JSON(JavaScript Object Notation is a lightweight data exchange format. It is based on ECMAScript A subset. JSON uses a completely language-independent text format, but also uses habits similar to those of the C language family (including C,C++,C#,Java,JavaScript,Perl,Python And so on. These features make JSON an ideal data exchange language. Easy to read and write, but also easy to machine parse and generation (generally used to improve network transmission rate).
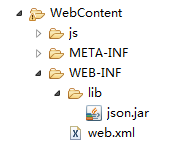
Transfer a single object:
Create a new servlet
package com.itnba.maya.a; import java.io.IOException; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.json.JSONObject; /** * Servlet implementation class C */ @WebServlet("/C") public class C extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public C() { super(); // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { request.setCharacterEncoding("utf-8"); response.setCharacterEncoding("utf-8"); //Simulated search from database Dog a=new Dog(); a.setName("Xiao Huang"); a.setAge(5); a.setZl("Siberian Husky"); JSONObject obj=new JSONObject(); obj.put("name", a.getName()); obj.put("age", a.getAge()); obj.put("zl", a.getZl()); JSONObject bb=new JSONObject(); bb.put("obj", obj); response.getWriter().append(bb.toString()); } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub doGet(request, response); } }
The results are as follows:
jsp page
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>Insert title here</title> <script type="text/javascript" src="js/jquery-1.11.1.min.js"></script> <script type="text/javascript"> $(document).ready(function(){ $("#k").click(function(){ $.ajax({ url:"C", data:{}, type:"POST", dataType:"JSON", success:function(httpdata){ $("#x").append("<li>"+httpdata.obj.name+"</li>"); $("#x").append("<li>"+httpdata.obj.age+"</li>"); $("#x").append("<li>"+httpdata.obj.zl+"</li>") } }) }); }); </script> </head> <body> <span id="k">See</span> <h1> <ul id="x"> </ul></h1> </body> </html>
The results are as follows:
Transfer sets or arrays:
servlet:
package com.itnba.maya.a; import java.io.IOException; import java.util.ArrayList; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.json.JSONArray; import org.json.JSONObject; /** * Servlet implementation class D */ @WebServlet("/D") public class D extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public D() { super(); // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { request.setCharacterEncoding("utf-8"); response.setCharacterEncoding("utf-8"); //Simulations are retrieved from the database Dog a1=new Dog(); a1.setName("Xiao Huang"); a1.setAge(5); a1.setZl("Siberian Husky"); Dog a2=new Dog(); a2.setName("Medium yellow"); a2.setAge(6); a2.setZl("Poodle"); Dog a3=new Dog(); a3.setName("Chinese rhubarb"); a3.setAge(7); a3.setZl("Pekingese"); ArrayList<Dog> list=new ArrayList<Dog>(); list.add(a1); list.add(a2); list.add(a3); JSONArray arr= new JSONArray(); //Ergodic set for(Dog d:list){ JSONObject obj=new JSONObject(); obj.put("name", d.getName()); obj.put("age", d.getAge()); obj.put("zl", d.getZl()); arr.put(obj); } response.getWriter().append(arr.toString()); } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub doGet(request, response); } }
The results are as follows:
jsp page:
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>Insert title here</title> <script type="text/javascript" src="js/jquery-1.11.1.min.js"></script> <script type="text/javascript"> $(document).ready(function(){ $("#k").click(function(){ $.ajax({ url:"D", data:{}, type:"POST", dataType:"JSON", success:function(httpdata){ for(var i=0;i<httpdata.length;i++){ var n=httpdata[i].name var a=httpdata[i].age var z=httpdata[i].zl var tr="<tr>" tr+="<td>"+n+"</td>" tr+="<td>"+a+"</td>" tr+="<td>"+z+"</td>" tr+="</tr>" $("#x").append(tr) } } }) }); }); </script> </head> <body> <span id="k">See</span> <h1> <table width="100%" id="x" border="1px"> </table> </h1> </body> </html>
The results are as follows: