day17 - csv file and excel file operation and object-oriented programming (10.12)
1. python reads csv files
python read csv file – read csv file
Create folder - ctrl+c – ctrl+v add csv file (cancel)
utf-8-sig – > signed UTF-8 – > with byte order mark (to ensure safe reading)
import csv with open('resources/2018 Settlement data of points in Beijing in.csv', 'r', encoding='utf-8-sig') as file: #Method 1 read # content = file.readline() #Read content line by line # while content: # print(content, end='') # content = file.readline() # Until no data can be read # Method 2 read (read by csv.reader method) # The delimiter parameter is comma by default, but if the value is not separated by comma, it must be specified again # The default value of the quotechar parameter is quotation marks, but if the value is not enclosed in quotation marks, it must be specified again reader = csv.reader(file, delimiter=',', quotechar='"') # Reader [observe what the file content separator is] for line in reader: print(type(line)) # list print(line)
2. python writes data to csv file
""" Write data to csv file """ # Utf-8-sig -- > signed UTF-8 -- > is marked with byte order (to ensure safe reading) import csv with open('resources/2018 Settlement data of points in Beijing in.csv', 'a', encoding='utf-8-sig', newline='') as file: writer = csv.writer(file, delimiter=',') # file object, newline = '' remove new empty lines writer.writerow(['6020', 'Chen Lai', '1981-06', 'Beijing P & G Technology Co., Ltd', '90.75']) # Write a line writer.writerows([ ['6021', 'Chen Lai', '1981-06', 'Beijing P & G Technology Co., Ltd', '90.75'], ['6022', 'Chen Lai', '1981-06', 'Beijing P & G Technology Co., Ltd', '90.75'], ['6023', 'Chen Lai', '1981-06', 'Beijing P & G Technology Co., Ltd', '90.75'] ]) # Write multiline data
3. Read csv file and write it into excel file
Exercise: read csv and write excel
Writing excel requires the support of third-party libraries. First install pip install openpyxl, and then import openpyxl
pip list to view the installed third-party library
The standard sequence of codes: Standard Library - > third-party library - > code, written in alphabetical order
# 1) Practice by yourself import csv import openpyxl # Writing excel requires the support of third-party libraries. First install pip install openpyxl, and then import openpyxl # pip list to view the installed third-party library # The standard sequence of codes: Standard Library - > third-party library - > code, written in alphabetical order from openpyxl.styles import Font, Alignment workbook = openpyxl.Workbook() sheet = workbook.active with open('resources/2018 Settlement data of points in Beijing in.csv', 'r', encoding='utf-8-sig') as file: reader = csv.reader(file, delimiter=',', quotechar='"') for line in reader: # List data sheet.append(line) # Sheet.append (sequence) workbook.save('Beijing integral settlement data.xlsx') #2) teacher answer workbook = openpyxl.Workbook() sheet = workbook.active # Get the first sheet1 sheet.title = '2018 year' # Modify the first table name with open('resources/2018 Settlement data of points in Beijing in.csv', 'r', encoding='utf-8-sig') as file: reader = csv.reader(file, delimiter=',', quotechar='"') # Separation number, package number ". for line in reader: sheet.append(line) # Append a row of data # Modifies the height of the specified row sheet.row_dimensions[1].height = 40 #The first row of dimensions starts from 1 and the height is 0 # Modifies the width of the specified column col_width = { 'A': 30, 'B': 50, 'C': 60, 'D': 180, 'E': 50 } for key in col_width: sheet.column_dimensions[key].width = col_width[key] #1) sheet.cell(1,1) # First row first column cell object #Modify cell formatting for col in range(1, 6): sheet.cell(1, col).font = Font(name='Microsoft YaHei ', size=22, bold=True, color='0000FF') # Modify font, color table sheet.cell(1, col).alignment = Alignment(horizontal='center', vertical='center') # Horizontal, vertical, centered workbook.save('resources/2018 Settlement data of points in Beijing in.xlsx') # Read excel file
4. * python reads excel file data
""" 04-python read excel data """ import openpyxl # Load excel file -- > Workbook workbook = openpyxl.load_workbook('resources/Mask sales data.xlsx') # Load Workbook # Get the names of all worksheets print(workbook.sheetnames) # Get table name # Method 1 # workbook.worksheets[0] #First worksheet # Get the first worksheet -- worksheet sheet = workbook.worksheets[0] print(sheet) # There are two ways to get cell data print(sheet.cell(3, 4).value) # Wang Dadao print(sheet['D3'].value) # Wang Dadao # Range of cells print(sheet.dimensions) #A1:G1000 # Gets the number of rows and columns of the representation print(sheet.max_row, sheet.max_column) # Loop through all the data # 1) Method 1 for row in range(1, sheet.max_row+1): for col in range(1, sheet.max_column+1): print(sheet.cell(row, col).value, end='\t') print() # 2) Method 2 for row in range(2, sheet.max_row+1): for col in 'ABCDEF': print(sheet[f'{col}{row}'].value, end='\t') print()
5. Object oriented programming
"""
Object oriented programming (OOP) (content after inquiry, daily content)
The concept of object is used to logically organize the data and the functions operating the data into a whole
In the object-oriented world, any problem we want to solve is to create an object first and then send a message to the object.
1. Object - entity receiving message - > specific concept
1) Everything is an object
2) Each object is unique
3) Objects have both static characteristics (properties) and dynamic characteristics (behaviors)
4) The object must belong to a class
2. Class object blueprint and template - > abstract concept
1) (capitalize each word)
2) Functions written in classes are called methods
3)def init(self): initialization, self object
*Implementation steps of object-oriented programming:
1. Definition class
1) Data abstraction: give static characteristics of objects – > attributes
2) Behavior abstraction: give the dynamic characteristics of the object – > behavior
2. Create object - > constructor syntax is class name ()
3. Send message to the object
"""
"""
Class: pen
Object: my black signature pen
Attributes (static features): (noun) shape (rectangular cylindrical), refill (black refill, tip) 0.35, manufacturer, Chenguang.
Behavior (dynamic characteristics): Writing (verb)
"""
Step 1: define class
class Rectangle: """rectangle""" # Static properties def __init__(self, width, height): # (rectangular object, properties) """ Initialization method :param width: Width of rectangle :param height: Height of rectangle """ self.width = width self.height = height # Initialize the properties of the rectangular object and specify the height (data abstraction) # Dynamic characteristics def perimeter(self): """Calculated perimeter""" return (self.width+self.height) * 2 # Perimeter def area(self): """Calculated area""" return self.width * self.height
Step 2: create object - > constructor syntax is class name ()
rect = Rectangle(width=3, height=5) # When the position passes parameters, the rectangular object automatically calls _init__
Step 3: Send a message to the object
print(f'Perimeter of rectangle:{ rect.perimeter()}') # Perimeter of rectangle: 16 print(f'Area of rectangle:{ rect.area()}') # Area of rectangle: 15
Exercise 1: define a student class: name. Age. Eat, play, study
class Student: """student""" def __init__(self, name, age): """ Initialization method :param name:full name :param age: Age """ self.name = name self.age = age def eat(self): """having dinner""" print(f'{self.name}I am eating') def play(self): """play""" print(f'{self.name}Playing') def study(self, course_name):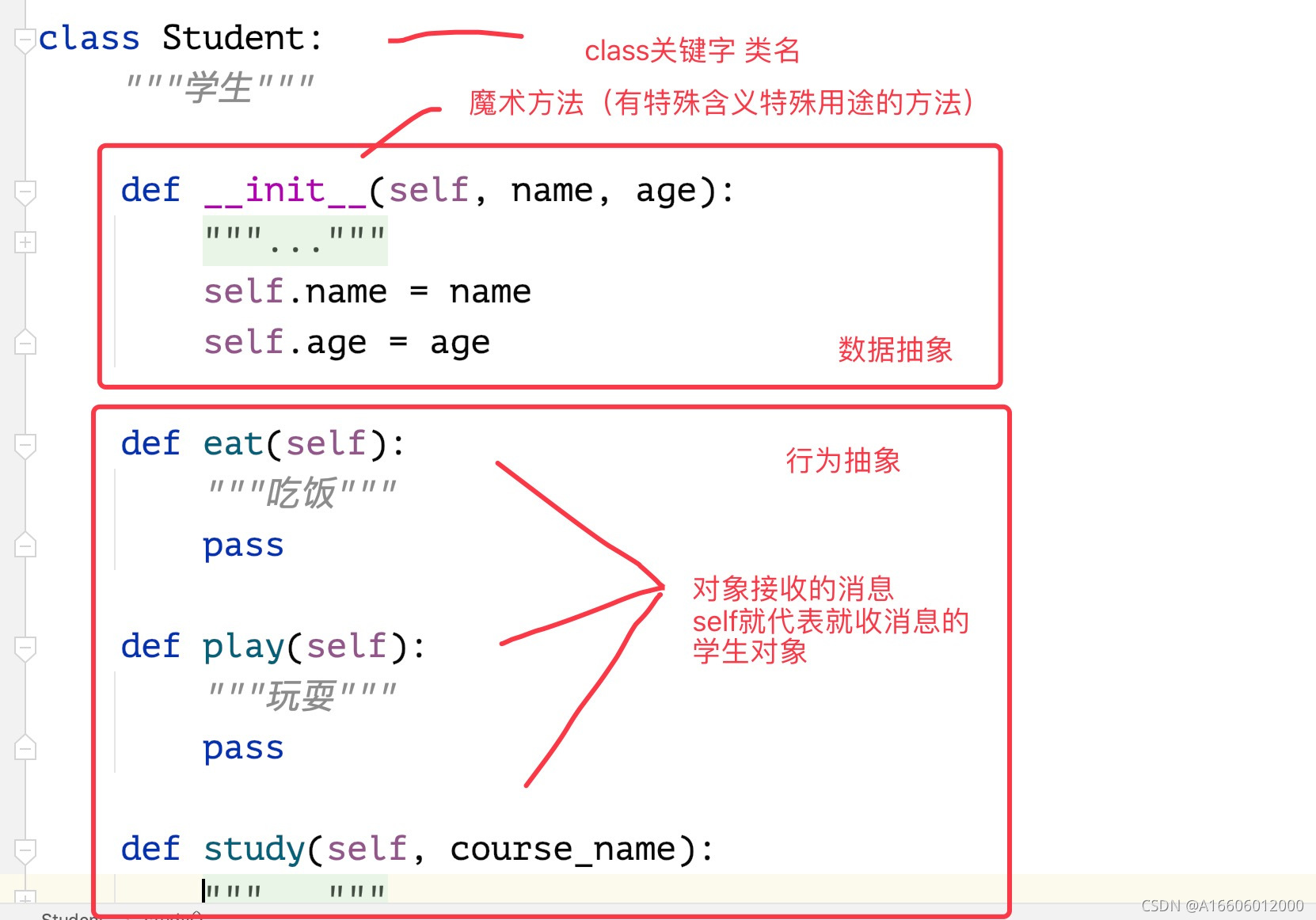 """ study :param course_name: Course name :return: """ print(f'{self.name}I am learning{course_name}') stu1 = Student('Chen Lai', 20) # Position transfer parameter stu2 = Student('Xiaolan', 17) stu1.play() stu1.eat() stu2.study('python study')
Exercise 2:
Object oriented programming: Exercise 2: the swimming pool is round, the radius is unknown, and there is a concentric circle corridor (3m wide) outside. The decoration is 28.5 per square meter and the enclosure is 38.2 per meter
Two objects
Three steps: define a class - > create an object - > solve the problem by sending a message to the object
# Own practice import math class Circle: def __init__(self, r): self.r = r def guodao(self): t1 = (3.14*(self.r+3)**2 - 3.14*(self.r**2))*28.5 return t1 def weiqiang(self): t2 = 2*3.14*(self.r+3)*38.2 return t2 rect = Circle(r=3) print(f'Expenses for corridor decoration:{rect.weiqiang()}element') print(f'Cost of wall decoration:{rect.guodao()}element') # teacher answer class Circle: def __init__(self, radius): self.radius = radius def perimeter(self): return 2 * math.pi * self.radius def area(self): return math.pi * self.radius ** 2 r = float(input('Please enter the radius of the swimming pool:')) c1, c2 = Circle(r), Circle(r+3) fence_price = c2.perimeter() * 38.2 aisle_price = (c2.area() - c1.area()) * 28.5 print(f'The cost of the aisle is:{aisle_price:.2f}element') print(f'The cost of the fence is:{fence_price:.2f}element')
Exercise 3:
Exercise 3: define the class to describe the digital clock: hour, minute and second, let it go and display the time
Three steps: define classes (data abstraction, behavior abstraction) - > create objects - > solve problems by sending messages to objects
Name of variable and function: snake_case
Class naming: CamelNotation
Multi summary, multi copy, English, typing speed
Job 1 countdown timer, countdown 1 minute. End at 00:00:00
import os import time class Clock: def __init__(self, hour=0, minute=0, second=0): self.hour = hour self.minute = minute self.second = second def go(self): self.second += 1 if self.second == 60: self.second = 0 self.minute += 1 if self.minute == 60: self.minute = 0 self.hour += 1 if self.hour == 24: self.hour = 0 def show(self): return f'{self.hour}When,{self.minute}Points,{self.second}second' # rect = Clock(2, 4, 20) # # while True: # print(f 'time display: {rect.show()}') # time.sleep(2) # rect.go() # teacher answer class Clock: def __init__(self, hour=0, minute=0, second=0, mode_12=False): """ Initialization method :param hour: Time :param minute: branch :param second: second """ self.hour = hour self.minute = minute self.sconde = second self.mode_12 = mode_12 #12 hours def show(self): #def show(self,mode_12=False):clock.show(True) """Display time""" # 07:01:02 if self.mode_12: if self.hour <12: return f'{self.hour:0>2d}:{self.minute:0>2d}:{self.sconde:0>2d} AM' else: hour = self.hour -12 if self.hour >12 else self.hour #Three eyes return f'{hour:0>2d}:{self.minute:0>2d}:{self.sconde:0>2d} PM' return f'{self.hour:0>2d}:{self.minute:0>2d}:{self.sconde:0>2d}' def run(self): """be in luck""" self.sconde += 1 if self.sconde == 60: self.sconde = 0 self.minute += 1 if self.minute == 60: self.minute = 0 self.hour += 1 if self.hour == 24: self.hour = 0 clock = Clock(12,12,12,mode_12=True) while True: # Call the system command (Terminal) through the system function of the os module # Clear screen: windows - > CLS / MacOS -- > # os.system('clear') print(clock.show()) time.sleep(1) clock.run()
Appendix to final document
1. Optimize import tool
[the external chain image transfer fails. The source station may have an anti-theft chain mechanism. It is recommended to save the image and upload it directly (img-dXRCvKv0-1634037226419)(C:\Users\z\Desktop\tupian.12].png)]
2. Color coding table
[the external chain image transfer fails. The source station may have an anti-theft chain mechanism. It is recommended to save the image and upload it directly (img-1RmQXax4-1634037226421)(C:\Users\z\Desktop\tupian.12.png)]
if self.hour == 24:
self.hour = 0
clock = Clock(12,12,12,mode_12=True)
while True:
#Call the system command (Terminal) through the system function of the os module
#Clear screen: windows - > CLS / MacOS – >
# os.system('clear')
print(clock.show())
time.sleep(1)
clock.run()
### Appendix to final document 1,Optimize import tool 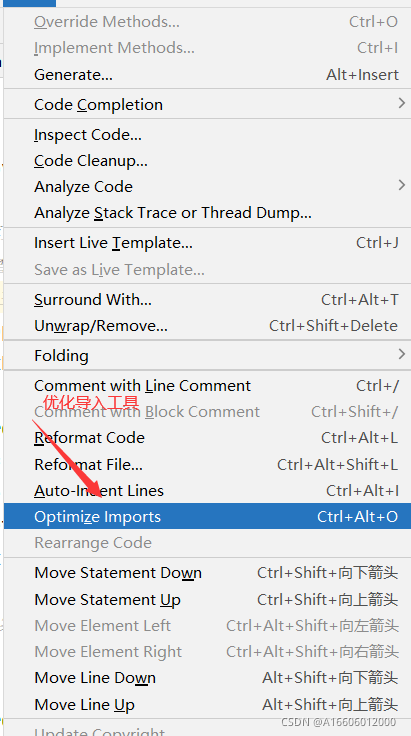 2,Color coding table 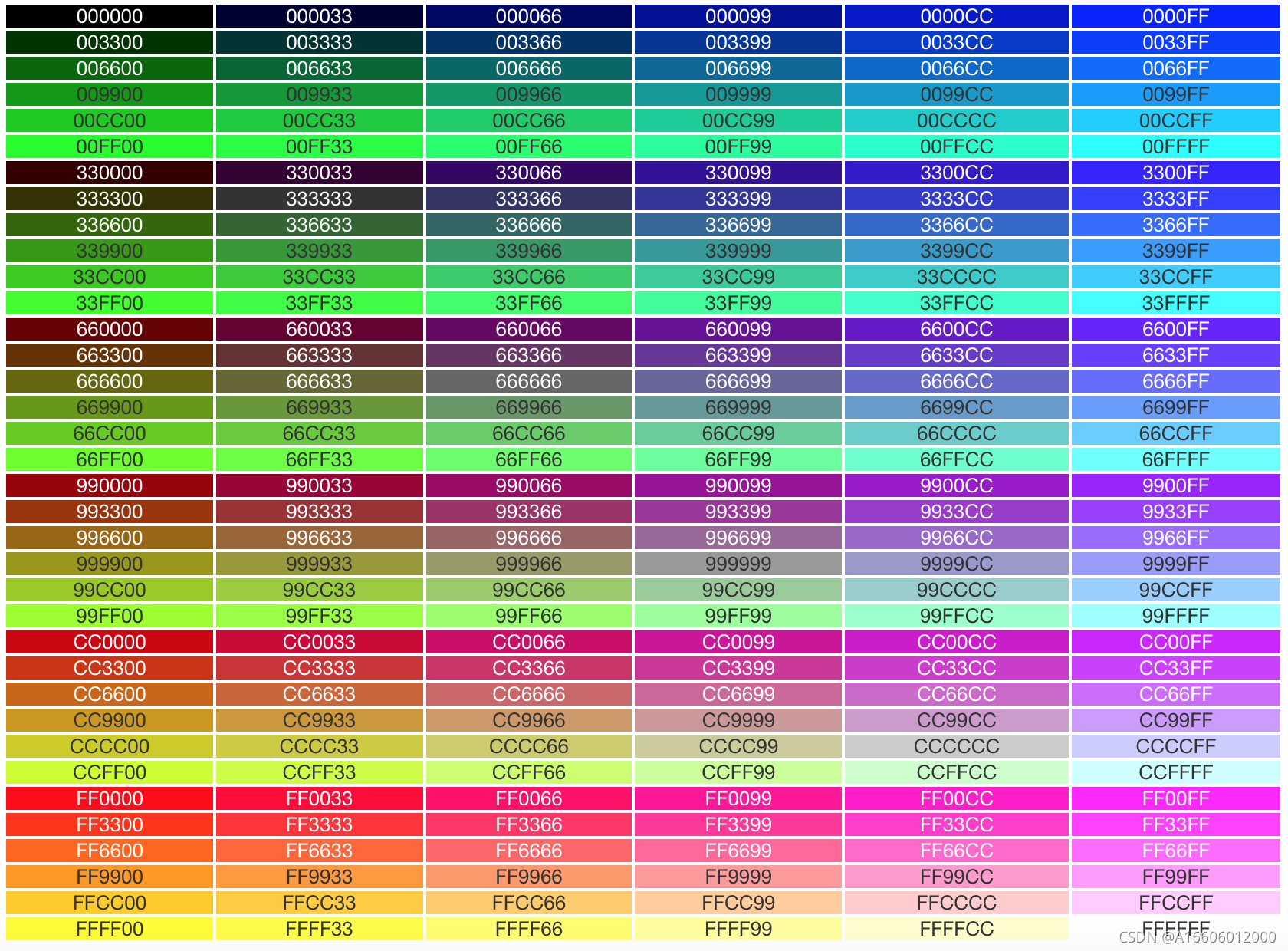