User:Hello I'm the forest
Date:2018-03-16
Mark:Book " Python Programming from introduction to practice
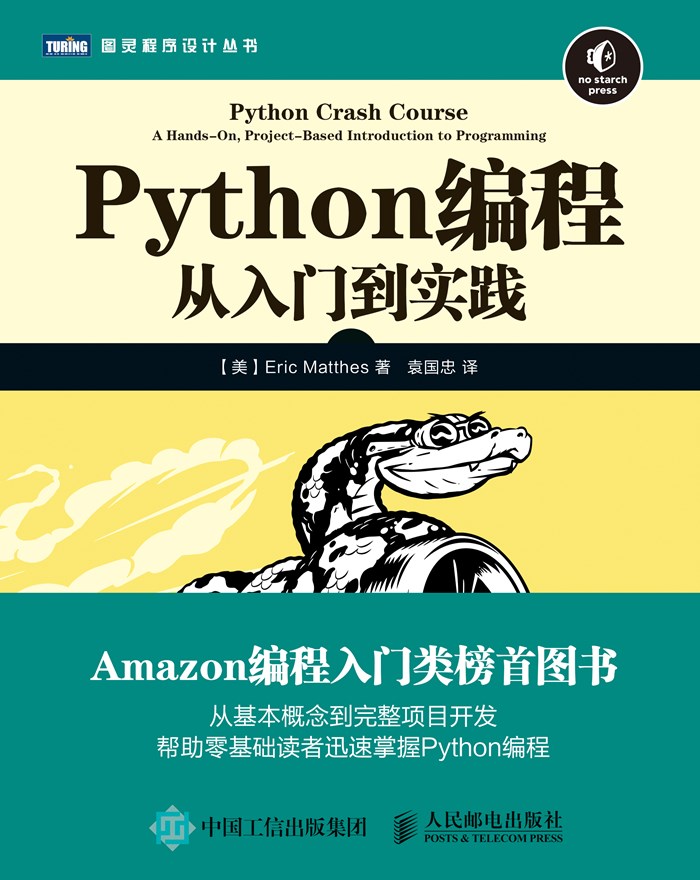
Chapter IV (II)
Use list
Section
To create a slice, specify the index of the first and last element to use. Like the function range(), Python stops after reaching the element before the second index you specify. To output the first three elements in the list, you need to specify indexes 0 to 3, which will output elements of 0, 1, and 2, respectively.
Code example:
players = ['charles', 'martina', 'michael', 'florence', 'eli'] print(players[0:3]) # Fetch from index 0 to the third end, and output the first three print(players[0:3]) # The output ends from index 1 to 4, print(players[:4]) # No index starts at the beginning of the list and ends at the specified index value print(players[2:]) # The index doesn't end until it ends print(players[-3:]) # The third from the bottom
Operation result:
['charles', 'martina', 'michael'] ['charles', 'martina', 'michael'] ['charles', 'martina', 'michael', 'florence'] ['michael', 'florence', 'eli'] ['michael', 'florence', 'eli']
Traversing slice
We used the for statement when traversing the list. Similarly, slicing can also be implemented with the for statement.
Code example:
players = ['charles', 'martina', 'michael', 'florence', 'eli'] print("Here are the first three players on my team:") for player in players[:3]: print(player.title())
Operation result:
Here are the first three players on my team: Charles Martina Michael
Copy slice
To copy a list, create a slice that contains the entire list by omitting both the start index and the end index ([:]). This lets Python create a slice that starts with the first element and ends with the last element, copying the entire list. In the process of copying, you can assign values or modify them. For example, add an element.
Code example:
my_foods = ['pizza', 'falafel', 'carrot cake'] friend_foods = my_foods[:] my_foods.append('cannoli') friend_foods.append('ice cream') print("My favorite foods are:") print(my_foods) print("\nMy friend's favorite foods are:") print(friend_foods)
Code result:
My favorite foods are: ['pizza', 'falafel', 'carrot cake', 'cannoli'] My friend's favorite foods are: ['pizza', 'falafel', 'carrot cake', 'ice cream']
tuple
Define tuple
The list is modifiable and is mainly suitable for storing data that may change during the running of the program. If you need to create immutable values, you need to use tuples, so tuples can be understood as: immutable list. But tuples are identified by square brackets. As with lists, you can use indexes to access elements.
Code example:
change_list = ('one','two','three','four') #Define a tuple print(change_list[0]) #Take value with tuple index of 0 change_list[1] = 'hello' #Changing the value of index 1 in a tuple will result in an error because tuples cannot be modified.
Traverse tuples
Traversal tuples and traversal lists are implemented with for.
The code is as follows:
change_list = (1,2,3,4) for number in change_list: print(number)
Operation result:
1 2 3 4
Change tuple variable
The variable of the modified tuple is not equal to the element of the modified tuple, that is, to assign a value to the variable of the stored tuple.
Code example:
change_list = (1,2,3,4) for number in change_list: print(number) change_list = (11,22,33,444) for number in change_list: print(number)
Operation result:
1 2 3 4 11 22 33 444