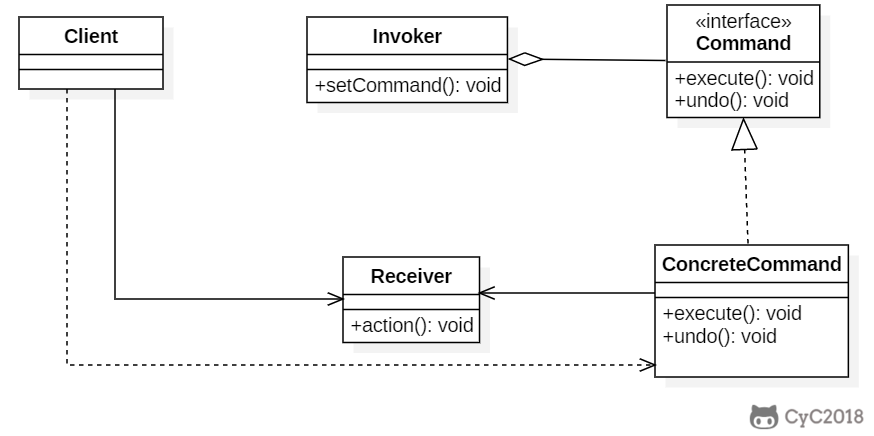
- command Pattern, in the process of software snake, we often need to send requests to some objects, but we don't know who the receiver of the request is, and what the requested operation is. We just need to make a specific request receiver when the program is running, at this time, we can use the command Pattern to design;
- The command mode makes the request sender and the request receiver decouple from each other, and makes the calling relationship between objects more flexible and decoupled;
- In the command mode, a request is also encapsulated as an object, so that different parameters can be used to represent different requests. At the same time, the command mode also supports revocable operations;
- Generally speaking, the general issues orders and the soldiers execute them. There are several roles: General (command issuer), soldier (command executor), command (connect soldier and soldier).
**2, Components of command mode
- Invoker: caller;
- Command: command role. All commands to be executed are here. They can be interfaces or abstract classes;
- Receiver: the receiver role, which knows how to implement and execute a request related operation.
- ConcreateCommand: a receiver object and an action are used to call the corresponding operation of the receiver to implement execute.
3, Notes and details of command mode
- Decouple the object that initiates the request and the object that executes the request. The object that initiates the request is the caller. The caller only needs to call the execute() method of the command object to let the receiver work, without knowing who the specific receiver object is and how it is implemented. The command object will be responsible for letting the commander execute the request action, that is, "request initiator" and "please" The decoupling between executors is realized by command objects, which act as a link and bridge.
- It is easy to design a command queue. As long as the command is put in the queue, it can be executed by multiple threads;
- It is easy to revoke and redo the request;
- Insufficient command mode: it will lead to too many specific command classes in some systems and increase the complexity of the system, which should be noted;
- Empty command is also a design mode, which saves the operation of empty judgment for us;
- Classic application scenario of command mode: a button in the interface is a command, simulating DOC command, order recovery and cancellation, trigger feedback mechanism.
4, Command mode example
public interface Command {
public void execute();
}
public class Light {
public void on() {
System.out.println("Light is on!");
}
public void off() {
System.out.println("Light is off!");
}
}
public class LightOffCommand implements Command {
private Light light;
public LightOffCommand(Light light) {
this.light = light;
}
@Override
public void execute() {
light.off();
}
}
public class LightOnCommand implements Command {
private Light light;
public LightOnCommand(Light light) {
this.light = light;
}
@Override
public void execute() {
light.on();
}
}
public class Invoker {
private Command[] onCommands;
private Command[] offCommands;
private final int slotNum = 7;
public Invoker() {
this.onCommands = new Command[slotNum];
this.offCommands = new Command[slotNum];
}
public void setOnCommand(Command command, int slot) {
onCommands[slot] = command;
}
public void setOffCommand(Command command, int slot) {
offCommands[slot] = command;
}
public void onButtonWasPushed(int slot) {
onCommands[slot].execute();
}
public void offButtonWasPushed(int slot) {
offCommands[slot].execute();
}
}
public class Client {
public static void main(String[] args) {
Invoker invoker = new Invoker();
Light light = new Light();
Command lightOnCommand = new LightOnCommand(light);
Command lightOffCommand = new LightOffCommand(light);
invoker.setOnCommand(lightOnCommand, 0);
invoker.setOffCommand(lightOffCommand, 0);
invoker.onButtonWasPushed(0);
invoker.offButtonWasPushed(0);
}
}