C programming (Fifth Edition) Tan Haoqiang -- Chapter four exercises after class
4. There are three integers a, B and C, which are input by keyboard and output the largest number.
#include<stdio.h>
#include<math.h>
#include<stdlib.h>
int main(){
int a,b,c;
printf("Please enter three integers:");
scanf("%d,%d,%d",&a,&b,&c);
printf("The maximum number is:");
if(a>b)
{
if(a>c)
printf("%d\n",a);
else
printf("%d\n",c);
}
else
{
if(b>c)
printf("%d\n",b);
else
printf("%d\n",c);
}
system("pause");
return 0;
}

5. Input a positive number less than 1000 from the keyboard, and ask to output its square root (if the square root is not an integer, then output its integer part). It is required to check whether the input data is a positive number less than 1000. If not, re-entry is required.
#include<stdio.h>
#include<math.h>
#include<stdlib.h>
int main(){
int i,j;
printf("Please enter an integer:");
scanf("%d",&i);
if(i>1000)
{
printf("Data does not meet the requirements, please re-enter:");
scanf("%d",&i);
}
j = sqrt((double)i);
printf("The integral part of the square root of the number is:%d\n",j);
system("pause");
return 0;
}
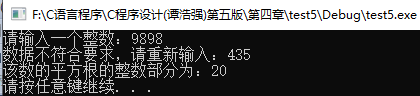
6. There is a function: y = x (x < 1); y = 2x-1 (1 ≤ x < 10); y = 3x-11 (x ≥ 10). Write a program, input the value of X, and output the corresponding value of Y.
#include<stdio.h>
#include<math.h>
#include<stdlib.h>
int main(){
int x,y;
printf("Please input x Value:");
scanf("%d",&x);
if(x<1)
y = x;
else if(x>=1 && x<10)
y = 2 * x - 1;
else
y = 3 * x - 11;
printf("Corresponding y The value is:%d\n",y);
system("pause");
return 0;
}

8. Give the score of the 100 point system, and output the grades' A ',' B ',' C ',' D ',' E '. More than 90 points are 'A', 80-89 points are 'B', 70-79 points are 'C', 60-69 points are 'D', and less than 60 points are 'E'.
#include<stdio.h>
#include<math.h>
#include<stdlib.h>
int main(){
double x;
char grade;
printf("Please enter the student's score:");
scanf("%lf",&x);
if(x>100 || x<0)
{
printf("Input error, please re-enter:");
scanf("%lf",&x);
}
switch((int)(x/10))
{
case 10:
case 9: grade = 'A'; break;
case 8: grade = 'B'; break;
case 7: grade = 'C'; break;
case 6: grade = 'D'; break;
default: grade = 'E';break;
}
printf("The student's grade is:%c\n",grade);
system("pause");
return 0;
}
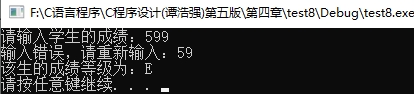
9. For a positive integer with no more than 5 digits, the requirements are: ① find out how many digits it is; ② output each digit separately; ③ output each digit in reverse order, for example, if the original number is 321, 123 should be output
#include<stdio.h>
#include<math.h>
#include<stdlib.h>
int main(){
int x;
int n,n1,n2,n3,n4,n5;
printf("Please enter a positive integer no greater than 99999:");
scanf("%d",&x);
if(x>9999)
n = 5;
else if(x>999)
n = 4;
else if(x>99)
n = 3;
else if(x>9)
n = 2;
else
n = 1;
printf("The number of digits is:%d\n",n);
n5 = x / 10000;
n4 = ( x / 1000 ) % 10;
n3 = ( ( x / 100) % 100 ) %10;
n2 = ( ( ( x / 10 ) % 1000 ) % 100 ) % 10;
n1 = ( ( ( x % 10000) % 1000 ) % 100 ) % 10;
printf("Each digit is:%d,%d,%d,%d,%d\n",n5,n4,n3,n2,n1);
switch(n)
{
case 5: printf("The reverse order of the number is%d%d%d%d%d\n",n1,n2,n3,n4,n5); break;
case 4: printf("The reverse order of the number is%d%d%d%d\n",n1,n2,n3,n4); break;
case 3: printf("The reverse order of the number is%d%d%d\n",n1,n2,n3); break;
case 2: printf("The reverse order of the number is%d%d\n",n1,n2); break;
case 1: printf("The reverse order of the number is%d\n",n1); break;
}
system("pause");
return 0;
}
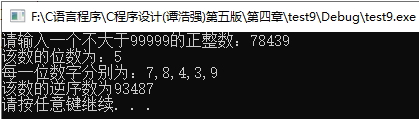
10. The bonus paid by the enterprise shall be based on the profit commission. If the profit I is less than or equal to 100000 yuan, the bonus can be increased by 10%; if the profit is higher than 100000 yuan, and lower than 200000 yuan (100000 < I ≤ 200000), the part lower than 100000 yuan will be increased by 10%, and the part higher than 100000 yuan will be increased by 7.5%; if 200000 < I ≤ 400000, the part lower than 200000 yuan will still be increased by the above method (the same below). 5% for the part higher than 200000 yuan; 3% for the part higher than 400000 when 400000 < I ≤ 600000 yuan; 1.5% for the part higher than 600000 when 600000 < I ≤ 1000000 yuan; 1% for the part higher than 1000000 yuan when I > 1000000 yuan. Input the profit I of the current month from the keyboard to calculate the total amount of bonus payable.
Requirements: (1) program with if statement. (2) Program with switch statement.
#include<stdio.h>
#include<math.h>
#include<stdlib.h>
int main(){
double x,y;
printf("Please enter the profit of the month:");
scanf("%lf",&x);
if(x <= 100000)
y = x * 0.1 ;
else if(x > 100000 && x <= 200000)
y = 100000 * 0.1 + ( x - 100000 ) * 0.075;
else if(x > 200000 && x <= 400000)
y = 100000 * 0.1 + 100000 * 0.075 + ( x - 200000 ) * 0.05;
else if(x > 400000 && x <= 600000)
y = 100000 * 0.1 + 100000 * 0.075 + 200000 * 0.05 + ( x - 400000 ) * 0.03;
else if(x > 600000 && x <= 1000000)
y = 100000 * 0.1 + 100000 * 0.075 + 200000 * 0.05 + 200000 * 0.03 + ( x - 600000 ) * 0.015;
else
y = 100000 * 0.1 + 100000 * 0.075 + 200000 * 0.05 + 200000 * 0.03 + 400000 * 0.015 + ( x - 1000000 ) * 0.01;
printf("The total amount of bonus payable is:%lf\n",y);
switch((int)(x/100000))
{
case 0: y = x * 0.1; break;
case 1: y = 100000 * 0.1 + ( x - 100000 ) * 0.075; break;
case 2:
case 3: y = 100000 * 0.1 + 100000 * 0.075 + ( x - 200000 ) * 0.05; break;
case 4:
case 5: y = 100000 * 0.1 + 100000 * 0.075 + 200000 * 0.05 + ( x - 400000 ) * 0.03; break;
case 6:
case 7:
case 8:
case 9: y = 100000 * 0.1 + 100000 * 0.075 + 200000 * 0.05 + 200000 * 0.03 + ( x - 600000 ) * 0.015; break;
case 10: y = 100000 * 0.1 + 100000 * 0.075 + 200000 * 0.05 + 200000 * 0.03 + 400000 * 0.015 + ( x - 1000000 ) * 0.01; break;
}
printf("The total amount of bonus payable is:%lf\n",y);
system("pause");
return 0;
}
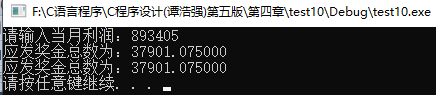
11. Input 4 integers and output them in order of small to large.
#include<stdio.h>
#include<math.h>
#include<stdlib.h>
int main(){
int a,b,c,d,temp;
printf("Please enter 4 integers:");
scanf("%d,%d,%d,%d",&a,&b,&c,&d);
if(a>b)
{
temp = a;
a = b;
b = temp;
}
if(a>c)
{
temp = a;
a = c;
c = temp;
}
if(a>d)
{
temp = a;
a = d;
d = temp;
}
if(b>c)
{
temp = b;
b = c;
c = temp;
}
if(b>d)
{
temp = b;
b = d;
d = temp;
}
if(c>d)
{
temp = c;
c = d;
d = temp;
}
printf("The order from small to large is:%d,%d,%d,%d\n",a,b,c,d);
system("pause");
return 0;
}

12. There are four circular towers with the centers of (2,2), (- 2, - 2), (- 2, - 2), (2, - 2) and the radius of 1. The height of the four towers is 10m, and there is no building outside the tower. Now enter any coordinate point to find the building height of the point (the height outside the tower is zero).
#include<stdio.h>
#include<math.h>
#include<stdlib.h>
int main(){
int h = 10;
double x1,x2,x3,x4,y1,y2,y3,y4,x,y,d1,d2,d3,d4;
x1 = x3 = 2; x2 = x4 = -2; y1 = y3 = 2; y2 = y4 = -2;
printf("Please enter coordinates:");
scanf("%lf,%lf",&x,&y);
d1 = sqrt ( ( x - x1 ) * ( x - x1 ) + ( y - y1 ) * ( y - y1 ) );
d2 = sqrt ( ( x - x2 ) * ( x - x2 ) + ( y - y2 ) * ( y - y2 ) );
d3 = sqrt ( ( x - x3 ) * ( x - x3 ) + ( y - y3 ) * ( y - y3 ) );
d4 = sqrt ( ( x - x4 ) * ( x - x4 ) + ( y - y4 ) * ( y - y4 ) );
if(d1>1 && d2>1 && d3>1 && d4>1)
h = 0;
printf("The height of the building at this point is:%d\n",h);
system("pause");
return 0;
}

