Single thread IP address resolution
Article directory
Target program
- The interface is as follows
- Design method: complete the single IP address resolution, call the method circularly, and complete the scanning.
- Pay attention to calculating time with stopwatch.
Implementation ideas
- First know how to resolve a single IP address.
- Resolve multiple IP addresses in a circular way.
- Interface of design program
- Design event
Implementation process
Try the process in the console
//Ideas for implementation //The scanning addresses are set by ourselves. We need to set variables to save them. //The scanned IP address is composed of two ends, one address prefix, the other starting and ending values //We need to output IP address, scan time, and dns host name //IP address can be converted by IPAddress.Parse //It can be resolved through Dns.GetHostEntry //Scanning time can be counted by stopwatch //It is also necessary to determine whether the IP address is legal string addressPrefix = "127.0.0.";//Used to store address prefixes int startingValue = 1;//Storage start value int stopingValue = 2;//Storage termination value // if (startingValue <= stopingValue && startingValue > 0 && startingValue <= 255 && stopingValue > 0 && stopingValue <= 255) //{no if judgment //Loop to scan multiple IP addresses for (int i = startingValue; i <= stopingValue; i++) { string ipString = addressPrefix + i; IPAddress ip;//It can't be defined directly in try, otherwise it can't be used when there's a try IPHostEntry iPHostEntry; string iphostname; try//Judge whether it is legal { // IPAddress ip = IPAddress.Parse(ipString); ip = IPAddress.Parse(ipString); //Dns analysis // IPHostEntry iPHostEntry = Dns.GetHostEntry(ip); // string iphostname = iPHostEntry.HostName; / / get the ip hostname // Console.WriteLine($"scan address: {ip}, scan time, host DNS name: {iPhone}"); //Can't try a lot //Be precise. } catch (Exception ex) { Console.WriteLine("IP Illegal address"); break; } Stopwatch sw = new Stopwatch();//Instantiate a stopwatch object sw sw.Start();//Stopwatch starts try { //Dns analysis iPHostEntry = Dns.GetHostEntry(ip); iphostname = iPHostEntry.HostName;//Get the host name of ip sw.Stop();//Stopwatch end } catch (Exception ex) { iphostname = "Not online"; } long time = sw.ElapsedMilliseconds;//Get the time of this process Console.WriteLine($"Scan address:{ip},Scanning time:{time}Millisecond,Host DNS Name:{iphostname}"); } //string ipString = addressPrefix + startingValue; / / spliced into IP address string Console.ReadLine(); } /* else { Console.WriteLine("IP Illegal address'); Console.ReadLine(); }*//
Design WPF interface
<Window x:Class="WpfApp_SingleThreadIPScan.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:local="clr-namespace:WpfApp_SingleThreadIPScan" mc:Ignorable="d" Title="Single thread IP scanning" Height="450" Width="800" WindowStartupLocation="CenterScreen"> <Grid> <Grid.RowDefinitions> <RowDefinition Height="Auto" /> <RowDefinition Height="Auto" /> <RowDefinition /> </Grid.RowDefinitions> <GroupBox Grid.Row="0" Header="Scanned IP Address range" VerticalContentAlignment="Center" Padding="10"> <DockPanel> <Label DockPanel.Dock="Left" Content="Address prefix:"/> <TextBox Name="txt1" Text="192.168.1."/> <Label Content="Starting value:" Margin="15 0 0 0"/> <TextBox Name="txtStart" Text="102" Margin="0,4,0,0" VerticalAlignment="Top"/> <Label Content="Termination value:" Margin="15 0 0 0"/> <TextBox Name="txtEnd" Text="105"/> <Button Name="btn1" Width="60" VerticalAlignment="Center" Content="Start scanning" Click="Button_Click"/> </DockPanel> </GroupBox> <Label Name="labelError" Grid.Row="1" Background="Red" Foreground="White" Content="IP Wrong address, please correct!" HorizontalContentAlignment="Center" /> <GroupBox Grid.Row="2" Header="Scan information"> <ListBox x:Name="listBoxInfo"/> </GroupBox> </Grid> </Window>
Background code
using System; using System.Collections.Generic; using System.Diagnostics; using System.Linq; using System.Net; using System.Text; using System.Threading.Tasks; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Windows.Navigation; using System.Windows.Shapes; namespace WpfApp_SingleThreadIPScan { /// <summary> ///Interaction logic of MainWindow.xaml /// </summary> public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); labelError.Visibility = Visibility.Collapsed;//At the beginning, hide the error labels } private void TextBox_TextChanged(object sender, TextChangedEventArgs e) { string strip1 = txt1.Text + txtStart.Text; string strip2 = txt1.Text + txtEnd.Text; IPAddress ip1, ip2; if (IPAddress.TryParse(strip1, out ip1) == false || IPAddress.TryParse(strip2, out ip2) == false) { btn1.IsEnabled = false; labelError.Visibility = Visibility.Visible; return; } else { btn1.IsEnabled = true; labelError.Visibility = Visibility.Collapsed; } } private void Button_Click(object sender, RoutedEventArgs e) { int startvalue = int.Parse(txtStart.Text); int stopvalue = int.Parse(txtEnd.Text); if (startvalue > stopvalue) { MessageBox.Show("The starting value must be greater than the ending value. The address range is wrong. Please correct!"); } listBoxInfo.Items.Clear(); string ip = ""; for (int i = startvalue; i <= stopvalue; i++) { ip = txt1.Text + i; Scan(ip); } } private void Scan(string ip) { string hostname; Stopwatch sw = Stopwatch.StartNew(); try { hostname = Dns.GetHostEntry(ip).HostName; } catch(Exception ex) { hostname = "(Not online)"; } sw.Stop(); long time = sw.ElapsedMilliseconds; listBoxInfo.Items.Add($"Scan address:{ip},Scanning time:{time}Millisecond, DNS Host name:{hostname}"); } } }
Knowledge required
IP address
An IP address consists of two parts:
- Network number: identify the network to which this address belongs. It is assigned by the Internet authority.
- Host number: indicates the host within the network, which is uniformly assigned by the administrator of each network.
Address scheme:
-
IPv4 addressing scheme
- The binary value composed of four bytes (decimal representation) is recognized, separated by dots in the middle. This method is called dotted decimal representation.
-
IPv6 addressing scheme
- Each IP address has 16 bytes (128 bit binary number), and its complete format is represented by 8 segments of hexadecimal, and the segments are separated by colons.
Subnet mask
A subnet mask is used to mask part of an IP address to distinguish between a network ID and a host ID. The subnet mask identifies all network bits (binary) with 1 and the host bit with 0.
- For example, if the subnet mask is set to 255.255.255.0, the network identification part of the IP address 192.168.1.X is 192.168.1; the host is marked as: X
The subnet mask can be used to determine whether two computers are in the same subnet
- The IP address and subnet mask are bitwise and calculated. If the result is the same, they are in the same subnet.
port
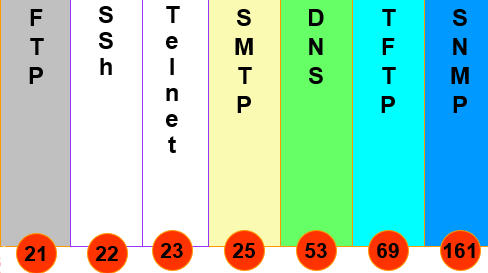
- Physical port. Such as the interface of ADSL Modem and hub.
- Port in logical sense, i.e. process identification
- Port numbers range from 0 to 65535
- Most port numbers within 1000 are used by standard protocols, so the free to use port numbers in applications generally use values greater than 1000. Such as 8888 port of pagoda panel.
The class of IP address translation in C ා
- Provide IPAddress class of IP address of internet protocol;
- IPEndPoint class containing IP address and port number;
- The IPHostEntry class that provides information containers for Internet or Intranet hosts.
Class IPAddress
- In System.Net namespace
- Provides IP address translation and processing functions
Parse method: converts an IP address string to an instance of IPAddress.
IPAddress ipa = IPAddress.Parse(ip);//Convert IP string to IPaddress type
However, if the string is not of IP address type, an exception will occur. So we need to do exception handling.
System.FormatException: 'an invalid IP address was specified
So in general, we use:
string ip = "127.0.0.1";//Define an IP string //IPAddress ipa = IPAddress.Parse(ip); / / convert IP string to IPaddress type try { IPAddress ipa = IPAddress.Parse(ip); } catch { Console.WriteLine("Please enter the correct IP Address!"); Console.ReadLine(); }
AddressFamily property
//AddressFamily IPAddress ip = IPAddress.Parse("::1"); IPAddress ip1 = IPAddress.Parse("127.0.0.1"); if (ip.AddressFamily == AddressFamily.InterNetworkV6) { Console.WriteLine("The IP Address adoption IPV6 Addressing"); Console.ReadLine(); }
The IPAddress class also provides seven read-only fields that represent the special IP addresses used in the program.
Common read-only fields
Name | Explain |
---|---|
Any | Provides an IPv4 address indicating that the server should listen for client activity on all network interfaces, which is equivalent to 0.0.0.0 |
Broadcast | Provides an IPv4 webcast address, which is equivalent to 255.255.255.255 |
IPv6Any | Provide all available IPv6 addresses |
IPv6Loopback | Represents the IPv6 loopback address of the system, equivalent to:: 1 |
Loopback | Represents the IPv4 loopback address of the system, equivalent to 127.0.0.1 |
IPEndPoint class
- Host and port information that applications need to connect to services on the host.
- Common constructors
IPAddress localAdress = IPAddress.Parse("127.7.0.1"); IPEndPoint iep = new IPEndPoint(localAdress, 21);//Initializing the IPEndPoint class string ipaddress = "IP Address is"+iep.Address;//Here, we use concatenated string to convert IPAddress to string type string port = "The port number is" + iep.Port; Console.WriteLine($"{ipaddress}\n{port}"); Console.ReadLine();
IPHostEntry class
Associate the host name of a domain name system (DNS) with a set of aliases and a set of matching IP addresses. This class is generally used with the DNS class.
Domain name resolution
Why is domain name system introduced?
- Network host uses IP address to locate
- IP is hard to remember, so you need to use the name to represent the host
- After the IP address changes, the domain name remains unchanged and can still be accessed
The. NET framework provides Dns classes in the System.Net namespace to complete the parsing
Method name | Explain |
---|---|
GetHostAddresses | Returns the Internet Protocol IP address of the specified host and the asynchronous method |
GetHostEntry | Resolve the host name or IP address to the IPHostEntry instance. This method also corresponds to the asynchronous method |
GetHostName | Get the host name of the local computer |
GetHostAddresses method
Specifies the IP address of the host and returns an array of IPAddress types.
-
public static IPAddress[] GetHostAddresses(string hostNameOrAddress);
- The hostNameOrAddress in the parameter indicates the hostname or IP address to resolve.
-
If hostNameOrAddress is an IP address, this address is returned directly; if it is an empty string, all IPv4 and IPv6 addresses of the machine are returned.
IPAddress[] ips = Dns.GetHostAddresses("baidu.com"); //Define an array to hold the IPAddress class parsed from the host IPAddress[] ips1 = Dns.GetHostAddresses("www.cctv.com"); IPAddress[] ips2 = Dns.GetHostAddresses("127.0.0.1");//If hostNameOrAddress is an IP address, it will be returned directly; IPAddress[] ips3 = Dns.GetHostAddresses("");//If it is an empty string, all IPv4 and IPv6 addresses of the local machine are returned. foreach (var i in ips3) { Console.WriteLine(i); } Console.ReadLine()
GetHostEntry method
An IP hostentry instance is returned based on the host name or IP address, which is used to query the list of IP addresses associated with a host name or IP address in the DNS server.
- public static IPHostEntry GetHostEntry (string hostNameOrAddress)
- hostNameOrAddress indicates the host name or IP address to be resolved
- When the parameter is an empty string, the IPHostEntry instance of the local host is returned.
IPHostEntry iPHostEntry = Dns.GetHostEntry(""); var iplist = iPHostEntry.AddressList;//Get IP address list var hostname = iPHostEntry.HostName;//Get host name foreach(var i in iplist) { Console.WriteLine(i); } Console.WriteLine($"The host name is{hostname}"); Console.ReadLine();
GetHostName method
This method is used to get the native hostname.
string hostname = Dns.GetHostName(); Console.WriteLine($"The host name of this machine is:{hostname}"); Console.ReadLine();