Copyright notice: This is the original article of the blogger. It can't be reproduced without the permission of the blogger. https://blog.csdn.net/u010046908/article/details/50750542
Builder Design in Java
Definition of 1Builder pattern
Separate the construction of a complex object from its representation, so that the same construction process of can build different representations.
Usage scenario of 2Builder mode
(1) the same method and different execution sequence produce different event results.
(2) multiple components can be assembled into one object, but the results are different.
(3) the product class is very complex, or the calling sequence is different in the product class, so it is very suitable to use the builder mode at this time.
(4) when initializing a complex object, such as many parameters, many parameters have default values.
UML diagram of 3Builder pattern
Product category - Product abstraction
Builder -- Abstract builder, which is used to standardize the components of products. Generally, the specific component process is implemented by subclasses.
ConcreateBuilder -- specific Builder class
Director -- unified component process.
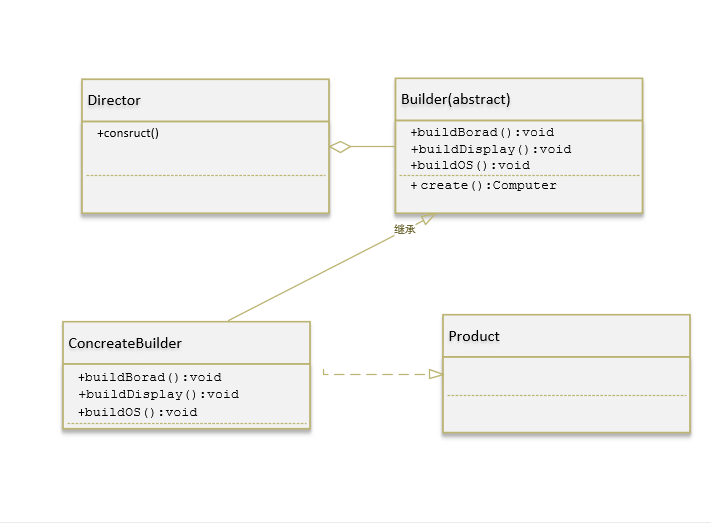
Code implementation
package builder; /** * Abstract deBuilder * @author lidong * */ public abstract class Builder { /** * Setting up the host computer * @param board */ public abstract void buildBorad(String board); /** * Setting up the display * @param display */ public abstract void buildDisplay(String display); /** * Set up operating system */ public abstract void buildOS(); /** * Create machine * @return */ public abstract Computer create(); }
package builder; /** * Computer abstract class * Product role * @author Administrator * */ public abstract class Computer { protected String mBoard;//A main board protected String mDisplay;//Monitor protected String mOS;//system protected Computer() { } public String getmBoard() { return mBoard; } public void setmBoard(String mBoard) { this.mBoard = mBoard; } public String getmDisplay() { return mDisplay; } public void setmDisplay(String mDisplay) { this.mDisplay = mDisplay; } //Abstract method public abstract void setOS(); @Override public String toString() { return "Computer [mBoard=" + mBoard + ", mDisplay=" + mDisplay + ", mOS=" + mOS + "]"; } }
package builder; /** * Specific builder class, Window computer * @author lidong * */ public class WindowBuilder extends Builder { private Computer mComputer = new WindowPC(); @Override public void buildBorad(String board) { mComputer.setmBoard(board); } @Override public void buildDisplay(String display) { mComputer.setmDisplay(display); } @Override public void buildOS() { mComputer.setOS(); } @Override public Computer create() { return mComputer; } }
package builder; /** * window Computer * @author lidong * */ public class WindowPC extends Computer{ protected WindowPC() { super(); } @Override public void setOS() { mOS = "window 7"; } }
package builder; /** * Director Responsible for building Compurter * @author lidong * */ public class Dirctor { Builder mBuilder = null; public Dirctor(Builder mBuilder) { super(); this.mBuilder = mBuilder; } /** * Building objects * @param borad * @param display */ public void construct(String borad,String display) { mBuilder.buildBorad(borad); mBuilder.buildDisplay(display); mBuilder.buildOS(); } }
Test class:
package builder; public class Test { public static void main(String[] args) { //constructor Builder builder1 = new WindowBuilder(); Dirctor pcDirctor1 = new Dirctor(builder1); pcDirctor1.construct("Intel 2", "1G Graphics card"); System.out.println("Computer info ="+builder1.create().toString()); } }
Code download address:
https://github.com/lidong1665/JAVA_DESIGN_PATTERNS/tree/master/JAVA_DESIGN_PATTERNS