Chapter III Experiments
Keywords:
P4
The purpose and requirements of the experiment
1. Familiar with the definition format of classes and the access rights of members in classes.
2. Timing and sequence of calling constructors and destructors.
3. To grasp the definition of the object and the timing and method of its initialization.
Experimental content
1. The following program sy3_1.cpp has errors with ERROR statements. Without deleting and adding lines of code, correct the erroneous statements to make them run correctly.
The operation procedure is as follows:
- #include<iostream>
- using namespace std;
- class Aa
- {
- public:
- Aa(int i=0){a=i;cout<<"Constructor"<<a<<endl;}
- ~Aa(){cout<<"Destructor"<<a<<endl;}
- void print(){cout<<a<<endl;}
- private:
- int a;
- };
- int main()
- {
- Aa a1(1),a2(2);
- a1.print();
- cout<<a2.a<<endl;
- return 0;
- }
The mistakes are as follows:
The amendment procedure is as follows:
- #include<iostream>
- using namespace std;
- class Aa
- {
- public:
- Aa(int i=0){a=i;cout<<"Constructor"<<a<<endl;}
- ~Aa(){cout<<"Destructor"<<a<<endl;}
- void print(){cout<<a<<endl;}
- private:
- int a;
- };
- int main()
- {
- Aa a1(1),a2(2);
- a1.print();
- a2.print();
- return 0;
- }
The results are as follows:
2. Debugging the following procedures:
- #include<iostream>
- using namespace std;
- class TPoint
- {
- public:
- TPoint(int x=0,int y=0){X=x,Y=y;}
- TPoint(TPoint &p);
- ~TPoint(){cout<<"Destructor is called\n";}
- int getx(){return X;}
- int gety(){return Y;}
- private:
- int X,Y;
- };
- TPoint::TPoint(TPoint &p)
- {
- X=p.X;
- Y=p.Y;
- cout<<"Copy-initialization Constructor is called\n";
- }
- int main()
- {
- TPoint p1(4,9);
- TPoint p2(p1);
- TPoint p3=p2;
- TPoint p4,p5(2);
- cout<<"p3=("<<p3.getx()<<","<<p3.gety()<<")\n";
- return 0;
- }
In the program, the constructor of TPoint class with two parameters is modified, and the following statement is added to the body of the function:
cout<<"Constructor is called\n";
(1) Write out the output result of the program and explain the output result;
The procedure is as follows:
- #include<iostream>
- using namespace std;
- class TPoint
- {
- public:
- TPoint(int x=0,int y=0){X=x,Y=y;}
- TPoint(TPoint &p);
- ~TPoint(){cout<<"Destructor is called\n";}
- int getx(){return X;}
- int gety(){return Y;}
- private:
- int X,Y;
- };
- TPoint::TPoint(TPoint &p)
- {
- X=p.X;
- Y=p.Y;
- cout<<"Constructor is called\n";
- cout<<"Copy-initialization Constructor is called\n";
- }
- int main()
- {
- TPoint p1(4,9);
- TPoint p2(p1);
- TPoint p3=p2;
- TPoint p4,p5(2);
- cout<<"p3=("<<p3.getx()<<","<<p3.gety()<<")\n";
- return 0;
- }
The results are as follows:
(2) Conduct debugging according to the following requirements:
In the main function body, add the following statement:
TPoint p4,p5(2)cpp] view plai
- #include<iostream>
- using namespace std;
- class TPoint
- {
- public:
- TPoint(int x,int y){X=x,Y=y;}
- TPoint(TPoint &p);
- ~TPoint(){cout<<"Destructor is called\n";}
- int getx(){return X;}
- int gety(){return Y;}
- private:
- int X,Y;
- };
- TPoint::TPoint(TPoint &p)
- {
- X=p.X;
- Y=p.Y;
- cout<<"Copy-initialization Constructor is called\n";
- cout<<"Constructor is called\n";
- }
- int main()
- {
- TPoint P4,P5(2);
- TPoint p1(4,9);
- TPoint p2(p1);
- TPoint p3=p2;
- cout<<"p3=("<<p3.getx()<<","<<p3.gety()<<")\n";
- return 0;
- }
What will happen to the debugger? Why? How to solve it? (Tip: Modify the existing constructors appropriately) Analyse how to use different constructors to create different objects combined with the results of operation.
Phenomenon:
Why: Because there is no constructor defined in the class without parameters and with one parameter;
How to solve this problem: changing the constructor with two parameters to the default constructor is to change TPoint(int x,int y) to TPoint(int x=0,int y=0); during the operation, TPoint p1(4,9) and TPoint p4,p5(2); calling the constructor, while TPoint p2(p1) and TPoint p3=p2 are using copy constructors. As follows:
-
- #include<iostream>
- using namespace std;
- class TPoint
- {
- public:
- TPoint(int x=0,int y=0){X=x,Y=y;}
- TPoint(TPoint &p);
- ~TPoint(){cout<<"Destructor is called\n";}
- int getx(){return X;}
- int gety(){return Y;}
- private:
- int X,Y;
- };
- TPoint::TPoint(TPoint &p)
- {
- X=p.X;
- Y=p.Y;
- cout<<"Copy-initialization Constructor is called\n";
- cout<<"Constructor is called\n";
- }
- int main()
- {
- TPoint P4,P5(2);
- TPoint p1(4,9);
- TPoint p2(p1);
- TPoint p3=p2;
- cout<<"p3=("<<p3.getx()<<","<<p3.gety()<<")\n";
- return 0;
- }
Result:
3. Make the following modifications to the main function of Li3_11.cpp in the textbook:
(1) Change Heapclass *pa1,*pa2 to Heapclass *pa1,*pa2,*pa3;
(2) After the statement pa2=new Heapclass, add the statement pa3=new Heapclass(5);
(3) Change the statement if (! PA1 |! Pa2) to if (! PA1 |! Pa2 |! Pa3)
(4) After the statement delete pa2, add the statement delete pa3;
Write out the output of the program and explain the output.
The procedure is as follows:
- #include<iostream>
- using namespace std;
- class Heapclass
- {
- public:
- Heapclass(int x);
- Heapclass();
- ~Heapclass();
- private:
- int i;
- };
- Heapclass::Heapclass(int x)
- {
- i=x;
- cout<<"Contstructor is called. "<<i<<endl;
- }
- Heapclass::Heapclass()
- {
- cout<<"Default Contstructor is called."<<endl;
- }
- Heapclass::~Heapclass()
- {
- cout<<"Default is called."<<endl;
- }
- int main()
- {
- Heapclass *pa1,*pa2,*pa3;
- pa1=new Heapclass(4);
- pa2=new Heapclass;
- pa3=new Heapclass(5);
- if(!pa1||!pa2||!pa3)
- {
- cout<<"Out of Mcmory!"<<endl;
- return 0;
- }
- cout<<"Exit main"<<endl;
- delete pa1;
- delete pa2;
- delete pa3;
- return 0;
- }
The results are as follows:
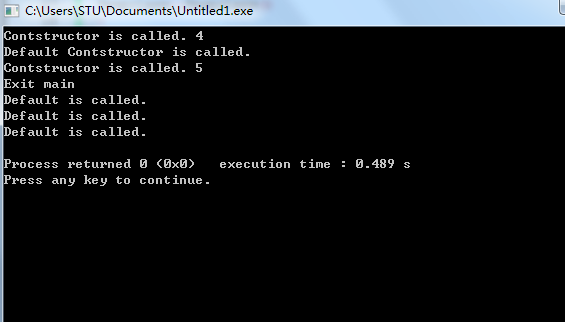
Interpretation: Pa1, pa2, and pa3 are two object pointers to Heapclass class. When they can be allocated enough memory, they are assigned values using the operator new, and the objects they refer to are initialized. Release the objects pointed to by these three pointers using delete, and output "Out of Memory" because pa1, pa2, or pa3 cannot be allocated enough memory.
4. Define a Rectangle class. Private data members are rectangular lengths and wids. Parametric constructors set len and wid to 0. Parametric constructors set len and wid to corresponding shape parameters. In addition, it includes finding rectangular circumference, rectangular area, rectangular length and width, modifying rectangular length and width to corresponding shape parameters, and outputting rectangular ruler. Public member functions such as inch. The format of output rectangular size is required to be "length: length, width: width".
The procedure is as follows:
- #include<iostream>
- using namespace std;
- class Rectangle
- {
- public:
- Rectangle()
- {
- len=0;
- wid=0;
- }
- Rectangle(double Len,double Wid)
- {
- len=Len;
- wid=Wid;
- }
- double Circumference()
- {
- return 2*(len+wid);
- }
- double Area()
- {
- return len*wid;
- }
- double getl()
- {
- return len;
- }
- double getw()
- {
- return wid;
- }
- void charge(double a,double b)
- {
- len=a;
- wid=b;
- }
- void s()
- {
- cout<<"length:"<<len<<" "<<"width:"<<wid<<endl;
- }
- private:
- int len,wid;
- };
- int main()
- {
- Rectangle q;
- Rectangle h(5.0,2.0);
- cout<<"q Rectangular size:"<<endl;
- q.s();
- cout<<"h Rectangular size:"<<endl;
- h.s();
- cout<<"h Circumference:"<<h.Circumference()<<endl;
- cout<<"h Area:"<<h.Area()<<endl;
- cout<<"h Length:"<<h.getl()<<endl;
- cout<<"h Width:"<<h.getw()<<endl;
- h.charge(8.0,6.0);
- cout<<"Modified rectangle size:"<<endl;
- h.s();
- return 0;
- }
The results are as follows:
1. Access rights of private members in a class.
Answer: Private members are hidden data that can only be referenced by member functions or friend functions of the class.
2. Calling order of constructor and destructor.
Answer: Constructors are called when creating objects, destructors are called when releasing objects, releasing memory allocated by constructors, and constructors are invoked in exactly the opposite order as destructors.
3. When does object initialization take place? How to proceed? (Tip: Pay attention to the discussion of general objects and heap objects)
Answer: General objects: When creating objects, they can be initialized with constructors or copy functions.
Heap object: use operator new to allocate memory and call constructor to initialize.
Posted by paruby on Thu, 16 May 2019 14:33:20 -0700