welcome to the front end technology camp! If you are also a front-end learner or have the idea of learning from the front-end, follow me to attack the front-end from scratch.
we are committed to introducing front-end knowledge and our own shortcuts as detailed and concise as possible, which is also a record on the way of learning. Welcome to explore
1, Data type
concept
The storage space occupied by different data is different. In order to divide the data into data with different memory sizes and make full use of the storage space, different data types are defined
Data type of variable
JavaScript is a weakly typed or dynamic language, which means that there is no need to declare the type of variables in advance, and the type will be determined automatically during program operation
classification
Data types are divided into simple data types and complex data types. Let's talk about simple data types first.
Simple data types include Number, Boolean, String, Undefined, and Null.
Number, default value: 0: numeric type, including integer value and floating-point value, such as 20 and 0.2
Digital hexadecimal: octal 0-7. Add 0 in front of the number in the program to represent octal;
hexadecimal 0-9 a-f program, preceded by 0x, indicates hexadecimal
Digital range:
//Maximum: console.log(Number.MAX_VALUE); //Minimum: console.log(Number.MIN_VALUE);
Three special values:
//Infinity console.log(Number.MAX_VALUE * 2); console.log(Infinity); //Infinitesimal console.log(-Number.MAX_VALUE * 2); console.log(-Infinity); //Non numeric, alert (NaN); Represents a non numeric value console.log('fds' - 12); //IsNaN is used to judge non numbers. The IsNaN () method judges non numbers and returns a value. If it is a number, it returns false, otherwise it returns true console.log(isNaN(12)); console.log(isNaN('Ngege'));
Boolean, default value: false, Boolean value type. There are two values: true and false, which are equivalent to 1 and 0
When Boolean and numeric types are added, the value of true is 1 and the value of false is 0:
console.log(true+1);//2 console.log(false+1);//1
String," " :
1. String type, such as "Zhang San". Note that the string in js is quoted, single and double quotation marks are OK. Single quotation marks are recommended
2.JS can use single quotation marks to nest double quotation marks, or double quotation marks to nest single quotation marks, and cannot mix and match
3. String escape character: String transfer character, special character, starting with \, written in quotation marks
\n: Newline, n means newline | \: slash\ |
---|---|
\': single quotation mark | \": double quotation marks |
\t: tab indent | \b: B means blank |
4. String length and splicing:
Length: a string is composed of several characters. The number of characters is the length of the string. The length of the whole string can be obtained through the length property of the string
//Detection string length var str = 'my name is gege'; console.log(str.length); // Display 15
Splicing: multiple strings can be spliced with +, that is, string + any type = new string after splicing
//String splicing, numerical addition, character concatenation, or variable splicing. Variables cannot be quoted console.log('I am'+'Your father'); console.log('I this year'+18+'year');
Undefined, default: undefined
var a; declares the variable a but does not give a value. In this case, a= undefined
//Undefined undefined, declared variable not assigned var str1; console.log(str1);//undefined var str2 = undefined; console.log(str2+'Mr. ness');//Mr. undefined ness console.log(str2+1);//NaN undefined is added to the number and the result is NaN
Null, default: null
var a = null; //Declared that variable a is null var str4 = null; console.log(str4+1);//1
Detection variable data type: typeof
var str5 = 20; console.log(typeof str5);//The test result is: number //The value from prompt is character type var age1 = prompt('Please enter age:'); console.log(age1); console.log(typeof age1);//string
Literal quantity: it is the representation of a fixed value in the source code, that is, how the literal quantity expresses this value
Number literal: 1, 20, 3
String literal: 'big front end'
Boolean literal: true, false
Data type conversion
Data type conversion: convert a variable of one data type to another
Convert to string type

// 1. Variable. toString () var str = num.toString(); // 2.String (variable) console.log(String(num));
Convert to numeric
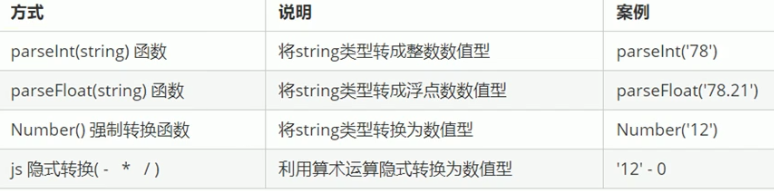
// ParseInt (variable): rounding, the unit will be removed, and NaN will not be displayed console.log(parseInt(age)); //Parseintfloat (variable): decimal, floating-point number, unit will be removed, and NaN will not be displayed console.log(parseFloat('30.25'));//30.25 //Using arithmetic operation - * / js has implicit conversion console.log('12'-0);//12 console.log('10'*2);//20
Convert to Boolean
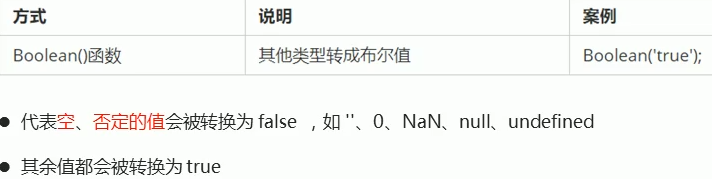
console.log(Boolean(''));//false console.log(Boolean('12'));//true console.log(Boolean('I am N Mr. ness'));//true
2, Operator
concept
Operators, also known as operators, are symbols used to assign, compare, and perform arithmetic operations
Expression: an expression composed of numbers, operators, variables, etc
The expression will eventually have a result, which will be returned to us, called the return value. In the program, calculate the right first, calculate the expression on the right, and return the return value to the left
Arithmetic operator
With priority, multiply and divide first, then add and subtract, and parentheses first
Floating point numbers will have problems in arithmetic operations, so try to avoid using decimals
Floating point numbers cannot be directly compared for equality
//Take a chestnut console.log(1*2);//2 console.log(1/2);//0.5 // %Surplus (mold taking) console.log(4%2);//0 //Floating point numbers cannot be directly compared for equality var num = 0.1+0.2; console.log(num == 0.3);
Increment decrement operator
In JavaScript, increment (+ +) and decrement (–) can be placed in front of or behind a variable. When they are placed in front, they are called pre increment (decrement) operators; when they are placed behind, they are called post increment (decrement) operators. However, they must be used with variables
Prepositional increment: + + num prepositional increment operator is to add 1, add 1 first, and then return the value, similar to num = num +1
If the pre auto increment and post auto increment are used separately, the effect is the same
// ++The increment operator before num is self adding 1, which is similar to num = num +1 var age = 10; ++age; ++age console.log(age);//12
Post auto increment: return the original value first, and then add 1 automatically
//num + + Post auto increment: first return the original value, and then add 1 var a = 10; console.log(a++ + 10);//20 console.log(a);//11
Comparison operator
Also called relational operator, it is the operator used when comparing two data, and then a Boolean value will be returned as the result of the comparison operation
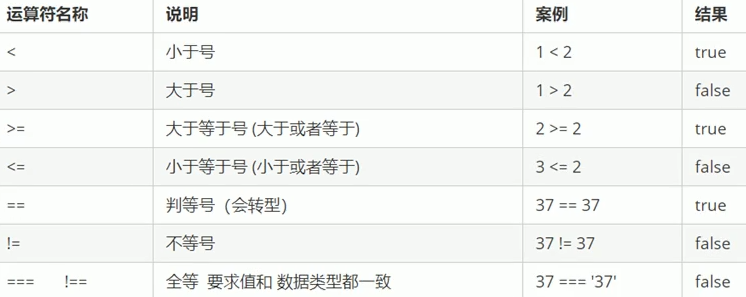
The equals sign is = =, which converts the data type by default and converts the string type to the number type
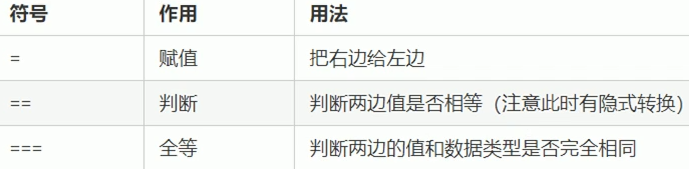
//Take a chestnut console.log(1<=2); console.log(12==12); console.log(12!=12);//false //The equals sign is = = the default conversion data type will convert the string type to the number type console.log(12=='12'); //true //As like as two peas = = = = data type and value are exactly the same. console.log(12==='12');//false
Logical operator
The operator used for Boolean operation. The return value is also Boolean. It is often used to judge multiple conditions
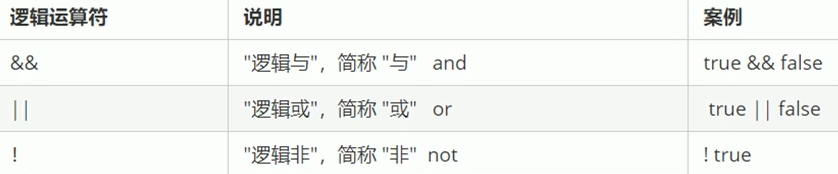
// Logic and & & and one false is false, and all true is true console.log(1>2 && 2>1);//false console.log(1<2 && 2>1);//true // Logical or | or, one true is true and all false is false console.log(2>1 || 3<2);//true console.log(1>2 || 2<1);//false // Logical not! Not console.log(!true);//false
Short circuit operation (logic interrupt): when there are multiple expressions (values) and the expression on the left can determine the result, the value of the expression on the right will not continue to be calculated
Logic and syntax: expression 1 & & expression 2
If the value of the first expression is true, expression 2 is returned
If the value of the first expression is false, the expression 1 is returned
If the empty or negative is false, 0 '' null undefined NaN, and the rest are true
console.log(123 && 456);//456 console.log(0 && 456);//0 console.log(0 && 1 + 2 && 456);//0
Logical or: Syntax: expression 1 | expression 2
Logical or returns expression 1 if the result of expression 1 is true
If the result of expression 1 is false, expression 2 is returned
console.log(123 || 456);//123 console.log(0 || 456 || 123);//456
Assignment Operators
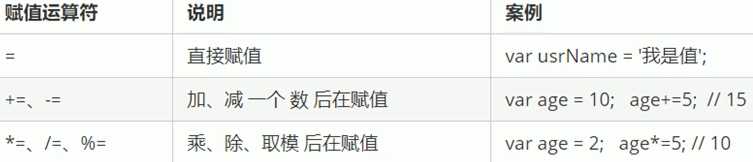
var num = 10; num = num + 2;//Namely: num += 2; num += 3; console.log(num);// 15
Operator priority
You can try the ox knife:
console.log( 4>=6 || 'people' != 'Avatar' && !(12 * 2 == 144) && true);//true var num = 10; console.log( 5 == num / 2 && (2 + 2 * num).tostring() === '22');//true
3, Interview questions
1, How to change the point of this pointer?
You can use the apply, call and bind methods to change the direction of this (without changing the scope of the function). The comparison is as follows:
(1) The first parameter of the three is the object to which this points, that is, the context to be specified. The context refers to the object calling the function (if not, it points to the global window);
(2) The second parameters of apply and bind are arrays, and call receives multiple parameters separated by commas;
(3) apply and call only change the original function, and bind will return a new function (you have to call it again to take effect).
2, How to understand synchronous and asynchronous?
Synchronization: a mode in which processing instructions are executed one by one according to the code writing order. The next code can be executed only after the previous code is executed.
Asynchrony: it can be understood as a way of parallel processing. You can perform other tasks without waiting for a program to finish executing.
The reason why JS needs to be asynchronous is that JS runs in a single thread. Common asynchronous scenarios include timer, ajax request and event binding.
This issue ends here. Thank you for reading! If you have any questions, leave a message and reply in time
- Point praise and attention, and continuously update practical skills, popular resources, software tutorials, etc
- If you have any software, film and television tutorial resources, textual research materials and other needs, just leave a message