Let's have a look at the renderings first, and then the code.
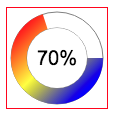
1. The HTML part of canvas is simply a canvas tag
The width and height of canvas is its own property. You should set the style between lines. If you set the width and height in style, the picture you draw will be deformed.
<canvas id="mycanvas" width="100" height="100"> 70% </canvas>
2. js code of canvas
Main idea: the rendering is composed of three circles, the outermost layer is a big circle with black edge, and the inner circle changes the progress of the progress bar and a real percentage circle.
Note: a new layer should be created for each circle drawn, so that the style of each layer can be set independently without mutual influence. Like ps layer, a complete design draft is composed of many layers.
var canvas = document.getElementById("mycanvas"); var context = canvas.getContext("2d"); function draw(i){ // Large round frame context.beginPath(); context.lineWidth = 1; context.arc(50,50,46,0,Math.PI*2); context.strokeStyle = "grey"; context.stroke(); // Great circle context.beginPath(); var grd = context.createLinearGradient(15,15,80,80); grd.addColorStop(0,"red"); grd.addColorStop(0.5,"yellow"); grd.addColorStop(1,"blue"); context.arc(50,50,38,0,Math.PI*2*(i/100)); context.lineWidth = 16; context.strokeStyle = grd; context.stroke(); // context.fillStyle = grd; // context.fill(); // Small circle context.beginPath(); context.arc(50,50,30,0,Math.PI*2); context.lineWidth = 1; context.strokeStyle = "grey"; context.stroke(); context.fillStyle = "white"; context.fill(); // word context.beginPath(); context.textBaseline = "middle"; context.textAlign = "center"; context.font = "20px Arial"; context.fillStyle = "black"; context.fillText(i+"%",50,50); }
3. Use the timer to refresh the canvas to achieve the effect of progress bar
Use the context.clearRect() method to empty the
var i = 0; var progress = parseInt(canvas.innerHTML); // console.log(progress); var timer = setInterval(function(){ if(i >= progress){ clearInterval(timer); } context.clearRect(0,0,canvas.width,canvas.height); draw(i); i++; },50);