2018-03-07
1.if
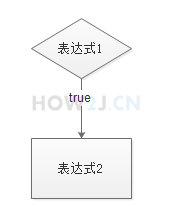
public class HelloWorld { public static void main(String[] args) { boolean b = true; //Print if it works yes if(b){ System.out.println("yes"); } } }
2. Multiple expressions and one expression
public class HelloWorld { public static void main(String[] args) { boolean b = false; //If there are multiple expressions, they must be enclosed in brackets if(b){ System.out.println("yes1"); System.out.println("yes2"); System.out.println("yes3"); } //Otherwise expression 23 regardless b Whether it is true Will execute if(b) System.out.println("yes1"); System.out.println("yes2"); System.out.println("yes3"); //If there's only one expression that doesn't need to be bracketed, it looks simpler. if(b){ System.out.println("yes1"); } if(b) System.out.println("yes1"); } }
3. pits that may be encountered in the use of if
In line 6, if is followed by a semicolon; and The semicolon is also a complete expression
If b is true, the semicolon is executed and yes is printed
If b is false, the semicolon is not executed and yes is printed
In this way, it seems that yes will be printed anyway.
public class HelloWorld { public static void main(String[] args) { boolean b = false; if (b); System.out.println("yes"); } }
4.if else
else stands for failure
public class HelloWorld { public static void main(String[] args) { boolean b = false; if (b) System.out.println("yes"); else System.out.println("no"); } }
5.else if
Other if is a multi-condition judgment
public class HelloWorld { public static void main(String[] args) { //If only used if,Four judgments will be executed int i = 2; if (i==1) System.out.println(1); if (i==2) System.out.println(2); if (i==3) System.out.println(3); if (i==4) System.out.println(4); //If used else if, Once the judgement is established in 18 rows, 20 rows and 22 rows of judgement will not be executed, saving computing resources. if (i==1) System.out.println(1); else if (i==2) System.out.println(2); else if (i==3) System.out.println(3); else if (i==4) System.out.println(4); } }
6.switch
The switch statement is equivalent to another expression of if El se. A switch can use byte,short,int,char,String,enum.
Note: There should be a break at the end of each expression.
Note: String was not supported before Java 1.7. Java has supported switch ing with String since 1.7. String is translated into hash value after compilation, but it is still an integer.
Note: enum is an enumeration type, in enumeration Chapters are explained in detail.
public class HelloWorld { public static void main(String[] args) { //If used if else int day = 5; if (day==1) System.out.println("Monday"); else if (day==2) System.out.println("Tuesday"); else if (day==3) System.out.println("Wednesday"); else if (day==4) System.out.println("Thursday"); else if (day==5) System.out.println("Friday"); else if (day==6) System.out.println("Saturday"); else if (day==7) System.out.println("Sunday"); else System.out.println("What kind of ghost is this?"); //If used switch switch(day){ case 1: System.out.println("Monday"); break; case 2: System.out.println("Tuesday"); break; case 3: System.out.println("Wednesday"); break; case 4: System.out.println("Thursday"); break; case 5: System.out.println("Friday"); break; case 6: System.out.println("Saturday"); break; case 7: System.out.println("Sunday"); break; default: System.out.println("What kind of ghost is this?"); } } }