linux Interprocess Communication Series 3, using socketpair, pipe
1. Using socketpair to realize interprocess communication is bidirectional.
2. Using pipe to realize interprocess communication
The key point of using pipe: fd[0] can only be used for receiving, fd[1] can only be used for sending, it is one-way.
3. Use pipe and write in with standard input.
Question: If you don't write wait function in code 2, the parent process can't end, but if you don't write wait function in code 3, the parent process can end???
1. Use socketpair:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <wait.h>
int main(){
int sv[2];
pid_t pid;
char buf[128];
memset(buf, 0, sizeof(buf));
if(socketpair(AF_UNIX, SOCK_STREAM, 0, sv) != 0){
perror("socketpair");
return 1;
}
pid = fork();
if(pid < 0){
perror("fork");
return 1;
}
if(pid == 0){
close(sv[0]);
read(sv[1], buf, sizeof(buf));
printf("child process : data from parant process [%s]\n", buf);
exit(0);
}
else {
int status;
close(sv[1]);
write(sv[0], "HELLO", 5);
printf("parent process : child process id %d\n", pid);
wait(&status);
}
return 0;
}
github source code
2. Use pipe:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <wait.h>
int main(){
int p[2];
pid_t pid;
char buf[128];
memset(buf, 0, sizeof(buf));
if(pipe(p) != 0){
perror("pipe");
return 1;
}
pid = fork();
if(pid < 0){
perror("fork");
return 1;
}
if(pid == 0){
close(p[1]);
read(p[0], buf, sizeof(buf));
printf("child process : data form parent process [%s]\n", buf);
exit(0);
}
else{
close(p[0]);
write(p[1], "aaaa", 4);
printf("parent process : child process is %d\n", pid);
int status;
wait(&status);
}
return 0;
}
github source code
3. Use pipe and write in with standard input.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <wait.h>
int main(){
int p[2];
pid_t pid;
char buf[1024];
memset(buf, 0, sizeof(buf));
if(pipe(p) != 0){
perror("pipe");
return 1;
}
pid = fork();
if(pid < 0){
perror("fork");
return 1;
}
if(pid == 0){
printf("child process : my_id=%d\n",getpid());
close(p[0]);
//Output the standard to Pipeline 1
dup2(p[1], fileno(stdout));
char *argv[ ]={"ls", "/home/ys/cpp/network"};
//Using the ls command, enter the name of the file in the folder into the standard output, which is connected to the pipe 1 above.
if(execve("/bin/ls", argv, NULL) < 0){
perror("exec");
return 1;
}
exit(0);
}else{
int n;
FILE* filep;
close(p[1]);
printf("parent process : child process id=%d\n", pid);
//Open Pipeline 1 first
filep = fdopen(p[0], "r");
if(filep == NULL){
perror("fdopen");
return 1;
}
//Read the data from Pipeline 1
while(fgets(buf, sizeof(buf), filep) != NULL){
printf("get:%s\n", buf);
}
int status;
wait(&status);
}
return 0;
}
github source code
c/c++ Learning Mutual Assistance QQ Group: 877684253
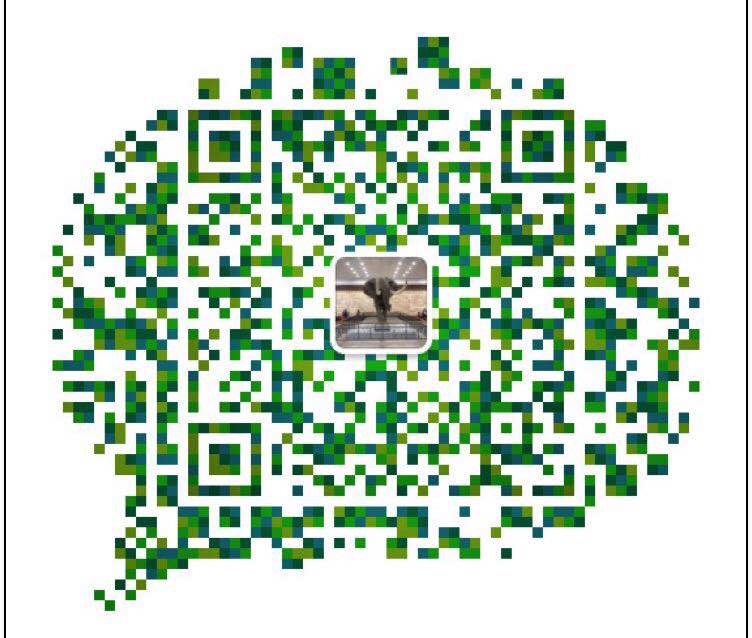
I am writing to xiaoshitou 5854