1, The solution prompt window flashes:
1. adopt system()Function solution: stay return 0;Previously added system("pause"); Function call. 2. With the help of VS Tool solution: On the project --->Right click ---> attribute ---> Configuration properties ---> Connector ---> system ---> Subsystem ---> Select console from the drop-down box (/SUBSYSTEM:CONSOLE)"
2, There are two ways to write helloworld:
1. With the help of VS Edit tool. Create project --> establish helloworld.c source file --> write helloworld program -->Ctrl + F5 Execution. 2. With the help of Notepad gcc Compiled by compiler tool. gcc Environment variable configuration of compilation tool: stay QT Found in the installation directory of gcc.exe Directory location. For example: C:\Qt\Qt5.5.0\Tools\mingw492_32\bin My computer -->attribute --> Advanced system settings --> environment variable -->System environment variable --> path --> take gcc.exe Directory location written to path In the value of. Create with Notepad helloworld.c Document - write in Notepad helloworld program --> use gcc Compilation tool, written in Notepad helloworld.c In the directory, execute gcc helloworld.c -o myhello.exe --> In the terminal (black window), run: myhello.exe
Single line note://
Multiline comment: / * comment content*/
Nesting is not allowed. Single rows can be nested in multiple rows.
3, system function:
Execute system commands, such as: pause,cmd,calc,mspaint,notepad..... system("cmd"); system("calc"); Screen clearing command: cls; system("cls");
4, gcc compilation 4 steps: [key points]
first deal with it briefly, and then translate it.
1. Preprocessing documents
gcc -E xxx.c -o xxx.i, - o is the file generated from xxx.c file, renamed xxx.i; or the file output from xxx.c file is xxx.i.
1.1 pretreatment works:
1)Expand the header file. --- Syntax errors are not checked. Any file can be expanded. 2)Macro definition replacement. --- Replace the macro name with the macro value. 3)Replace notes. --- Become a blank line 4)Expand conditional compilation --- Expand instructions based on conditions.
1.2 header file expansion: any file can be expanded
preprocessing will expand the included header file. You can expand any file. This file can be defined by yourself or the library file of the system.
(1) first create a new file, the file name is MyselfFW.h, and the content of the file is:
hhhhh 1111 112222
(2) include MyselfFW.h in the helloworld.cpp file
(3) preprocess the helloworld.cpp file with gcc -E helloworld.cpp -o helloworld.i to get the helloworld.i file. Then open helloworld.i to view it.
1.3 macro definition replacement
preprocessing replaces the macro name in the program with the macro value.
(1) define a macro in the program: PI 3.14
(2) use gcc -E helloworld.cpp -o helloworld.i preprocessing to view the helloworld.i file again. The PI is replaced with 3.14:
1.4 replacement notes
preprocessing will swap comments in the program for blank lines.
(1) write a few lines of comments in the program:
(2) use gcc -E helloworld.cpp -o helloworld.i preprocessing to view the helloworld.i file again. The comment is replaced with a blank line:
1.5 expand conditional compilation - expand instructions based on conditions
preprocessing expands instructions according to conditions.
(1) in the program, write a conditional compilation, which means that if PI is defined, the content in #ifdef... #endif will appear in the program. #endif is the end flag of the conditional compilation content
#include <stdio.h> #include "/oracle/heima/CBasics/day01/MyselfFW.h" #define PI 3.14 int main() { #ifdef PI printf("PI=%lf\n",PI); #endif printf("helloworld.\n"); return 0; }
(2) use gcc -E helloworld.cpp -o helloworld.i preprocessing to view the helloworld.i file again. printf("PI=%lf\n",PI); in the program:
(3) comment out #define PI 3.14, then preprocess it with gcc -E helloworld.cpp -o helloworld.i, and view the helloworld.i file again. printf("PI=%lf\n",PI); does not appear in the program:
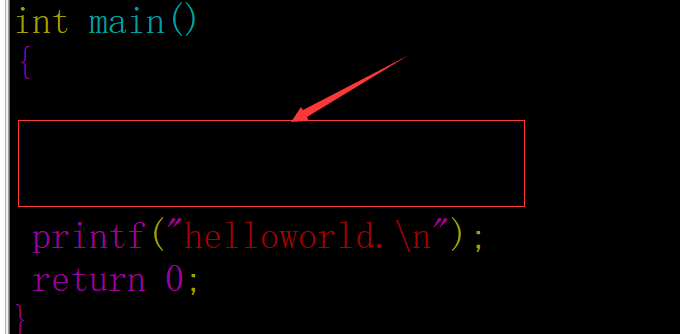
2. Compile - S xxx.s assembly file
gcc -S hello.i -o hello.s 1)Checking grammatical errors line by line is equivalent to translation. We must check the grammatical errors --- The most time-consuming process in the whole compilation 4 steps. 2)take C The program is translated into assembly instructions.s Assembly file.
2.1 check line by line for syntax errors
we remove the macro definition of PI, but Pi is also used in the program, which is wrong.
//#define PI 3.14 int main() { printf("PI=%lf\n",PI); printf("helloworld.\n"); return 0; }
(1) we deliberately make a mistake and still use the preprocessor, gcc -E helloworld.c -o helloworld.i.
(2) if the preprocessed program is compiled, an error will be reported. gcc -S helloworld.i -o helloworld.s. if the compilation is wrong, the helloworld.s file and subsequent target files will not be generated.
2.2 translating C program into assembly instructions
modify the program back, preprocess normally, and then compile. gcc -S helloworld.i -o helloworld.s. translate the C program into assembly instructions:
3. Compile -c xxx.o object file
gcc -c hello.s -o hello.o 1)Translation: translate assembly instructions into corresponding binary codes.
(1) Execute the assembly, translate the assembly instructions into the corresponding binary code, and generate the helloworld.o file
(2) View the helloworld.o file: there is a garbled code and the opening method is wrong.
4. Link xxx.exe executable.
Windows Below: gcc hello.o -o hello.exe Linux lower: gcc hello.o -o hello;no need.exe 1)Data segment merging 2)Data address backfill 3)Library introduction
5, VS debugger:
Add line number: tool-->option -->text editor-->C/C++ -->Line number selected. 1. Set breakpoints. F5 Start debugging 2. The stop position is the instruction that has not been executed. 3. Execute one sentence at a time( F11): Enter the function and execute tracking one by one. 3. Execute the following items one by one( F10): Do not enter the function, execute the program one by one. 4. Add monitoring: debugging -->window -->Monitor: enter the monitor variable name. Automatically monitor the change of variable value.
6, CPU internal structure and register
6.1 differences between 64 bit and 32-bit systems
Register is CPU The most basic internal storage unit CPU External communication is through the bus(Address, control, data)To interact with external devices, the width of the bus is 8 bits Time CPU The register is also 8 bits, so this CPU Just call eight CPU If the bus is 32 bits and the register is 32 bits, this CPU It's 32 bits CPU There is one CPU The internal register is 32 bits, but the bus is 16 bits, subject to 32 CPU All 64 bits CPU Compatible with 32-bit instructions, 32-bit should be compatible with 16 bit instructions, so in 64 bit CPU It is Can recognize 32-bit instructions In 64 bit CPU If a 64 bit software operating system is running on the architecture, the system is 64 bit In 64 bit CPU On the architecture, if a 32-bit software operating system is running, the system is 32-bit 64 Bit software cannot run on 32-bit CPU above
6.2 relationship among register, cache and memory
according to the distance from the CPU, the nearest is the register, then the cache (CPU cache), and finally the memory.
when calculating, the CPU reads the data to be used from the hard disk to the memory in advance, and then reads the data to be used to the register. Therefore, the CPU < --- > register < --- > memory, which is the information exchange between them.
then why is there a cache? Because if you often operate on the data at the same address in memory, it will affect the speed. Therefore, a cache is set between register and memory. Fetching from cache is much faster than memory. Of course, the price of cache must be much higher than that of memory. Otherwise, there will be no memory in the machine.
it can be seen that from a distance: CPU - > register - > cache - > memory.