Deterministic jump table |
---|
♣Definition of linked list
_About the definition of tables, here I will simply explain that tables are abstract like graphs and sets. data type . It is worth pointing out that each data type has its own operations, such as digit type redundancy.

/*Structural pointer * PtrToNode, and then PtrToNode XXXX can be used to define a structure, which is equivalent to struct Node *xxx. The operand on the left of the point operator is an expression of "result as structure"; The operand to the left of the arrow operator is a pointer to the structure. PtrToNode It saves a header address, (* Position).Element, and the result is the same as that of Postion - > Element. Here it is written as struct Node * type. Personal understanding should be that you want to read and write structural members with - > operator, so as to compare point operators. */ typedef struct Node *PtrToNode; //Create node data types to facilitate subsequent code use typedef PtrToNode List; //Create a linked list structure with [values] and [addresses of the next node] typedef PtrToNode Position; // struct Node { int Element; Position Next; };
I 1-2-3 deterministic jump table
- Property: The capacity of each clearance (except for possible zero clearance between head and tail) is 1, 2 or 3;

_Brief analysis: There are two gaps with capacity of 3 in the figure above: the first is three elements with height of 1 between 25 and 45, and the second is three elements with height of 2 between head and tail nodes.
II Code Implementation
-
Realization effect
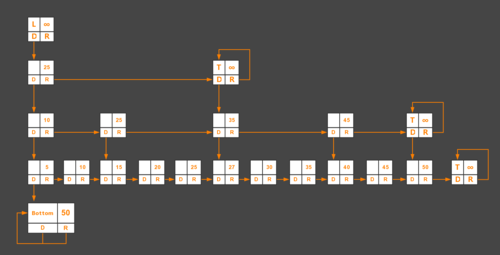
_Brief analysis: The initial point of the structure design is Top&Right; each node has a Right pointer and a Down pointer; the details will be analyzed below.
-
Structural type
#include <stdio.h> #include <stdlib.h> #Define Error (str) printf ("% s n", str), exit (1) // / defines an error termination operation function; #Definition Infinity (10000)// Infinity of Representation Graph: ___________ typedef int ElementType; // Define int alias ElementType for easy remembering typedef struct SkipNode *SkipList; //Define the struct SkipNode * type, which is used to create the header in the code. typedef struct SkipNode *Position; // The insertion operation generates new nodes, which are mainly created by Position. struct SkipNode { ElementType Element; SkipList Right; SkipList Down; }; static Position Bottom = NULL; /*Need to be initialized to empty*/ static Position Tail = NULL; /* Need to be initialized to empty*/
_Brief analysis: Obviously, to implement the linked list as shown in the figure, a structure needs three variables, namely, the data variable that saves the value, the pointer variable that points to the Next node and the pointer variable that points to Down. Tail is defined to determine whether the current node is located in order to facilitate programming; Bottom is a structure that temporarily stores values. Each insertion operation, each operation will be stored in Bottom first, and then the new node will transfer the values.
-
Initialization
SkipList Initialize() { SkipList L; if(Bottom == NULL ) { // Here is a knowledge point sizeof(Bottom)=4; sizeof(struct SkipNode)=12; Bottom = malloc( sizeof(struct SkipNode)); if(Bottom == NULL ) Error("out of space"); Bottom->Right = Bottom->Down = Bottom; Tail = malloc( sizeof(struct SkipNode)); if(Tail == NULL ) Error("out of space"); Tail->Element = Infinity ; Tail->Right = Tail; } /* Create Header Node */ L = malloc( sizeof( struct SkipNode ) ); if( L == NULL ) Error( "Out of space!!!" ); L->Element = Infinity; L->Right = Tail; L->Down = Bottom; return L; }
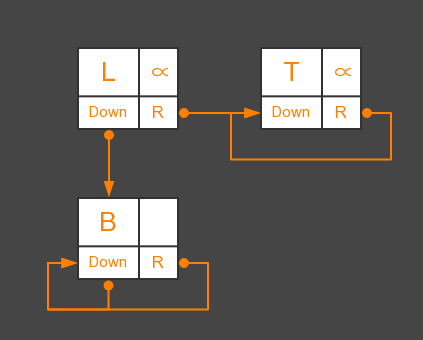
Brief analysis: Bottom - > Right, Bottom - > Down, Tail - > Right points to themselves; Tail - > Down = NULL; L is always the Header node in the program;
-
Insert operation
A key:
while(Item > Current->Element)
if( Current->Element > Current->Down->Right->Right->Element )
♥♥♥♥♥
SkipList Insert(ElementType Item, SkipList L) { Position Current = L; Position NewNode; Bottom->Element = Item; while( Current != Bottom ) { while(Item > Current->Element ) Current = Current->Right; //The following code is the key point if( Current->Element > Current->Down->Right->Right->Element ) { NewNode = malloc( sizeof( struct SkipNode )); if(NewNode == NULL ) Error("out of space "); NewNode->Right = Current->Right; NewNode->Down = Current->Down->Right->Right; Current->Right = NewNode; NewNode->Element = Current->Element; Current->Element = Current->Down->Right->Element; } else Current = Current->Down; } //Increase the height of the node if necessary (here it is necessary to ensure that the header is always at the top level) if(L->Right != Tail ) { NewNode = malloc( sizeof(struct SkipNode )); if(NewNode == NULL ) Error("out of space"); NewNode->Right = Tail; NewNode->Down = L; NewNode->Element = Infinity; L= NewNode; } return L; }
_Brief Analysis: Explanation of the above figure:
Variable analysis of___:
variable | L | C | B | D | R | N |
---|---|---|---|---|---|---|
Meaning | Root (top) node | Current node | Bottom (temporary) node | Down pointer | Right pointer | New node |
Firstly, put 5 in the temporary structure Bottom.
Conditional while (Item > Current - > Element) judgment is not valid;
Conditions if (Current - > Element > Current - > Down - > Right - > Right - > Element) hold;
Then new node N, exchange pointers, assign values;
Conditions if (L - > Right!= Tail) are established, new nodes exchange pointers and assign values;
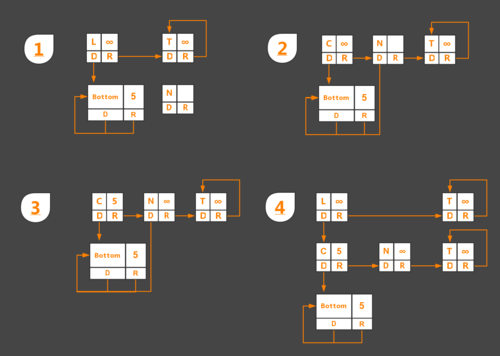
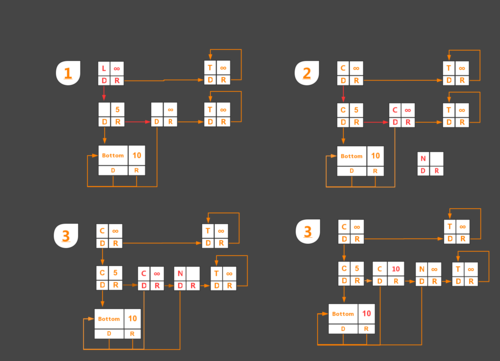
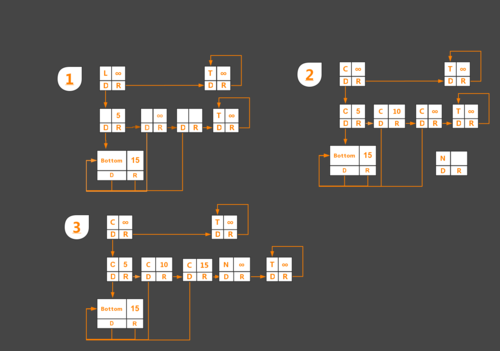

-
Lookup operation
Key points: while (item!= current - > Element)
♥♥♥
Position Find(ElementType item , SkipList L ) { Position current = L; Bottom->Element = item; while(item != current->Element) { if( item < current->Element ) { current = current->Down; } else current = current->Right; } return current; }
_Brief analysis: Understanding the insertion, for the search, no problem, relax and look at a few minutes to understand the basic. I started looking at the insertion and spent several A4 pages to compare it. It was also an IQ rush.*
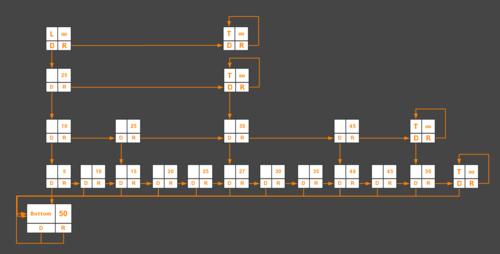
-
Learning ING
For the time being, Chapters 11 and 12 are very confused after reading "Data Structure and Algorithmic Analysis C Language Description" for half a month.····
As a standard mechanical man, he was not good at learning cutting, welding and milling. He devoted most of his three years of good time to programming from the beginning of his freshman year. He read more professional books on electronics and software than he did majoring in. I don't know whether it's a blessing or a curse. In fact, I want to ask such a question: a wide range of interests, no expertise, in the current social situation, is it a blessing or a curse?