1.1 basic concept of Servlet
1.1.1 what is a Servlet
It is essentially a Java class that runs in Tomcat and is called by Tomcat. Function: generate a web page and output it to the browser.
1.1.2 differences between servlet and Java program
- A Servlet is essentially a Java class
- All servlets must implement the javax.servlet.Servlet interface
- It runs in the Tomcat container to receive the browser's request and respond.
1.2 implementation of Servlet
1.2.1 servlet 2.5 development
Create a class that inherits from HttpServlet Class, which has been implemented Servlet Interface. rewrite doGet or doPost Method to process the data sent by the browser get or post Request. to configure web/WEB-INF/web.xml Files, configuring servlet Access address for.
- Write a common class to implement the Servlet interface and directly inherit HttpServlet.
package cn.guardwhy; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; import java.io.PrintWriter; public class HelloServletDemo01 extends HttpServlet { @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // 1. Set the type and code of response content response.setContentType("text/html;charset=utf-8"); // 2. Get print stream PrintWriter out = response.getWriter(); // 3. Output text to browser out.print("<h3>Hello, Servlet!!!<h3>"); } @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { // super.doPost(req, resp); } }
2. Write Servlet mapping
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd" version="4.0"> <!--to configure Servlet--> <servlet> <!--servlet Name of--> <servlet-name>demo01</servlet-name> <!--to configure servlet Full name of class--> <servlet-class>cn.guardwhy.HelloServletDemo01</servlet-class> </servlet> <!--Configure access address--> <servlet-mapping> <servlet-name>demo01</servlet-name> <!--Access address must be in/start /Quite so web This root directory --> <url-pattern>/demo01</url-pattern> </servlet-mapping> </web-app>
1.2.2 access process

1.2.3 servlet 3.0 development
- Create a Servlet class and annotate @ WebServlet without configuring web.xml.
@WebServlet annotation | explain |
---|---|
name | The name of the Servlet, which is equivalent to < Servlet name > |
urlPatterns | Configure the access address, which is equivalent to < URL pattern > Multiple access addresses can be configured |
value | Is the access address, which is the same as urlPatterns. If only one value attribute is set, the name of value can be omitted |
2. Write a common class to implement the Servlet interface
package cn.guardwhy; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; import java.io.PrintWriter; @WebServlet("/demo02") public class HelloServletDemo02 extends HttpServlet { @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // 1. Set the type and code of response content response.setContentType("text/html;charset=utf-8"); // 2. Get print stream PrintWriter out = response.getWriter(); // 3. Output text to browser out.print("<h3>Hello, Servlet 3.0<h3>"); } @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { // super.doPost(req, resp); } }
results of enforcement
1.3 Servlet inheritance and Implementation

1.3.1 servlet interface
Basic concepts
The javax.servlet.Servlet interface is used to define the methods that all servlets must implement.
Common methods
Method declaration | effect |
---|---|
void init(ServletConfig config) | Called by the servlet container to indicate to the servlet that the servlet is being put into the service |
void service(ServletRequest req, ServletResponse res) | Called by the servlet container to allow the servlet to respond to requests |
void destroy() | It is executed when the Servlet is destroyed and when the server is shut down. |
ServletConfig getServletConfig( ) | Returns the ServletConfig object, which contains the initialization and startup parameters of this servlet. |
String getServletInfo() | Returns information about the servlet, such as author, version, and copyright |
1.3.2 GenericServlet class
Basic concepts
- javax.servlet.GenericServlet class is mainly used to define a general and protocol independent servlet, which implements the servlet interface.
- If you write a general servlet, you only need to rewrite the service abstract method.
Common methods
Method declaration | effect |
---|---|
abstract void service(ServletRequest req,ServletResponse res) | Invoked by the servlet container to allow the servlet to respond to requests. |
1.3.3 HttpServlet class
Basic concepts
- The javax.servlet.http.HttpServlet class is an abstract class and inherits the GenericServlet class.
- Used to create an HTTP Servlet for a web site. Subclasses of this class must override at least one method.
Common methods
Method declaration | effect |
---|---|
void doGet(HttpServletRequest req,HttpServletResponse resp) | Process GET requests from clients |
void doPost(HttpServletRequest req,HttpServletResponse resp) | Handle POST requests from clients |
void destroy() | Free resources when deleting instances |
void init() | Perform initialization |
void service(HttpServletRequest req,HttpServletResponse resp) | Decide whether to call the doGet or doPost method according to the request |
1.4 Servlet life cycle
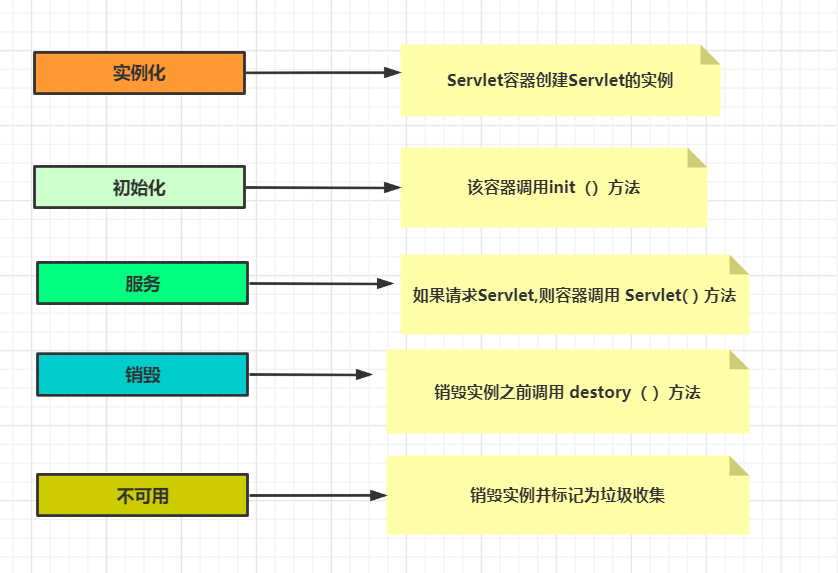
- The constructor is called only once. When the Servlet is requested for the first time, the constructor is called to create an instance of the Servlet.
- The init method is called only once. When the Servlet instance is created, this method is called immediately to initialize the Servlet.
- The service method is called many times. Whenever there is a request, the service method will be called to respond to the request.
- The destroy method is called only once, and when the Web application of the Servlet instance is unloaded, the method is called to release the resources currently occupied.
1.5 principle of Servlet
Servlet is called by the web server, which implements the following steps after receiving the browser request
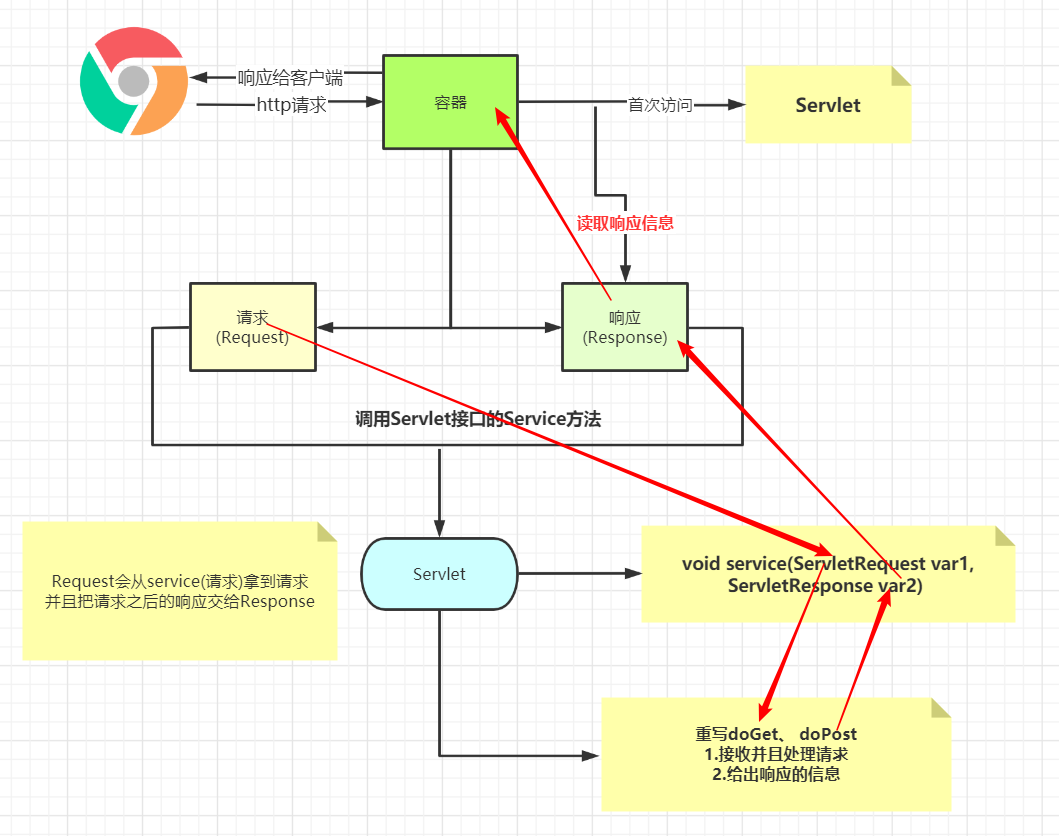