Summary of knowledge points:
C++ I/O: Definition in iostream header file istream // Universal Input Stream and other Input Stream Base Classes. ostream // Universal Output Stream and other stream-based classes. iostream//Universal I/O Stream and other I/O Stream Base Classes. Definition in fstream header file ofstream // File Write Operation Memory Write to Storage Device ifstream // File Read Operation, Storage Device Read Area into Memory fstream // read and write operation, open files can be read and write operation Definition in strstream header file istrstream // input string stream ostrstream // Output String Stream strstream // I/O String Stream cin,cout: cin, the object of a derived class of istream, is the standard input stream. cout, the object of a derived class of ostream, is the standard output stream. Operation steps: (1) Establishing file stream objects; (2) Opening or establishing documents; (3) read and write operations; (4) Close files; Format control output: dec 10-ary output, hex 16-ary output oct 8-ary output. setfill(c) sets the fill character c, and setprecision (n) sets the n-bit accuracy of the real number. setw(n) Set field width n setiosflags(ios::fixed) Sets floating-point numbers to display in fixed decimal digits setiosflags(ios::left) output data is left aligned, and setiosflags(ios::right) output data is right aligned. Class ios: void open(const char* filename,ios_base::openmode mode); How do mode open files ios::binary: Open files in binary mode, default mode is text mode ios::in: Files are opened as input (file data is entered into memory) (this is the default way for ifstream objects) ios::out: Files are opened as output (memory data is exported to files) (the default way to open ofstream objects) Common sense: Text file and binary file Text file: It is composed of character sequence. It takes character as the smallest unit of information access. It is also called ASCII code file. Binary file: composed of binary numbers. Relevant functions: void open( const char *filename ); // The function open() is used for file streaming. It opens filename and associates it with the current stream. void close(); // Function closes the associated file stream. ostream &flush(); // The flush() function can cause the buffer of the front stream to be written out to the attached device. int get(); // There are many overloaded functions to read a character and return its value. ostream &put( char ch ); // The function put() is used for the output stream and the character ch is written into the stream. istream &get( char &ch ); // Read a character and store it in ch. istream &getline( char *buffer, streamsize num ); // The getline() function is used for input streams to read characters into buffer s. istream &read( char *buffer, streamsize num ); // The function read() is used for the input stream to read num bytes from the stream before putting characters into the buffer. If you encounter EOF, read() stop ostream & write (const char * buffer, streamsize num); // The write() function is used to write num bytes from the buffer to the current output stream.
Look at a graph of I/O related classes
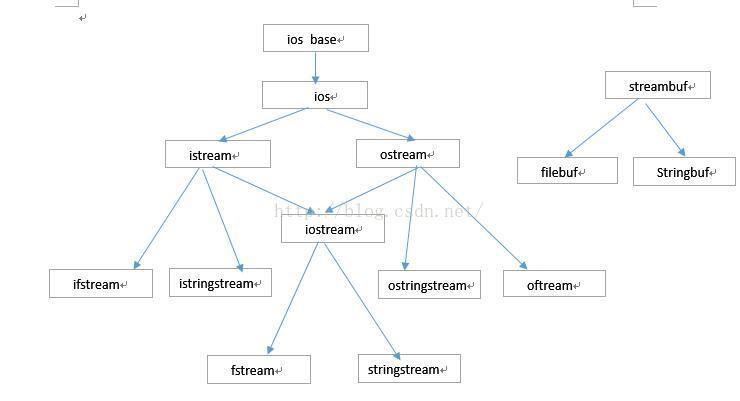
Diagram of c++ Stream. jpg
Interpretation of Common Objects
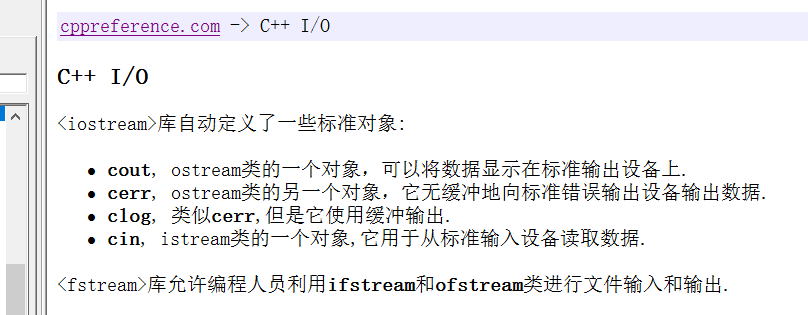
c++ I-O.PNG
Look at code
#include<iostream> #include<string> #include<fstream> #include<iomanip> void display(); void writeFile(); void readFile(); void readBinaryFile(); void copyFile(); using namespace std; int main() { display(); //The use of format controllers. writeFile(); readFile(); readBinaryFile(); copyFile(); system("pause"); return 0; } void display() { int a = 123; float b = 1.2345f; double c = 1.23456; string d = "dflx"; cout << "dec:" << dec << a << endl; cout << "hex:" << hex << a << endl; cout << "oct:" << oct << a << endl; cout << setw(5) << d << endl; cout << setfill('*') << setw(5) << d << endl; cout << setw(5) << setiosflags(ios::right) << a << endl; cout << setw(5) << setiosflags(ios::left) << a << endl; cout << setiosflags(ios::scientific) << setprecision(8); cout << "c=" << c << endl; cout << "c=" << setprecision(5) << c << endl; cout << "------------------"<<endl; cout.put('a') << endl; putchar('b'); cout << endl; cout << "enter a sentence:"; int ch; while ((ch = cin.get()) != '#') cout.put(ch); cout <<endl<< "--------------------Format Control End------------------------" << endl; } //Textual Writing void writeFile() { /* ofstream outfile; Establishing ifstream objects outfile.open("D:\\workspace_java\\File\\g.txt"); Associating Objects with Files Equivalent. */ cout << "-----------Write text files------------" << endl; ofstream outfile("D:\\workspace_java\\File\\g.txt", ios::out); if (!outfile) { cerr << "open error" << endl; exit(1); } string str= "hello c plus plus \n"; outfile << str; //Use this object to output data to a file outfile.close(); } //Textual reading void readFile() { cout << "-----------Read text files------------" << endl; ifstream ifs; ifs.open("D:\\workspace_java\\File\\g.txt"); char str[255]; //Define an array of characters //Ifs > > str; // Read the data in the file into str. But the c++ insert operator stops output when it encounters null characters. ifs.getline(str,255); cout << str << endl; cout << "------------------" << endl; /*If we want to write a paragraph on the command line, and the replacement includes spaces and carriage returns, So we should use the third parameter of the getline() function above.*/ ifstream file("D:\\workspace_java\\File\\a.txt"); if (!file) { cerr << "Failed to open the file" << endl; } char str1[1024]; file.getline(str1, 255, 0); cout << str1 << endl; } /* For binary file reading and writing, we must emphasize that opening file properties must add: ios::binary, In fact, it tells the system that I have to read and write files in binary format. */ //Binary file reading void readBinaryFile() { cout << "-----------Read binary files------------" << endl; ifstream file; file.open("D:\\workspace_java\\File\\g.txt",::ios::binary); if (!file) { cerr << "Failed to open the file" << endl; } char str[1024] = { 0 }; file.read(str, sizeof(char) * 1024); cout << str << endl; file.close(); } /* Remember, write has two parameters, the first is the character array parameter, and the second is the data that needs to be entered into the file. Size Lift up a son: ofstream fout("text.bat", ios::binary);Relevant time. string str = "Hello word"; fout.write(str.c_str(),sizeof(char)*(str.size())); */ //File replication void copyFile() { ifstream copy_file("D:\\workspace_java\\File\\Soft spring rain.txt"); ofstream new_file("D:\\workspace_java\\File\\My spring rain.txt"); if (!copy_file || !copy_file) { cerr << "Failed to open the file" << endl; return; } while (!copy_file.eof()) { char buf[1024]; copy_file.read(buf, sizeof(char) * 1024); new_file.write(buf, sizeof(char) * 1024); } cout << "Successful File Replication" << endl; }
give the result as follows
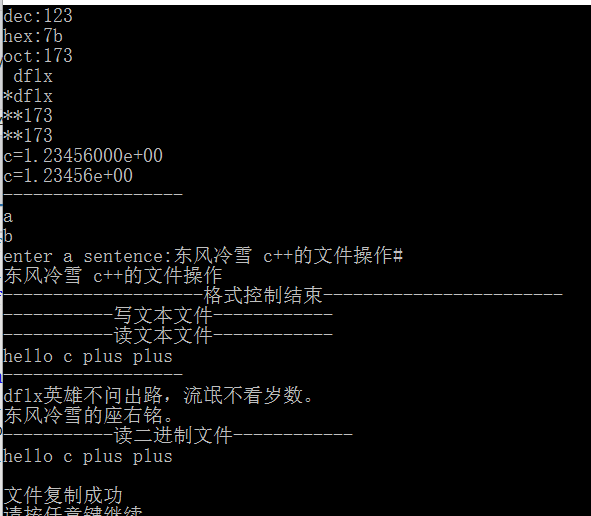
Result.PNG
Summary of key knowledge points:
Summarize a few important things: //The flow of file operation (1)Establishing File Stream Objects; ofstream //File Write Operation Memory Write Storage Device ifstream //File read operation, storage device read area into memory (2)Open or create files; void open(const char* filename,ios_base::openmode mode); mode How to open a file: ios::binary: Open files in binary mode, default mode is text ios::in: Files are opened as input(File data entered into memory)(ifstream This is the default way for objects. ios::out: Files are opened as output(Output of memory data to files)(ofstream Default Opening Method for Objects (3)Read and write; istream &getline( char *buffer, streamsize num ); istream &read( char *buffer, streamsize num ); //The function read() is used for the input stream to read num bytes from the stream before putting characters into the buffer. //If you encounter EOF, read() aborts ostream &write( const char *buffer, streamsize num ); //The write() function is used to write num bytes from the buffer to the current output stream. (4)Close file; close(); //In file replication: bool eof(); //If it reaches the end of the associated input file, the eof() function returns true, or false.
The I/O of c++ is not worse than that of java, but they are implemented in different ways.