Before the text
Since the curriculum design, we have started production practice. Our teacher is looking for an internship in the technical department of Henan Weihua Group. After a period of maladjustment (for example, the basic tasteless food here in Henan Province, one of us now has a meal or an old godmother), I finally started a new learning journey.
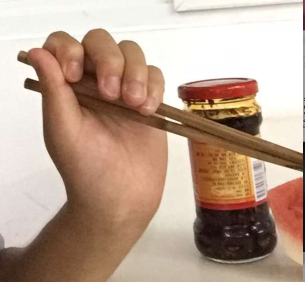
I am a confused programming enthusiast. I don't know where I am going. I also have a reward today for not wasting the machinery. That's what I want to do on the Internet, but I don't have that skill. Although all kinds of programming skills will be: Web front-end, Linux server operation and maintenance, Python crawler, algorithm analysis, Wechat applet, some other applications of Javascript, C language, database, PHP, but it is estimated that they are not up to the standard of work. No way, no one in my school asked me to do the project, and I have been a lonely walker. Basically, I study slowly by myself, so I am not good at it, or I can't get the gist. Really powerful people are one or two native languages, and then other basic touches can be developed in a few days. After all, the foundation is there, and the words that are close to the truth can be used in all kinds of ways. I envy that realm. So, this internship was boring and I found C++, a language that my senior valued very much. To be honest, this is the first language I have come into contact with. I have had classes in my freshman year, and then I have reviewed it in the second level of the computer exam (disgrace, no, later I learned C by myself). Following is a small project that I transformed after I finished my basic study, combined with the case analysis of the book with a lot of loopholes and the relatively correct C++ training content on the internet. If you don't know what to do after you finish the basic study of C++, you can have a look at it.
In the text
I'm just a programmer ape, not to mention it, but I'm going to go directly to the code on the picture (github upload is actually walled, hehe, what else can I say, it can all be walled):
https://github.com/HustWolfzzb/Bank_Management_System.git
By the way, it seems that the walls are so fierce these days.

The pictures are as follows:
- Code Framework:
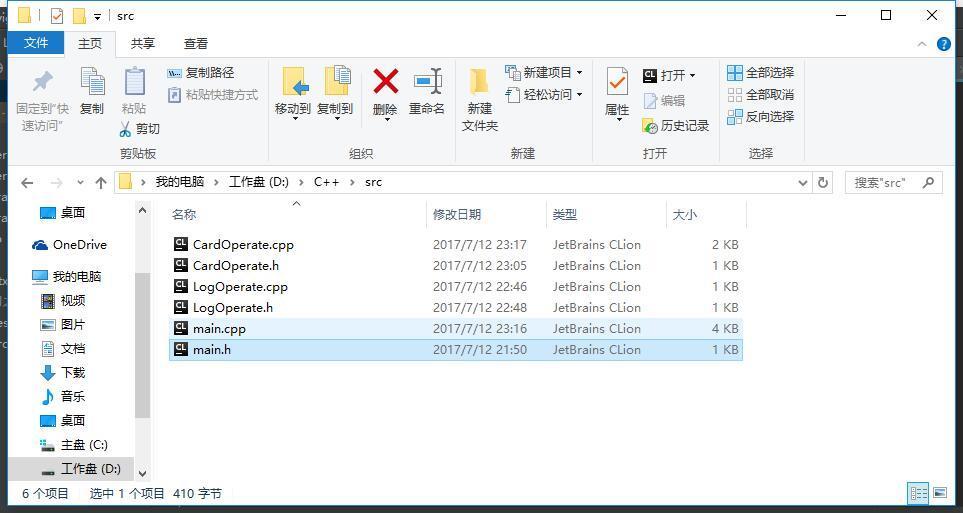
- Editing interface
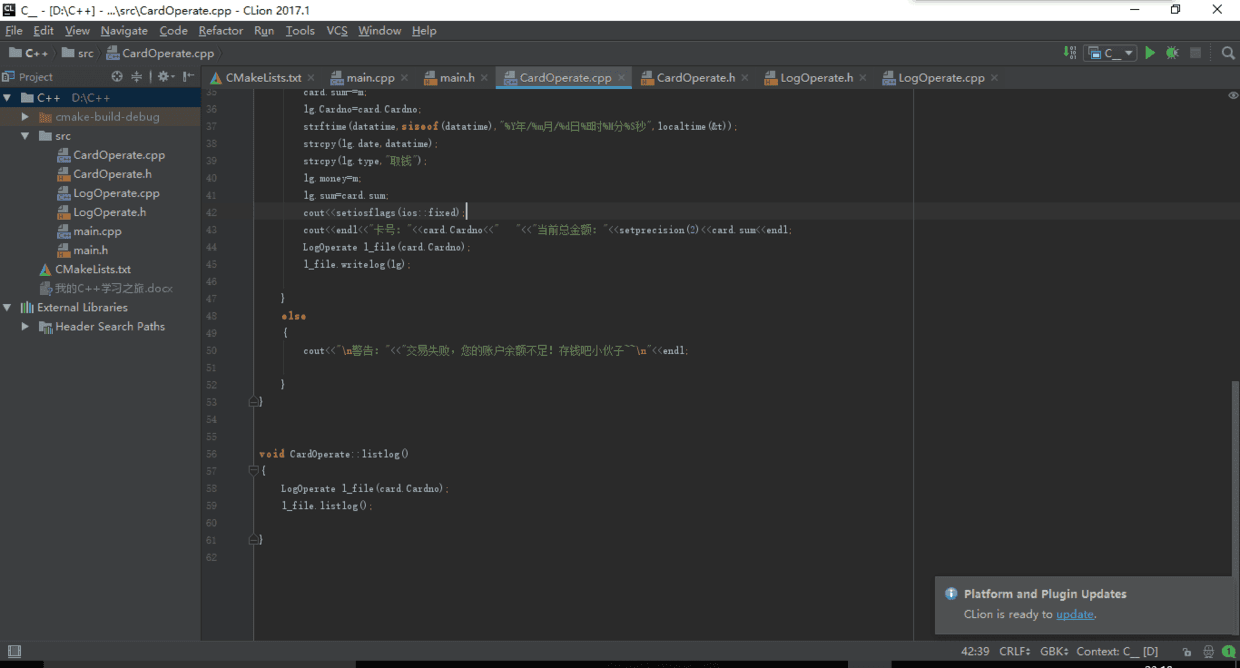
* Scenarios after bug cleaning
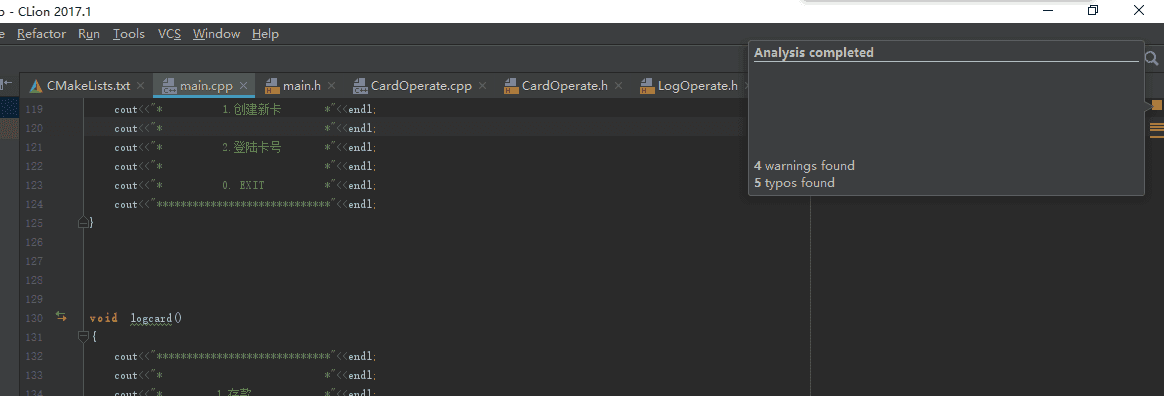
- Product - a mentally retarded bank card
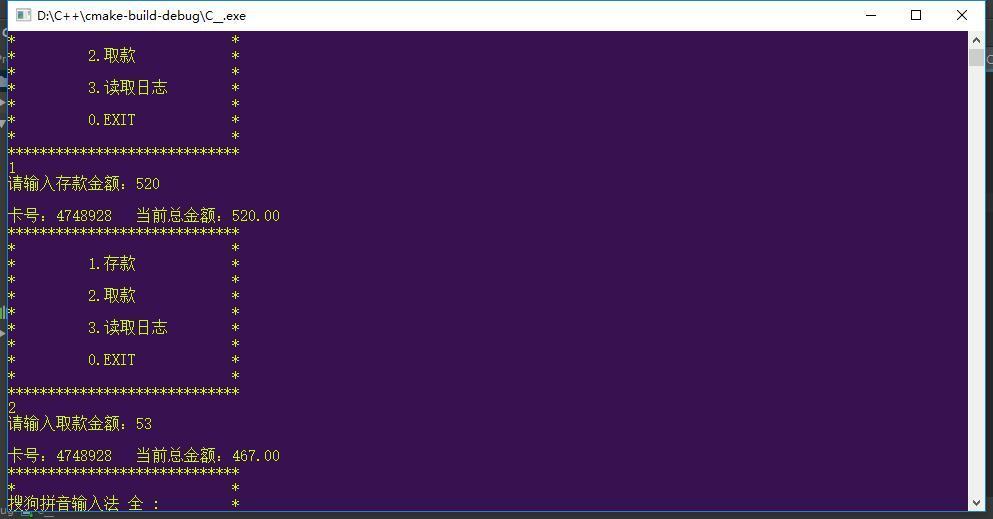
The code is as follows:
If you use the Make system, you have to keep it:
- CMakelists.txt
cmake_minimum_required(VERSION 3.7) project(C__) set(CMAKE_CXX_STANDARD 11) set(SOURCE_FILES src/main.cpp) file(GLOB SOURCES "src/**/*.cpp" "src/**/*.hpp" "src/**/*.h" "src/*.h" "src/*.cpp" "src/*.c" ) add_executable(C__ ${SOURCE_FILES} ${SOURCES}) set(CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS} -Wall -std=c++0x")
Here is the code:
- main.cpp
#include <iostream> #include <cstring> #include <fstream> #include<stdlib.h> #include<iomanip> #include<time.h> #include "CardOperate.h" #include "LogOperate.h" #define Length 100ยท using namespace std; void CreateNewCard(); void Logoperate(int cd); void MainInterFace(); void logcard(); int main() { char select; int CardID; do { MainInterFace(); cin>>select; switch(select) { case '1': { CreateNewCard(); }; case '2': { cout<<"\n Please enter the card number:"; cin>>CardID; Logoperate(CardID); } case '0': break; default:cout<<"Man? F**k U!\n"<<endl; } } while(select!='0'); return 0; } void CreateNewCard() { char filename[Length]; Card t_cd; int i_cd; char s_name[Length]; cout<<"\n Enter the card number:"; cin>>i_cd; cout<<"Please enter a user name:"; cin>>s_name; t_cd.Cardno=i_cd; strcpy(t_cd.username,s_name); t_cd.sum=0; itoa(t_cd.Cardno,filename,10); fstream writefile(strcat(filename,".txt"),ios::out); writefile.write((char *)&t_cd,sizeof(t_cd)); writefile.close(); } void Logoperate( int CardID) { char in; double money; CardOperate cdop(CardID); do { logcard(); cin>>in; switch (in) { case '1': { cout << "Please enter the amount of deposit:"; cin >> money; cdop.CardIn(money); break;} case '2': { cout << "Please enter the amount of withdrawal:"; cin >> money; cdop.CardOut(money); break; } case '3': { cdop.listlog(); break; } case '0': { break; } default:cout<<"FXXk you Man?\n"; } } while (in!='0'); } void MainInterFace() { cout<<"*****************************"<<endl; cout<<"* Your bank card steward *"<<endl; cout<<"* *"<<endl; cout<<"* 1.Create a new card *"<<endl; cout<<"* *"<<endl; cout<<"* 2.Landing Card No. *"<<endl; cout<<"* *"<<endl; cout<<"* 0. EXIT *"<<endl; cout<<"*****************************"<<endl; } void logcard() { cout<<"*****************************"<<endl; cout<<"* *"<<endl; cout<<"* 1.deposit *"<<endl; cout<<"* *"<<endl; cout<<"* 2.Withdraw money *"<<endl; cout<<"* *"<<endl; cout<<"* 3.Read logs *"<<endl; cout<<"* *"<<endl; cout<<"* 0.EXIT *"<<endl; cout<<"* *"<<endl; cout<<"*****************************"<<endl; }
- main.h
#define CDNO 6 #define LENGTH 20 #include<iostream> #include<fstream> #include<string.h> #include<stdlib.h> #include<iomanip> #include<time.h> struct Card //Information Architecture of Definition Card { int Cardno; char username[LENGTH]; double sum; }; struct Log //Define login log information { int Cardno; char date[64]; char type[6]; double money; double sum; };
- CardOperate.h
#include<iostream> #include<fstream> #include<string.h> #include<stdlib.h> #include<iomanip> #include<time.h> #define CDNO 6 #include"main.h" class CardOperate { public: CardOperate(int cardindex){};//Constructor ~CardOperate(){};//Destructive function void CardIn(double m);//Deposit Operation Module void CardOut(double m);//Withdrawal operation module void listlog();//Query the Deposit and Deposit Log private: Card card; Log lg; char filename[CDNO]; time_t t; char datatime[64]; };
- CardOperate.cpp
#include<iostream> #include<fstream> #include<string.h> #include<stdlib.h> #include<iomanip> #include<time.h> #define CDNO 6 #include "CardOperate.h" #include "Logoperate.h" using namespace std; void CardOperate::CardIn(double m) { t=time(0); card.sum+=m; lg.Cardno=card.Cardno; strftime(datatime,sizeof(datatime),"%Y year/%m month/%d day%H time%M branch%S second",localtime(&t)); strcpy(lg.date,datatime); strcpy(lg.type,"Save money"); lg.money=m; lg.sum=card.sum; cout<<setiosflags(ios::fixed); cout<<endl<<"Card number:"<<card.Cardno<<" "<<"Current total amount:"<<setprecision(2)<<card.sum<<endl; LogOperate l_file(card.Cardno); l_file.writelog(lg); } void CardOperate::CardOut(double m) { if (card.sum>=m) { t=time(0); card.sum-=m; lg.Cardno=card.Cardno; strftime(datatime,sizeof(datatime),"%Y year/%m month/%d day%H time%M branch%S second",localtime(&t)); strcpy(lg.date,datatime); strcpy(lg.type,"Withdraw money"); lg.money=m; lg.sum=card.sum; cout<<setiosflags(ios::fixed); cout<<endl<<"Card number:"<<card.Cardno<<" "<<"Current total amount:"<<setprecision(2)<<card.sum<<endl; LogOperate l_file(card.Cardno); l_file.writelog(lg); } else { cout<<"\n Warning:"<<"Deal failed, your account balance is insufficient! Save your money, boy~~\n"<<endl; } } void CardOperate::listlog() { LogOperate l_file(card.Cardno); l_file.listlog(); }
- LogOperate.h
#include<iostream> #include<fstream> #include<string.h> #include<stdlib.h> #include<iomanip> #include<time.h> #define CDNO 6 class LogOperate { char Cardlog[CDNO]; Log lf[100]; int top; int Cardno; public: LogOperate(int n) { Cardno=n; top=-1; }; ~LogOperate() { }; void readlog(); void writelog(Log lg); void listlog(); };
- LogOperate.cpp
#include<iostream> #include<fstream> #include<string.h> #include<stdlib.h> #include<iomanip> #include<time.h> #define CDNO 6 #include "CardOperate.h" #include "Logoperate.h" using namespace std; void LogOperate::readlog() { Log l; itoa(Cardno,Cardlog,10); fstream file(strcat(Cardlog,"Log"),ios::in); while(1) { file.read((char *)&l, sizeof(l)); if(!file) break; top++; lf[top]=l; } file.close(); } void LogOperate::writelog(Log lg) { readlog(); top++; lf[top]=lg; fstream file(Cardlog,ios::out); for (int i = 0; i <=top ; i++) { file.write((char *)&lf[i], sizeof(lf[i])); } file.close(); } void LogOperate::listlog() { readlog(); for (int i = 0; i <= top; i++) { cout << "Card number:" << lf[i].Cardno << "Date:" << lf[i].date << "Method:" << lf[i].type << "Input amount:" << lf[i].money << "Total amount:" << lf[i].sum << endl; } }
[Ps: The bug just fixed, the code fresh out of the box]
- CardOperate.h 2.0
#include<iostream> #include<fstream> #include<string.h> #include<stdlib.h> #include<iomanip> #include<time.h> #define CDNO 6 #include"main.h" class CardOperate { public: CardOperate(int cardindex){ { t = time(0); itoa(cardindex, filename, 10); std::fstream file(strcat(filename, ".txt"), std::ios::in);//Link the two char types while (file) { file.read((char*)&card, sizeof(card)); } file.close(); } };//Constructor ~CardOperate(){ std::fstream file(filename, std::ios::out); file.write((char *)&card, sizeof(card)); file.close(); };//Destructive function void CardIn(double m);//Deposit Operation Module void CardOut(double m);//Withdrawal operation module void listlog();//Query the Deposit and Deposit Log private: Card card; Log lg; char filename[CDNO]; time_t t; char datatime[64]; };
After the text
ok, actually, it's been a long time since it was written, so I couldn't help but write and withdraw. In practice, I also want to write a good article carefully typesetting? Over my dead body!
In addition, I have been thinking about whether to take the postgraduate entrance examination or go to work recently. Hua Ke's machinery to go out to work is also OK, but these days in the office to see them draw pictures, and then bring themselves in, found that I can not accept the future has been living like this, I would rather go to write code to the end of the world, the university head teacher gave me the answer is:

I have to think about it carefully. Do you have a good idea? What's the market's view on programmed apes of non-academic background? Will the company refuse to recruit because students'skills are not up to standard for the time being?