Before learning to merge and sort, please have a general look Eight sorting algorithms that programmers must master
(1) Basic ideas
Merge sorting is to merge two (or more) ordered tables into a new ordered table, that is, to divide the sequence to be sorted into several subsequences, each subsequence is ordered. Then the ordered subsequences are combined into the whole ordered sequences.
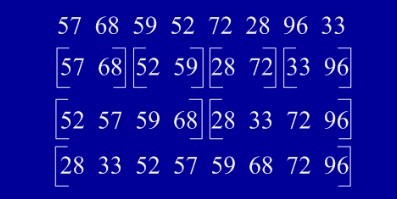
7-1.jpg
(2) Code implementation
import java.util.Arrays; public class Sort { public static void mergeSort(int[] array) { sort(array, 0, array.length - 1); } private static void sort(int[] data, int left, int right) { if (left < right) { // Find the middle index int center = (left + right) / 2; // Recurse the left array sort(data, left, center); // Recurse the right array sort(data, center + 1, right); // merge merge(data, left, center, right); } } private static void merge(int[] data, int left, int center, int right) { int[] tmpArr = new int[data.length]; int centerNext = center + 1; // Index of record temporary array int tmp = left; int index = left; while (left <= center && centerNext <= right) { //Take the smallest of the two arrays and put it into the temporary array if (data[left] <= data[centerNext]) { tmpArr[tmp++] = data[left++]; } else { tmpArr[tmp++] = data[centerNext++]; } } // If there are remaining elements in the right array, put them in the temporary array in turn while (centerNext <= right) { tmpArr[tmp++] = data[centerNext++]; } // If there are remaining elements in the left array, put them in the temporary array in turn while (left <= center) { tmpArr[tmp++] = data[left++]; } // Copy the contents of the temporary array back to the original array while (index <= right) { data[index] = tmpArr[index++]; } } public static void main(String[] args) { int[] arr = {57, 68, 59, 52, 72, 28, 96, 33}; System.out.println("Original array: " + Arrays.toString(arr)); mergeSort(arr); System.out.println("Sorted array: " + Arrays.toString(arr)); } }
Operation result:
> javac -encoding utf-8 Sort.java > java Sort Original array: [57, 68, 59, 52, 72, 28, 96, 33] Sorted array: [28, 33, 52, 57, 59, 68, 72, 96]
TopCoder & codeforces & atcoder communication QQ group: 648202993
More content, please pay attention to WeChat official account.
wechat_public_header.jpg