catalogue
1, Preliminary cognition of class and object
2, Class and class instantiation
3.1. Member variables / attributes
(1) Call the member variable through the this keyword
(2) Calling member methods with the this keyword
(3) this keyword calls the constructor
1. How to realize encapsulation
1, Preliminary cognition of class and object
2, Class and class instantiation
1. Basic syntax of class
class Person { public int age;//Member properties public String name; public String sex; public void eat() {//Member method System.out.println("having dinner!"); } public void sleep() { System.out.println("sleep!"); } }
2. Class instantiation
2. A class can instantiate multiple objects. Only the instantiated objects occupy the actual physical space and store class member variables.
Let's instantiate an object through the class of the previous example:
class Person { public int age; //Member property instance variable public String name; public String sex; public void eat() { //Member method System.out.println("having dinner!"); } public void sleep() { System.out.println("sleep!"); } } public class Main{ public static void main(String[] args) { Person person = new Person(); //Instantiate objects through new person.eat(); //Member method calls need to be called by reference to the object person.sleep(); Person person2 = new Person(); Person person3 = new Person(); } } //Operation results having dinner! sleep!
3. Member of class
3.1. Member variables / attributes
class Person { public String name; //Member variable public int age; } class Test { public static void main(String[] args) { Person person = new Person(); System.out.println(person.name); System.out.println(person.age); } } // results of enforcement null 0
Note: if the initial value is not explicitly set for the field of an object, a default initial value will be set.
- For various number types, the default value is 0 (float and double are 0.0 by default)
- For boolean types, the default value is false
- For char type, the default is null character, '\ u0000'
- For reference types (String, Array, and custom classes), the default value is null
3.2 understanding
3.3 method
class Person { public int age = 18; public String name = "Zhang San"; public void show() { System.out.println("My name is" + name + ", this year" + age + "year"); } } class Test { public static void main(String[] args) { Person person = new Person(); person.show(); } } // results of enforcement My name is Zhang San, He is 18 years old
3, Construction method
Basic syntax:
Construction method is a special method. When a new object is instantiated with the keyword new, it will be automatically called to complete the initialization operation.
class Person { private String name;//Member variable private int age; private String sex; //Default parameterless constructor public Person() { this.name = "caocao"; this.age = 10; this.sex = "male"; } //Constructor with 3 arguments public Person(String name,int age,String sex) { this.name = name; this.age = age; this.sex = sex; } public void show(){ System.out.println("name: "+name+" age: "+age+" sex: "+sex); } } public class Main{ public static void main(String[] args) { Person p1 = new Person();//Call the constructor without parameters. If the program does not provide it, it will call the constructor without parameters p1.show(); Person p2 = new Person("zhangfei",80,"male");//Call the constructor with 3 parameters p2.show(); } } // results of enforcement name: caocao age: 10 sex: male name: zhangfei age: 80 sex: male
4, Keywords
1. this keyword
(1) Call the member variable through the this keyword
class Person{ int age; //Member variable age public Person(int age){ //Local variable age this.age=age; //Assign the value of the local variable age to the member variable age } }
As in the above example, if "age" is used in the construction method, local variables are accessed, and if "this.age" is used, member variables are accessed.
(2) Calling member methods with the this keyword
As follows:
class Person{ public void openMouth(){ .... } public void speak(){ this.openMouth(); } }
Through the above example, we used the this keyword to call the openMouth() method. It should be noted that the this keyword here can be omitted without writing, and the effect is the same. Therefore, we won't repeat it, but it means that it can be called in this way.
(3) this keyword calls the constructor
The construction method is automatically called by the Java virtual machine when instantiating the object. In the program, other construction methods can be called in the form of "this [parameter 1, parameter 2,...]" in one construction method.
class Person{ public Person(){ System.out.println("The parameterless constructor was called..."); } public Person(int age){ this(); System.out.println("The constructor with parameters was called..."); } } public class testdemo{ public static void main(String[] args){ Person p=new Person(18); } } //Operation results The parameterless constructor was called... The constructor with parameters was called...
Analyze the above code: when instantiating the Person object, the constructor with parameters is called, and the constructor without parameters is called through this() in the constructor with parameters. Therefore, the running results show that both constructors have been called.
When using this to call the constructor of a class, you should pay attention to the following points:
1. this can only be used in constructor to call other constructor methods, not in other member methods.
2. In a construction method, the statement that uses this to call the construction method must be the first execution statement of the method and can only appear once.
2. static keyword
First, the static keyword can be used to——
(1) Modifier attribute
Java static attributes are related to classes, not specific instances. In other words, different instances of the same class can share the same static attribute.
When the attribute is modified by static, it is shared by all classes and does not belong to an object. The access method is: class name. Attribute.
class TestDemo{ public int a; public static int count; //Define static member variables } public class Main{ public static void main(String[] args) { TestDemo t1 = new TestDemo(); t1.a++; TestDemo.count++; //The class name. Attribute is used directly when calling System.out.println(t1.a); System.out.println(TestDemo.count); System.out.println("============"); TestDemo t2 = new TestDemo(); t2.a++; TestDemo.count++; System.out.println(t2.a); System.out.println(TestDemo.count); } } //Operation results 1 1 ============ 1 2
be careful:
1. Static keyword can only be used to modify member variables, not local variables (that is, a variable cannot be declared as static in non static methods), otherwise an error will be reported during compilation!
Why? Because variables decorated with static can be accessed by instantiated objects of any class, and variables declared in methods are local. After the method is executed, it needs to be destroyed to free up space.
(2) Modification method
class TestDemo{ public int a; public static int count; public static void change() { count = 100; //a = 10; error non static data members cannot be accessed } } public class Main{ public static void main(String[] args) { TestDemo.change(); //Can be called without creating an instance object System.out.println(TestDemo.count); } } //Operation results 100
be careful:
Static methods have nothing to do with objects, but are related to classes. Therefore, two situations are caused:
1. Static methods cannot directly use non static data members or call non static methods (because non static data members and methods are instance related).
class Testdemo{ public int a; public static int count; } public class Main{ public static void main(String[] args) { Testdemo t1 = new Testdemo(); t1.a++; Testdemo.count++; System.out.println(t1.a); System.out.println(Testdemo.count); System.out.println("============"); Testdemo t2 = new Testdemo(); t2.a++; Testdemo.count++; System.out.println(t2.a); System.out.println(Testdemo.count); } } //Operation results 1 1 ============ 1 2
Its distribution in memory is as follows:
As can be seen from the above figure:
Java has stack memory and heap memory. The stack memory is used to store basic type variables (local variables) and object reference variables (such as t1 and t2 in this example), and the heap memory is used to store objects (such as member variable a in this example) and arrays created by new.
However, the static variable (count in this example) has been generated during compilation and belongs to the class itself, and there is only one copy. It is stored in the method area.
5, Class encapsulation
1. How to realize encapsulation
Class encapsulation means that the state information of the object is hidden inside the object, and the external program is not allowed to directly access the internal information of the object, but to access the internal information through the methods provided by the class.
Its specific implementation process is to privatize the attributes in the class, that is, to modify them with the private keyword. If the outside world wants to access private properties, it needs to provide some common methods decorated with public.
class Person { private String name = "Zhang San"; private int age = 18; public void show() { System.out.println("My name is" + name + ", this year" + age + "year"); } } class Test { public static void main(String[] args) { Person person = new Person(); person.show(); } } // results of enforcement My name is Zhang San, He is 18 years old
- At this point, the field has been decorated with private. The caller of class cannot use it directly, but needs to use the show method. At this point, the user of the class does not have to understand the implementation details of the Person class.
- At the same time, if the implementer of the class modifies the name of the member variable, the caller of the class does not need to make any modification (because the caller of the class can't access variables such as name and age), so the code maintenance cost is greatly reduced.
2.getter and setter methods
When we use private to decorate a field, we can't use this field directly. At this time, if you need to get or modify the private property, you need to use the getter / setter method.
class Person { private String name; //Instance member variable private int age; public void setName(String name){ this.name = name; } public String getName(){ return name; } public void show(){ System.out.println("name: "+name+" age: "+age); } } public static void main(String[] args) { Person person = new Person(); person.setName("caocao"); String name = person.getName(); System.out.println(name); person.show(); } // Operation results caocao name: caocao age: 0
6, Code block
- Common code block
- Tectonic block
- Static block
1. Common code block
Common code blocks are: code blocks defined in methods.
public class Main{ public static void main(String[] args) { { int x = 10 ; //Directly use {} definition, common method block System.out.println("x1 = " +x); } int x = 100 ; System.out.println("x2 = " +x); } } // results of enforcement x1 = 10 x2 = 100
2. Construct code block
class Person{ private String name; //Instance member variable private int age; private String sex; public Person() { System.out.println("I am Person init()!"); } //Instance code block (also known as construction code block) { this.name = "Zhang San"; this.age = 12; this.sex = "man"; System.out.println("I am instance init()!"); } public void show(){ System.out.println("name: "+name+" age: "+age+" sex: "+sex); } } public class Main { public static void main(String[] args) { Person p1 = new Person(); p1.show(); } } // Operation results I am instance init()! I am Person init()! name: Zhang San age: 12 sex: man
From the above example -- the instance code block takes precedence over the constructor.
3. Static code block
class Person{ private String name; //Instance member variable private int age; private String sex; private static int count = 0;//Static member variables are shared by classes and stored in the method area public Person(){ System.out.println("Execute constructor!"); } //Instance code block { this.name = "Zhang San"; this.age = 12; this.sex = "man"; System.out.println("Execute instance code block!"); } //Static code block static { count = 10; //Only static data members can be accessed System.out.println("Execute static code block!"); } public void show(){ System.out.println("name: "+name+" age: "+age+" sex: "+sex); } } public class Main { public static void main(String[] args) { Person p1 = new Person(); Person p2 = new Person(); p2.show(); } } //Operation results Execute static code block! Execute instance code block! Execute constructor! Execute instance code block! Execute constructor! name: Zhang San age: 12 sex: man
From the above example, we can conclude that:
1. No matter how many objects are generated, static code blocks are executed only once and first.
2. If static, instance and constructor exist, the execution order is: static code block > instance code block > constructor.
7, toString method
Let's start with a sample code:
class Person { private String name; private int age; public Person(String name,int age) { this.age = age; this.name = name; } public void show() { System.out.println("name:"+name+" " + "age:"+age); } } public class Main { public static void main(String[] args) { Person person = new Person("haha",19); System.out.println(person); } } // results of enforcement Person@1c168e5
We found that the hash value of an address is printed here. The reason: the toString method of Object is called. This method hashes the memory address of the Object and returns a hash value of type int.
In actual development, we usually hope that the toString () method returns not only the basic information of the object, but some more useful information. Therefore, we can rewrite the toString () method.
class Person { private String name; private int age; public Person(String name,int age) { this.age = age; this.name = name; } public void show() { System.out.println("name:"+name+" " + "age:"+age); } //Override the toString method of Object @Override public String toString() { return "Person{" + "name='" + name + '\'' + ", age=" + age + '}'; } } public class Main { public static void main(String[] args) { Person person = new Person("haha",19); System.out.println(person); } } // results of enforcement Person{name='haha', age=19}
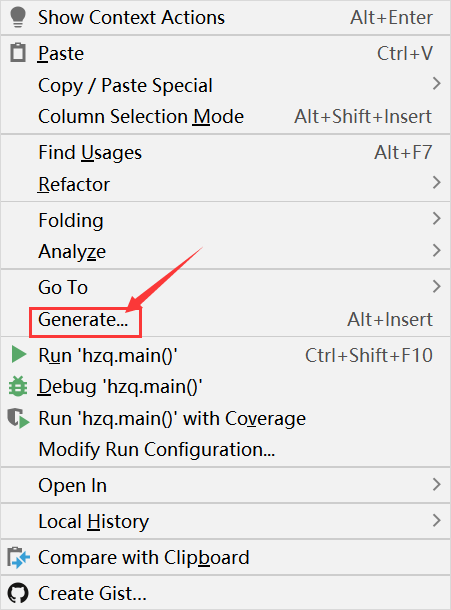
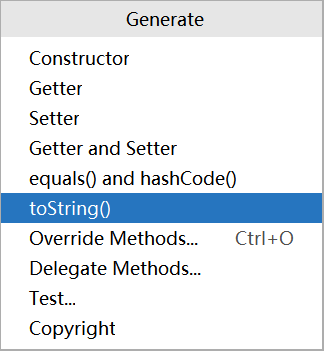
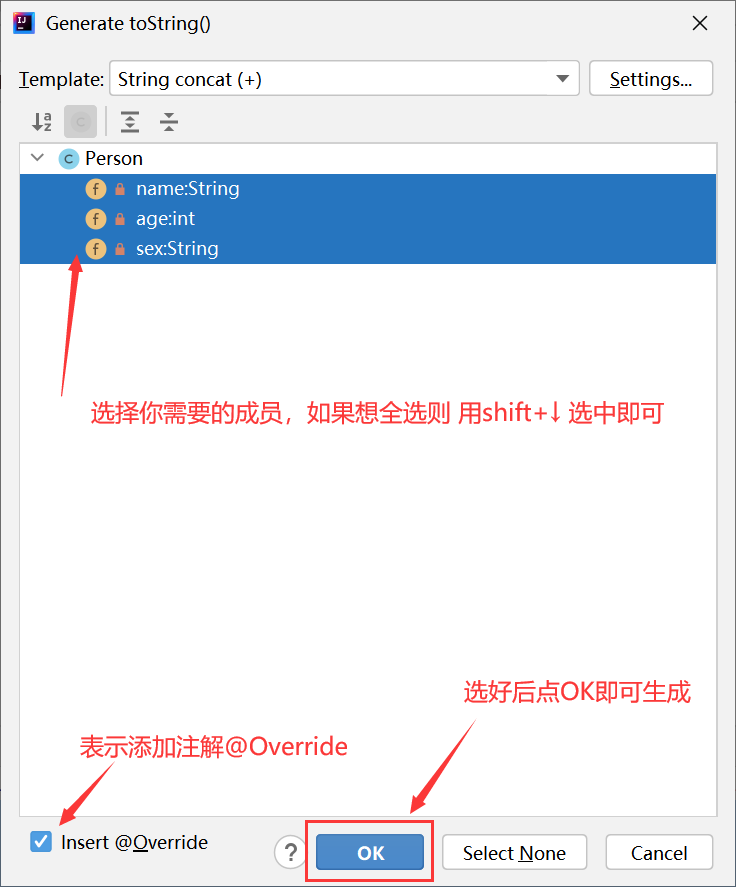
8, Anonymous object
class Person { private String name; private int age; public Person(String name,int age) { this.age = age; this.name = name; } public void show() { System.out.println("name:"+name+" " + "age:"+age); } } public class Main { public static void main(String[] args) { new Person("Zhang San",19).show(); //Calling methods through anonymous objects } } // results of enforcement name: Zhang San age: 19
9, Try ox knife at the end
After learning the above knowledge, let's end with a small topic ~ you can also knock on the code.
Title: realize the exchange of the values of two variables. Requirements: you need to exchange the values of arguments (don't fake exchange)
Think about it and watch it again
3
2
1
The answers are announced below——
class MyValue { public int val; } public class Main { public static void swap(MyValue myV1,MyValue myV2) { int tmp = myV1.val; myV1.val = myV2.val; myV2.val = tmp; } public static void main(String[] args) { MyValue myValue1 = new MyValue(); myValue1.val = 10; MyValue myValue2 = new MyValue(); myValue2.val = 20; swap(myValue1,myValue2); System.out.println("myValue1:"+myValue1.val+";" +"myValue2:"+myValue2.val); } } //Operation results myValue1:20;myValue2:10
Some students may not understand it. It seems that this writing method has no change from the previous values. In fact, we pass references. It's better to draw a picture.
This section is over. See you next section~
to be continue →
Welcome to discuss and exchange with each other. Welcome to catch insects!