In the development of Android projects, if we need to call the functions in web in Android programs, we can use Jsbridge as a bridge for communication invocation.
Sample demonstration:
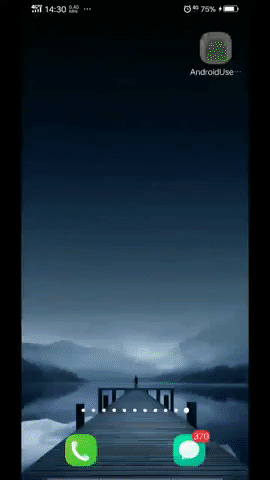
Jsbridge mutual call demonstration
Jsbridge library introduction:
implementation 'com.github.lzyzsd:jsbridge:1.0.4'
One side provides the method called by the other side, which needs to be registered with the registerHandler() method, and the callback reception called by the other side requires the callHandler() method to get the callback.
Android part code:
private void initView() { tvJs = (TextView) findViewById(R.id.tv_androidcalljs); tvShowmsg = (TextView) findViewById(R.id.tv_showmsg); webview = (BridgeWebView) findViewById(R.id.webview); WebSettings webSettings = webview.getSettings(); webSettings.setBuiltInZoomControls(true); webSettings.setSupportZoom(true); //Interaction with js must be set webSettings.setJavaScriptEnabled(true); webview.loadUrl("file:///android_asset/html.html"); } private void callJs(){ tvJs.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { webview.callHandler("functionInJs", "Android Pass to js Data", new CallBackFunction() { @Override public void onCallBack(String data) { tvShowmsg.setText(data); } }); } }); } private void registerInJs() { webview.registerHandler("functionInAndroid", new BridgeHandler() { @Override public void handler(String data, CallBackFunction function) { tvShowmsg.setText("js Called Android"); //Return to html function.onCallBack("Android Pass back to js Data"); } }); }
html part code:
<body> <h3>html Modular</h3> <p> <input type="button" id="enter" value="Call Android's methods" onclick="onClick();"/> </p> <p> <h3 id="showmsg"></h3> </p> <script> // Register event listening function connectWebViewJavascriptBridge(callback) { if (window.WebViewJavascriptBridge) { callback(WebViewJavascriptBridge) } else { document.addEventListener( 'WebViewJavascriptBridgeReady' , function() { callback(WebViewJavascriptBridge) }, false ); } } // Send a message to Android function onClick() { var data = document.getElementById("showmsg").value; window.WebViewJavascriptBridge.callHandler( 'functionInAndroid' , {'param': "js Pass to Android Data"} , function(responseData) { document.getElementById("showmsg").innerHTML = responseData; } ); } // Register the default Handler in JS connectWebViewJavascriptBridge(function(bridge) { //Initialization bridge.init(function(message, responseCallback) { var data = { 'Javascript Responds': 'HelloWorld' }; responseCallback(data); }); //Receive messages from Android and return them to Android for notification bridge.registerHandler("functionInJs", function(data, responseCallback) { document.getElementById("showmsg").innerHTML = data; var responseData = "js Pass back to Android Data"; responseCallback(responseData); }); }) </script> </body>
xml part code:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.example.androidusejs.MainActivity" android:orientation="vertical"> <LinearLayout android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1" android:padding="10dp" android:orientation="vertical"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Android Modular" android:textColor="#000" android:textStyle="bold" android:textSize="18sp"/> <TextView android:id="@+id/tv_androidcalljs" android:layout_width="170dp" android:layout_height="30dp" android:text="Android call Js" android:background="@drawable/roundsmall_bggreen" android:gravity="center" android:layout_marginTop="10dp" android:textColor="@color/white"/> <TextView android:id="@+id/tv_showmsg" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textColor="#000" android:layout_marginTop="20dp" android:textSize="20sp"/> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1"> <com.github.lzyzsd.jsbridge.BridgeWebView android:id="@+id/webview" android:layout_width="match_parent" android:layout_height="match_parent" /> </LinearLayout> </LinearLayout>