Front words
This article will use canvas Implementing Particle Clock Effect
Effect display
Dot matrix number
digit.js It is a three-dimensional array that contains two-dimensional lattices of 0 to 9 and a colon (digit[10]). The lattice representation of each number is a two-dimensional array of 7*10 sizes.
By traversing the two-dimensional array of digital lattices, when the value of the position is 1, a particle is drawn, otherwise it is not drawn.
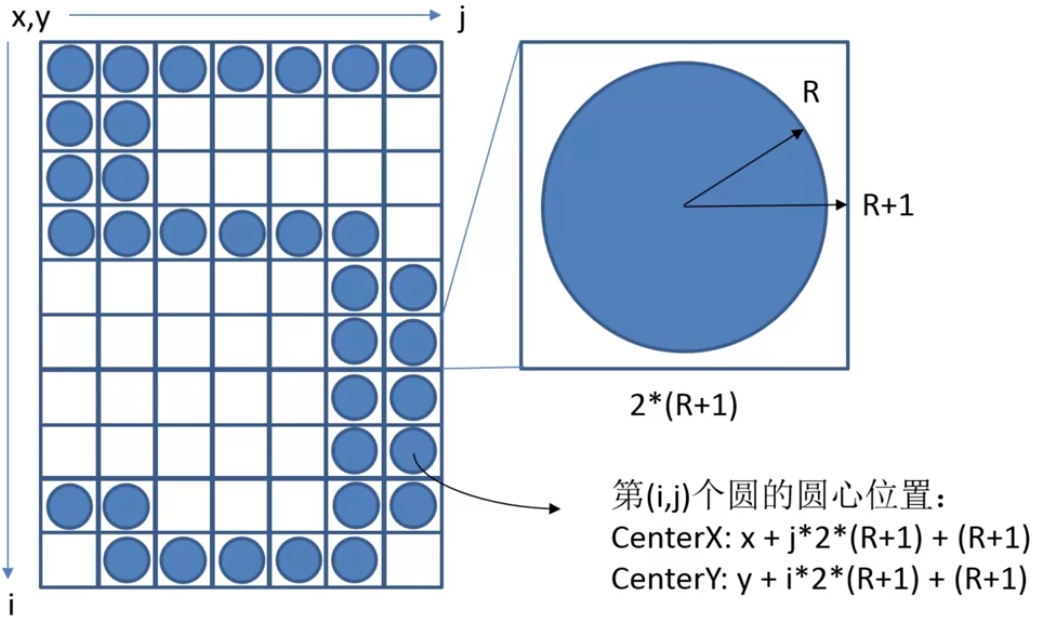
Name the function that draws numbers renderDigit(). In this function, the particle is plotted as a small circle. The radius of the small circle is R, and the width (height) of the rectangle occupied by the small circle is 2(R+1). Since the digital lattice is a two-dimensional array of 10*7, a number has a width of 14(R+1) and a height of 20(R+1).
Assuming the height of the number is 100px, the radius of the circle R=4
<div id="test"> <button>1</button> <button>2</button> <button>3</button> <button>4</button> <button>5</button> <button>6</button> <button>7</button> <button>8</button> <button>9</button> <button>10</button> </div> <canvas id="canvas">Current browsers do not support canvas,Please change the browser and try again.</canvas> <script src="http://files.cnblogs.com/files/xiaohuochai/digit.js"></script> <script>var canvas = document.getElementById('canvas'); if(canvas.getContext){ var cxt = canvas.getContext('2d'); } function renderDigit(num){ //Reset the width of the canvas to clear the canvas canvas.height = 100; var R = canvas.height/20-1; for(var i = 0; i < digit[num].length; i++){ for(var j = 0; j < digit[num][i].length; j++){ if(digit[num][i][j] == 1){ cxt.beginPath(); cxt.arc(j*2*(R+1)+(R+1),i*2*(R+1)+(R+1),R,0,2*Math.PI); cxt.closePath(); cxt.fill(); } } } } var test = document.getElementById('test'); test.onclick = function(e){ e = e || event; var target = e.target || e.srcElement; if(!isNaN(target.innerHTML)){ renderDigit(target.innerHTML); } } </script>
Clock implementation
Based on the lattice number of the previous step, a particle clock is implemented. The function of clock implementation is named digitTime(). Clock implementation consists of two parts: acquiring time data and rendering clock.
[Time data]
The simplest form of clock consists of two hours, two minutes and two seconds separated by colons. adopt Date object To get the current time, as well as the current hours, minutes and seconds. But what you ultimately need is a digital clock.
For example, 12:02:36 time data is represented in data[1,2,10,0,2,10,3,6]
[Rendering Clock]
After obtaining the time data, renderDigit() is recycled to render every number in the clock. At this point, one thing that needs to be changed is the x coordinates in the arc() function, otherwise they will be superimposed.
In order to make the digital representation of clocks clearer, a certain distance is added between each number. The width of each digit is 14(R+1). Assuming that the index of seven digits in the data array is index, the starting X coordinate of each digit can be equal to 14(R+2)*index.
Finally adopted timer Update time after each interval
<canvas id="canvas" style="width:400px;">Current browsers do not support canvas,Please change the browser and try again.</canvas> <script src="http://files.cnblogs.com/files/xiaohuochai/digit.js"></script> <script> var canvas = document.getElementById('canvas'); if(canvas.getContext){ var cxt = canvas.getContext('2d'); } canvas.height = 100; canvas.width = 700; function renderDigit(index,num){ var R = canvas.height/20-1; for(var i = 0; i < digit[num].length; i++){ for(var j = 0; j < digit[num][i].length; j++){ if(digit[num][i][j] == 1){ cxt.beginPath(); cxt.arc(14*(R+2)*index + j*2*(R+1)+(R+1),i*2*(R+1)+(R+1),R,0,2*Math.PI); cxt.closePath(); cxt.fill(); } } } } function digitTime(){ /*Obtaining time data*/ var temp = /(\d)(\d):(\d)(\d):(\d)(\d)/.exec(new Date()); //Storage time digits consist of seven digits: ten-bit hour, one-bit hour, colon, ten-bit minute, one-bit minute, colon, ten-bit second and one-bit second. var data = []; data.push(temp[1],temp[2],10,temp[3],temp[4],10,temp[5],temp[6]); /*Render clock*/ //Reset the width of the canvas to clear the canvas canvas.height = 100; for(var i = 0; i < data.length; i++){ renderDigit(i,data[i]); } } digitTime(); clearInterval(oTimer); var oTimer = setInterval(function(){ digitTime(); },500); </script>
Stochastic parabola
The random parabolic motion in this section is the preparation for the next section of particle animation. With DOM nodes Throwing against the wall On the basis of this, a random parabolic motion of a small sphere is realized by canvas
Split the motion of the ball into x-axis and y-axis. The X-axis moves at a uniform speed, and the y-axis first slows down upward and then accelerates downward. Stop the timer when the ball leaves the canvas area
<button id="btn">Button</button> <canvas id="canvas" style="border:1px solid black">Current browsers do not support canvas,Please change the browser and try again.</canvas> <script> var canvas = document.getElementById('canvas'); if(canvas.getContext){ var cxt = canvas.getContext('2d'); } //statement canvas Width and height var H = 100,W = 200; canvas.height = H; canvas.width = W; var R = canvas.height/20-1; var numArray = [1,2,3,4]; var colorArray = ["#3BE","#09C","#A6C","#93C","#9C0","#690","#FB3","#F80","#F44","#C00"]; btn.onclick = function(){ //statement x,y Axis coordinate var x=Math.floor(Math.random() * 60 + 10); var y=Math.floor(Math.random() * 60 + 10); //statement x,y Steps of axes var stepY = -3*numArray[Math.floor(Math.random()*numArray.length)]; var stepX = Math.floor(Math.random() * 10 -5); //statement y Axis change value var disY = numArray[Math.floor(Math.random()*numArray.length)]; var color =colorArray[Math.floor(Math.random()*colorArray.length)]; clearInterval(oTimer); var oTimer = setInterval(function(){ stepY += disY; x += stepX; y += stepY; canvas.height = 100; cxt.beginPath(); cxt.arc(x,y,R,0,2*Math.PI); cxt.fillStyle = color; cxt.closePath(); cxt.fill(); if(x > W + R || y > H + R){ clearInterval(oTimer); } },50); } </script>
Particle animation
The following is the implementation of particle animation. In the instant of time-number change, the same particles are generated repeatedly on the new numbers composed of many particles, and the newly generated particles do random parabolic motion.
Therefore, the first step is to determine which or which numbers have changed when the time is updated. Then, through these change information, the ball to be moved is generated. During the running interval of the timer, the status of the moving ball is updated. Finally, uniform rendering of clocks and running balls
<canvas id="canvas" style="width:500px;">Current browsers do not support canvas,Please change the browser and try again.</canvas> <script src="http://files.cnblogs.com/files/xiaohuochai/digit.js"></script> <script> var canvas = document.getElementById('canvas'); if(canvas.getContext){ var cxt = canvas.getContext('2d'); } //statement canvas Width and height var H = 100,W = 700; canvas.height = H; canvas.width = W; //Storage time data var data = []; //Storage of moving balls var balls = []; //Setting particle radius var R = canvas.height/20-1; (function(){ var temp = /(\d)(\d):(\d)(\d):(\d)(\d)/.exec(new Date()); //Storage time digits consist of seven digits: ten-bit hour, one-bit hour, colon, ten-bit minute, one-bit minute, colon, ten-bit second and one-bit second. data.push(temp[1],temp[2],10,temp[3],temp[4],10,temp[5],temp[6]); })(); /*Generating Lattice Numbers*/ function renderDigit(index,num){ for(var i = 0; i < digit[num].length; i++){ for(var j = 0; j < digit[num][i].length; j++){ if(digit[num][i][j] == 1){ cxt.beginPath(); cxt.arc(14*(R+2)*index + j*2*(R+1)+(R+1),i*2*(R+1)+(R+1),R,0,2*Math.PI); cxt.closePath(); cxt.fill(); } } } } /*Update clock*/ function updateDigitTime(){ var changeNumArray = []; var temp = /(\d)(\d):(\d)(\d):(\d)(\d)/.exec(new Date()); var NewData = []; NewData.push(temp[1],temp[2],10,temp[3],temp[4],10,temp[5],temp[6]); for(var i = data.length-1; i >=0 ; i--){ //Time changes if(NewData[i] !== data[i]){ //The numeric values that will change and the values that will change in the __________ data The index in the array is stored changeNumArray Array changeNumArray.push(i+'_'+(Number(data[i])+1)%10); } } //Increase ball for(var i = 0; i< changeNumArray.length; i++){ addBalls.apply(this,changeNumArray[i].split('_')); } data = NewData.concat(); } /*Update Ball Status*/ function updateBalls(){ for(var i = 0; i < balls.length; i++){ balls[i].stepY += balls[i].disY; balls[i].x += balls[i].stepX; balls[i].y += balls[i].stepY; if(balls[i].x > W + R || balls[i].y > H + R){ balls.splice(i,1); i--; } } } /*Increase the number of balls to play*/ function addBalls(index,num){ var numArray = [1,2,3]; var colorArray = ["#3BE","#09C","#A6C","#93C","#9C0","#690","#FB3","#F80","#F44","#C00"]; for(var i = 0; i < digit[num].length; i++){ for(var j = 0; j < digit[num][i].length; j++){ if(digit[num][i][j] == 1){ var ball = { x:14*(R+2)*index + j*2*(R+1)+(R+1), y:i*2*(R+1)+(R+1), stepX:Math.floor(Math.random() * 4 -2), stepY:-2*numArray[Math.floor(Math.random()*numArray.length)], color:colorArray[Math.floor(Math.random()*colorArray.length)], disY:1 }; balls.push(ball); } } } } /*Rendering*/ function render(){ //Reset the width of the canvas to clear the canvas canvas.height = 100; //Render clock for(var i = 0; i < data.length; i++){ renderDigit(i,data[i]); } //Render ball for(var i = 0; i < balls.length; i++){ cxt.beginPath(); cxt.arc(balls[i].x,balls[i].y,R,0,2*Math.PI); cxt.fillStyle = balls[i].color; cxt.closePath(); cxt.fill(); } } clearInterval(oTimer); var oTimer = setInterval(function(){ //Update clock updateDigitTime(); //Update Ball Status updateBalls(); //Rendering render(); },50); </script>
Bulletin Board Extension
The js part of canvas particle clock is encapsulated as canvasTime.js Add the following code to the bulletin board to achieve the effect of inserting a clock in the bulletin board
<canvas id="canvas" style="width:100%;">Current browser does not support canvas, please change the browser and try again </canvas>. <script src="http://files.cnblogs.com/files/xiaohuochai/canvasTime.js"></script>
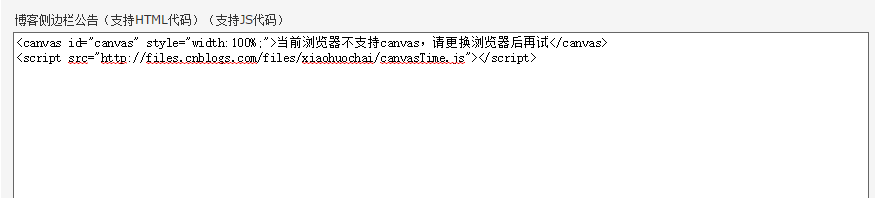
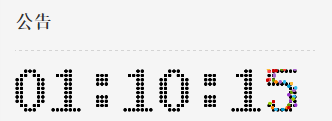