Preface
Silicon Valley - Java Course - Notes (for self review)
Eventually I finished looking at Object Oriented🤣,To sum up, the basics are over.
Classes and Objects
1. Three main lines of object-oriented learning
-
Java classes and their members: properties, methods, constructors; code blocks, internal classes
-
Three object-oriented features: encapsulation, inheritance, polymorphism, (abstraction)
-
Other keywords: this, super, static, final, abstract, interface, package, import, etc.
"Start small, look big"
2. Object-Oriented and Process-Oriented (Understanding)
- Process-oriented: Emphasizes functional behavior and considers what to do with functions as the smallest unit.
- Object-oriented: Emphasizes functional objects, with classes/objects as the smallest unit, considering who does it.
Example comparison: People put elephants in the refrigerator.
3. Ideas for completing a project (or function)
4. Two important concepts in object-oriented
- Class: An abstract, conceptual definition of a class of things.
- Object: is each individual of this kind of thing that actually exists and is therefore called an instance.
-Object-oriented programming focuses on class design
-The design class is a member of the design class.
The relationship between them:
Object, derived from class new.
5. Rules for the implementation of object-oriented ideas
- Create classes, design class members
- Create object of class
- Calling the structure of an object through Object-Property or Object-Method
Supplement: Instructions for the use of several concepts
- Attribute=Member Variable=field=Domain,field
- method=Member method=Function=method
- Object Creating Class=Instantiation of Class=Instantiation of Class
6. Creation of Objects and Memory Resolution of Objects
Typical code:
Person p1 = new Person(); Person p2 = new Person(); Person p3 = p1;//No new object was created, sharing an object entity in a heap space.
Explain:
If more than one object of a class is created, each object has its own set of attributes of the class. (not static)
Meaning: if we modify the property a of one object, it will not affect the value of another object property a.
Memory Resolution:
7. Anonymous Objects
The object we created was not explicitly assigned a variable name. It is an anonymous object
Feature: Anonymous objects can only be called once.
Give an example:
new Phone().sendEmail(); new Phone().playGame(); new Phone().price = 1999; new Phone().showPrice();//0.0 Scenarios: PhoneMall mall = new PhoneMall(); //Use of anonymous objects mall.show(new Phone()); Where, class PhoneMall{ public void show(Phone phone){ phone.sendEmail(); phone.playGame(); } }
8. Understand "Everything is an object"
- In the Java language category, we all encapsulate functions, structures, etc. into classes, and invoke specific functional structures by instantiating classes
- Scanner,String, etc.
- File:File
- Network resource: URL
- When the Java language interacts with the front-end Html and the back-end database, the front-end and back-end structures are reflected as classes and objects when interacting at the Java level.
JVM Memory Structure
After compiling the source program, one or more byte code files are generated.
We use the loader and interpreter of classes in the JVM to interpret and run the generated byte code file. This means that the class corresponding to the byte code file needs to be loaded into memory, involving memory parsing.
JVM Specification
The virtual machine stack is the usual stack structure. We store local variables in the stack structure
Heap, we load the new structure (such as arrays, objects) into the heap space.
Supplement: Object properties (not static) are loaded in heap space.
Method area: load information for classes, constant pools, static fields
2. One of the structures of a class: attributes
One of the two important structures in class design is attributes
Contrast: Attribute vs Local Variable
1. Same point: all variables
- Define the format of the variable: data type variable name = variable value;
- Declare before use
- Variables all have their corresponding scopes
2. Differences:
2.1 Different positions declared in classes
Properties: directly defined within a pair of {} classes
Local variables: variables declared within methods, method parameters, code blocks, constructor parameters, and constructors
2.2 Differences about permission modifiers
Attribute: When declaring an attribute, you can specify its permissions, using the permission modifier.
Common permission modifiers: private, public, default, protected ->encapsulation
Currently, when declaring attributes, you can use the default.
Local variable: Permission modifiers cannot be used.
2.3 Default initialization value:
Attribute: The property of a class, according to its type, is initialized by default.
- Integer (byte, short, int, long:0)
- Floating Point (float, double:0.0)
- Character type (char:0 (or'\u0000'))
- Boolean (boolean:false)
Reference data type (class, array, interface: null)
Local variable: There is no default initialization value. This means that we must explicitly assign a value before calling a local variable.
Specifically: when a parameter is called, we assign a value.
2.4 Location to load in memory:
Attribute: Load into heap space (non-static)
Local variable: loaded into stack space
Supplement: Review the classification of variables:
Mode 1: According to data type:
Mode 2: According to the position declared in the class:
3. Structure of Classes 2: Method
Two important structures in class design are methods
Method: Describe what the class should do.
For example:
- Math class: sqrt()\random()...
- Scanner class: nextXxx()...
- Arrays class: sort() \ binarySearch() \ toString() \ equals() \ ...
- Give an example:
public void eat(){} //No formal parameters, return public void sleep(int hour){} //Tangible parameters public String getName(){} //Has returned public String getNation(String nation){}
- Method declaration:
Permission modifier returns value type method name (parameter list){
Method Body
}
Note: static, final, abstract are used to modify the method, which will be discussed later.
3. Description
3.1 About permission modifiers
Privilege modifiers for default methods all use public first
Four permission modifiers specified in Java: private, public, default, protected --> Encapsulation and more
3.2 Return Value Type: Return Value vs No Return Value
- If a method has a return value, the type of return value must be specified when the method is declared.
At the same time, the method requires the return keyword to return a variable or constant of the specified type: "return data". - If the method does not return a value, void is used when the method is declared.
Normally, in a method that does not return a value, you do not need to use return. However, if you do, you can only "return;" to indicate the end of the method.
3.2.2 Should we define a method that should not return a value?
1. Title requirements
Second, based on experience: specific analysis of specific issues
3.3 Method Name: Identifier, follow the rules and specifications of identifiers, "Know what you see"
3.4 Parameter List: Method can declare 0, 1, or more parameters.
3.4.1 Format: Data type 1 parameter 1, Data type 2 parameter 2,...
3.4.2 Should we define parameters when we define methods?
1. Title requirements
Second, based on experience: specific analysis of specific issues
3.5 Method Body: The reflection of method function.
3. When using a method, you can call the properties or methods of the current class
Special: Method A: Recursive method is called in method A.
In a method, you cannot define a method.
return keyword
1. Scope of use: Use in method body
2. Role:
(1) End method
(2) For methods of return value type, use the "return data" method to return the desired data.
3. Note: Execution statements cannot be declared after the return keyword.
4. Return Value Type: Return Value vs No Return Value
- If a method has a return value, the type of return value must be specified when the method is declared.
At the same time, the method requires the return keyword to return a variable or constant of the specified type: "return data". - If the method does not return a value, void is used when the method is declared.
Normally, in a method that does not return a value, you do not need to use return. However, if you do, you can only "return;" to indicate the end of the method.
overload
1. Concepts
Definition: More than one method with the same name is allowed in the same class as long as they have different number or type of parameters.
Summary: "Two identical but different"
- Same class, same method name
- Different parameter lists: different number of parameters, different parameter types
2. Examples
Examples of overloads:
Example 1: Arrays Overloaded in class sort() / binarySearch();PrintStream In println() Example 2: //The following four methods make up the overload public void getSum(int i,int j){ System.out.println("1"); } public void getSum(double d1,double d2){ System.out.println("2"); } public void getSum(String s ,int i){ System.out.println("3"); } public void getSum(int i,String s){ System.out.println("4"); }
Examples that do not constitute an overload:
//The following three methods do not overload the four above methods public int getSum(int i,int j){ return 0; } public void getSum(int m,int n){ } private void getSum(int i,int j){ }
3. How can I determine if it constitutes a method overload?
- Judged strictly by definition: two identical are different.
- It doesn't matter with the permission modifier, return value type, parameter variable name, method body of the method!
4. How to determine the call to a method in a class:
Method Name - > Parameter List
Interview Question: The difference between overloading and rewriting methods?
throws\throw
String\StringBuffer\StringBuilder
Collection\Collections
final\finally\finalize ...
What is the difference between an abstract class and an interface?
What is the difference between sleep ()/ wait ()?
Methods for Variable Number of Parameters
1. Instructions for use:
- Additions to jdk 5.0
- Specific use:
- Format of variable number of parameters: data type...variable name
- When calling a method with variable number of parameters, the number of parameters passed in can be: 0, 1, 2,...
- Methods with variable number of parameters have the same method name as those in this class, and overloads occur between methods with different parameters
- Methods with variable number of parameters have the same method name as those in this class, and arrays with the same parameter type do not constitute overloads. In other words, they cannot coexist.
- Variable number of parameters In method parameters, must be declared at the end
- Variable number of parameters Among the parameters of a method, at most one variable parameter can be declared.
2. Examples:
public void show(int i){ } public void show(String s){ System.out.println("show(String)"); } public void show(String ... strs){ System.out.println("show(String ... strs)"); for(int i = 0;i < strs.length;i++){ System.out.println(strs[i]); } } //Cannot coexist with the previous method // public void show(String[] strs){ // // } On call: test.show("hello"); test.show("hello","world"); test.show(); test.show(new String[]{"AA","BB","CC"});
Value transfer mechanism in java
1. Examples of assignments to variables within a method:
System.out.println("***********Basic data types:****************"); int m = 10; int n = m; System.out.println("m = " + m + ", n = " + n); n = 20; System.out.println("m = " + m + ", n = " + n); System.out.println("***********Reference data type:****************"); Order o1 = new Order(); o1.orderId = 1001; Order o2 = o1;//After assignment, the address values of o1 and o2 are the same, pointing to the same object entity in heap space. System.out.println("o1.orderId = " + o1.orderId + ",o2.orderId = " +o2.orderId); o2.orderId = 1002; System.out.println("o1.orderId = " + o1.orderId + ",o2.orderId = " +o2.orderId);
Rules:
If the variable is a basic data type, the value assigned is the data value that the variable holds.
If the variable is a reference data type, then the value assigned is the address value of the data the variable holds.
2. Parameter concepts for methods
Parameters: Parameters in parentheses declared when a method is defined
Arguments: The data actually passed to the parameter when the method is called
3. Parameter Passing Mechanism in java: Value Passing Mechanism
Rules:
If the parameter is a basic data type, then the argument is assigned to the parameter the data value it actually stores.
If the parameter is a reference data type, then the argument is assigned to the parameter, which stores the address value of the data.
Extension:
If the variable is = the base data type, then the value assigned is the data value that the variable holds.
If the variable is a reference data type, then the value assigned is the address value of the data the variable holds.
4. Typical examples and memory parsing:
[Example 1] The parameter type is: Basic data type
[Example 2] The parameter type is: Reference data type
Recursive Method
1. Definition
Call itself within a method body.
2. How do you understand recursion?
- Method recursion contains an implicit loop that repeats a piece of code without loop control.
- Recursion must recurse in a known direction, otherwise it becomes infinite, similar to a dead cycle.
3. Examples:
// Example 1: Calculate the sum of natural numbers between 1-n public int getSum(int n) {// 3 if (n == 1) { return 1; } else { return n + getSum(n - 1); } }
// Example 2: Calculate the product of natural numbers between 1-n: n! public int getSum1(int n) { if (n == 1) { return 1; } else { return n * getSum1(n - 1); } }
//Example 3: A known sequence is f(0) = 1,f(1) = 4,f(n+2)=2*f(n+1) + f(n), //Where n is an integer greater than 0, find the value of f(10). public int f(int n){ if(n == 0){ return 1; }else if(n == 1){ return 4; }else{ // return f(n + 2) - 2 * f(n + 1); return 2*f(n - 1) + f(n - 2); } }
//Example 4: Fibonacci sequence law: From the third number, each number equals the sum of its first two numbers. //fibonacci(n) = fibonacci(n-1) + fibonacci(n-2) public int fibonacci(int n){ if (n == 1 || n == 2) { return 1; }else if (n > 2) { return fibonacci(n - 1) + fibonacci(n - 2); //Recursive call } return -1; //If n is entered incorrectly, return -1 }
//Example 5: Hannotta problem package com.atguigu.exer1; import java.util.Scanner; public class Hanoi2 { public static void main(String[] args) { //Principal function Scanner in = new Scanner(System.in); //Define Root Column char A = 'A'; char B = 'B'; char C = 'C'; System.out.println("**************************************************************"); System.out.println("Hannotta problem A Tower number from small to large discs from A Tower Pass B Auxiliary towers moved to C Up the Tower"); System.out.println("**************************************************************"); System.out.print("Please enter the number of disks:"); //Total number of initialization disks int n = in.nextInt(); Hanoi2.hanoi(n, A, B, C); //Hannotta executes the function (move details inside the function) System.out.println(">>Moved" + count + "Secondly, put A The discs on the move to C upper"); System.out.println("End"); in.close(); } static int count = 0;// Number of Marker Moves // Functions Implementing Move**************************** public static void move(int num, char start, char end) { //Number plates from small to large, num each time, moving from the start post to the end post System.out.println("No." + (++count) + " Secondary movement : " + " hold " + num + " Disc number from " + start + " Move columns to " + end +"On the number post"); }
// Functions that recursively implement Hannota************* public static void hanoi(int n, char A, char B, char C) { //n is the total number of plates //Default A column is the starting column //Default B post is auxiliary post //Default C post is end post /* Ideas: Move n-1 plate above from A to B (no need to think about moving details), and then move the last plate (bottom largest) to end post C first Finally, just move n-1 plates of column B to C with the help of column A. */ if (n == 1)// When there is only one disk, just move it from Tower A to Tower C Hanoi2.move(1, A, C); else{// otherwise hanoi(n - 1, A, C, B);// Recursively, n-1 plates move from A to B, with the help of C Hanoi2.move(n, A, C);// Move the last plate (the largest bottom plate) from A to C hanoi(n - 1, B, A, C);// Recursively, move n-1 plates from B to C, using A } } }
Example 6: Quick Row
4. Object-Oriented Features 1: Encapsulation
Object-oriented feature one: encapsulation and hiding
1. Why should encapsulation be introduced?
Programming pursues "high cohesion, low coupling".
High cohesion: the internal data manipulation details of a class are self-contained and external interference is not allowed;
Low coupling: Only a small number of methods are exposed for use.
Hide the complexity inside the object, and only expose simple interfaces to the outside world. Easy to call from outside, so as to improve the scalability and maintainability of the system.
Generally speaking, hide the hidden and expose the exposed. This is the design idea of encapsulation.
2. Problem introduction
When we create an object of a class, we can assign the attributes of the object in the way of Object.Attribute.
Here, the assignment operation is constrained by the data type and storage range of the attribute, but there are no other constraints.
However, in practice, we often need to add additional restrictions to attribute assignment.
This condition cannot be reflected in the property declaration, we can only add restrictions through the method.
(For example, setLegs() and we need to avoid users from assigning attributes in the way of Object.Attribute.
You need to declare the property private.
- At this point, encapsulation is reflected for attributes.
3. Code representation of encapsulation idea
Implications 1: Privateize the class property xxx while providing a public method to get (getXxx) and set (setXxx) the value of this property
private double radius; public void setRadius(double radius){ this.radius = radius; } public double getRadius(){ return radius; }
Reflections 2: Private methods of not exposing to the outside world
Reflect three: the singleton pattern (privatizing the constructor)
Reflection 4: If you do not want the class to be called outside the package, you can set the class as the default.
4. Four permission modifiers specified in Java
4.1 Permissions from smallest to largest are
Private < default < protected < public
4.2 Specific range of modifications: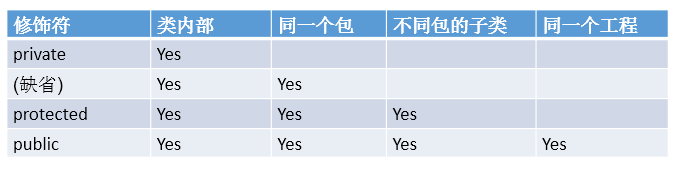
4.3 Permission modifiers can be used to modify the structure of the description:
Four permissions can be used to modify the internal structure of a class: attributes, methods, constructors, internal classes
Modifier class, can only be used: default, public
5. Structure of Classes 3: Constructors
1. Constructor (or construction method): Constructor
The role of the constructor:
- create object
- Information for initializing objects
2. Instructions for use
-
If there is no constructor that explicitly defines the class, a constructor with an empty parameter is provided by default
-
Define the format of the constructor: Permission modifier class name (parameter list) {}
-
Multiple constructors defined in a class that overload each other
-
Once we explicitly define the constructor for the class, the default empty parameter constructor is no longer available
-
There will be at least one constructor in a class.
3. Examples:
//constructor public Person(){ System.out.println("Person()....."); } public Person(String n){ name = n; } public Person(String n,int a){ name = n; age = a; }
Attribute Assignment Order
Summary: Order of attribute assignments
Default Initialization
(2) Explicit initialization
(3) Initialization in the constructor
(4) Assignment (which can be repeated) by means of "object-method" or "object-property"
The sequence of the above operations is: 1-2-3-4
The concept of JavaBean
A JavaBean is a Java class that meets the following criteria:
Classes are public
A public constructor with no parameters
Property, and corresponding get, set methods
Keyword: this
1. Callable structures: properties, methods; constructors
2.this calls properties and methods
this is understood as: the current object or the object currently being created
2.1 In a class's methods, we can call the current object's properties or methods using the "this.property" or "this.method" method.
In particular, if the formal parameters of a method and the attributes of a class have the same name, we must explicitly
2.2 In the constructor of a class, we can use the "this.attribute" or "this.method" to invoke the object properties or methods that are currently being created. However, in general, we choose to omit "this." In special cases, if the constructor parameters and class properties have the same name, we must explicitly use "this.variable"This means that the variable is an attribute, not a formal parameter.
3.this calls the constructor:
(1) In the constructor of a class, we can explicitly use the "this (parameter list)" method to invoke other constructors specified in this class
(2) You cannot call yourself in the constructor by "this (parameter list)"
(3) If there are n constructors in a class, at most n-1 constructors use "this (parameter list)"
(4) Provision: "This (list of formal parameters)" must be declared at the beginning of the current constructor
Inside a constructor, at most one "this (parameter list)" can be declared to invoke other constructors
Keyword: package/import
1. Use of packages
1.1 Instructions for use:
- To better manage the classes in your project, provide the concept of a package
- Use package to declare the package to which the class or interface belongs, declaring at the beginning of the source file
- A package, belonging to an identifier, that follows the naming rules, specifications (xxxyyzzz), and "Knowing what the name means"
- Each'. 'represents a layer of file directory.
1.2 Examples:
Example 1: A shipping software system includes a set of domain objects, GUI and reports subsystems
Example 2: MVC design pattern
The main introduction in 1.3 JDK:
2.Use of import
import:Import
- Import the classes and interfaces under the specified package explicitly using the import structure in the source file
- Declarations are between package and class declarations
- If multiple structures need to be imported, write them side by side
- You can use "xxx. *" to indicate that the structure under the XXX package can be imported
- If the class or interface used is defined under the java.lang package, the import structure can be omitted
- If the class or interface used is defined under this package, the import structure can be omitted
- If a class with the same name under different packages is used in the source file, at least one class must be displayed as the full class name.
- Use "xxx. *" to indicate that the structure under the XXX package can be invoked. However, if you are using the structure under the XXX subpackage, you still need to explicitly import it
- import static: Imports a static structure: property or method in a specified class or interface.