1, Qt 5 main window composition
QMainWindow is a class that provides users with main window programs, including a menu bar, multiple tool bars, multiple dock widgets, a status bar and a central widget. It is the basis of many applications (such as text editor, picture editor, etc.). This is described in detail in this chapter. The interface layout of Qt main window is shown in Figure 5.1.
1. Menu bar
A menu is a list of commands. In order to achieve the consistency of commands such as menus, toolbar buttons and keyboard shortcuts, Qt uses Action to represent these commands. Qt's menu is a list composed of a series of QAction Action objects, and the menu bar is the panel containing the menu, which is located below the title bar of the main window. A main window can only have one menu bar.
2. Status bar
The status bar usually displays some status information of the GUI application, which is located at the bottom of the main window. Users can add and use Qt widgets on the status bar. A main window can only have one status bar.
3. Toolbar
A toolbar is a panel arranged by a series of actions similar to buttons. It is usually composed of some frequently used commands (actions). The toolbar is located below the menu bar and above the status bar. It can be docked in the up, down, left and right directions of the main window. A main window can contain multiple toolbars.
The toolbar is a movable window. Its dockable area is determined by the allowAreas of qttoolbar, including Qt::LeftToolBarArea, Qt::RightToolBarArea, Qt::TopToolBarArea, Qt::BottomToolBarArea and Qt::AllToolBarAreas. The default is Qt::AllToolBarAreas, which appears at the top of the main window by default after startup. You can specify the dockable area of the toolbar by calling the setAllowAreas() function, for example:
fileTool->setAllowedAreas(Qt::TopToolBarArea|Qt::LeftToolBarArea);
This function restricts the file toolbar to the top or left of the main window only. The toolbar can also set the mobility by calling the setMovable() function, for example:
fileTool->setMovable(false);
Specifies that the file toolbar is not removable and appears only at the top of the main window.
4. Anchoring parts
The anchor assembly is used as a container to contain other widgets to achieve certain functions. For example, the property editor and object monitor of Qt designer are implemented by anchor parts containing other Qt window parts. It is located inside the toolbar area. It can float freely on the main window as a window, or dock in the upper, lower, left and right directions of the main window like a toolbar. A main window can contain multiple anchor parts.
5. Central component
The central part is located inside the anchor part area and in the center of the main window. A main window can only have one central part.
2, Comprehensive example - text editor
(1) File operation functions: including creating a new file, opening an existing file by using the QFileDialog class of the standard file dialog box, reading the file content by using QFile and QTextStream, and printing the file (text printing and image printing). This paper introduces the use method of QPrintDialog class of standard printing dialog box through an example, and uses QPrinter as QPaintDevice drawing tool to realize image printing.
(2) Common functions in image processing software: including image scaling, rotation, mirror image and other coordinate transformations. Various coordinate transformations of images are realized by QMatrix.
(3) Develop text editing function: This paper introduces the method of embedding controls in the toolbar by setting shortcut buttons such as text font, font size, bold, italic, underline and font color on the toolbar. Among them, the use of QColorDialog class of standard color dialog box is introduced by setting the font color function.
(4) Typesetting function: sort text by selecting a sort method, and realize text alignment (including left alignment, right alignment, center alignment and both ends alignment) and undo and redo methods.
1. Design page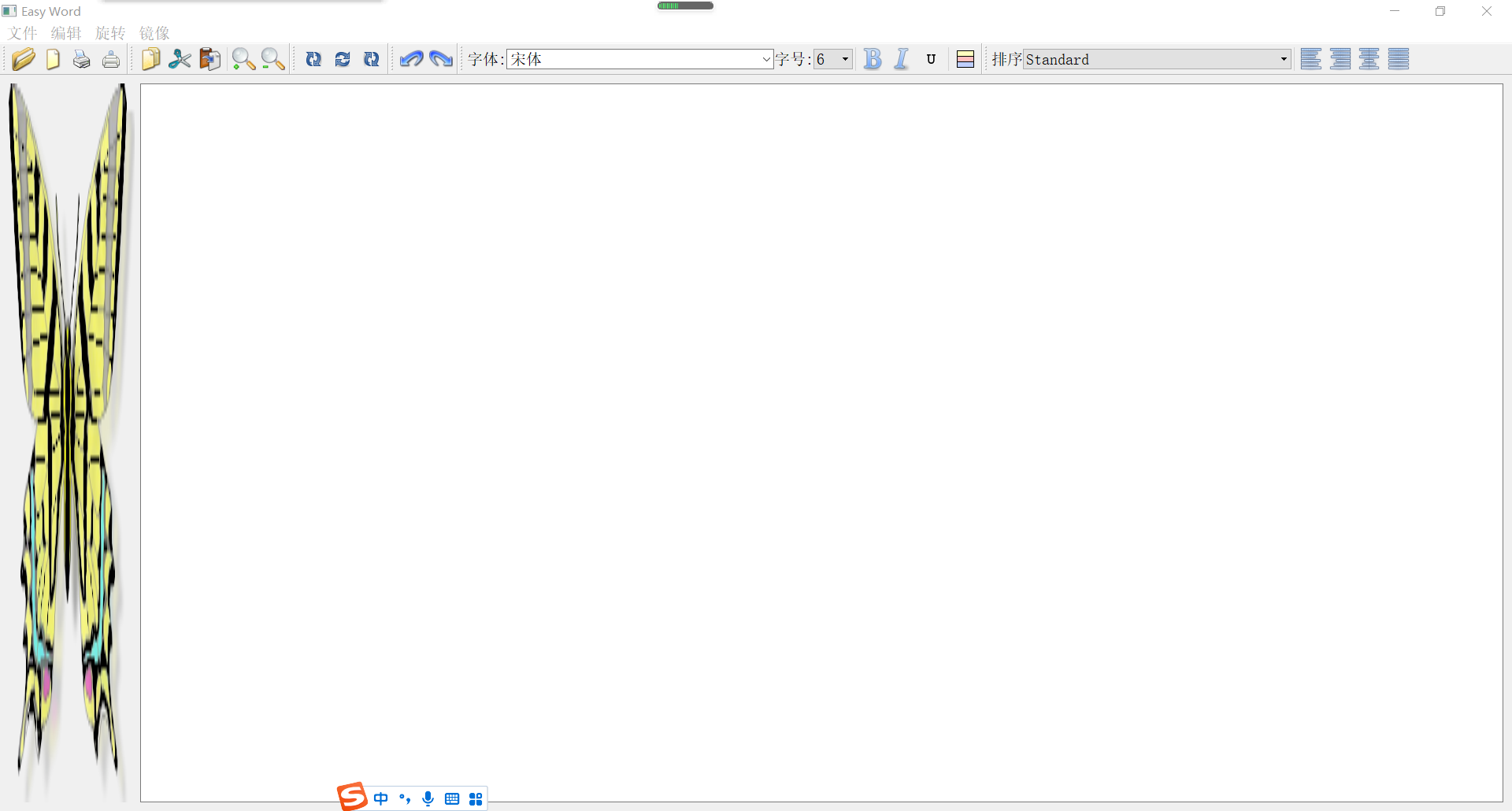
2. Project composition
showwidget It mainly displays the file where the text edit box function is located
ImgProcessor mainly provides the implementation of the text editor function. The createActions() function in the ImgProcessor class declaration is used to create all actions, the createMenus() function is used to create menus, and the createToolBars() function is used to create toolbars; Then declare the elements needed to realize the main window, including menu, toolbar and various actions; Finally, declare the slot function used
3. showwidget file
showwidget.h
#ifndef SHOWWIDGET_H #define SHOWWIDGET_H #include <QWidget> #include <QLabel> #include <QTextEdit> #include <QImage> class ShowWidget : public QWidget { Q_OBJECT public: explicit ShowWidget(QWidget *parent = 0); QImage img; //Images displayed in imageLabel QLabel *imageLabel; //label used to display pictures QTextEdit *text; //Text edit box signals: public slots: }; #endif // SHOWWIDGET_H
showwidget.cpp
#include "showwidget.h" #include <QHBoxLayout> ShowWidget::ShowWidget(QWidget *parent) : QWidget(parent) { imageLabel =new QLabel; imageLabel->setScaledContents(true); //Picture adaptive label size text =new QTextEdit; QHBoxLayout *mainLayout =new QHBoxLayout(this); //Horizontal layout mainLayout->addWidget(imageLabel); mainLayout->addWidget(text); }
4. imgprocessor file
imgprocessor.h, which declares slot functions for actions, menus, toolbars, and text editing functions
#ifndef IMGPROCESSOR_H #define IMGPROCESSOR_H #include <QMainWindow> #include <QImage> #include <QLabel> #include <QMenu> #include <QMenuBar> #include <QAction> #include <QComboBox> #include <QSpinBox> #include <QToolBar> #include <QFontComboBox> #include <QToolButton> #include <QTextCharFormat> #include "showwidget.h" class ImgProcessor : public QMainWindow { Q_OBJECT public: ImgProcessor(QWidget *parent = 0); ~ImgProcessor(); void createActions(); //Create action void createMenus(); //create menu void createToolBars(); //Create toolbar void loadFile(QString filename); //Load picture void mergeFormat(QTextCharFormat); //Modify text formatting private: QMenu *fileMenu; //Menu bar QMenu *zoomMenu; QMenu *rotateMenu; QMenu *mirrorMenu; QImage img; QString fileName; ShowWidget *showWidget; QAction *openFileAction; //File menu item QAction *NewFileAction; QAction *PrintTextAction; QAction *PrintImageAction; QAction *exitAction; QAction *copyAction; //Edit menu item QAction *cutAction; QAction *pasteAction; QAction *aboutAction; QAction *zoomInAction; QAction *zoomOutAction; QAction *rotate90Action; //Rotate menu item QAction *rotate180Action; QAction *rotate270Action; QAction *mirrorVerticalAction; //Mirror menu item QAction *mirrorHorizontalAction; QAction *undoAction; QAction *redoAction; QToolBar *fileTool; //toolbar QToolBar *zoomTool; QToolBar *rotateTool; QToolBar *mirrorTool; QToolBar *doToolBar; QLabel *fontLabel1; //Font setting item QFontComboBox *fontComboBox; QLabel *fontLabel2; QComboBox *sizeComboBox; QToolButton *boldBtn; QToolButton *italicBtn; QToolButton *underlineBtn; QToolButton *colorBtn; QToolBar *fontToolBar; //Font toolbar QLabel *listLabel; //Sort settings QComboBox *listComboBox; QActionGroup *actGrp; QAction *leftAction; QAction *rightAction; QAction *centerAction; QAction *justifyAction; QToolBar *listToolBar; //Sort toolbar protected slots: void ShowNewFile(); void ShowOpenFile(); void ShowPrintText(); void ShowPrintImage(); void ShowZoomIn(); void ShowZoomOut(); void ShowRotate90(); void ShowRotate180(); void ShowRotate270(); void ShowMirrorVertical(); void ShowMirrorHorizontal(); void ShowFontComboBox(QString comboStr); void ShowSizeSpinBox(QString spinValue); void ShowBoldBtn(); void ShowItalicBtn(); void ShowUnderlineBtn(); void ShowColorBtn(); void ShowCurrentFormatChanged(const QTextCharFormat &fmt); void ShowList(int); void ShowAlignment(QAction *act); void ShowCursorPositionChanged(); }; #endif // IMGPROCESSOR_H
imgprocessor.cpp Define the specific implementation of the function
#include "imgprocessor.h" #include <QFileDialog> #include <QFile> #include <QTextStream> #include <QPrintDialog> #include <QPrinter> #include <QPainter> #include <QColorDialog> #include <QColor> #include <QTextList> ImgProcessor::ImgProcessor(QWidget *parent) : QMainWindow(parent) { setWindowTitle(tr("Easy Word")); //Set form title showWidget =new ShowWidget(this); //Create a QWidget object showWidget that places the image QLabel and the text edit box QTextEdit, and set the QWidget object as the central part. setCentralWidget(showWidget); //Embed controls on toolbars //Set font fontLabel1 =new QLabel(tr("typeface:")); fontComboBox =new QFontComboBox; fontComboBox->setFontFilters(QFontComboBox::ScalableFonts); //setFontFilters, filter fonts scaleable fonts display scalable fonts fontLabel2 =new QLabel(tr("Font size:")); sizeComboBox =new QComboBox; QFontDatabase db; //All available format information in the current system, mainly font and font size foreach(int size,db.standardSizes()) //standardSizes returns a list of available standard font sizes sizeComboBox->addItem(QString::number(size)); //Insert font size drop-down list box boldBtn =new QToolButton; boldBtn->setIcon(QIcon(":/image/bold.png")); boldBtn->setCheckable(true); //This property holds whether the button can be checked italicBtn =new QToolButton; italicBtn->setIcon(QIcon(":/image/italic.png")); italicBtn->setCheckable(true); underlineBtn =new QToolButton; underlineBtn->setIcon(QIcon(":/image/underline.png")); underlineBtn->setCheckable(true); colorBtn =new QToolButton; colorBtn->setIcon(QIcon(":/image/color.png")); colorBtn->setCheckable(true); //sort listLabel =new QLabel(tr("sort")); listComboBox =new QComboBox; listComboBox->addItem("Standard"); listComboBox->addItem("QTextListFormat::ListDisc"); listComboBox->addItem("QTextListFormat::ListCircle"); listComboBox->addItem("QTextListFormat::ListSquare"); listComboBox->addItem("QTextListFormat::ListDecimal"); listComboBox->addItem("QTextListFormat::ListLowerAlpha"); listComboBox->addItem("QTextListFormat::ListUpperAlpha"); listComboBox->addItem("QTextListFormat::ListLowerRoman"); listComboBox->addItem("QTextListFormat::ListUpperRoman"); /* Functions for creating actions, menus, toolbars */ createActions(); createMenus(); createToolBars(); if(img.load(":/image/image.png")) { //Place the image in the imageLabel object showWidget->imageLabel->setPixmap(QPixmap::fromImage(img)); } connect(fontComboBox,SIGNAL(activated(QString)), this,SLOT(ShowFontComboBox(QString))); connect(sizeComboBox,SIGNAL(activated(QString)), this,SLOT(ShowSizeSpinBox(QString))); connect(boldBtn,SIGNAL(clicked()),this,SLOT(ShowBoldBtn())); connect(italicBtn,SIGNAL(clicked()),this,SLOT(ShowItalicBtn())); connect(underlineBtn,SIGNAL(clicked()),this,SLOT(ShowUnderlineBtn())); connect(colorBtn,SIGNAL(clicked()),this,SLOT(ShowColorBtn())); connect(showWidget->text,SIGNAL(currentCharFormatChanged(QTextCharFormat&)),this,SLOT(ShowCurrentFormatChanged(QTextCharFormat&))); connect(listComboBox,SIGNAL(activated(int)),this,SLOT(ShowList(int))); connect(showWidget->text->document(),SIGNAL(undoAvailable(bool)),redoAction,SLOT(setEnabled(bool))); connect(showWidget->text->document(),SIGNAL(redoAvailable(bool)),redoAction,SLOT(setEnabled(bool))); connect(showWidget->text,SIGNAL(cursorPositionChanged()),this,SLOT(ShowCursorPositionChanged())); } void ImgProcessor::createActions() { //Open action openFileAction =new QAction(QIcon(":/image/open.png"),tr("open"),this);//When you create the open file action, you specify the icon, name, and parent window used by the action. openFileAction->setShortcut(tr("Ctrl+O")); //Set the key combination for this action to Ctrl+O. openFileAction->setStatusTip(tr("Open a file")); //The status bar display is set. When the mouse cursor moves to the menu item or toolbar button corresponding to this action, the prompt of "open a file" will be displayed on the status bar. connect(openFileAction,SIGNAL(triggered()),this,SLOT(ShowOpenFile())); //New action NewFileAction =new QAction(QIcon(":/image/new.png"),tr("newly build"),this); NewFileAction->setShortcut(tr("Ctrl+N")); NewFileAction->setStatusTip(tr("Create a new file")); connect(NewFileAction,SIGNAL(triggered()),this,SLOT(ShowNewFile())); //Exit action exitAction =new QAction(tr("sign out"),this); exitAction->setShortcut(tr("Ctrl+Q")); exitAction->setStatusTip(tr("Exit program")); connect(exitAction,SIGNAL(triggered()),this,SLOT(close())); //Copy action copyAction =new QAction(QIcon(":/image/copy.png"),tr("copy"),this); copyAction->setShortcut(tr("Ctrl+C")); copyAction->setStatusTip(tr("Copy file")); connect(copyAction,SIGNAL(triggered()),showWidget->text,SLOT (copy())); //Cut action cutAction =new QAction(QIcon(":/image/cut.png"),tr("shear"),this); cutAction->setShortcut(tr("Ctrl+X")); cutAction->setStatusTip(tr("Cut file")); connect(cutAction,SIGNAL(triggered()),showWidget->text,SLOT (cut())); //Paste action pasteAction =new QAction(QIcon(":/image/paste.png"),tr("paste"),this); pasteAction->setShortcut(tr("Ctrl+V")); pasteAction->setStatusTip(tr("Paste file")); connect(pasteAction,SIGNAL(triggered()),showWidget->text,SLOT (paste())); //About action aboutAction =new QAction(tr("about"),this); connect(aboutAction,SIGNAL(triggered()),this,SLOT (QApplication::aboutQt())); //Print text action PrintTextAction =new QAction(QIcon(":/image/printText.png"),tr("Print text"), this); PrintTextAction->setStatusTip(tr("Print a text")); connect(PrintTextAction,SIGNAL(triggered()),this,SLOT(ShowPrintText())); //Print image action PrintImageAction =new QAction(QIcon(":/image/printImage.png"),tr("Print image"), this); PrintImageAction->setStatusTip(tr("Print an image")); connect(PrintImageAction,SIGNAL(triggered()),this,SLOT(ShowPrintImage())); //Zoom in action zoomInAction =new QAction(QIcon(":/image/zoomin.png"),tr("enlarge"),this); zoomInAction->setStatusTip(tr("Enlarge a picture")); connect(zoomInAction,SIGNAL(triggered()),this,SLOT(ShowZoomIn())); //Zoom out action zoomOutAction =new QAction(QIcon(":/image/zoomout.png"),tr("narrow"),this); zoomOutAction->setStatusTip(tr("Zoom out a picture")); connect(zoomOutAction,SIGNAL(triggered()),this,SLOT(ShowZoomOut())); //Action for image rotation //Rotate 90 ° rotate90Action =new QAction(QIcon(":/image/rotate90.png"),tr("Rotate 90°"),this); rotate90Action->setStatusTip(tr("Rotate a picture 90°")); connect(rotate90Action,SIGNAL(triggered()),this,SLOT(ShowRotate90())); //Rotate 180 ° rotate180Action =new QAction(QIcon(":/image/rotate180.png"),tr("Rotate 180°"), this); rotate180Action->setStatusTip(tr("Rotate a picture 180°")); connect(rotate180Action,SIGNAL(triggered()),this,SLOT(ShowRotate180())); //Rotate 270 ° rotate270Action =new QAction(QIcon(":/image/rotate270.png"),tr("Rotate 270°"), this); rotate270Action->setStatusTip(tr("Rotate a picture 270°")); connect(rotate270Action,SIGNAL(triggered()),this,SLOT(ShowRotate270())); //Action to implement image mirroring //Portrait mirror mirrorVerticalAction =new QAction(QIcon(":/image/mirrorVertical.png"), tr ("Portrait mirror"),this); mirrorVerticalAction->setStatusTip(tr("Mirror a picture vertically")); connect(mirrorVerticalAction,SIGNAL(triggered()),this,SLOT(ShowMirrorVertical())); //Landscape mirror mirrorHorizontalAction =new QAction(QIcon(":/image/mirrorHorizontal.png"), tr("Landscape mirror"),this); mirrorHorizontalAction->setStatusTip(tr("Mirror a picture horizontally")); connect(mirrorHorizontalAction,SIGNAL(triggered()),this,SLOT(ShowMirrorHorizontal())); //Sort: align left, align right, center, and align both ends actGrp =new QActionGroup(this); leftAction =new QAction(QIcon(":/image/left.png"),"Align left",actGrp); leftAction->setCheckable(true); rightAction =new QAction(QIcon(":/image/right.png"),"Right align",actGrp); rightAction->setCheckable(true); centerAction =new QAction(QIcon(":/image/center.png"),"Center",actGrp); centerAction->setCheckable(true); justifyAction =new QAction(QIcon(":/image/justify.png"),"Align both ends",actGrp); justifyAction->setCheckable(true); connect(actGrp,SIGNAL(triggered(QAction*)),this,SLOT(ShowAlignment (QAction*))); //Action to undo and restore //Revocation and reinstatement undoAction =new QAction(QIcon(":/image/undo.png"),"revoke",this); connect(undoAction,SIGNAL(triggered()),showWidget->text,SLOT (undo())); redoAction =new QAction(QIcon(":/image/redo.png"),"redo",this); connect(redoAction,SIGNAL(triggered()),showWidget->text,SLOT (redo())); } void ImgProcessor::createMenus() { //File menu fileMenu =menuBar()->addMenu(tr("file")); //Directly call the menuBar() function of QMainWindow to get the menu bar pointer of the main window, and then call the addMenu() function of QMenuBar to insert a new menu fileMenu in the menu bar. fileMenu is a QMenu class object. fileMenu->addAction(openFileAction); //Call the addAction() function of QMenu and add the menu items "open", "new", "print text" and "print image" to the menu. fileMenu->addAction(NewFileAction); fileMenu->addAction(PrintTextAction); fileMenu->addAction(PrintImageAction); fileMenu->addSeparator(); //Add split line fileMenu->addAction(exitAction); //Zoom menu zoomMenu =menuBar()->addMenu(tr("edit")); zoomMenu->addAction(copyAction); zoomMenu->addAction(cutAction); zoomMenu->addAction(pasteAction); zoomMenu->addAction(aboutAction); zoomMenu->addSeparator(); zoomMenu->addAction(zoomInAction); zoomMenu->addAction(zoomOutAction); //Rotate menu rotateMenu =menuBar()->addMenu(tr("rotate")); rotateMenu->addAction(rotate90Action); rotateMenu->addAction(rotate180Action); rotateMenu->addAction(rotate270Action); //Mirror menu mirrorMenu =menuBar()->addMenu(tr("image")); mirrorMenu->addAction(mirrorVerticalAction); mirrorMenu->addAction(mirrorHorizontalAction); } void ImgProcessor::createToolBars() { //File toolbar fileTool =addToolBar("File"); //Directly call the addToolBar() function of QMainWindow to obtain the toolbar object of the main window. Call the addToolBar() function every time a toolbar is added, and give a different name to add a toolbar in the main window fileTool->addAction(openFileAction); //Call the addAction() function of QToolBar to insert actions belonging to this toolbar in the toolbar. Similarly, implement Edit Toolbar rotate toolbar undo and redo toolbar. The display of toolbar can be selected by the user. Right click on the toolbar to pop up the selection menu of toolbar display. The user can select the toolbar to be displayed. fileTool->addAction(NewFileAction); fileTool->addAction(PrintTextAction); fileTool->addAction(PrintImageAction); //Edit Toolbar zoomTool =addToolBar("Edit"); zoomTool->addAction(copyAction); zoomTool->addAction(cutAction); zoomTool->addAction(pasteAction); zoomTool->addSeparator(); zoomTool->addAction(zoomInAction); zoomTool->addAction(zoomOutAction); //Rotate toolbar rotateTool =addToolBar("rotate"); rotateTool->addAction(rotate90Action); rotateTool->addAction(rotate180Action); rotateTool->addAction(rotate270Action); //Undo and redo toolbar doToolBar =addToolBar("doEdit"); doToolBar->addAction(undoAction); doToolBar->addAction(redoAction); //Font toolbar fontToolBar =addToolBar("Font"); fontToolBar->addWidget(fontLabel1); fontToolBar->addWidget(fontComboBox); fontToolBar->addWidget(fontLabel2); fontToolBar->addWidget(sizeComboBox); fontToolBar->addSeparator(); fontToolBar->addWidget(boldBtn); fontToolBar->addWidget(italicBtn); fontToolBar->addWidget(underlineBtn); fontToolBar->addSeparator(); fontToolBar->addWidget(colorBtn); //Sort toolbar listToolBar =addToolBar("list"); listToolBar->addWidget(listLabel); listToolBar->addWidget(listComboBox); listToolBar->addSeparator(); listToolBar->addActions(actGrp->actions()); } void ImgProcessor::ShowNewFile() //New file function { ImgProcessor *newImgProcessor =new ImgProcessor; newImgProcessor->show(); } void ImgProcessor::ShowOpenFile() { fileName =QFileDialog::getOpenFileName(this); if(!fileName.isEmpty()) { if(showWidget->text->document()->isEmpty()) //Check whether there is an open file in the current central form { loadFile(fileName); } else { ImgProcessor *newImgProcessor =new ImgProcessor; newImgProcessor->show(); newImgProcessor->loadFile(fileName); } } } void ImgProcessor::loadFile(QString filename) //Read the contents of the file { printf("file name:%s\n",filename.data()); QFile file(filename); if(file.open(QIODevice::ReadOnly|QIODevice::Text)) { QTextStream textStream(&file); while(!textStream.atEnd()) { showWidget->text->append(textStream.readLine()); printf("read line\n"); } printf("end\n"); } } void ImgProcessor::ShowPrintText() { QPrinter printer; //Create a new QPrinter object QPrintDialog printDialog(&printer,this); //Create a QPrintDialog object with the parameter QPrinter object if(printDialog.exec()) //Judge whether the user clicks print after the standard print dialog box is displayed. If you click Print, the relevant print attributes can be obtained from the QPrinter object used when creating the QPrintDialog object; If the user clicks the Cancel button, subsequent printing operations will not be performed. { //Get the document of QTextEdit object QTextDocument *doc =showWidget->text->document(); doc->print(&printer); //Print } } void ImgProcessor::ShowPrintImage() { QPrinter printer; //Create a new QPrinter object QPrintDialog printDialog(&printer,this); //Create a QPrintDialog object with the parameter QPrinter object if(printDialog.exec()) { QPainter painter(&printer); //Create a QPainter object and specify the drawing device as a QPrinter object QRect rect =painter.viewport(); //Gets the rectangular area of the view of the QPainter object QSize size = img.size(); //Gets the size of the image /* Resets the rectangular area of the view according to the scale size of the drawing */ size.scale(rect.size(),Qt::KeepAspectRatio); painter.setViewport(rect.x(),rect.y(),size.width(),size.height()); painter.setWindow(img.rect()); //Set the QPainter window size to the size of the image painter.drawImage(0,0,img); //Print image } } void ImgProcessor::ShowZoomIn() { if(img.isNull()) //Validity judgment return; QMatrix matrix; //Declare an instance of the QMatrix class matrix.scale(2,2); //Zoom in horizontally and vertically at 2x scale img = img.transformed(matrix); //And convert the currently displayed graphics according to the coordinate matrix //Reset display graphics showWidget->imageLabel->setPixmap(QPixmap::fromImage(img)); } void ImgProcessor::ShowZoomOut() { if(img.isNull()) return; QMatrix matrix; matrix.scale(0.5,0.5); //Reduce the horizontal and vertical directions by 0.5x img = img.transformed(matrix); showWidget->imageLabel->setPixmap(QPixmap::fromImage(img)); } void ImgProcessor::ShowRotate90() { if(img.isNull()) return; QMatrix matrix; matrix.rotate(90); //Rotate 90 degrees img = img.transformed(matrix); showWidget->imageLabel->setPixmap(QPixmap::fromImage(img)); } void ImgProcessor::ShowRotate180() { if(img.isNull()) return; QMatrix matrix; matrix.rotate(180); img = img.transformed(matrix); showWidget->imageLabel->setPixmap(QPixmap::fromImage(img)); } void ImgProcessor::ShowRotate270() { if(img.isNull()) return; QMatrix matrix; matrix.rotate(270); img = img.transformed(matrix); showWidget->imageLabel->setPixmap(QPixmap::fromImage(img)); } void ImgProcessor::ShowMirrorVertical() { if(img.isNull()) return; img=img.mirrored(false,true); //mirror vertically showWidget->imageLabel->setPixmap(QPixmap::fromImage(img)); } void ImgProcessor::ShowMirrorHorizontal() { if(img.isNull()) return; img=img.mirrored(true,false); //Horizontal mirror showWidget->imageLabel->setPixmap(QPixmap::fromImage(img)); } void ImgProcessor::ShowFontComboBox(QString comboStr) //Set font { QTextCharFormat fmt; //Create a QTextCharFormat object fmt.setFontFamily(comboStr); //The selected font name is set to the QTextCharFormat object mergeFormat(fmt); //Applies the new format to the characters in the cursor selection } void ImgProcessor::mergeFormat(QTextCharFormat format) { QTextCursor cursor =showWidget->text->textCursor(); //Gets the cursor in the edit box if(!cursor.hasSelection()) //Determine whether to select text cursor.select(QTextCursor::WordUnderCursor); //Take the word where the cursor is located as the selection area, and distinguish the word by the front and back spaces or punctuation marks such as "," " cursor.mergeCharFormat(format); //Call the mergeCharFormat() function of QTextCursor to apply the format represented by the parameter format to the character where the cursor is located showWidget->text->mergeCurrentCharFormat(format); //Call the merge CurrentCharFormat() function of QTextEdit to apply the format to all characters in the selection } void ImgProcessor::ShowSizeSpinBox(QString spinValue) //Set font size { QTextCharFormat fmt; fmt.setFontPointSize(spinValue.toFloat()); //Set the font size according to the value of spinValue showWidget->text->mergeCurrentCharFormat(fmt); } void ImgProcessor::ShowBoldBtn() //Set text display bold { QTextCharFormat fmt; fmt.setFontWeight(boldBtn->isChecked()?QFont::Bold:QFont::Normal);//Set thickness value showWidget->text->mergeCurrentCharFormat(fmt); } void ImgProcessor::ShowItalicBtn() //Set text display Italic { QTextCharFormat fmt; fmt.setFontItalic(italicBtn->isChecked()); showWidget->text->mergeCurrentCharFormat(fmt); } void ImgProcessor::ShowUnderlineBtn() //Set text underline { QTextCharFormat fmt; fmt.setFontUnderline(underlineBtn->isChecked()); showWidget->text->mergeCurrentCharFormat(fmt); } void ImgProcessor::ShowColorBtn() //Set text color { QColor color=QColorDialog::getColor(Qt::red,this); //Use the color dialog box to set the font color if(color.isValid()) { QTextCharFormat fmt; fmt.setForeground(color); showWidget->text->mergeCurrentCharFormat(fmt); } } void ImgProcessor::ShowCurrentFormatChanged(const QTextCharFormat &fmt) //Triggered when the character format changes { fontComboBox->setCurrentIndex(fontComboBox->findText(fmt.fontFamily())); sizeComboBox->setCurrentIndex(sizeComboBox->findText(QString::number(fmt.fontPointSize()))); boldBtn->setChecked(fmt.font().bold()); italicBtn->setChecked(fmt.fontItalic()); underlineBtn->setChecked(fmt.fontUnderline()); } void ImgProcessor::ShowAlignment(QAction *act) { if(act==leftAction) showWidget->text->setAlignment(Qt::AlignLeft);//Align left if(act==rightAction) showWidget->text->setAlignment(Qt::AlignRight); if(act==centerAction) showWidget->text->setAlignment(Qt::AlignCenter); if(act==justifyAction) showWidget->text->setAlignment(Qt::AlignJustify); } void ImgProcessor::ShowCursorPositionChanged() { if(showWidget->text->alignment()==Qt::AlignLeft) leftAction->setChecked(true); if(showWidget->text->alignment()==Qt::AlignRight) rightAction->setChecked(true); if(showWidget->text->alignment()==Qt::AlignCenter) centerAction->setChecked(true); if(showWidget->text->alignment()==Qt::AlignJustify) justifyAction->setChecked(true); } void ImgProcessor::ShowList(int index) { //Gets the QTextCursor object pointer of the edit box QTextCursor cursor=showWidget->text->textCursor(); if(index!=0) { QTextListFormat::Style style=QTextListFormat::ListDisc;//(a) switch(index) //Set style property value { default: case 1: style=QTextListFormat::ListDisc; break; case 2: style=QTextListFormat::ListCircle; break; case 3: style=QTextListFormat::ListSquare; break; case 4: style=QTextListFormat::ListDecimal; break; case 5: style=QTextListFormat::ListLowerAlpha; break; case 6: style=QTextListFormat::ListUpperAlpha; break; case 7: style=QTextListFormat::ListLowerRoman; break; case 8: style=QTextListFormat::ListUpperRoman; break; } /* Set indent value */ //(b) cursor.beginEditBlock(); QTextBlockFormat blockFmt=cursor.blockFormat(); QTextListFormat listFmt; if(cursor.currentList()) { listFmt= cursor.currentList()->format(); } else { listFmt.setIndent(blockFmt.indent()+1); blockFmt.setIndent(0); cursor.setBlockFormat(blockFmt); } listFmt.setStyle(style); cursor.createList(listFmt); cursor.endEditBlock(); } else { QTextBlockFormat bfmt; bfmt.setObjectIndex(-1); cursor.mergeBlockFormat(bfmt); } } ImgProcessor::~ImgProcessor() { }
5. mian file
#include "imgprocessor.h" #include <QApplication> int main(int argc, char *argv[]) { QApplication a(argc, argv); QFont f("ZYSong18030",12); //Format the displayed font a.setFont(f); ImgProcessor w; w.show(); return a.exec(); }