ESLint
whether it's a multi person collaboration or a personal project, code specification is very important. This can not only avoid basic syntax errors to a great extent, but also ensure the readability of the code.
preparation
1, VSCode ESLint plug in
The ESLint plug-in is used to display some error prompts in the editor according to the. eslintrc.js configuration file of the project directory.
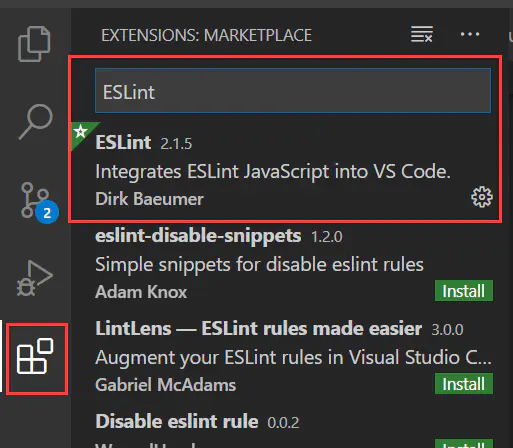
2, Create project based on Vue cli
The specific steps are as follows:
-
Create project
-
Select Linter/Formatter when creating (selected by default)
-
Next, select ESLint with error prevention only
-
Next, select Lint on save (check according to the code format after editing and saving)
-
Next, select in dedicated config files
-
The rest of the steps default to the next step, which is to complete the project creation
Configuration item
All configuration files are in. eslintrc.js. By default, plugin:vue/essential is used to verify the code. Of course, you can also select the strictest plugin:vue/recommended to verify the code.
module.exports = { extends: ['plugin:vue/recommended', 'eslint:recommended'] //You can change it to extensions: ['plugin: Vue / essential ','eslint: recommended'] }
eslint rules
Default eslint rule:
- Semicolon cannot be added at the end of code
- Cannot have multiple blank lines in code
- The tab key cannot be used. It must be replaced with two spaces
- Variables declared but not used cannot exist in code
Custom rules
If the default eslint rules don't fit your habits, you can customize them. Find the configuration file named. eslintrc.js, where the rules are configuration rules.
rules: { 'no-console': process.env.NODE_ENV === 'production' ? 'warn' : 'off', 'no-debugger': process.env.NODE_ENV === 'production' ? 'warn' : 'off' }
Common rules are as follows:
rules: { 'vue/max-attributes-per-line': [ 2, { singleline: 10, multiline: { max: 1, allowFirstLine: false } } ], 'vue/singleline-html-element-content-newline': 'off', 'vue/multiline-html-element-content-newline': 'off', 'vue/name-property-casing': ['error', 'PascalCase'], 'vue/no-v-html': 'off', 'accessor-pairs': 2, //Using getter/setter in objects 'arrow-spacing': [ 2, { before: true, after: true } ], //=>Parentheses before / after 'block-spacing': [2, 'always'], //Block needs space // The {after if while function must be on the same line as if, java style. 'brace-style': [ 2, '1tbs', { allowSingleLine: true } ], //Mandatory hump naming camelcase: [ 0, { properties: 'always' } ], // Array and object key value pair last comma, never parameter: cannot have comma at the end, always parameter: must have comma at the end, // Always multiline: multiline mode must have comma, single line mode cannot have comma 'comma-dangle': [2, 'never'], // Control spaces before and after commas 'comma-spacing': [ 2, { before: false, after: true } ], 'comma-style': [2, 'last'], // Controls whether commas appear at the end of a line or at the beginning of a line // Forces the parent constructor to be called with super() in the subclass constructor, and the compiler of typeflip will also prompt 'constructor-super': 2, curly: [2, 'multi-line'], // Enforce consistent bracket style for all control statements // Force the position of. In object.key. Parameters: // Property, the '.' number should be on the same line as the property // Object, the '.' number should be on the same line as the object name 'dot-location': [2, 'property'], 'eol-last': 2, // Force wrap at end of file eqeqeq: ['error', 'always', { null: 'ignore' }], // Use = = = instead== 'generator-star-spacing': [ 2, { before: true, after: true } ], //Space before and after generator function * 'handle-callback-err': [2, '^(err|error)$'], //nodejs processing error indent: [ 2, 2, { SwitchCase: 1 } ], //Indent style 'jsx-quotes': [2, 'prefer-single'], // Use double or single quotes consistently in JSX properties //Spaces before and after colons in object literals 'key-spacing': [ 2, { beforeColon: false, afterColon: true } ], 'keyword-spacing': [ 2, { before: true, after: true } ], //The function name must be called in new mode in upper case and in lower case without new mode in the first line 'new-cap': [ 2, { newIsCap: true, capIsNew: false } ], 'new-parens': 2, //new must be parenthesized 'no-array-constructor': 2, //Array constructor prohibited 'no-caller': 2, //Prohibit using arguments.caller or arguments.call 'no-console': 'off', //Do not use console 'no-class-assign': 2, //Prohibit assigning to class 'no-cond-assign': 2, //Prohibit using assignment statements in conditional expressions 'no-const-assign': 2, //Variables declared by const cannot be modified 'no-control-regex': 0, //Prohibit using control characters in regular expressions 'no-delete-var': 2, //You cannot use the delete operator on a var declared variable 'no-dupe-args': 2, //Function arguments cannot be repeated 'no-dupe-class-members': 2, //Duplicate declarations are not allowed in a class 'no-dupe-keys': 2, //Key repetition {a:1,a:1} is not allowed when creating object literals 'no-duplicate-case': 2, //case labels in switch cannot be duplicate 'no-empty-character-class': 2, //[] content in regular expression cannot be empty 'no-empty-pattern': 2, // Disable empty deconstruction mode 'no-eval': 2, //No eval 'no-ex-assign': 2, //Forbid the assignment of exception parameter in catch statement 'no-extend-native': 2, //Disable extending native objects 'no-extra-bind': 2, //Disable unnecessary function binding 'no-extra-boolean-cast': 2, //Disable unnecessary bool conversion 'no-extra-parens': [2, 'functions'], //Do not allow unnecessary brackets 'no-fallthrough': 2, //Disable switch penetration 'no-floating-decimal': 2, //It is forbidden to omit 0.53 in floating-point numbers 'no-func-assign': 2, //Prohibit duplicate function declaration 'no-implied-eval': 2, //Do not use implicit eval 'no-inner-declarations': [2, 'functions'], //Prohibit the use of declarations (variables or functions) in block statements 'no-invalid-regexp': 2, //Suppress invalid regular expressions 'no-irregular-whitespace': 2, //Can't have irregular spaces 'no-iterator': 2, //Disable use of the iterator property 'no-label-var': 2, //The label name cannot be the same as the variable name declared by var 'no-labels': [ //Prohibited label declaration 2, { allowLoop: false, allowSwitch: false } ], 'no-lone-blocks': 2, //Suppress unnecessary nested blocks 'no-mixed-spaces-and-tabs': 2, //Do not mix tab s and spaces 'no-multi-spaces': 2, //Can't use extra space 'no-multi-str': 2, //String cannot wrap with 'no-multiple-empty-lines': [ //No more than 1 empty line 2, { max: 1 } ], 'no-native-reassign': 2, //Cannot override native object 'no-negated-in-lhs': 2, //The left side of the in operator cannot have! 'no-new-object': 2, //Do not use new Object() 'no-new-require': 2, //Do not use new require 'no-new-symbol': 2, //Do not use new symbol 'no-new-wrappers': 2, //Do not use new to create a wrapper instance, new String new Boolean new Number 'no-obj-calls': 2, //You cannot call built-in global objects, such as Math() JSON() 'no-octal': 2, //Octal numbers are prohibited 'no-octal-escape': 2, //Octal escape sequence prohibited 'no-path-concat': 2, //You cannot use dirname or filename for path splicing in node 'no-proto': 2, //Disable use of proto attribute 'no-redeclare': 2, //Do not declare variables repeatedly 'no-regex-spaces': 2, //Prohibit multiple spaces / foo bar in regular expression literals/ 'no-return-assign': [2, 'except-parens'], //The return statement cannot have an assignment expression 'no-self-assign': 2, //Self distribution 'no-self-compare': 2, //Can't compare themselves 'no-sequences': 2, //Do not use comma operator 'no-shadow-restricted-names': 2, //The restriction identifier specified in strict mode cannot be used as the variable name when declaring 'no-spaced-func': 2, //There can be no space between function name and () when a function is called 'no-sparse-arrays': 2, //Disallow sparse array, [1,, 2] 'no-this-before-super': 2, //this or super cannot be used until super() is called 'no-throw-literal': 2, //It is forbidden to throw literal error throw "error"; 'no-trailing-spaces': 2, //No spaces after the end of a line 'no-undef': 2, //Cannot have undefined variables 'no-undef-init': 2, //Variable initialization cannot be directly assigned as undefined 'no-unexpected-multiline': 2, //Avoid multiline expressions 'no-unmodified-loop-condition': 2, //Check whether the reference is modified in the loop 'no-unneeded-ternary': [ // Prohibit the use of ternary operators when there are simpler alternative expressions 2, { defaultAssignment: false } ], 'no-unreachable': 2, //Can't have code that can't be executed 'no-unsafe-finally': 2, // The negative operator is prohibited for the left operand of the relational operator. The original no-connected-in-lhs has been replaced by this 'no-unused-vars': [ //Cannot have variables or parameters that are not used after declaration 2, { vars: 'all', args: 'none' } ], 'no-useless-call': 2, //Do not call or apply unnecessarily 'no-useless-computed-key': 2, //There is no need to use calculation properties with text 'no-useless-constructor': 2, //Class constructors that can be safely removed without changing the way a class works 'no-useless-escape': 0, // Disable unnecessary escape characters 'no-whitespace-before-property': 2, // Blank before prohibited attribute 'no-with': 2, //Disable with 'one-var': [ //declaration of continuity 2, { initialized: 'never' } ], 'operator-linebreak': [ //Is the operator at the end of a line or at the beginning of a line when wrapping 2, 'after', { overrides: { '?': 'before', ':': 'before' } } ], 'padded-blocks': [2, 'never'], //Whether the first and last line of a block statement should be empty quotes: [ //Quote type ` ` '' ' 2, 'single', { avoidEscape: true, allowTemplateLiterals: true } ], semi: [2, 'never'], //Statement force semicolon end 'semi-spacing': [ //Space before and after semicolon 2, { before: false, after: true } ], 'space-before-blocks': [2, 'always'], //Do you want a space in front of the block {that does not start with a new line 'space-before-function-paren': [2, 'never'], //Do you want spaces before parentheses when defining functions 'space-in-parens': [2, 'never'], //Do you want spaces in parentheses 'space-infix-ops': 2, //Do you want spaces around infix operators 'space-unary-ops': [ //Do you want spaces before / after unary operators 2, { words: true, nonwords: false } ], 'spaced-comment': [ //Comment style, no spaces or anything 2, 'always', { markers: ['global', 'globals', 'eslint', 'eslint-disable', '*package', '!', ','] } ], 'template-curly-spacing': [2, 'never'], // Requires or disables the use of spaces around embedded expressions in Template Strings 'use-isnan': 2, //NaN is prohibited for comparison, only isNaN() 'valid-typeof': 2, //Legal typeof value must be used 'wrap-iife': [2, 'any'], //Bracket style for immediate function expression execution 'yield-star-spacing': [2, 'both'], // Force spaces around * in yield * expressions yoda: [2, 'never'], //Prohibited Yoda conditions 'prefer-const': 2, //First choice const 'no-debugger': process.env.NODE_ENV === 'production' ? 2 : 0, //Disable debugger 'object-curly-spacing': [ //Allow unnecessary spaces in braces 2, 'always', { objectsInObjects: false } ], 'array-bracket-spacing': [2, 'never'] //Allow extra space in non empty array }
Note: if you do not extract the eslint configuration file when creating a project, you can configure eslint in the package.json file.
"eslintConfig": { root: true, env: { node: true }, 'extends': [ 'plugin:vue/essential', 'eslint:recommended' ], parserOptions: { parser: 'babel-eslint' }, rules: { 'no-console': process.env.NODE_ENV === 'production' ? 'warn' : 'off', 'no-debugger': process.env.NODE_ENV === 'production' ? 'warn' : 'off' } }
Cancel ESLint verification
1, Projects created by vue-cli2
The simplest way is to comment out the eslint rules in the build/webpack.base.conf.js configuration file.
2, Projects created by vue-cli3
- Just comment out the configuration of eslint in the package.json file
- vue.config.js sets the following three to false
devServer: { overlay: { warnings: false, errors: false }, } lintOnSave: false