Categories of code blocks:
Construct blocks of code. The brackets used to construct blocks of code must be in the member position (within the class, outside the method)
2. Local code block. Local block brackets are within the method. Function: Shorten the life cycle of local variables and save a little memory. Rarely used
3. Static code block. Static code blocks are executed when classes belonging to static code blocks are loaded into memory.
Application scenario of static code block: Later, it is mainly used to prepare the initialization of a project.
For example, read the database username and password from the configuration file.
Constructing code blocks: Unified initialization of all objects.
The function of the constructor: initialize the corresponding object.
Constructing a code block is called once when an object of the class to which the code block belongs is created.
Construct code block format:
{
Construct code blocks;
}
Note: The braces for building blocks of code must be in the member position (within the class, outside the method)
** * Author: Liu Zhiyong * Version: Version_1 * Date: 2016 10 April 14, 2001:28:49 * Desc: Construct code blocks, local code blocks */ class Boby { int id; String name; //Construct code blocks { System.out.println("The construction block is executed..."); cry(); } public Boby(){ System.out.println("The parametric-free construction method is executed..."); // cry(); } public Boby(int id, String name){ System.out.println("The parametric construction method is executed..."); // cry(); } public void cry(){ System.out.println("Wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow,"); } } class Demo38 { public static void main(String[] args) { //Local code block function: shorten the life cycle of local variables, save a little memory. Rarely used { new Boby(); Boby b1 = new Boby(22, "Dog baby"); System.out.println(b1.id+"#"+b1.name+"....................."); new Boby(33, "Duck's egg"); } System.out.println(b1.id+"#"+b1.name+"..............."; // There will be an error, and the local variable life cycle is over. } }
The braces of the local code block are within the method. Function: Shorten the life cycle of local variables and save a little memory. Rarely used
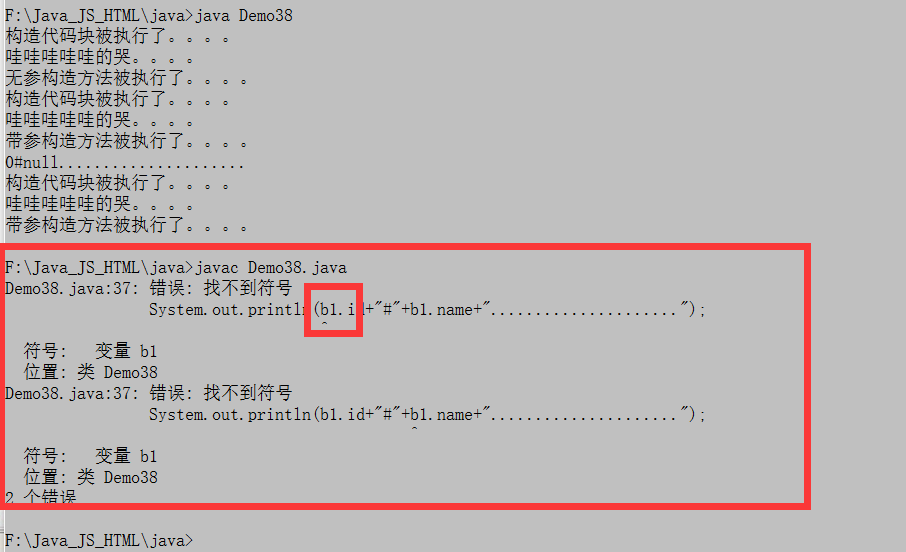
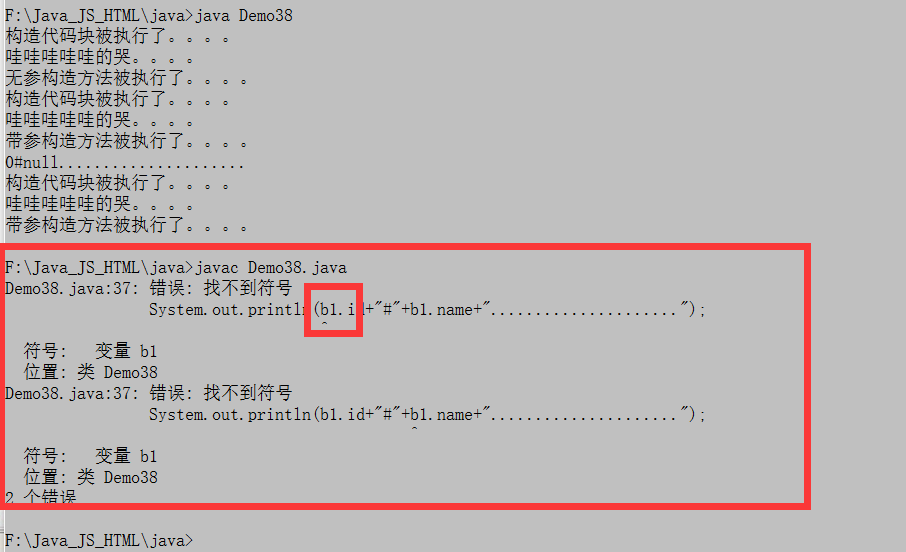
Notes to construct code blocks:
1. When a java compiler compiles a java source file, it brings the declaration statement of member variables to the front end of a class.
2. Initialization of member variables is actually performed in constructors.
3. Once compiled by the java compiler, the code block of the constructor block will be moved to the constructor to execute (using javap decompilation to observe the compiled results). It is executed before the constructor method, and the code in the constructor is executed finally.
4. The explicit initialization of member variables and the code block that constructs the code block are executed in the order of the current code.
Understand code 1:
/** * Author: Liu Zhiyong * Version: Version_1 * Date: 2016-4-14 10:55:59 * Desc: Notes to construct code blocks: 1.java When compiling a java source file, the compiler brings the declaration statement of member variables to the front end of a class. 2.Initialization of member variables is actually performed in constructors. 3.Once compiled by the java compiler, the block of code that constructs the block of code is moved to the constructor to execute. The code in the constructor is last executed. 4.The explicit initialization of member variables and the code block that constructs the code block are executed in the order of the current code. */ class Demo39 { public Demo39(){//Constructor Initializers i = 400; } //Debugging: Changing the order of member variables and building blocks to see the value of i int i = 22;//Membership variables display initialization.... Note that the location of the defined member variables is here. Why is there no error???? //Debugging: Changing the order of member variables and building blocks to see the value of i //Construct code block initialization { i = 100; } public static void main(String[] args) { Demo39 d = new Demo39(); System.out.println(d.i); } }
Understand code 2:
/** * Author: Liu Zhiyong * Version: Version_1 * Date: 2016 April 14, 2001, 10:28:49 * Desc: Construct code blocks, local code blocks */ class Boby { int id; String name; //Construct code blocks { cry(); } public Boby(){ } public Boby(int i, String n){ id = i; name = n; } public void cry(){ System.out.println(name + "Wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow, wow,"); } } class Demo38 { public static void main(String[] args) { Boby b1 = new Boby(22, "Dog baby"); System.out.println(b1.id+"#"+b1.name+"....................."); } }
Code 2 results:
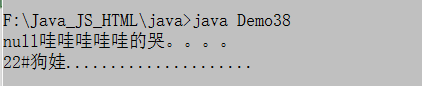