Java shortcuts
alt + /: intelligent supplementary keyword with prompt
crtl+alt + /: intelligent completion keyword without prompt
crtl+1: code correction
JAVA main class structure
The main() method is called the main class
The file name must have the same name as the name of the main class
Class becomes a global variable
Method becomes a local variable
Main method: public static void main(String[] args) {}
Import API class library
import . . .
Basic data type
Numeric:
Integrity: byte, short, int, long
Floating point type: float, double
Character type, boolean type
int a=1;
float f=12.3f;
doble d=2342.12d;
char x = 'w', only a single character can be written;
boolean b=true
identifier
Letters, underscores, dollar symbols and numbers. The first one cannot be a number or a reserved word
Reserved word
int continue public this finally boolean abstract continue float long short throw for static return
break else if goto package super default byte void do
try case switch private import protected extends double implemenets char . . .
constant
final data type constant name = value
final double p=12313.123d
Variable range
Variables defined in the class body are valid throughout the class
Member variable: no static
Static variable: with static, it can be used in this application, cross class, and value in the form of class name and static variable
Local variable: the variable in the method body. The local variable is destroyed after the method call. It will be overwritten if it has the same name as the member variable
Ternary operator
Conditional? Value 1: value 2;
From low to high: byte short int long float double
Direct assignment from low to high: float a=12;
High to low force: int a=(int)123.5f;
Compound statement
Block: {}
if conditional statement
if(){
}
if(){
}else{
}
if(){
}else if(){
}else if(){
}else{
}
switch(){
case():
{};
case():
{};
case():
{};
deflaut:{};
}
while(){
}
do{
}while(); / / loop at least once
for(int i=0;i<100;i++){
}
foreach(int x:obj){
}//x is every element in obj
break; jump out of the loop, but it's just a layer of loop
continue; jump out of this cycle
String class
The String class becomes an object
Declaration: String str
char can only be a single character.
Create: char
1,String s=new String(" ")
2,Strin s=new String(a,index,length)
3,String s=" "
4. Character array, String str [] = {}
String formatting
Date formatting
String.format(" ",date)
regular expression
reg="[]"
Connection string
+, connect two strings directly
Different types are connected to strings and are converted to strings
Get string length
str.length()
String lookup
Str.indexof ("substring"), you can find both the character and the index of the first occurrence of the substring
str.lastindexof("substring") can find both the character and the index of the last occurrence of the substring
Gets the character at the specified position
Str.charat (int index) returns the character at the index
str.substring(int beginindex) returns a substring from the start index position to the last position
str.substring(int beginindex,int lastindex) returns a substring from the start index position to the specified end index position, but does not include the character of the end index
Remove spaces before and after the string
str.trim()
String substitution
str.replace(char/string old,char/string new) if the original string is repeated many times, it will be replaced completely, and the case must be consistent
Determine the end of the string
startwidth(String) determines whether to start with a string
endwidth(String) determines whether to end with a string
The returned is Boolean
To judge whether the string is the same, you cannot use equal because it is to judge whether the string address is the same.
Use * * str1.equals (str2) to compare, and return true if it has the same characters and length
Compare with str1.equalsIgnoreCase(str2) * *, ignoring case.
Compare compareto() in dictionary order
str1.compareto(str2). str1 returns - 1 before str2, then 1, and the same returns 0
toggle case
To lowercase() to lowercase
toUppercase() to uppercase
Split string
str.split("") is divided by what delimiter, and the returned string array;
Str.splt (split character, split times)
format string
Date time string format: String.format("")
. . .
Frequently modify string
StringBuilder str=new StringBuilder("stringbuilder"); str.append("");Additional content str.insert(5,"word");Inserts content to the specified index location str.delete(3,5);The deleted content starts from 3 to 5 and does not contain the last index Output required str.toString();
StringBuilder str=new StringBuilder()
str.append()
str.insert()
str.delete()
The last of this class is toString()
array
State first:
int arr[];
int arr[][];
Allocate memory space for:
arr=new int[5];
arr=new int[2][4];
Allocate memory space for the declaration at the same time
int arr[]=new int[5];
Initialize one-dimensional array:
int arr[]=new int[]{1,2,3,4};
int arr[]={1,2,3,4};
int arr[][]={{1,2},{3,4,}};
Array element type [] name;
Array element type name [];
Array name [] = new int[length];
int numeric name [] = new int [];
initialization
int array name [] = new int[]{1,2,3,4,5}
2D array:
Array element type [] [] array name;
Array element type array name [] [];
Array name = new int [] []
initialization
int array name [] [] = new int[][]{1,2,3,4,5}
int array name [] [] = {}, {}};
Array length = array. Length;
Array length is solved with arr.length; different from string, str.length();
Fill replacement array
Arrays.fill(arr, object)
Arrays.fill(arr, start, end, object)
sort
Arrays.sort(obj); ascending
Copy array
copyof (arr, length) copies the entire array to an array of the specified length
Copyrange of (arr, fromindex, lastindex) does not include the last index
Array query
Sort before query;
Arrays.binarySearch(arr,obj)
Arrays.binarySearch(arr,fromindex,lastindex,obj)
Three sorting algorithms
Bubble sorting
import java.util.Arrays; public class BubbleSort { // Main method public static void main(String[] args) { int array[]=new int[] {63,4,24,1,3,15}; BubbleSort sort=new BubbleSort();//Create an object of bubble sort class and a sort object of BubbleSort class sort.sort(array);//Sort is the method of the sort object sort.showarray(array); } public void sort(int array[]) { for(int i=1;i<array.length;i++) { for(int j=0;j<array.length-i;j++) { if(array[j]>array[j+1]) { int temp; temp=array[j]; array[j]=array[j+1]; array[j+1]=temp; } } } } public void showarray(int array[]) { for(int i=0;i<array.length;i++) { System.out.println(array[i]); } } }
Direct selection sort
/** * */ /** * @author ASUS * */ public class SelectSort { /** * @param args */ public static void main(String[] args) { // Method stub automatically generated by TODO int array[]=new int[] {63,4,24,1,3,15}; SelectSort sort=new SelectSort(); sort.sort(array); BubbleSort Bubble=new BubbleSort(); Bubble.showarray(array); } public void sort(int array[]) { int index; for(int i=1;i<array.length;i++) { index=0;//Each time you start with the first element for(int j=1;j<=array.length-i;j++) { if(array[j]>=array[index]) { index=j; } int temp=array[array.length-i];//Swaps the last element of the specified unordered number with the maximum value array[array.length-i]=array[index]; array[index]=temp; } } } }
Reverse sort
public class ReverseSort { public static void main(String[] args) { // Method stub automatically generated by TODO int array[]= {10,20,30,40,50,60}; ReverseSort sort=new ReverseSort(); sort.sort(array); } public void sort(int array[]) { int temp; int len=array.length; for(int i=0;i<len/2;i++) { temp=array[i]; array[i]=array[len-i-1]; array[len-i-1]=temp; } BubbleSort bubble=new BubbleSort(); bubble.showarray(array); } }
object-oriented
object
An object is an instance abstracted from a class. An object conforms to an instance generated by a class
class
Classes are carriers that encapsulate attributes and methods
public class Test { [public|private|protected][static]<void|return_type><method_name>([paramList]) { // Method body } }
Formal parameters are the parameters that appear in the parameter list when defining a method, and actual parameters are the parameters passed for the method when calling the method.
A formal parameter variable allocates memory units only when it is called. At the end of the call, the allocated memory units are released immediately. Therefore, a formal parameter is only valid inside the method. After the method call ends and returns to the calling method, the formal parameter variable can no longer be used.
The number, type and order of arguments and formal parameters should be strictly consistent, otherwise the error of "type mismatch" will occur.
The data transfer in the method call is one-way, that is, the value of the argument can only be transferred to the formal parameter, but the value of the formal parameter cannot be transferred to the argument in the opposite direction. Therefore, during the method call, the value of the formal parameter changes, but the value in the actual parameter does not change
The data transfer of argument variable to formal parameter variable is "value transfer", that is, it can only be passed from argument to formal parameter, not from formal parameter to argument. When calling member method in the program, Java copies the argument value to a temporary storage area (stack), and any modification of formal parameter is carried out in the stack. When exiting the member method, Java automatically clears the contents of the stack
Member variable
Permission modifier (public, private, protected) variable type variable name;
Member method
Permission modifiers (public, private, protected) return value type method name (parameter type parameter name){
Method body;
Return return value;
}
void is used when there is no return value
If the member method has the same local variable as the member variable, the variable is treated as a local variable
Permission modifier
private: can only be used in this class
public: this class, subclass or other classes in the same package or other package classes
protected: this class, subclass or other classes in the same package
Declaut: only other classes and subclasses within a package can access this class
Do you
this
This keyword represents the application of this class object. Use this to call member variables and member methods in the class.
It can also be used as the return value of the method
return this returns the object of the class; This means:
public class testThis { public static void main(String[] args) { testThis t= new testThis(); t.A().B(); } testThis A() { System.out.println("A"); return this; } void B() { System.out.println("B"); } }
t.A() returns the instantiated object of testThis class, which is t Province, and then it can continue to execute t.B();
Construction method
Whenever a class instantiates an object, it will automatically call the construction method. The construction method has no return value, and the method name is the same as the class name; No need to decorate void
for example
public book(){ Member variable assignment initialization; }
Constructors can have parameters or no parameters. A parametric constructor is used to create a constructor with parameters when creating an object. A nonparametric constructor corresponds to a nonparametric constructor when creating an object.
public class Teacher { private String name; private int age; private double salary;//Three member variables public Teacher(String name,int age,double salary){ this.name=name; this.age=age; this.salary=salary; } public static void main(String[] args) { // Method stub automatically generated by TODO Teacher t1=new Teacher("hua",18,35000); System.out.println(t1.name+t1.age+t1.salary); } }
public void run() { jump(); System.out.println("Executing run method"); } public void run() { // Use this reference to the object that calls the run() method this.jump(); System.out.println("Executing run method"); }
When a method in a class calls other methods in the class, you can use the this. Method (), or directly use the method ();
This can also call the constructor. Only when this is
this() cannot be used in ordinary methods, but can only be written in constructor methods.
When used in a constructor, it must be the first statement.
public class AnyThing { public static void main(String[] args) { AnyThing test=new AnyThing(); } public AnyThing() { this("WO YI DING XING"); System.out.println("wucan"); } public AnyThing(String str) { System.out.println(str); } }
Static variables, constants, methods
static, which can be called by this class or other classes, with class name. static class member; It means that you can share the same data without creating it separately.
Static methods cannot use this, and static methods cannot directly call non static methods
Local variables in the method body cannot also be declared static
Main method of class
public static void main(String[] args){
}
The main method is static, so other methods in the direct calling class must also be static; The main method has no return value;
Args is a parameter of the program. You can use args,length;
object
establish
Creation of parameterless object: class name object name = new class name (); Automatic call to parameterless constructor
Creation of parameterized object: class name object name = new class name ("parameter"); Automatically call a parameterized constructor
Access object properties and behavior
Objects. Class members
Calling non static member variables
public class TransferProperty { int i=47; public void call() { System.out.println("call call()method"); for(int i=0;i<3;i++) { System.out.println(i); if(i==2) { System.out.println("\n"); } } } public TransferProperty() { } //main public static void main(String[] args) { TransferProperty t1=new TransferProperty(); TransferProperty t2=new TransferProperty(); t2.i=60; System.out.println("First instance object call t1:"+t1.i++); t1.call(); System.out.println("Second instance object call t2:"+t2.i); t2.call(); } }
When calling member variables, different objects call the same member variables, which are independent of each other because they point to different memory spaces.
Call static member variable
public class TransferProperty { static int i=47; public void call() { System.out.println("call call()method"); for(int i=0;i<3;i++) { System.out.println(i); if(i==2) { System.out.println("\n"); } } } public TransferProperty() { } //main public static void main(String[] args) { TransferProperty t1=new TransferProperty(); TransferProperty t2=new TransferProperty(); t2.i=60; System.out.println("First instance object call t1:"+ t1.i++); t1.call(); System.out.println("Second instance object call t2:"+t2.i); t2.call(); } }
Points to the same memory space, so the value of the static member variable changes. java.lang.Class Class Class object name = java.lang.Class.forName(Full name of the class to instantiate); Class name object name = (Class name)Class Class object name.newInstance(); call java.lang.Class Class forName() Method, you need the full name of the class to be instantiated (for example com.mxl.package.Student)Pass it as a parameter, and then call java.lang.Class Class object newInstance() Method to create an object. Class c1 = Class.forName("Student"); Student student2 = (Student)c1.newInstance(); use Class Class newInstance() Method when creating an object, it will call the default constructor of the class, that is, the parameterless constructor.
Class name object name = (Class name)Created class object name.clone(); // Call the clone() method of the object to create the object Student student3 = (Student)student2.clone(); use Object Class clone() Method when creating an object, it will not call the constructor of the class. It will create a copied object. This object has different memory addresses from the original object, but their property values are the same.
Anonymous object
If an object needs to be used only once, anonymous objects can be used, and anonymous objects can also be passed as actual parameters.
An anonymous object is an object without an explicit name. It is a short form of an object. Generally, anonymous objects are used only once, and anonymous objects only open up space in heap memory, without stack memory references.
new Person("Zhang San", 30)
No stack memory references it, so this object is used once and waits to be recycled by GC (garbage collection mechanism)
Therefore, you can directly use the new class name () to create objects;
Object reference
Class name object name; reference only stores the memory address of the object, not an object,
new class name (); is the creation object, class name and object name are references.
Object comparison
==Compare whether the addresses are the same
equals() compares whether the contents are the same
Destruction of objects
Garbage collection mechanism: object reference is out of scope; object assignment is null
example
import java.util.Scanner; public class MemberTest { public static void main(String[] args) { // Method stub automatically generated by TODO Member admin=new Member("admin","123456"); Scanner input=new Scanner(System.in); System.out.println("Please enter the original password:"); String pwd=input.next(); if(pwd.equals(admin.getUsername())) { System.out.println("Please enter a new password:"); admin.setPassword(input.next()); }else { System.out.println("The password entered is incorrect and cannot be modified"); } /*The object is printed directly. It seems that toString() is not called. In fact, toString() is called automatically by default *Consistent with the effect of admin.toString()*/ System.out.println("User information\n"+admin); } } implement System.out.println(obj);And System.out.println(obj.toString());The result of the method is the same.
public class Member { private String username; private String password; public String getUsername() { return this.username; } public void setUsername(String username) { this.username=username; } public String getPassword() { return this.password; } public void setPassword(String password) { this.password=password; } public Member(String username,String password) { this.username=username; this.password=password; } public String toString() { return "user name:"+this.username+"\n password:"+this.password; } }
Class annotation
Class annotation
Class annotations must generally be placed after all "import" statements and before class definition. It mainly declares what the class can do, as well as some information such as creator, creation date, version and package name. The following is a template of class annotations.
/** * @projectName(Project name): project_name * @package(package_name.file_name * @className(Class name): type_name * @description(Class description): describe the functions of this class in one sentence * @author(Created by: user * @createDate(Creation time): datetime * @updateUser(Modified by: user * @updateDate(Modification time): datetime * @updateRemark(Modification remarks): describe the content of this modification * @version(Version: v1.0 */
Method notes
- Method notes
The method annotation must be immediately in front of the method definition and mainly declare the method parameters, return values, exceptions and other information. In addition to general labels, you can also use the following labels starting with @.
@Param variable description: add a description to the parameter part of the current method, which can occupy multiple lines. All @ param tags of a method must be put together.
@Return type description: adds a return value part to the current method, which can span multiple lines.
@throws exception class description: indicates that this method may throw an exception.
/** * @param num1: Addend 1 * @param num2: Addend 2 * @return: Sum of two addends */ public int add(int num1,int num2) { int value = num1 + num2; return value; }
- field comment
Field comments are used to describe the meaning of the field before the definition field. The following is an example of a field annotation.
Plain text copy
/**
- user name
*/
public String name;
Access scope
Private friendly (default) protected public
The same class is accessible accessible accessible accessible
Other classes in the same package are not accessible
Subclasses in different packages are inaccessible inaccessible accessible
Non subclasses in different packages are inaccessible inaccessible inaccessible
Static code block refers to the static {} code block in Java classes, which is mainly used to initialize classes, assign initial values to static variables of classes, and improve program performance.
If the class contains multiple static code blocks, the Java virtual machine will execute them in the order they appear in the class, and each static code block will be executed only once.
When accessing non static methods, you need to access them through instance objects. When accessing static methods, you can access them directly, through class names, or through instantiated objects
Static variables can be shared by all instances of the class, so static variables can be used as shared data between instances to increase the interaction between instances.
If all instances of a class contain the same constant property, you can define this property as a static constant type, saving memory space. For example, define a static constant PI in a class.
packing
Consider these wrappers as classes. All wrapper classes (Integer, Long, Byte, Double, Float, Short) are subclasses of the abstract class Number.
Packaging basic data type
Boolean boolean
Byte byte
Short short
Integer int
Long long
Character char
Float float
Double double
Integer class
Construction method
Two construction methods with parameters:
Integer number=new Integer(123);
Integer number=new Integer(“123”); This method is no longer supported in jdk1.9
Now, Integer.ValueOf("") shall prevail,
Integer.toString(int par1,int par2),par1 Represents the number to be converted to a string, par2 Represents the hexadecimal representation to convert to Compare returned integer values obj.compareTo(obj2); str.compareTo(str2); Integer valueOf(int i): Returns a value representing the specified int Value Integer example. Integer valueOf(String s):Return to save the specified String Value of Integer Object. Integer valueOf(String s, int radix): Return a Integer Object, which saves the specified value when parsing with the cardinality provided by the second parameter String Value extracted from.
String
x.toString();
Integer.toString(12)
x.compareTo(3); If the specified number is equal to the parameter, 0 is returned.; Returns - 1 if the specified number is less than the parameter. Returns 1 if the specified number is greater than the parameter.
x.equals(Object o) return values True, False
The round() method returns the closest int, long value, rounded, math. Round (numeric value)
type Method and description byte byteValue() : with byte Returns the specified value as. abstract double doubleValue() : with double Returns the specified value as. abstract float floatValue() : with float Returns the specified value as. abstract int intValue() : with int Returns the specified value as. abstract long longValue() : with long Returns the specified value as. short shortValue() :
Connection string
The String class provides a method to connect two strings:
string1.concat(string2);
Returns the specified value as a short.
Math.min(a,b)
Math.max(a,b)
format string
system.out.printf(),String.format()
System.out.printf("The value of a floating-point variable is " + "%f, The value of an integer variable is " + " %d, The value of the string variable is " + "is %s", floatVar, intVar, stringVar); String fs; fs = String.format("The value of a floating-point variable is " + "%f, The value of an integer variable is " + " %d, The value of the string variable is " + " %s", floatVar, intVar, stringVar);
StringBuffer
Serial number Method description 1 public StringBuffer append(String s) Appends the specified string to this character sequence. 2 public StringBuffer reverse() Replace this character sequence with its inverted form. 3 public delete(int start, int end) Remove characters from substrings of this sequence. 4 public insert(int offset, int i) take int The string representation of the parameter is inserted into this sequence. 5 insert(int offset, String str) take str The string of the parameter is inserted into this sequence. 6 replace(int start, int end, String str) Use given String Replace the characters in the substring of this sequence with the characters in.
StringBuffer object. Methods (append(), insert(), delete(), replace(), reverse())
Character
\t Insert one here tab key \b Insert a back key here \n Line feed in the text \r Insert carriage return here in the text \f Insert a page feed here in the text \' Insert single quotation marks here \" Insert double quotation marks here in the text \\ Insert a backslash here in the text
Character.isUpperCase('c')Is the character to be tested c Capital letters of Character.toUpperCase('a')Characters to convert
char ch = 'a'; // Unicode character representation char uniChar = '\u039A'; // Character array char[] charArray ={ 'a', 'b', 'c', 'd', 'e' };
random class
Math.random() will generate a random double number of 0-1;
Instantiate the random class
Random number=new Random()
Packaging method
number.nextInt(n) randomly generates integers 0-n;
Randomly generated integer:
public class MathRandom { // /* * num1 Is the minimum and num2 is the maximum */ /** * * @param num1 Starting value * @param num2 Termination value * @return The return value is a random number */ // The document comment is / * * + enter //The multiline comment is ctrl+shift+/ public static int GetNum(double num1,double num2) { int s=(int)num1+(int)(Math.random()*(num2-num1));//Cast is (int) with parentheses, return s; /* * if(s%2==0) { return s; } else { return s+1; }Random even number */ } public static void main(String[] args) { // Method stub automatically generated by TODO System.out.println(GetNum(2,32));//Static methods can be called directly } }
Randomly generated characters:
Note the conversion relationship between character and int type
public class RandomChar { public static char GetChar(char a, char b) { return (char)(a+(Math.random()*(b-a+1))); } public static void main(String[] args) { // Method stub automatically generated by TODO System.out.println(GetChar('a','g')); } }
method
Modifier return value type method name(Parameter type parameter name){ ... Method body ... return Return value; }
Method overload
If you pass an int parameter when calling the max method, the max method with an int parameter will be called;
If a double parameter is passed, the max method of double type will be called, which is called method overloading;
That is, two methods of a class have the same name, but have different parameter lists.
Construction method
When an object is created, the constructor is used to initialize the object. The constructor has the same name as its class, but the constructor has no return value. Construction methods are usually used to assign initial values to instance variables of a class, or perform other necessary steps to create a complete object. No matter whether you customize the constructor or not, all classes have constructor, because Java automatically provides a default constructor. The access modifier of the default constructor is the same as that of the class (the class is public, and the constructor is also public; the class is changed to protected, and the constructor is also changed to protected). Once you define your own constructor, the default constructor will fail.
class MyClass { int x; // Here are the constructors MyClass() { x = 10; } }
main
When using the main() method, you should pay attention to the following points:
1. Access control permissions are public.
2. The main() method is static. If you want to call other methods in this class in the main() method, the method must also be static, otherwise you need to create the instance object of this class first, and then call the member method through the object.
3. The main() method has no return value and can only use void.
4. The main() method has a string array parameter that is used to receive command-line parameters for executing a Java program. Command line parameters are used as strings to correspond to the elements in the string array in order.
5. The name of the array in the string (args in the code) can be set arbitrarily, but according to custom, the name of this string array is generally consistent with the name of the main() parameter in the Java specification example, named args, and other contents in the method are fixed.
6. The definition of main() method must be "public static void main(String [] string array parameter name)".
7. A class can only have one main() method, which is a technique commonly used for unit testing of classes (checking and verifying the smallest testable unit in software).
Variable parameters
The syntax format for declaring variable parameters is as follows:
methodName({paramList},paramType...paramName)
Where, methodName represents the method name; paramList represents the fixed parameter list of the method; paramType indicates the type of variable parameter Is the identification of declaring variable parameters; paramName indicates the name of the variable parameter. Variable parameters must be defined at the end of the parameter list.
public void print(String...names) { int count = names.length; // Get total number
The Java construction method has the following characteristics:
1. The method name must be the same as the class name
3. You can have 0, 1, or more parameters
4. There are no return values, including void
5. The default return type is the object type itself
6. Can only be used with the new operator
Deconstruction method
protected void finalize() { // Object cleanup }
encapsulation
Encapsulation: the class hides the internal data and provides the user with the interface of object properties and behaviors
The specific steps to realize encapsulation are as follows:
Modify the visibility of attributes to restrict access to attributes, which is generally set to private.
Create a pair of setter and getter methods for each attribute, which are generally set to public for attribute reading and writing.
Add attribute control statements in the assignment and value taking methods (judge the legitimacy of attribute values)
Use get to access values and set assignments
public class Employee { private String name; // full name private int age; // Age private String phone; // contact number private String address; // Home address public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { // Age limits if (age < 18 || age > 40) { System.out.println("Age must be between 18 and 40!"); this.age = 20; // Default age } else { this.age = age; } } public String getPhone() { return phone; } public void setPhone(String phone) { this.phone = phone; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } }
public class EmployeeTest { public static void main(String[] args) { // Method stub automatically generated by TODO Employee people=new Employee(); people.setName("Wang Lili"); people.setAddress("Shijiazhuang, Hebei"); people.setAge(21); people.setPhone("12314141251"); System.out.println("full name:"+people.getName()); System.out.println("Age:"+people.getAge()); System.out.println("Address:"+people.getAddress()); System.out.println("Telephone:"+people.getPhone()); } }
inherit
For the relationship between two classes, subclasses inherit some properties and behaviors of the parent class, add properties and behaviors that the parent class does not have, or directly override some methods of the parent class.
extends indicates inheritance
Permission modifier return value type method name (parameter type parameter name){
}
Class name as return value type
If the return value type of a method is a class name, an object of that class will be returned
- You can use super() in a subclass to call the constructor of the parent class, or you can use super. Method in a subclass to call the member method of the parent class
- However, you do not have permission to call private methods. You can only call public and protected in the parent class
- You can also define some new methods in subclasses
The syntax format of Java subclass inheriting parent class is as follows:
Modifier class class_name extends_class{
//Body of class
}
Where class_name represents the name of the subclass (derived class); extend_class represents the name of the parent class (base class); the extends keyword directly follows the subclass name, followed by the name of the parent class to be inherited by the class. For example:
public class Student extends Person{}
rewrite
Keep the method name of the parent class, override the content of the method, modify permissions, and return value types.
restructure
The only difference is that the implementation content of the method is different.
Modify permissions
When overriding a method, the modification permission can only be from small to large, that is, the modification scope of the method cannot be reduced.
For example, the parent class method is protected, and the child class modification can only be public
return type
The overridden return value type must be a subclass of the same method return value type in the parent class, i.e
Test2 is a subclass of test (parent class)
When instantiating a child object, the parent object will also be instantiated, that is, the parameterless construction method of the parent class will be called automatically, and the object will be instantiated first from the top-level parent class, then the upper level parent class, and finally the child class, and can only be a parameterless constructor. The constructor with parameters must be called through the call displayed in super
Object
Object is the parent class of all classes. Extensions object is omitted. Any class can override its methods, such as clone(), finalize(), equals(), toString()
Methods of final type cannot be overridden, such as getClass(), notify(), notifyAll(), wait()
1. getClass() returns the Class instance executed by the object; get the name of the Class through getName();
2. toString() returns the object as a string,
3. equals returns false when comparing different instantiated objects with the same comparison content
Upward transformation
The parent class reference points to the child class object, which is an upward transformation. The syntax format is as follows:
fatherClass obj = new sonClass();
Where fatherClass is the parent class name or interface name, obj is the created object, and sonClass is the child class name.
Treating a child object as a parent object is called an upward transformation
Parent class name parent class object = new subclass name ();
public class People { public String name; public int age; public String sex; public String sn; public People(String name,int age,String sex,String sn) { this.name=name; this.age=age; this.sex=sex; this.sn=sn; } public String toString() { return "full name:"+name+"\n Age:"+age+"\n Gender:"+sex+"\n ID"+sn; } } class Student extends People{ private String stuNo; private String department; /*If a constructor with parameters exists in the parent class and no constructor without parameters is overloaded, * Then the subclass must contain a construction method with parameters, * Because if there is no constructor in the subclass, * By default, the parameterless constructor in the parent class will be called, but there is no parameterless constructor in the parent class, so an error will occur.*/ public Student(String name,int age,String sex,String sn,String stuNo,String department) { super(name,age,sex,sn);//Parameterized constructor this.department=department; this.stuNo=stuNo; } /*The Student class also has the properties and methods of the People class. Here, the toString() method in the parent class is overridden.*/ public String toString() { return "full name:"+name+"\n Age:"+age+"\n Gender:"+sex+"\n ID"+sn+"\n Student No.:"+stuNo+"\n Major:"+department; } } class Teachers extends People{ private int tYear; private String tDept; public Teachers(String name, int age, String sex, String sn,int tYear,String tDept) { super(name, age, sex, sn); this.tYear=tYear; this.tDept=tDept; } public String toString() { return "full name:"+name+"\n Age:"+age+"\n Gender:"+sex+"\n ID"+sn+"\n Teaching age:"+tYear+"\n Major taught:"+tDept; } } class PeopleTest{ public static void main(String[] args) { //Student s can be replaced by People Student p=new Student("Wang Li",23,"female","12312412541","123124","Computer application"); System.out.println(p); //Teachers can be replaced by People Teachers p1=new Teachers("Zhang Zong",23,"female","12312412541",5,"Computer application"); System.out.println(p1); } }
super
Functions of super keyword:
Explicitly call the parent class constructor in the subclass constructor
Access member methods and variables of the parent class.
Calling the parameterless constructor of the parent class must be written in the first sentence of the subclass constructor
super Keyword can explicitly call the construction method of the parent class in the construction method of the child class. The basic format is as follows: super(parameter-list);
Using super to access members in the parent class is similar to using the this keyword, except that it refers to the parent class of the child class: super.member
What's the difference between super and this
this refers to the reference of the current object, and super refers to the reference of the parent object of the current object
super Keyword usage: super.Parent class property name: call the property in the parent class super.Parent class method name: call the method in the parent class super(): Call the parameterless constructor of the parent class super(parameter): Call the parameterized constructor of the parent class If the first line of the constructor is not this() and super(),The system will add by default super(). this Keyword usage: this.Property name: represents the property of the current object this.Method name(parameter): Represents the method that calls the current object
Downward transformation
Cast a parent object to a child object,
Subclass name subclass object name = (subclass name) parent object name;
In contrast to the upward transformation, the reference of the subclass object to the parent class is a downward transformation. The syntax format is as follows:
sonClass obj = (sonClass) fatherClass;
Where fatherClass is the parent class name, obj is the created object, and sonClass is the child class name.
Cast type
((Cat)animal).str = ""; // Compilation succeeded ((Cat)animal).eatMethod(); // Compilation succeeded
instanceof judge object type
myobject instanceof exampleClass
Judge whether an instance object myobject belongs to a class, exampleClass. If it belongs to, it returns true, but not false.
It is usually judged whether the parent object is an instance of a child object
1) Declare an object of class and judge whether obj is an instance object of class (a very common usage), as shown in the following code:
Integer integer = new Integer(1);
System.out.println(integer instanceof Integer); // true
public class Parellelogram extends Qua{ public static void main(String[] args) { // Method stub automatically generated by TODO Qua q=new Qua(); if(q instanceof Parellelogram) { Parellelogram p=(Parellelogram) q;//Cast down } if(q instanceof squ) { squ s=(squ) q;//Cast down } } } class Qua{ public static void draw(Qua q) { } } class squ extends Qua{ } class anything1{ }
heavy load
The requirements of method overloading are the same and different: the method name in the same class is the same, and the parameter list is different, such as method return value type, modifier, etc., which has nothing to do with method overloading.
More than one method with the same name is allowed in the same class, as long as the number of parameters or parameter types of these methods are different.
public class OverLoadTest { public static int add(int a,int b) { return a+b; } public static double add(double a, double b) { return a+b; } public static int add(int a) { return a; } public static int add(int a,double b) { return 1; } public static int add(double a, int b) { return 1; } public static void main(String[] args) { // Method stub automatically generated by TODO System.out.println(add(1,2)); System.out.println(add(2.1,3.3)); System.out.println(add(1)); System.out.println(add(2.1,3)); } }
Different parameter types, numbers and sequences can constitute overloads
Variable length parameter overload
In essence, an array is passed in, with parameter type... Parameter name
public class OverLoadTest2 { public static int add(int a,int b) { return a+b; } public static double add(double a, double b) { return a+b; } public static int add(int a) { return a; } public static int add(int a,double b) { return 1; } public static int add(double a, int b) { return 1; } /* * Variable length parameter overload */ public static int add(int...a) { int sum=0; for(int i=0;i<a.length;i++) { sum+=a[i]; } return sum; } public static void main(String[] args) { // Method stub automatically generated by TODO System.out.println(add(2)); System.out.println(add(1,2,3,4,5,6,7,8,9)); } }
polymorphic
Inheritance: there must be subclasses and parent classes with inheritance relationship in polymorphism.
Override: a subclass redefines some methods in the parent class. When these methods are called, the subclass's methods will be called.
Upward Transformation: in polymorphism, you need to assign the reference of the subclass to the parent object. Only in this way can the reference call both the methods of the parent class and the methods of the subclass.
Parent class objects are applied to the characteristics of child classes. Polymorphism can make the program extensible and have universal processing for all class objects.
You cannot make static references to non static fields
class Square extends Quadrangle{ public Square() { System.out.println("square"); } } class Parellelogramgle extends Quadrangle{ public Parellelogramgle() {//The constructor does not return a value, so there is no return value type System.out.println("rectangle"); } } public class Quadrangle { private Quadrangle qtest[]=new Quadrangle[6];//Member variable, decorated as private, instantiated array object private int nextindex=0;//Private member variable // Quadrangle q is the object of this class public void draw(Quadrangle q) {//Public member method if(nextindex<qtest.length) { qtest[nextindex]=q; System.out.println(nextindex); nextindex++; } } /*Different classes of objects call the draw() method as parameters, which can deal with different graphic problems * Just instantiate a collection of subclass objects that inherit the parent class and call the corresponding methods*/ public static void main(String[] args) { // Method stub automatically generated by TODO Quadrangle q=new Quadrangle();//Instantiation of this class of objects q.draw(new Square());//The square object calls draw() as a parameter; q.draw(new Parellelogramgle());//The rectangular object calls draw() as a parameter; } }
No matter whether the object of the figure variable is Rectangle or Triangle, they are all subclasses of the figure class, so they can be transformed upward to this class to realize polymorphism
public class Figure { double dim1; double dim2; Figure(double d1,double d2){ this.dim1=d1; this.dim2=d2; } double area() { System.out.println("The method of calculating the object area in the parent class has no practical significance and needs to be overridden in the child class."); return 0; } } class Rectangle extends Figure{ Rectangle(double d1,double d2){ super(d1,d2); } double area() { System.out.println("Area of rectangle"); return super.dim1*super.dim2; } } class Triangle extends Figure{ Triangle(double d1,double d2){ super(d1, d2); } double area() { System.out.println("Area of triangle"); return super.dim1*super.dim2/2; } } class Test{ public static void main(String[] args) { Figure figure;//Declare a variable of type figure //Upward transformation /*In the main() method of this class, first declare the variable figure of figure class, * Then specify different objects for the figure variable, * And call the area() method of these objects*/ figure=new Rectangle(9,9); System.out.println(figure.area()); figure=new Triangle(6,8); System.out.println(figure.area()); figure=new Figure(10,10); System.out.println(figure.area()); } }
abstract class
public abstract class Test{ abstract void test();Define abstract methods }
The abstract class that hosts the abstract method must be inherited, otherwise it has no meaning. To declare an abstract method, the class that hosts the abstract method must be defined as an abstract class.
All subclasses of an abstract class must override the abstract methods in the abstract class, that is:
Parent class: abstract void test()
Subclass: void test()
Abstract method has no method body
In Java, the syntax format of abstract classes is as follows:
<abstract>class<class_name> { <abstract><type><method_name>(parameter-iist); }
Abstract means that the class or method is abstract; class_name indicates the name of the abstract class; method_name represents the abstract method name, and parameter list represents the method parameter list.
If a method is modified with abstract, it indicates that the method is an abstract method, which has only a declaration but no implementation. It should be noted that abstract
Keywords can only be used in ordinary methods, not static methods or constructor methods.
The three characteristics of abstract methods are as follows: abstract methods have no method body. Abstract methods must exist in subclasses of abstract classes. When overriding the parent class, all abstract methods of the parent class must be overridden
Interface
[public] interface interface_name [extends interface1_name[, interface2_name,...]] { // Interface body, which can contain defined constants and declared methods [public] [static] [final] type constant_name = value; // Define constants [public] [abstract] returnType method_name(parameter_list); // Declaration method }
The interface with public access control character allows any class to use; No public interface is specified, and its access will be limited to the package to which it belongs.
The method declaration does not require other modifiers. The methods declared in the interface will be implicitly declared as public and abstract.
The variables declared in the Java interface are constants. The variables declared in the interface will be implicitly declared as public, static and final, that is, constants. Therefore, the variables defined in the interface must be initialized.
The interface has no constructor and cannot be instantiated
public interface drawTest{ void draw();Methods within the interface } Permission modifier interface Interface keyword interface name{ }
The method defined in the interface must be defined as public or abstract.
Any field in the interface is automatically static and final
Implementation interface
Implementing an interface is similar to inheriting a parent class. You can obtain constants and methods defined in the implemented interface. If a class needs to implement multiple interfaces, multiple interfaces are separated by commas.
A class can inherit a parent class and implement multiple interfaces at the same time. The implements part must be placed after the extensions part.
After a class implements one or more interfaces, the class must fully implement all the abstract methods defined in these interfaces (that is, rewrite these abstract methods); Otherwise, the class will retain the abstract methods inherited from the parent interface, and the class must also be defined as an abstract class.
public> class <class_name> [extends superclass_name] [implements interface1_name[, interface2_name...]] { // subject }
Similarities between interface and class:
An interface can have multiple methods.
The interface file is saved in a file ending in. java, and the file name uses the interface name.
The bytecode file of the interface is saved in the file at the end of. class.
The bytecode file corresponding to the interface must be in the directory structure matching the package name.
Differences between interfaces and classes:
Interfaces cannot be used to instantiate objects.
Interface has no constructor.
All methods in the interface must be abstract methods. After Java 8, you can use the default keyword to modify non abstract methods in the interface.
An interface cannot contain member variables except static and final variables.
Interfaces are not inherited by classes, but implemented by classes.
The interface supports multiple inheritance.
Interface characteristics
Each method in the interface is also implicitly abstract. The methods in the interface will be implicitly specified as public abstract (only public abstract, and other modifiers will report errors).
The interface can contain variables, but the variables in the interface will be implicitly specified as public static final variables (and can only be public. Modifying with private will report compilation errors).
The methods in the interface cannot be implemented in the interface. The methods in the interface can only be implemented by the class that implements the interface.
...implements Interface name[, Other interface names, Other interface names..., ...] ...
use interface For this keyword, all methods in the interface must only declare the method id, not the specific method body, because the implementation of the specific method body is implemented by the class that inherits the interface. Therefore, the interface does not care about the specific implementation. The default attribute in the interface is Public Static Final.A class implementing this interface must implement all the abstract methods defined in this interface.
A simple interface is like this: it has global variables and abstract methods.
Use the implements keyword to implement the interface:
Declare the USB interface first: it specifies that the read() and write() methods must be implemented to implement the USB interface.
Define an interface
interface USB { void read(); void write(); }
Write two types to implement this interface
class YouPan implements USB { @Override public void read() { System.out.println("U Disk passing USB Function reading data"); } @Override public void write() { System.out.println("U Disk passing USB Function write data"); } } class JianPan implements USB { @Override public void read() { System.out.println("Keyboard passing USB Function reading data"); } @Override public void write() { System.out.println("Keyboard passing USB Function write data"); } }
public class Main { public static void main(String[] args) { //Generate a USB drive object that can realize USB interface (standard) YouPan youPan = new YouPan(); //Call the read() method of U SB flash disk to read data youPan.read(); //Call the write() method of U SB flash disk to write data youPan.write(); //Generate a keyboard object that implements a USB interface (standard) JianPan jianPan = new JianPan(); //Call the read() method of the keyboard to read the data jianPan.read(); //Call the write() method of the keyboard to write data jianPan.write(); } }
rewrite
In a subclass, if a method with the same name, return value type and parameter list as the parent class is created, but the implementation in the method body is different to achieve functions different from the parent class, this method is called method override, also known as method override.
1. The parameter list must be exactly the same as the overridden method parameter list.
2. The return type must be the same as the return type of the overridden method
3. The access permission cannot be lower than that of the overridden method in the parent class (public > protected > Default > private).
4. Rewriting methods must not throw new check exceptions or check exceptions broader than the declaration of the rewritten method. For example, a method of the parent class declares a check Exception IOException. When overriding this method, you cannot throw an Exception, only the subclass exceptions of IOException can be thrown, and non check exceptions can be thrown.
Overridden methods can be identified using the @ Override annotation.
Member methods of a parent class can only be overridden by its subclasses.
Methods declared final cannot be overridden.
Methods declared as static cannot be overridden, but can be declared again.
Constructor cannot be overridden.
When the subclass and the parent are in the same package, the subclass can override all methods of the parent, except those declared as private and final.
When the subclass and the parent class are not in the same package, the subclass can only override the non final methods declared as public and protected by the parent class.
If you cannot inherit a method, you cannot override it.
Use the reference of interface type to point to an object that implements the interface, and you can call the methods in the interface
This is the core meaning of the interface. The above implementation interface can also be written as follows:
public class Main { public static void main(String[] args) { //Generate a USB drive object that can realize USB interface (standard) //But use an interface reference to point to an object //The USB interface class reference can point to an object that implements the USB interface USB youPan = new YouPan(); //Call the read() method of U SB flash disk to read data youPan.read(); //Call the write() method of U SB flash disk to write data youPan.write(); //Generate a keyboard object that implements a USB interface (standard) //But use an interface reference to point to an object //The USB interface class reference can point to an object that implements the USB interface USB jianPan = new JianPan(); //Call the read() method of the keyboard to read the data jianPan.read(); //Call the write() method of the keyboard to write data jianPan.write(); } }
new YouPan() is the object to implement the interface, which points to the USB interface class reference
new JianPan() is the object to implement the interface, which points to the USB interface class reference
In Java, multi inheritance of classes is illegal, but interfaces allow multi inheritance.
In the multi inheritance of an interface, the extends keyword only needs to be used once, followed by the inherited interface. As follows:
public interface Hockey extends Sports, Event
package
The package statement should be placed on the first line of the source file. There can only be one package definition statement in each source file
package Package name;
The naming rules for Java packages are as follows:
Package names are all lowercase letters (multiple words are all lowercase).
If the package name contains multiple levels, each level is separated by ".".
The package name usually starts with an inverted domain name, such as com.baidu, without www.
Custom packages cannot start with java.
Function of package
1. Organize similar or related classes or interfaces in the same package to facilitate the search and use of classes.
2. Like folders, packages are stored in a tree directory. The class names in the same package are different, and the class names in different packages can be the same. When calling classes with the same class name in two different packages at the same time, the package name should be added to distinguish them. Therefore, the package can avoid name conflicts.
3. Packages also limit access rights. Only classes with package access rights can access classes in a package.
package pkg1[.pkg2[.pkg3...]]; for example package net.java.util; net/java/util/Something.java Package name.Class name
import package1[.package2...].(classname|*); use import Keyword introduction, using wildcards *: import payroll.*; use import Keyword introduction Employee Class: import payroll.Employee;
Inner class
If class B is defined in class A, class B is an inner class, also known as a nested class. Relatively speaking, class A is an outer class. If there is multi-layer nesting, for example, there is an inner class B in class A and an inner class C in class B, the outermost class is usually called the top-level class (or top-level class).
The internal class is still an independent class. After compilation, the internal class will be compiled into an independent. Class file, but preceded by the class name and $symbol of the external class.
Inner classes cannot be accessed in the normal way. The inner class is a member of the outer class, so the inner class can freely access the member variables of the outer class, whether private or not.
If the internal class is declared static, you can't access the member variables of the external class at will, but you can only access the static member variables of the external class.
There are only two access levels for external classes: public and default; Internal classes have four access levels: public, protected, private and default.
package com.dao; //public and default public class Test { // The external class accesses the internal class directly through the class name of the internal class InnerClass ic = new InnerClass(); // public, protected, private, and default. public class InnerClass{ public int getSum(int x,int y) { return x+y; } } public static void main(String[] args) { // Method stub automatically generated by TODO Test.InnerClass ti=new Test().new InnerClass(); int i=ti.getSum(2, 5); System.out.println(i); } }
public class Outer { int a = 10; class Inner { int a = 20; int b1 = a; int b2 = this.a; int b3 = Outer.this.a; } public static void main(String[] args) { Inner i = new Outer().new Inner(); System.out.println(i.b1); // Output 20 System.out.println(i.b2); // Output 20 System.out.println(i.b3); // Output 10 } }
Create a static inner class instance
1)When you create an instance of a static inner class, you do not need to create an instance of an outer class. Plain text copy public class Outer { static class Inner { } } class OtherClass { Outer.Inner oi = new Outer.Inner(); }
Accessing members of static inner classes
2)Static members and instance members can be defined in static inner classes. Classes other than the external class need to access the static members in the static internal class through the complete class name. If you want to access the instance members in the static internal class, you need to access the instance of the static internal class. Plain text copy public class Outer { static class Inner { int a = 0; // Instance variable a static int b = 0; // Static variable b } } class OtherClass { Outer.Inner oi = new Outer.Inner(); int a2 = oi.a; // Access instance members int b2 = Outer.Inner.b; // Accessing static members }
Accessing members of an external class
Static internal classes can directly access static members of external classes. If you want to access instance members of external classes, you need to access them through instances of external classes. Plain text copy public class Outer { int a = 0; // Instance variable static int b = 0; // Static variable static class Inner { Outer o = new Outer; int a2 = o.a; // Access instance variables int b2 = b; // Accessing static variables } }
Local inner class
A local inner class is an inner class defined in a method. The example code is as follows:
public class Test {
public void method() {
class Inner {
//Local inner class
}
}
}
Local internal classes have the following characteristics:
1) Like local variables, local internal classes cannot be modified with access control modifiers (public, private and protected) and static modifiers.
2) Local inner classes are only valid in the current method.
public class Test {
Inner i = new Inner(); // Compilation error
Test.Inner ti = new Test.Inner(); // Compilation error
Test.Inner ti2 = new Test().new Inner(); // Compilation error
public void method() {
class Inner{
} Inner i = new Inner(); }
}
3) static members cannot be defined in local inner classes
4) Local inner classes can also contain inner classes, but these inner classes cannot be decorated with access control modifiers (public, private and protected) and static modifiers.
5) All members of the external class can be accessed in the local internal class.
Anonymous class
An anonymous class is an internal class without a class name. It must be declared with a new statement when it is created. Its grammatical form is as follows:
Plain text copy
New < class or interface > (){
//Body of class
};
Inherit a class and override its methods.
Implement an interface (which can be multiple) and implement its methods.
Effectively final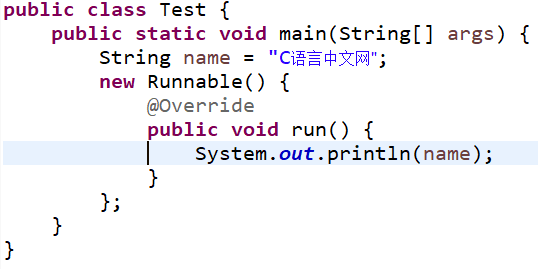
A non final local variable or method parameter whose value has never changed after initialization, then the variable is effectively final.
Lambda expression: take the function as the parameter of a method (the function is passed into the method as the parameter)
Printf mainly inherits some features of printf in C language and can be formatted for output.
print is the general standard output, but does not wrap.
println is basically no different from print, but it will wrap in the end.
Lambda The standard syntax of the expression is as follows: (parameter list) -> { // Lambda expression body } ->Is called an arrow operator or Lambda Operator, arrow operator Lambda The expression is split into two parts: left: Lambda A list of arguments to the expression. right: Lambda The function to be executed in the expression, using{ }Wrap it up, i.e Lambda Body.
package com.lzw; public class Test { /** * Through the operator, the calculation is carried out * * @param opr Operator * @return Implement Calculable interface object */ public static Calculable cal(char opr) { Calculable res; if(opr=='+') { // Implementation of Calculable interface with Lambda expression res=(int a,int b)-> { return a+b; }; }else { // Anonymous function implementation interface /* new Class name / interface{ Class body; }*/ res=new Calculable() { @Override public int calculate(int a,int b) { return a-b; } }; } return res;//The return value is the object that implements the Calculable interface } public static void main(String[] args) { // Method stub automatically generated by TODO int n1=10; int n2=5; Calculable f1=cal('+'); Calculable f2=cal('-'); System.out.println(f1.calculate(n1,n2)); System.out.println(f2.calculate(n1,n2)); } }
Lambada expression can only have one abstract method interface, @ FunctionalInterface. This annotation can be used for the definition of an interface. Once this annotation is used to define an interface, the compiler will forcibly check whether the interface does have and only has one abstract method, otherwise an error will be reported
package com.lzw; @FunctionalInterface public interface Calculable { int calculate(int a,int b) ; }
The internal class this. Member directly calls the internal class member, and the external class. This. Member calls the external class member
abnormal
An exception is an exception that occurs when a program is running
There are three main reasons for an exception in Java:
1. An exception occurred in the Java internal error, which is generated by the Java virtual machine.
2. Exceptions caused by errors in the written program code, such as null pointer exceptions, array out of bounds exceptions, etc.
3. The exception manually generated by throw statement is generally used to inform the caller of the method of some necessary information.
The process of generating an exception object and submitting it to the runtime system is called throw exception. The runtime system looks up in the method call stack until it finds an object that can handle this type of exception. This process is called catch exception
In Java, all exception types are subclasses of the built-in java.lang.Throwable class, namely Throwable
It is at the top level of the Exception class hierarchy. Under the Throwable class, there are two Exception branches, Exception and Error
Exception class is used for possible exceptions in user programs. It is also used to create custom exception type classes.
Error defines an exception that you do not want to be caught by a program in a normal environment. It generally refers to JVM errors, such as stack overflow.
Runtime exceptions are RuntimeException class and its subclasses, such as NullPointerException, IndexOutOfBoundsException, etc., which are caused by program logic errors.
Non runtime exceptions refer to exceptions other than RuntimeException, which belong to Exception class and its subclasses. From the perspective of program syntax, they are exceptions that must be handled. If they are not handled, the program cannot be compiled. For example, IOException, ClassNotFoundException, and user-defined Exception.
Runtime exception
Non runtime exception
1) Runtime exception:
NullPropagation: null pointer exception;
ClassCastException: type cast exception
IllegalArgumentException: illegal parameter exception passed
IndexOutOfBoundsException: subscript out of bounds exception
NumberFormatException: number format exception
2) Non runtime exception:
ClassNotFoundException: the exception for the specified class was not found
IOException: IO operation exception
3) Error:
NoClassDefFoundError: class definition exception not found
StackOverflowError: an exception thrown when the stack is exhausted due to deep recursion
OutOfMemoryError: memory overflow exception
exception handling
The try catch statement is used to catch and handle exceptions, the finally statement is used for code that must be executed in any case (except special cases), the throw statement is used to throw exceptions, and the throws statement is used to declare possible exceptions.
In the method, try catch statements are used to catch and handle exceptions. There can be multiple catch statements to match multiple exceptions.
For the exception that cannot be handled or the exception to be transformed, throw the exception through the throw statement at the method declaration, that is, the calling method of the upper layer will handle it.
Basic structure
try { Logic block } catch(ExceptionType1 e) { Processing code block 1 } catch (ExceptionType2 e) { Processing code block 2 throw(e); // Then throw this "exception" } finally { Release resource code block }
try { // Statements with possible exceptions } catch(ExceptionType e) { // Handling exception statements } try The variable declared in the block is only a local variable in the code block, which is only try The variable is valid in the block and cannot be accessed elsewhere. printStackTrace() Methods: point out the type, nature, stack level and location of exceptions in the program getMessage() Method: output the nature of the error. toString() Methods: the types and properties of exceptions are given. If try If an exception occurs in the statement block, a corresponding exception object will be thrown, and then catch Statement will be caught and processed according to the type of exception object thrown. After processing, the program will skip try For the remaining statements in the statement block, go to catch The first statement after the statement block begins execution. If try If no exception occurs in the statement block, then try The block ends normally, followed by catch The statement block is skipped and the program will start from catch The first statement after the statement block begins execution.
try { // Statements with possible exceptions } catch(ExceptionType e) { // Handling exception statements } catch(ExceptionType e) { // Handling exception statements } catch(ExceptionType e) { // Handling exception statements ... } In multiple catch In the case of code blocks, when one catch When the code block catches an exception, the others catch The code block is no longer matched.
When there is a parent-child relationship between multiple exception classes captured, the child class is usually captured first, and then the parent class. Therefore, the subclass exception must be in front of the parent exception, otherwise the subclass cannot catch it
- When you cast an object that does not have an inheritance relationship, a Java cast exception (java.lang.ClassCastException) will be thrown
- The statement in the finally block is executed after the return statement in try or catch is executed and before the return. Moreover, the "interference" in the finally statement may or may not affect the return value determined by the return in try and catch. If there is also a return in finally, the return statement in try and catch will be overwritten and returned directly.
- The idea of throwing an exception using the throws declaration is that the current method does not know how to handle this type of exception, and the exception should be handled by the caller up one level; If the main method does not know how to handle this type of exception, it can also throw an exception using the throws declaration, which will be handed over to the JVM. The JVM handles exceptions by printing the trace stack information of the exception and stopping the program. This is why the previous program ends automatically after encountering an exception.
- The exception type thrown by the subclass method declaration should be a subclass or the same as the exception type thrown by the parent method declaration. The subclass method declaration is not allowed to throw more exceptions than the parent method declaration.
throw ExceptionObject;
The differences between the throws keyword and the throw keyword are as follows:
1. throws is used to declare all exception information that may be thrown by a method, indicating a possibility of exceptions, but these exceptions do not necessarily occur; Throw refers to a specific exception type thrown. Executing throw must throw an exception object.
Usually, the exception information that may be thrown by a method (class) is declared through throws at the declaration of a method (class), while a specific exception information is declared through throw inside a method (class).
2. throws usually captures exceptions without display, and the system can automatically throw all captured exception information to the superior method; Throw requires the user to capture relevant exceptions, then wrap them, and finally throw the wrapped exception information.
Multi exception capture
try{ // Statements with possible exceptions } catch (IOException | ParseException e) { // Call methodA processing method }
//When multiple exceptions are caught, the exception variable is decorated with final by default
//So the following code is wrong
e = new ArithmeticException("test");
//When a type of exception is caught, the exception variable has no final modification
//So the following code is completely correct
e = new RuntimeException("test");
Custom exception
To implement the custom Exception class, you need to inherit the Exception class or its subclasses. If the custom runtime Exception class needs to inherit the RuntimeException class or its subclasses.
The syntax form of the custom exception is: <class><Custom exception name><extends><Exception> In the coding specification, the class name of the custom exception class is generally named XXXException,among XXX Used to represent the role of the exception.
Custom exception classes generally contain two construction methods: one is the default construction method without parameters, and the other receives a custom exception message in the form of string and passes the message to the construction method of superclass.
class IntegerRangeException extends Exception { public IntegerRangeException() { super(); } public IntegerRangeException(String s) { super(s); } }
Exception tracking stack
The printStackTrace() method of the exception object is used to print the tracking stack information of the exception. According to the output result of the printStackTrace() method, developers can find the source of the exception and track the process of triggering the exception all the way.
Exception in thread "main" Test.SelfException: custom exception information
at Test.PrintStackTraceTest.thirdMethod(PrintStackTraceTest.java:26)
at Test.PrintStackTraceTest.secondMethod(PrintStackTraceTest.java:22)
at Test.PrintStackTraceTest.firstMethod(PrintStackTraceTest.java:18)
at Test.PrintStackTraceTest.main(PrintStackTraceTest.java:14)
The content between lines 2 and 5 is the exception tracking stack information. From the printed exception information, we can see that the exception is triggered from the thirdMethod method, passed to the secondMethod method, then to the firstMethod method, and finally to the main method. When the main method terminates, this process is the exception tracking stack of Java
Java.util.logging: JDK's own logging class
If you want to generate a simple log record, you can use the global logger and call its info method. The code is as follows:
Logger.getGlobal().info("Print information");
Collection
The Collection interface is the parent interface of the List, Set and Queue interfaces, and is not used directly under normal circumstances
Method name Description
Boolean add (E) adds an element to the collection. If the collection object is changed by the addition operation, it returns true. E is the data type of the element
boolean addAll(Collection c) adds all elements in collection c to the collection. If the collection object is changed by the addition operation, it returns true.
void clear() clears all elements in the collection and changes the length of the collection to 0.
boolean contains(Object o) determines whether the specified element exists in the collection
boolean containsAll(Collection c) determines whether the collection contains all elements in collection c
boolean isEmpty() determines whether the collection is empty
Iterator iterator () returns an iterator object to iterate over the elements in the collection
boolean remove(Object o) deletes a specified element from the collection. When the collection contains one or more elements o, this method only deletes the first qualified element, and this method will return true.
boolean removeAll(Collection c) deletes all elements appearing in collection c from the collection (equivalent to subtracting collection c from the collection calling the method). If the operation changes the collection that calls the method, the method returns true.
Boolean retain all (collection c) deletes the elements not contained in collection c from the collection (equivalent to turning the collection calling the method into the intersection of the collection and collection c). If the operation changes the collection calling the method, the method returns true.
int size() returns the number of elements in the collection
Object[] toArray() converts a collection into an array, and all collection elements become corresponding array elements.
package com.list; import java.util.*; public class Muster { public static void main(String[] args) { // Method stub automatically generated by TODO Collection <String> list= new ArrayList<>();//Instantiate the Collection interface list.add("a"); list.add("b"); list.add("c"); System.out.println(list.size()); Iterator <String> it=list.iterator(); while(it.hasNext()) { String str=(String)it.next(); System.out.println(str); } } }
List collection
public static void main(String[] args) { ArrayList list1 = new ArrayList(); // Create collection list1 ArrayList list2 = new ArrayList(); // Create collection list2 list1.add("one"); list1.add("two"); list1.add("three"); System.out.println("list1 Number of elements in the collection:" + list1.size()); // Output the number of elements in list1 list2.add("two"); list2.add("four"); list2.add("six"); System.out.println("list2 Number of elements in the collection:" + list2.size()); // Output the number of elements in list2 list2.remove(2); // Delete the 3rd element System.out.println("\nremoveAll() After method list2 Number of elements in the collection:" + list2.size()); System.out.println("list2 The elements in the collection are as follows:"); Iterator it1 = list2.iterator(); while (it1.hasNext()) { System.out.print(it1.next() + ","); } list1.removeAll(list2); System.out.println("\nremoveAll() After method list1 Number of elements in the collection:" + list1.size()); System.out.println("list1 The elements in the collection are as follows:"); Iterator it2 = list1.iterator(); while (it2.hasNext()) { System.out.print(it2.next() + ","); } }
The retainAll() method is the opposite of the removeAll() method, that is, the same elements in the two collections are retained and all other elements are deleted.
List
List implements the Collection interface, which mainly has two common implementation classes: ArrayList class and LinkedList class
List<> list=new ArrayList<>(); List<> list=new LinkedList<>();
package com.list; import java.util.List; import java.util.ArrayList; import java.util.Iterator; public class Gather { /** * @param args */ public static void main(String[] args) { // Method stub automatically generated by TODO //Instantiate List collection List<String> list=new ArrayList<>(); list.add("a"); list.add("b"); list.add("c"); int i=(int) (Math.random()*list.size()); System.out.println(list.get(i)); list.remove(2); Iterator it1=list.iterator(); while(it1.hasNext()) { String str=(String)it1.next(); System.out.println(str); } } }
Common methods of ArrayList class
Method name Description
- E get(int index) gets the element with the specified index position in the collection. E is the data type of the element in the collection
- int index(Object o) returns the index of the specified element for the first time in the collection. If the collection does not contain the element, - 1 is returned
- int lastIndexOf(Object o) returns the index of the last occurrence of the specified element in the collection. If the collection does not contain the
Element, returns - 1 - E set(int index, Eelement) modifies the element with the specified index position in this set to the object specified by the element parameter. This method returns the original element of the specified index location in this collection
- List sublist (int fromindex, int tolndex) returns a new set containing all elements between fromindex and tolndex index. Contains the element at fromindex, not the element at tolndex index
package com.list; import java.util.ArrayList; import java.util.Iterator; import java.util.List; public class Test { public static void main(String[] args) { // Method stub automatically generated by TODO Product pd1=new Product(4,"wood",10); Product pd2=new Product(5,"water",11); Product pd3=new Product(6,"fire",12); List list=new ArrayList<>();//The object creation order table of class does not need to indicate the element type list.add(pd1); list.add(pd2); list.add(pd3); for(int i=0;i<list.size();i++) { Product product=(Product)list.get(i);//Cast Product type /*The obtained value is the Object class, so you need to convert the value to the Product class*/ System.out.println(product); } List list1=new ArrayList(); list1.add("one"); list1.add("|"); list1.add("two"); list1.add("|"); list1.add("three"); list1.add("|"); list1.add("Four"); System.out.println(list1.size()); Iterator it1=list1.iterator(); while(it1.hasNext()) { String str=(String)it1.next(); System.out.println(str); } System.out.println(list1.indexOf("|")); System.out.println(list1.lastIndexOf("|")); List list2=new ArrayList(); list2.add("one"); list2.add("two"); list2.add("three"); list2.add("four"); list2.add("five"); list2.add("six"); list2.add("seven"); System.out.println(list2.size()); Iterator it2=list2.iterator(); while(it2.hasNext()) { String str=(String)it2.next(); System.out.println(str); } List sublist=new ArrayList(); sublist=list2.subList(2, 5); System.out.println(sublist.size()); Iterator it3=sublist.iterator(); while(it3.hasNext()) { String str=(String)it3.next(); System.out.println(str); } } }
The LinkedList class uses a linked list structure to store objects. The advantage of this structure is that it is easy to insert or delete elements into the collection. When you need to frequently insert and delete elements into the collection, LinkedList is more effective than ArrayList
LinkList Methods in classes Method name explain void addFirst(E e) Adds the specified element to the beginning of this collection void addLast(E e) Adds the specified element to the end of this collection E getFirst() Returns the first element of this collection E getLast() Returns the last element of this collection E removeFirst() Delete the first element in this collection E removeLast() Delete the last element in this collection
LinkedList is a generic type in Java, which is used to specify the data type of elements in the collection. For example, if the element type specified here is String, elements of non String type cannot be added to the collection.
package com.list; import java.util.LinkedList; import java.util.List; public class Test1 { public static void main(String[] args) { // Method stub automatically generated by TODO LinkedList<String> products=new LinkedList<String> (); String p1=new String("Hexagon"); String p2=new String("10A"); String p3=new String("Tape measure"); String p4=new String("Template"); products.add(p1); products.add(p2); products.add(p3); products.add(p4); String p5=new String("Cabinet"); products.addLast(p5); for(int i=0;i<products.size();i++) { System.out.println(products.get(i)); } System.out.println(products.getFirst()); System.out.println(products.getLast()); products.removeLast(); for(int i=0;i<products.size();i++) { System.out.println(products.get(i)); } } }
The difference between ArrayList class and LinkedList class
- ArrayList is based on the implementation of dynamic array data structure, and the speed of accessing elements is better than LinkedList. LinkedList is an implementation based on linked list data structure, which occupies a large memory space, but it is better than ArrayList when inserting or deleting data in batch.
- For the requirement of fast access to objects, the implementation efficiency using ArrayList will be better. When you need to frequently insert and delete elements into the collection, LinkedList is more effective than ArrayList
HashSet
HashSet has the following characteristics:
The arrangement order of elements cannot be guaranteed. The order may be different from the addition order, and the order may also change.
Hashsets are not synchronized. If multiple threads access or modify a HashSet at the same time, they must be synchronized through code.
Collection element value can be null.
When an element is stored in the HashSet set, the HashSet will call the hashCode() method of the object to obtain the hashCode value of the object, and then determine the storage location of the object in the HashSet according to the hashCode value. If two elements are compared through the equals() method and the returned result is true, but their hashcodes are not equal, the HashSet will store them in different locations and can still be added successfully.
That is, if the hashCode values of two objects are equal and the result returned by the equals() method is true, the HashSet set considers the two elements equal.
HashSet hs = new HashSet(); // Call the parameterless constructor to create a HashSet object HashSet<String> hss = new HashSet<String>(); // Create a generic HashSet collection object
package com.list; import java.util.HashSet; import java.util.Iterator; public class Test2 { public static void main(String[] args) { // Method stub automatically generated by TODO HashSet<String> cour=new HashSet<String>(); String s1=new String("JAVA"); String s2=new String("Python"); String s3=new String("C++"); String s4=new String("GO"); cour.add(s1); cour.add(s2); cour.add(s3); cour.add(s4); Iterator it=cour.iterator(); while(it.hasNext()) { System.out.println((String)it.next()); } System.out.println(cour.size()); } }
First, a Set collection is created using the construction method of HashSet class, then four String objects are created and stored in the Set collection. Use the iterator() method in the HashSet class to obtain an Iterator object, call its hasNext() method to traverse the collection elements, and then cast the elements read with the next() method to String type. Finally, call the size() method in the HashSet class to get the number of collection elements. * *
If two identical elements are added to the Set collection, the later added element will overwrite the previously added element, that is, the same element will not appear in the Set collection.
**
TreeSet class
TreeSet class implements both Set interface and SortedSet interface. SortedSet interface is a sub interface of Set interface, which can realize natural sorting (ascending) of sets
TreeSet can only sort class objects that implement the Comparable interface
Implement the comparison method of Comparable interface class objects
Class comparison method
Wrapper classes (BigDecimal, Biglnteger, Byte, Double
Float, Integer, Long, and Short) are compared by number size
Character compares the numeric size of the Unicode value of a character
String compares the numeric size of the Unicode value of the character in the string
Common methods of TreeSet class
Method name Description
E first() returns the first element in this collection. Where e represents the data type of the element in the collection
E last() returns the last element in this collection
E poolFirst() gets and removes the first element in this collection
E poolLast() gets and removes the last element in this collection
SortedSet subSet(E fromElement,E toElement) returns a new set, which contains the fromElement object and toElement in the original set
All objects between objects. Contains fromElement objects, not toElement objects
Sortedset headset < e toElement > returns a new set. The new set contains all objects before the toElement object in the original set. It does not contain the toElement object
SortedSet tailSet(E fromElement) returns a new set containing all objects after the fromElement object in the original set
TreeSet is sorted naturally and in ascending order. This is done by default. It is already done when creating the instantiated object of TreeSet. No manual operation is required
When using natural sorting, only objects of the same data type can be added to the TreeSet collection, otherwise ClassCastException will be thrown. If an object of Double type is added to the TreeSet collection, only Double objects can be added later, and no other types of objects can be added
package com.list; import java.util.Iterator; import java.util.Scanner; import java.util.SortedSet; import java.util.TreeSet; public class Test3 { public static void main(String[] args) { // Method stub automatically generated by TODO TreeSet<Double>scores=new TreeSet<Double>(); Scanner input =new Scanner(System.in); for(int i=0;i<5;i++) { System.out.println("The first"+i+"A good score:"); double score=input.nextDouble(); //Convert the student grade to Double type and add it to the TreeSet collection scores.add(Double.valueOf(score)); } Iterator<Double>it=scores.iterator(); System.out.println("Student achievement:"); while(it.hasNext()) { System.out.println(it.next()); } System.out.println("query"); double search=input.nextDouble(); if(scores.contains(search)) { System.out.println(search+"existence"); }else { System.out.println(search+"non-existent"); } SortedSet<Double> score1=scores.headSet(60.0);//Excluding 60.0 System.out.println("\n Failing grades:"); for(int i=0;i<score1.toArray().length;i++) { System.out.println(score1.toArray()[i]+"\t"); } SortedSet<Double>score2=scores.tailSet(90.0);//Including 90.0 System.out.println("\n Outstanding achievements:"); for(int i=0;i<score2.toArray().length;i++) { System.out.println(score2.toArray()[i]+"\t"); } SortedSet<Double>score3=scores.subSet(60.0, 100.0);//Excluding 100.0 System.out.println("\n From 60-100 Between the results are"); for(int i=0;i<score3.toArray().length;i++) { System.out.println(scores.toArray()[i]+"\t"); } } }
Map
Map is a set of key value pairs. Each element in the map set contains a key object and a value object. Used to save data with mapping relationship.
Two sets of values are saved in the Map set. One set of values is used to save the key in the Map, and the other set of values is used to save the value in the Map. Both key and value can be data of any reference type. The key of Map cannot be repeated, but the value can be repeated
There is a one-way one-to-one relationship between key and value in Map, that is, the unique and determined value can always be found through the specified key. When retrieving data from the Map, you can retrieve the corresponding value as long as you give the specified key.
The Map interface mainly has two implementation classes: HashMap class and TreeMap class. Among them, HashMap class accesses key objects according to hash algorithm, while TreeMap class can sort key objects.
Map Common methods of interface Method name explain void clear() Delete the Map All in object key-value yes. boolean containsKey(Object key) query Map Contains the specified key,Return if included true. boolean containsValue(Object value) query Map Does the include one or more value,Return if included true. V get(Object key) return Map The value corresponding to the specified key object in the collection. V Represents the data type of the value V put(K key, V value) towards Map Add key to collection-Value pair, if current Map There is already one in the key Equal key-value Yes, it's new key-value Yes, it will overwrite the original key-value yes. void putAll(Map m) Will specify Map Medium key-value Copy to this Map Yes. V remove(Object key) from Map Delete from collection key Corresponding key-Value pair, return key Corresponding value,If key Does not exist, returns null boolean remove(Object key, Object value) This is Java 8 Add a new method and delete the specified method key,value Corresponding key-value yes. If from this Map The was successfully deleted from key-value Yes, the method returns true,Otherwise return false. Set entrySet() return Map All keys in the collection-Value pair Set Collection, this Set The data type of the element in the collection is Map.Entry Set keySet() return Map Of all key objects in the collection Set aggregate boolean isEmpty() Query this Map Is it empty (i.e. does not contain any information) key-value Yes), if it is blank, return true. int size() Return to this Map in key-value Number of pairs Collection values() Return to this Map All in value Composed Collection
foreach traversal object
for (Object obj : objs) {
}
Traverse Map
1) Use entries in the for loop to traverse the Map (the most common and commonly used).
public static void main(String[] args) { Map<String, String> map = new HashMap<String, String>(); map.put("Java Getting Started tutorial", "http://c.biancheng.net/java/"); map.put("C Introduction to language", "http://c.biancheng.net/c/"); for (Map.Entry<String, String> entry : map.entrySet()) { String mapKey = entry.getKey(); String mapValue = entry.getValue(); System.out.println(mapKey + ": " + mapValue); } }
2) Use the for each loop to traverse keys or values, which is generally applicable when only the keys or values in the Map are required. The performance is better than entrySet.
Map<String, String> map = new HashMap<String, String>(); map.put("Java Getting Started tutorial", "http://c.biancheng.net/java/"); map.put("C Introduction to language", "http://c.biancheng.net/c/"); // Print key set for (String key : map.keySet()) { System.out.println(key); } // Print value set for (String value : map.values()) { System.out.println(value); }
3) Traversal with Iterator
Map<String, String> map = new HashMap<String, String>(); map.put("Java Getting Started tutorial", "http://c.biancheng.net/java/"); map.put("C Introduction to language", "http://c.biancheng.net/c/"); Iterator<Entry<String, String>> entries = map.entrySet().iterator(); while (entries.hasNext()) { Entry<String, String> entry = entries.next(); String key = entry.getKey(); String value = entry.getValue(); System.out.println(key + ":" + value); }
4) Traversal through key value finding is inefficient because it is a time-consuming operation.
for(String key : map.keySet()){ String value = map.get(key); System.out.println(key+":"+value); }
Collections operation
sort
Collections provides the following methods for sorting List collection elements. Void reverse (List): the specified List
The collection elements are sorted in reverse. Void shuffle (List): shuffle the List collection elements randomly
Methods the "shuffle" action was simulated. Void sort (List): sorts the elements of the specified List set in ascending order according to the natural order of the elements.
Void sort (List, Comparator C): sort the List according to the order generated by the specified Comparator
Sort the collection elements. Void swap (List, int i, int j): the elements at I and j in the List collection will be specified
Exchange elements at. Void rotate (list, int distance): when distance is a positive number, list
Move the "whole" of the last distance element of the set to the front; When distance is negative, the first distance of the list set is
Move element "whole" to the back. This method does not change the length of the collection.
Find and replace operations
Collections also provides the following common methods for finding and replacing collection elements.
Int binarysearch (List, object key): use the binary search method to search the specified List set to obtain the index of the specified object in the List set. If you want this method to work properly, you must ensure that the elements in the List are already in an orderly state.
Object max(Collection coll): returns the largest element in a given collection according to the natural order of elements.
Object max(Collection coll, Comparator comp): returns the largest element in a given collection according to the order specified by the Comparator.
Object min(Collection coll): returns the smallest element in a given collection according to the natural order of elements.
Object min(Collection coll, Comparator comp): returns the smallest element in a given collection according to the order specified by the Comparator.
Void fill (List, object obj): replaces all elements in the specified List collection with the specified element obj.
int frequency(Collection c, Object o): returns the number of occurrences of the specified element in the specified collection.
int indexOfSubList(List source, List target): returns the location index of the child List object in the parent List object for the first time; If no such child List appears in the parent List, - 1 is returned.
int lastIndexOfSubList(List source, List target): returns the location index of the last occurrence of the child List object in the parent List object; If such a child List does not appear in the parent List, - 1 is returned.
Boolean replaceall (List, object oldVal, object newVal): replace all the old values oldVal of the List object with a new value newVal.
copy
The copy() static method of the Collections class is used to copy all elements in the specified collection to another collection. After the copy() method is executed, the index of each copied element in the target collection will be equal to the index of that element in the source collection.
copy() The syntax format of the method is as follows: void copy(List <? super T> dest,List<? extends T> src)
Where dest represents the target collection object and src represents the source collection object.
Immutable set
Set set = Set.of("Java", "Kotlin", "Go", "Swift"); List list = List.of(34, -25, 67, 231); Map map = Map.of("chinese", 89, "mathematics", 82, "English", 92); Map map2 = Map.ofEntries(Map.entry("chinese", 89), Map.entry("mathematics", 82), Map.entry("English", 92));
generic paradigm
package com.fanxing; public class Test<T> { private T over; private T getover() { return over; } private void setover(T over) { this.over=over; } public static void main(String[] args) { Test<Boolean>o1=new Test<Boolean>();//Instantiate a Boolean object Test<Float>o2=new Test<Float>(); o1.setover(true); o2.setover(12.1f); System.out.println((o1.getover() instanceof Boolean)?"yes":"no"); System.out.println((o2.getover() instanceof Float)?"yes":"no"); } }
When defining a generic class, the general type name is expressed in T and the container element is expressed in E
Multiple generic definitions
Type < T1, T2 >
Generic array definition
package com.fanxing; public class Test1 <T>{ private T[] array; public void Set(T[] array) { this.array=array; } public T[] Get() { return array; } public static void main(String[] args) { // Method stub automatically generated by TODO Test1<String>test=new Test1<String>(); String[] array=new String[] {"1","2","3","4"}; test.Set(array); for(int i=0;i<array.length;i++) { System.out.println(test.Get()[i]); } } }
You cannot use generic classes to build arrays
Generic collection
Use K and V to represent the key value in the container and the specific value corresponding to the key value.
For example: ArrayList, HashMap < K, V > and HashSet
Genericity is used directly in the main method without defining generic classes
package com.fanxing; import java.util.HashMap; import java.util.Map; public class Test2<K,V> { public Map<K,V>map=new HashMap<K,V>(); public void put(K k,V v) { map.put(k, v); } public V get(K k) { return map.get(k); } public static void main(String[] args) { // Method stub automatically generated by TODO Test2<Integer,String>test=new Test2<Integer,String>(); for(int i=0;i<5;i++) { test.put(i, "I'm a member of the collection"); } for(int i=0;i<test.map.size();i++) { System.out.println(test.map.get(i)+i); } } }
After an Object is "dropped" into the collection, the collection will "forget" the data type of the Object. When the Object is retrieved again, the compiled type of the Object becomes the Object type
A generic type is essentially a "type parameter" that provides a type, that is, a parameterized type.
package com.list; import java.util.ArrayList; import java.util.HashMap; public class Test6 { public static void main(String[] args) { // Method stub automatically generated by TODO Book book1=new Book(1, "Three hundred Tang Poems", 8); Book book2=new Book(2, "Little star", 12); Book book3=new Book(3, "Complete collection of Idioms", 22); HashMap<Integer,Book>books=new HashMap<Integer,Book>(); books.put(1001, book1); books.put(1002, book2); books.put(1003, book3); for(Integer id:books.keySet()) { System.out.print(id + "-"); System.out.println( books.get(id));// No type conversion is required } ArrayList<Book>booklist=new ArrayList(); booklist.add(book1); booklist.add(book2); booklist.add(book3); for(int i=0;i<booklist.size();i++) { System.out.println( booklist.get(i));// No type conversion is required here } } }
Generic class
In addition to defining generic collections, you can also directly qualify the type parameters of generic classes. The syntax format is as follows:
public class class_name<data_type1,data_type2,...>{}
Where, class_name indicates the name of the class, data_ type1 and so on represent type parameters. Java generics support declaring more than one type parameter. You only need to separate the types with commas.
Generic classes are generally used when the type of attribute in the class is uncertain. When declaring a property, use the following statement:
private data_type1 property_name1; private data_type2 property_name2;
package com.list; public class Test14 { public static void main(String[] args) { // Method stub automatically generated by TODO Stu<String,Integer,Character>stu=new Stu<String,Integer,Character>("Zhang Xiaoling", 28, 'female'); String name=stu.getName(); Integer age=stu.getAge(); Character sex=stu.getSex(); System.out.println("Student Name:" + name + ",Age:" + age + ",Gender:" + sex); } }
generic method
The syntax format for defining generic methods is as follows:
[Access modifier] [static] [final] <Type parameter list> Return value type method name([Formal parameter list])
For example:
public static <T> List find(Class<T> cs,int userId){}
1. Restrict the available types of generics
class name
anyClass refers to an interface or class. After using the generic restriction, the type of the generic class must implement or inherit the anyClass interface or class. Regardless of whether anyClass is an interface or a class, the extends keyword must be used when making generic restrictions.
// Generic types that restrict ListClass must implement the List interface public class ListClass<T extends List> { public static void main(String[] args) { // Instantiate the generic class ListClass using ArrayList. It is correct ListClass<ArrayList> lc1 = new ListClass<ArrayList>(); // Instantiate the generic class LlstClass using LinkedList. It is correct ListClass<LinkedList> lc2 = new ListClass<LinkedList>(); // Instantiating ListClass, a generic class using HashMap, is an error because HasMap does not implement the List interface // ListClass<HashMap> lc3=new ListClass<HashMap>(); } }
2. Use type wildcards
The syntax format for using generic type wildcards is as follows:
Generic class name<? extends List>a = null;
Among them, "<? Extensions list >" as a whole indicates that the type is unknown. When generic objects need to be used, they can be instantiated separately.
A<? extends List>a = null; a = new A<ArrayList> (); // correct b = new A<LinkedList> (); // correct c = new A<HashMap> (); // error
In the above code, because the HashMap class does not implement the List interface, an error will be reported during compilation
3. Inherit generic classes and implement generic interfaces
Classes and interfaces defined as generics can also be inherited and implemented. For example, the following example code demonstrates how to inherit generic classes.
public class FatherClass{} public class SonClass<T1,T2,T3> extents
FatherClass{}
interface interface1{}
interface SubClass<T1,T2,T3> implements
Interface1{}
enumeration
Enumeration is a collection of named integer constants. It is used to declare a set of constants with identifiers. enum keyword must be used when declaring enumeration, and then define the name, accessibility, basic type and member of enumeration. The syntax of enumeration declaration is as follows:
enum-modifiers enum enumname:enum-base { enum-body, }
Among them, enum modifiers indicates that the modifiers of enumeration mainly include public, private and internal; enumname indicates the declared enumeration name; Enum base represents the base type; Enum body represents the member of an enumeration. It is a named constant of an enumeration type.
Any two enumeration members cannot have the same name, and its constant value must be within the range of the underlying type of the enumeration. Multiple enumeration members are separated by commas.
If the enumeration of the underlying type is not explicitly declared, it means that its corresponding underlying type is int.
Enumeration members are modified by final, public and static by default. When using enumeration type members, you can call the members directly with the enumeration name.
Enum Common methods of class Method name describe values() Returns all members of an enumerated type as an array valueOf() Converts a normal string to an enumerated instance compareTo() Compares the order in which two enumeration members are defined ordinal() Gets the index location of the enumeration member
package com.meiju; public class Test2 { enum C2{ //Enumeration members CA("enumeration A"), CB("enumeration B"), CC("enumeration C"), CD(3); // You must add a semicolon after the last member of the enumeration instance, and you must define the enumeration instance first. private int i=4; private String ds; private C2() { } private C2(String ds) { this.ds=ds; } private C2(int i) { this.i=i+i; } @SuppressWarnings("unused") private String getds() { return this.ds; } @SuppressWarnings("unused") private int geti() { return this.i; } } public static void main(String[] args) { // Method stub automatically generated by TODO for(int i=0;i<C2.values().length;i++) { System.out.println(C2.values()[i]+C2.values()[i].getds()); } } }
Add method for enumeration
Java provides some built-in methods for enumeration types, and enumeration constants can also have their own methods. At this time, note that you must add a semicolon after the last member of the enumeration instance, and you must first define the enumeration instance.
package com.meiju; interface d{ String getstr(); int getid(); } enum Eum implements d{ CA{ public String getstr() { return ("CA"); } public int getid() { return i; } }, CB{ public String getstr() { return ("CB"); } public int getid() { return i; } }, CC{ public String getstr() { return ("CC"); } public int getid() { return i; } }, CD{ public String getstr() { return ("CD"); } public int getid() { return i; } }; private static int i=5; } public class Test3 { public static void main(String[] args) { // Method stub automatically generated by TODO for(int i=0;i<Eum.values().length;i++) { System.out.println(Eum.values()[i].getid()+Eum.values()[i].getstr()); } } }
Reflection mechanism
-
Compile time refers to the process of giving the source code to the compiler to compile into a computer executable file. In Java, that is, the process of compiling java code into class files. The compilation period only does some translation functions, does not run the code in memory, but only operates the code as text, such as checking errors.
-
Run time is to give the compiled file to the computer for execution until the program runs. The so-called runtime puts the code in the disk into memory for execution.
-
Reflection mechanism means that a program can obtain its own information at run time. In Java, as long as the name of the class is given, all the information of the class can be obtained through the reflection mechanism
-
First, get the class type object corresponding to each bytecode file (. Class)
All Java classes inherit the Object Class. A getClass() method is defined in the Object Class, which returns the same Object of type Class. For example, the following example code:
Class labelCls = label1.getClass(); // label1 is an object of JLabel class
Advantages and disadvantages of java reflection mechanism: it can dynamically obtain class instances at runtime, which greatly improves the flexibility and scalability of the system. With Java
The combination of dynamic compilation can realize incomparably powerful functions. For Java
This language that compiles first and then runs allows us to easily create flexible code. These codes can be matched when running without linking the source code between components, making it easier to realize object-oriented.
Disadvantages: Reflection consumes certain system resources. Therefore, if you do not need to dynamically create an object, you do not need to use reflection;
When calling a method by reflection, you can ignore the permission check and obtain the private methods and properties of this class. Therefore, it may destroy the encapsulation of the class and lead to security problems.
annotation
Annotation does not change the running results of the program, nor does it affect the performance of the program. Some annotations can prompt or warn users at compile time, and some annotations can read and write section code file information at run time.
@Override annotation
@The Override annotation is used to specify method overrides. It can only modify methods and can only be used for method overrides. It cannot modify other elements. It can force a subclass to Override the parent method or the method that implements the interface.
@Deprecated
@Deprecated can be used to annotate classes, interfaces, member methods and member variables, and to indicate that an element (class, method, etc.) is obsolete. The compiler warns when other programs use outdated elements.
@SuppressWarnings: suppresses compiler warnings
@The SuppressWarnings annotation instructs the program element modified by the annotation (and all child elements in the program element) to suppress the specified compiler warning and will always act on all child elements of the program element. For example, if you use @ SuppressWarnings to modify a class to suppress a compiler warning and modify a method in the class to suppress another compiler warning, the method will suppress both compiler warnings.
@The SuppressWarnings annotation is mainly used to cancel the blocking of the columns on the left side of the code by some warnings generated by the compiler. Sometimes this will block the breakpoints we hit during breakpoint debugging. As shown in the figure below.
If a collection without generic restrictions is used in a program, it will cause compiler warnings. To avoid such compiler warnings, you can use the @ SuppressWarnings annotation to eliminate these warnings.
Annotations can be used in the following three ways:
Suppress single type warnings: @ SuppressWarnings("unchecked")
Suppress multiple types of warnings: @ SuppressWarnings("unchecked", "rawtypes")
Suppress all types of warnings: @ SuppressWarnings("unchecked")
public class HelloWorld { public static void main(String[] args) { // Pass variable parameters, which are generic collections display(10, 20, 30); // Pass a variable parameter, which is a non generic collection display("10", 20, 30); // Without @ SafeVarargs, there will be a compilation warning } @SafeVarargs public static <T> void display(T... array) { for (T arg : array) { System.out.println(arg.getClass().getName() + ": " + arg); } } }
@FunctionalInterface
If there is only one abstract method in an interface (it can contain multiple default methods or multiple static methods), the interface is a functional interface@ Functional interface is used to specify that an interface must be a functional interface, so @ functional interface can only modify interfaces, not other program elements.
@Documented
@Documented is a tag annotation with no member variables. Annotation classes decorated with @ documented annotations will be extracted into documents by JavaDoc tools. By default, JavaDoc does not include annotations, but if @ documented is specified when declaring annotations, it will be processed by tools such as JavaDoc, so the annotation type information will be included in the generated help document.
@Target
@The Target annotation is used to specify the scope of use of an annotation, that is, where the annotation modified by @ Target can be used@ The Target annotation has a member variable (value) to set the applicable Target. Value is an array of java.lang.annotation.ElementType enumeration types. The following table shows the commonly used enumeration constants of ElementType.
Name Description
CONSTRUCTOR is used to construct methods
FIELD is used for member variables (including enumeration constants)
LOCAL_VARIABLE is used for local variables
METHOD for METHOD
PACKAGE for packages
PARAMETER is used for type parameters (new in JDK 1.8)
TYPE is used for class, interface (including annotation TYPE) or enum declarations
@Retention
@Retention is used to describe the life cycle of an annotation, that is, the length of time the annotation is retained@ The member variable (value) in the retention annotation is used to set the retention policy. Value is the java.lang.annotation.RetentionPolicy enumeration type. RetentionPolicy has three enumeration constants, as shown below.
SOURCE: valid in SOURCE file (i.e. SOURCE file retention)
class: valid in class file (i.e. class reserved)
RUNTIME: valid at RUNTIME (i.e. reserved at RUNTIME)
@Inherited
@Inherited is a tag annotation that specifies that the annotation can be inherited. A Class class annotated with @ inherited indicates that this annotation can be used for subclasses of this Class. That is, if a Class uses an annotation modified by @ inherited, its subclass will automatically have the annotation.
@Repeatable
@The Repeatable annotation is a new addition to Java 8. It allows repeated annotations in the same program element. When the same annotation needs to be used multiple times, it often needs the help of @ Repeatable annotation. Before the Java 8 version, there can only be one annotation of the same type in front of the same program element. If you need to use multiple annotations of the same type in front of the same element, you must use the annotation "container".
I/O
System.in is an object of the InputStream class, so the System.in.read() method of the above code actually accesses the read() method defined by the InputStream class. This method can read one or more characters from the keyboard. For System.out, the output stream is mainly used to output the specified content to the console.
System.out and System.error are objects of the PrintStream class. Because PrintStream is an output stream derived from OutputStream, it also executes a low-level write() method. Therefore, in addition to the print() and println() methods, system. Out can also call the write() method to implement console output.
If you want to realize encoding conversion, you can use the getBytes(String charset) method in the String class. This method can set the specified encoding. The format of this method is as follows:
public byte[] getBytes(String charset);
File class
The File class provides the following three formal construction methods.
File(String path): if path is an actual path, the file object represents a directory; If path is a file name, the file object represents a file.
File(String path, String name): path is the path name and name is the file name.
File(File dir, String name): dir is the path object and name is the file name.
When used in Java, the path should be written as D:/javaspace/hello.java or D:\javaspace\hello.java
Be sure to use the File.separator to represent the separator when operating the file. To create a file, you need to call the createNewFile() method, and to delete a file, you need to call the delete() method. Whether you create or delete a file, you usually call the exists() method to determine whether the file exists.
To create a directory, you need to call the mkdir() method
Traverse directory
By traversing the directory, you can find files in the specified directory or display a list of all files. The list() method of File class provides the function of traversing the directory. This method has the following two overloaded forms.
- String[] list()
This method returns a string array consisting of the names of all files and subdirectories in the File object directory. If the File object called is not a directory, null is returned.
Tip: the array returned by the list() method contains only the file name, not the path. However, there is no guarantee that the same strings in the resulting array will appear in a specific order, especially in alphabetical order.
2. String[] list(FilenameFilter filter)
The function of this method is the same as that of the list() method, except that the returned array only contains files and directories that meet the filter filter. If the filter is null, all names are accepted.
The character array returned by the list() method contains only the File name, so in order to obtain the File type and size, you must first convert it to a File object and then call its method.
filter
First, you need to create a file filter, which must implement the java.io.FilenameFilter interface and specify the allowed file types in the accept() method.
The following is the filter implementation code that allows SYS, TXT and BAK format files:
public class ImageFilter implements FilenameFilter {
//Implement the FilenameFilter interface
@Override
public boolean accept(File dir, String name) {
//Specify the allowed file types
return name.endsWith(".sys") || name.endsWith(".txt") || name.endsWith(".bak");
} }
The filter name created by the above code is ImageFilter. Next, you only need to pass the name to the list() method to filter the file. The modified list() method is shown below. Other codes are the same as those in example 4 and will not be repeated here.
String fileList[] = f.list(new ImageFilter());
Byte input stream
The objects of InputStream class and its subclasses represent byte input streams. The common subclasses of InputStream class are as follows.
ByteArrayInputStream class: converts a byte array into a byte input stream from which bytes are read.
FileInputStream class: reads data from a file.
PipedInputStream class: connect to a PipedInputStream (pipeline output stream).
SequenceInputStream class: concatenates multiple byte input streams into one byte input stream.
ObjectInputStream class: deserializing objects
Byte output stream
The objects of the OutputStream class and its subclasses represent a byte output stream. Common subclasses of the OutputStream class are as follows.
ByteArrayOutputStream class: writes data to a byte array in a memory buffer.
FileOutputStream class: write data to a file.
PipedOutputStream class: connect to a PipedlntputStream (pipe input stream).
ObjectOutputStream class: serializes objects.
Byte array input stream
ByteArrayInputStream class can read data from the byte array in memory. This class has the following two overloaded forms of construction methods.
ByteArrayInputStream(byte[] buf): create a byte array input stream. The data source of byte array type is specified by the parameter buf.
Bytearrayinputstream (byte [] buf, int offset, int length): create a byte array input stream, where parameter buf specifies the data source of byte array type, offset specifies the starting subscript position to start reading data in the array, and length specifies the number of elements to read.
Byte array output stream
ByteArrayOutputStream class can write data to the byte array in memory. The construction method of this class has the following two overloaded forms.
ByteArrayOutputStream(): creates a byte array output stream. The initial size of the output stream buffer is 32 bytes.
ByteArrayOutputStream(int size): create a byte array output stream. The initial capacity of the output stream buffer is specified by the parameter size.
In the ByteArrayOutputStream class, in addition to the common methods in the byte output stream described above, there are the following two methods.
intsize(): returns the current number of bytes in the buffer.
byte[] toByteArray(): returns the current content in the output stream in the form of byte array.
When creating the object of FileInputStream class, if the specified file is not found, a FileNotFoundException exception will be thrown, which must be caught or declared.
File input stream
The common construction methods of FileInputStream mainly include the following two overload forms.
FileInputStream(File file): create a FileInputStream by opening a connection to the actual file, which is specified by the file object file in the file system.
FileInputStream(String name): create a FileInputStream by opening a link to the actual file, which is specified by the pathname name in the file system.
File output stream
The FileOutputStream class inherits from the OutputStream class and overrides and implements all methods in the parent class. The object of FileOutputStream class represents a file byte output stream. You can write a byte or a batch of bytes to the stream. When creating the object of FileOutputStream class, if the specified file does not exist, create a new file; If the file already exists, the contents of the original file are cleared and rewritten.
The construction methods of FileOutputStream class mainly include the following four overload forms.
FileOutputStream(File file): creates a file output stream. The parameter file specifies the target file.
FileOutputStream(File file,boolean append): create a file output stream. The parameter file specifies the target file, and append specifies whether to add data to the end of the content of the target file. If it is true, it is added at the end; If false, the original content will be overwritten; The default value is false.
FileOutputStream(String name): create a file output stream. The parameter name specifies the file path information of the target file.
FileOutputStream(String name,boolean append): create a file output stream. The parameters name and append have the same meanings as above.
Note: use the constructor FileOutputStream(String name,boolean append) to create a file output stream object that appends data to the end of an existing file. The string name indicates the original file. If it is only for attaching data rather than rewriting any existing data, the value of the boolean type parameter append should be true.
The file output stream is described in the following four points:
When the target file is specified in the constructor of the FileOutputStream class, the target file may not exist.
The name of the target file can be arbitrary, such as D:\abc, D:\abc.de and D:\abc.de.fg. You can use tools such as Notepad to open and browse the contents of these files.
The directory where the target file is located must exist, otherwise a java.io.FileNotFoundException will be thrown.
The name of the destination file cannot be an existing directory. For example, if there is a Java folder under disk D, you cannot use Java as the file name, that is, you cannot use D:\Java, otherwise a java.io.FileNotFoundException exception will be thrown.
Character input stream
Common subclasses of Reader class are as follows.
CharArrayReader class: converts a character array into a character input stream from which characters are read.
StringReader class: converts a string into a character input stream and reads characters from it.
BufferedReader class: provides a read buffer for other character input streams.
PipedReader class: connect to a PipedWriter.
InputStreamReader class: converts byte input stream to character input stream, and character encoding can be specified.
Character output stream
Common subclasses of the Writer class are as follows.
CharArrayWriter class: writes data to a character array in a memory buffer.
StringWriter class: writes data to the string (StringBuffer) of the memory buffer.
BufferedWriter class: provides a write buffer for other character output streams.
PipedWriter class: connect to a PipedReader.
OutputStreamReader class: converts byte output stream to character output stream, and character encoding can be specified.
Character file input stream
FileReader (file): creates a new FileReader object given the file to read data. Where file represents the file from which to read data.
FileReader(String fileName): creates a new FileReader object given the file name from which to read data. Where fileName represents the name of the file from which data is to be read, and represents the full path of a file.
package com.in; import java.io.FileReader; import java.io.IOException; public class Test9 { public static void main(String[] args) { // Method stub automatically generated by TODO FileReader f=null; try { // Create FileReader object f=new FileReader("C:\\Test1.java"); int i=0; //read() reads a character and returns - 1 if it reaches the end of the input stream while((i=f.read())!=-1) { System.out.print((char) i); // Cast the read content to char type } }catch(Exception e) { e.printStackTrace(); }finally { try { f.close(); }catch(IOException e) { e.printStackTrace(); } } } }
Character file output stream
FileWriter, the construction method of this class has the following four overloaded forms.
FileWriter (file): construct a FileWriter object when a file object is specified. Where file represents the file object to write data to.
FileWriter (File, Boolean append): construct a FileWriter object when the File object is specified. If the value of append is true, the bytes will be written to the end of the File instead of the beginning of the File.
FileWriter(String fileName): construct a FileWriter object with a specified file name. Where fileName represents the file name to write characters, and represents the full path.
FileWriter(String fileName,boolean append): constructs a FileWriter object when the file name and the location of the file to be written are specified. Where append is a boolean value. If true, the data will be written to the end of the file instead of the beginning of the file.
package com.in; import java.io.FileWriter; import java.io.IOException; import java.util.Scanner; public class Test10 { public static void main(String[] args) { // Method stub automatically generated by TODO Scanner input =new Scanner(System.in); FileWriter fw=null; try { // Create FileWriter object fw=new FileWriter("C:\\book.txt"); for(int i=0;i<4;i++) { System.out.println("Please enter page" +(i+1)+ "String (s):"); String name=input.next();// Read input fw.write(name+"\r\n"); } System.out.println("Input completed!"); }catch(Exception e) { System.out.println(e.getMessage()); }finally { try { fw.close(); }catch(IOException e) { e.printStackTrace(); } } } }
Character buffer input stream
BufferedReader class is mainly used to assist other character input streams. It has a buffer, which can first read a batch of data to the memory buffer. The next read operation can directly obtain data from the buffer without reading data from the data source and character encoding conversion every time, which can improve the data reading efficiency.
The construction method of BufferedReader class has the following two overloaded forms.
1. BufferedReader(Reader in): create a BufferedReader to modify the character input stream specified by parameter in.
2. BufferedReader(Reader in,int size): create a BufferedReader to decorate the character input stream specified by the parameter in. The parameter size is used to specify the size of the buffer, in characters.
In addition to providing a buffer for the character input stream, BufferedReader also provides a readLine () method, which returns a string containing the contents of the line, but the string does not contain any terminator. If the end of the stream has been reached, it returns null. The readLine() method means that a line of text is read one at a time. When a line feed (\ n), carriage return (\ r) or carriage return is encountered, a line can be considered terminated by directly following the line feed flag.
package com.in; import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class Test11 { public static void main(String[] args) { // Method stub automatically generated by TODO FileReader fr=null; BufferedReader br=null; try { fr=new FileReader("C:\\book.txt"); br=new BufferedReader(fr); String readline=""; while((readline=br.readLine())!=null) { System.out.println(readline); } }catch(Exception e) { e.printStackTrace(); }finally { try { fr.close(); br.close(); }catch(IOException e) { e.printStackTrace(); } } } }
Character buffer output stream
BufferedWriter class is mainly used to assist other character output streams. It also has a buffer. You can write a batch of data to the buffer first. When the buffer is full, the data in the buffer will be written to the character output stream at one time. Its purpose is to improve the data writing efficiency.
The construction method of BufferedWriter class has the following two overloaded forms.
BufferedWriter(Writer out): create a BufferedWriter to decorate the character output stream specified by the parameter out.
BufferedWriter(Writer out,int size): create a BufferedWriter to decorate the character output stream specified by the parameter out. The parameter size is used to specify the size of the buffer, in characters.
In addition to providing a buffer for the character output stream, this class also provides a new method newLine(), which is used to write a line separator. The line separator string is defined by the system attribute line.separator and is not necessarily a single new line (\ n) character.
InputStreamReader is used to convert byte input stream to character input stream, and OutputStreamWriter is used to convert byte output stream to character output stream. Using the conversion stream can avoid garbled code to some extent, and can also specify the character set used for input and output.
Object oriented review again
Class is an object template that defines how to create instances. Therefore, class itself is a data type.
Instance is an object instance. Instance is an instance created according to class. You can create multiple instances. Each instance has the same type, but its properties may be different.
Define class
Through class, a group of data is collected on an object to realize data encapsulation.
Definition of Book class:
class Book {
public String name;
public String author;
public String isbn;
public double price;
}
Create instance
class is defined, but only the object template is defined. To create a real object instance according to the object template, you must use the new operator.
The new operator can create an instance. Then, we need to define a variable of reference type to point to the instance:
Person ming = new Person();
Person ming is the variable ming that defines the person type, and new Person() is to create the person instance.
Instance variables can be used as variables.field
ming.name = "Xiao Ming"; // Assign a value to the field name ming.age = 12; // Assign value to field age System.out.println(ming.name); // Access field name Person hong = new Person(); hong.name = "Xiao Hong"; hong.age = 15;
The two instance s have the name and age fields defined by the class, and each has an independent data, which does not interfere with each other.
A Java source file can contain multiple class definitions, but only one public class can be defined, and the public class name must be consistent with the file name. If you want to define multiple public classes, you must split them into multiple Java source files.
Variables pointing to instance are all reference variables
method
Modify the field with private and deny external access: class Person{
private String name;
private int age; }
Use method s to allow external code to modify field s indirectly
That is, get and set. External code can call methods setName() and setAge() to indirectly modify the private field, so that it can be judged in the set method
Modifier method return type method name(Method parameter list) { Several method statements; return Method return value; } Method return value passed return Statement. If there is no return value, the return type is set to void,Can be omitted return.
this variable
Inside the method, you can use an implicit variable this, which always points to the current instance. Therefore, you can access the fields of the current instance through this.field.
If there is no naming conflict, you can omit this. For example:
class Person { private String name; public String getName() { return name; // Equivalent to this.name } }
However, if the local variable and field have the same name, the local variable has higher priority, and this must be added:
class Person { private String name; public void setName(String name) { this.name = name; // The previous this is indispensable. If it is missing, it will become the local variable name } }
Method parameters
A method can contain 0 or any parameters. Method parameters are used to receive variable values passed to the method. When calling a method, they must be passed one by one in strict accordance with the definition of the parameters. For example:
class Person { ... public void setNameAndAge(String name, int age) { ... } }
Variable parameters
Variable parameters are defined by * * type... * * and are equivalent to array types:
class Group { private String[] names; public void setNames(String... names) { this.names = names; } }
setNames() above defines a variable parameter. When called, it can be written as follows:
Group g = new Group(); g.setNames("Xiao Ming", "Xiao Hong", "Xiao Jun"); // Pass in 3 strings g.setNames("Xiao Ming", "Xiao Hong"); // Pass in 2 strings g.setNames("Xiao Ming"); // Pass in 1 String g.setNames(); // 0 strings passed in
Variable parameters can be rewritten to String [] type:
class Group { private String[] names; public void setNames(String[] names) { this.names = names; } }
However, the caller needs to construct String [], which is troublesome. For example:
Group g = new Group(); g.setNames(new String[] {"Xiao Ming", "Xiao Hong", "Xiao Jun"}); // Pass in 1 String []
Parameter binding
The transfer of basic type parameters is the copy of caller values. Subsequent amendments made by both parties shall not affect each other. Do not change the source value.
The transfer of reference type parameters, the caller's variables, and the receiver's parameter variables point to the same object. The modification of this object by either party will affect the other party (because it points to the same object) and change the source value.
Construction method
When you create an instance, you actually initialize the instance by constructing a method
If a class does not define a constructor, the compiler will automatically generate a default constructor for us. It has no parameters and no execution statements, like this:
class Person {
public Person() {
} }
When the field is not initialized in the constructor, the field of reference type is null by default, the field of numeric type uses the default value, the default value of int type is 0, and the default value of boolean type is false:
You can also initialize fields directly:
class Person {
private String name = "Unamed";
private int age = 10; }
When creating an object instance, initialize it in the following order:
1. Initialize the field first, for example, int age = 10; Indicates that the field is initialized to 10, double salary; Indicates that the field is initialized to 0 by default, String name; Indicates that the reference type field is initialized to null by default;
2. The code that executes the construction method is initialized.
Therefore, since the code of the construction method runs later, the field value of new Person("Xiao Ming", 12) is finally determined by the code of the construction method
Multi construction method
Multiple construction methods can be defined. When called through the new operator, the compiler will automatically distinguish by the number, position and type of parameters of the construction method
One constructor can call other constructors to facilitate code reuse. The syntax for calling other constructor methods is this(...). Called according to the parameter form, number and position of different construction methods
class Person { private String name; private int age; public Person(String name, int age) { this.name = name; this.age = age; } public Person(String name) { this(name, 18); // Call another constructor Person(String, int) } public Person() { this("Unnamed"); // Call another constructor Person(String) } }
Method overloading
Methods with the same name but different parameters are called method Overload. Note: the return value types of method overloads are usually the same.
inherit
The child class automatically obtains all fields of the parent class. It is strictly prohibited to define fields with the same name as the parent class!
Inheritance tree
Notice that we didn't write extends when defining Person. In Java, if you do not explicitly write the extends class, the compiler will automatically add the extends Object. Therefore, any class, except Object, will inherit from a class. The following figure shows the inheritance tree of Person and Student:
┌───────────┐
│ Object
└───────────┘
▲
┌───────────┐
│ Person
└───────────┘
▲
┌───────────┐
│ Student
└───────────┘
A class has only one parent. Only Object is special. It has no parent class.
Inheritance has a feature that subclasses cannot access the private field or private method of the parent class. For example, the Student class cannot access the name and age fields of the Person class:
class Person { private String name; private int age; } class Student extends Person { public String hello() { return "Hello, " + name; // Compilation error: unable to access the name field } }
Fields decorated with protected can be accessed by subclasses:
class Person { protected String name; protected int age; } class Student extends Person { public String hello() { return "Hello, " + name; // OK! } }
Protected keyword can control the access rights of fields and methods within the inheritance tree. A protected field and method can be accessed by its subclasses and subclasses of subclasses
super
Super keyword indicates a parent class (superclass). When a subclass references a field of a parent class, you can use super.fieldName
class Student extends Person { public String hello() { return "Hello, " + super.name; } }
Inheriting person, having name, using super.name, or this.name, or name, the effect is the same. The compiler automatically navigates to the name field of the parent class
If the parent class does not have a default constructor, the child class must explicitly call super() and give parameters so that the compiler can locate an appropriate constructor of the parent class.
Subclasses do not inherit the constructor of any parent class. The default construction method of subclasses is automatically generated by the compiler, not inherited.
class Student extends Person { protected int score; public Student(String name, int age, int score) { super(name, age); // Call the constructor Person(String, int) of the parent class this.score = score; } }
Unified: this. Calls the instance of this class, and super calls the parent class
Block inheritance
Normally, as long as a class does not have a final modifier, any class can inherit from that class
Upward transformation
If the type of a reference variable is Student, it can point to an instance of Student type:
Student s = new Student();
If a variable of reference type is Person, it can point to an instance of Person type:
Person p = new Person();
Student is inherited from Person. A variable with reference type of Person points to an instance of student type
Person p = new Student();
Student inherits from Person, so it has all the functions of Person. If a variable of Person type points to an instance of student type and operates on it, there is no problem!
This assignment that safely changes a subclass type to a parent type is called upcasting. The subclass inherits the parent class, and the subclass has the public and protected functions and variables of the parent class; The reference variable of a parent class points to an instance of a child class:
Parent type reference name=new Subclass type();
The upward transformation is actually to safely change a subtype into a more abstract parent type
Downward transformation
The downward transformation is likely to fail. In case of failure, the Java virtual opportunity reports ClassCastException.
In order to avoid downward transformation errors, Java provides the instanceof operator, which can first judge whether an instance is of a certain type:
Person p = new Person();
System.out.println(p instanceof Person); // true
System.out.println(p instanceof Student); // false
Student s = new Student();
System.out.println(s instanceof Person); // true
System.out.println(s instanceof Student); // true
Student n = null;
System.out.println(n instanceof Student); // false
Instanceof actually determines whether the instance pointed to by a variable is a specified type or a subclass of this type. If a reference variable is null, the judgment of any instanceof is false.
Because the subclass has more functions than the parent class, more functions cannot be changed out of thin air
Person p = new Student(); if (p instanceof Student) { // Only by judging success can we transition downward: Student s = (Student) p; // It will succeed }
Distinguish between inheritance and composition
The has relationship should not use inheritance, but use combination, that is, students can hold a Book instance:
class Student extends Person {
protected Book book;
protected int score;
}
Therefore, inheritance is an is relationship and composition is an has relationship.
It means that there should be logical rationality between classes. Books cannot inherit students. Students can only define the members of books in the student class
polymorphic
In the inheritance relationship, if a subclass defines a method with exactly the same signature as the parent method, it is called Override.
class Person { public void run() { System.out.println("Person.run"); } } In subclass Student In, overwrite this run()method: class Student extends Person { @Override public void run() { System.out.println("Student.run"); } }
Java instance method calls are dynamic calls based on the actual type at runtime, rather than the declared type of variables.
public class Main { public static void main(String[] args) { Person p = new Student(); p.run(); // Should I print Person.run or Student.run? } } class Person { public void run() { System.out.println("Person.run"); } } class Student extends Person { @Override public void run() { System.out.println("Student.run"); } }
Print Student.run because p actually points to an instance of Student type.
Override Object method
All class es ultimately inherit from Object, and Object defines several important methods:
1. toString(): output instance as String;
2. equals(): judge whether two instance s are logically equal;
3. hashCode(): calculates the hash value of an instance.
Call super
In the overridden method of a subclass, if you want to call the overridden method of the parent class, you can call it through super.
class Person { protected String name; public String hello() { return "Hello, " + name; } } Student extends Person { @Override public String hello() { // Call the hello() method of the parent class: return super.hello() + "!"; } }
final
Inheritance allows subclasses to Override the methods of the parent class. If a parent class does not allow subclasses to Override one of its methods, you can mark the method as final. The method decorated with final cannot be overridden, which is applicable to both methods and classes.
If a class does not want any other classes to inherit from it, you can mark the class itself as final. Classes decorated with final cannot be inherited.
The instance field of a class can also be decorated with final. Fields decorated with final cannot be modified after initialization.
class Person { protected String name; public final String hello() { return "Hello, " + name; } } Student extends Person { // compile error: overwrite is not allowed @Override public String hello() { } }
An error will be reported if the final field is re assigned. You can initialize the final field in the constructor.
class Person { public final String name; public Person(String name) { this.name = name; } }
This method is more commonly used because it can ensure that the final field of an instance cannot be modified once it is created.
abstract class
Due to polymorphism, each subclass can override the methods of the parent class. If the methods of the parent class do not need to implement any functions, but only to define the method signature, so that the subclass can override it, then the methods of the parent class can be declared as abstract methods
abstract class Person { public abstract void run(); }
The class of an abstract method is an abstract class and cannot instantiate an abstract class. An abstract class can force its subclass to implement its defined abstract method. An abstract method is actually equivalent to defining a "specification".
Abstract oriented programming
Refer to the instance of a specific subclass through an abstract class type:
Person s = new Student();
Person t = new Teacher();
//Similarly, I don't care how the new subclass implements the run() method:
Person e = new Employee();
Try to refer to high-level types and avoid referring to actual subtypes, which is called abstract oriented programming.
1. The upper layer code only defines specifications (e.g. abstract class Person);
2. Business logic can be implemented without subclasses (normal compilation);
3. The specific business logic is implemented by different subclasses, and the caller does not care.
Interface
In abstract classes, abstract methods essentially define interface specifications: that is, specify the interface of high-level classes, so as to ensure that all subclasses have the same interface implementation
If an abstract class has no fields and all methods are abstract methods, rewrite the abstract class as interface: interface.
interface Person { void run(); String getName(); }
A pure abstract interface that is more abstract than an abstract class because it can't even have fields. Because all methods defined by the interface are public abstract by default, these two modifiers do not need to be written out
When a specific class implements an interface, it needs to use the implements keyword
A class can only inherit from another class, not from multiple classes. However, a class can implement multiple interface s
class Student implements Person, Hello { // Two interface s are implemented ... }
Interface inheritance
One interface can inherit from another interface. Interface inherits from interface and uses extensions, which is equivalent to extending the methods of the interface
The instantiated object can only be a specific subclass, but it is always referenced through the interface, because the interface is more abstract than the abstract class
List list = new ArrayList(); // Use the List interface to reference instances of specific subclasses Collection coll = list; // Transform upward to Collection interface Iterable it = coll; // Upward transformation to Iterable interface
default method
You can implement an interface without overriding methods
Static fields and static methods
The instance field has its own independent "space" in each instance, but the static field has only one shared "space", which will be shared by all instances.
class Person { public String name;// Define instance field number: public int age; // Define static field number: public static int number; }
Class name. Static field to access static object, Person.number
Static method
If you call a static method, you do not need an instance variable. You can call it through the class name,
Inside the static method, you cannot access this variable or instance fields. It can only access static fields.
Static field of interface
interface can have static fields, and the static fields must be of final type:
public interface Person { public static final int MALE = 1; public static final int FEMALE = 2; }
Because the field of interface can only be public static final, we can remove these modifiers. The above code can be abbreviated as:
public interface Person {
//The compiler will automatically add public statc final:
int MALE = 1;
int FEMALE = 2; }
package
The package has no parent-child relationship. Java.util and java.util.zip are different packages, and they have no inheritance relationship.
The class that does not define the package name uses the default package, which is very easy to cause name conflicts. Therefore, it is not recommended not to write the package name
Package scope
package hello;
import
First, write out the complete class name directly, for example: // Person.java package ming; public class Person { public void run() { mr.jun.Arrays arrays = new mr.jun.Arrays(); } }
The second way is to use import Statement, import Xiaojun's Arrays,Then write a simple class name: // Person.java package ming; // Import full class name: import mr.jun.Arrays; public class Person { public void run() { Arrays arrays = new Arrays(); } }
Writing import Can be used when*,Means to put all the items under this package class All imported (but excluding sub packages) class): // Person.java package ming; // Import all class es of mr.jun package: import mr.jun.*; public class Person { public void run() { Arrays arrays = new Arrays(); } }
In order to avoid name conflicts, we need to determine the unique package name. The recommended practice is to use inverted domain names to ensure uniqueness. For example, org.apache
The org.apache.commons.log com.liaoxuefeng.sample sub package can be named according to the function.
Be careful not to have the same name as the class in the java.lang package, that is, do not use these names for your own class: String System Runtime
Be careful not to have the same name as the common JDK class: java.util.list, java.text.format, java.math.biginteger
...
Scope
public
Classes and interface s defined as public can be accessed by any other class:
package abc;
public class Hello {
public void hi() {
}
}
The above Hello is public, so it can be accessed by other package classes:
package xyz;
class Main {
void foo() {
//Main can access Hello
Hello h = new Hello();
}
}
Fields and method s defined as public can be accessed by other classes, provided that you have the permission to access class first:
package abc;
public class Hello {
public void hi() {
}
}
The above hi() method is public and can be called by other classes. The premise is to access the Hello class first:
package xyz;
class Main {
void foo() {
Hello h = new Hello();
h.hi();
}
}
private
Fields and method s defined as private cannot be accessed by other classes:
package abc;
public class Hello {
//Cannot be called by other classes:
private void hi() {
}
public void hello() { this.hi(); }
}
In fact, to be exact, private access is limited to the inside of class,
protected
Protected acts on inheritance relationships. Fields and methods defined as protected can be accessed by subclasses, and subclasses of subclasses:
package abc;
public class Hello {
//protected method:
protected void hi() {
}
}
The above protected method can be accessed by inherited classes:
package xyz;
class Main extends Hello {
void foo() {
//You can access the protected method:
hi();
}
}
package
Finally, package scope refers to a class that allows access to the same package without public and private modifications, as well as fields and methods without public, protected and private modifications.
package abc;
//package permission class:
class Hello {
//package permission method:
void hi() {
}
}
local variable
Variables defined inside a method are called local variables. The scope of a local variable starts from the variable declaration to the end of the corresponding block. Method parameters are also local variables
Modifying a class with final prevents it from being inherited
Modifying method with final can prevent it from being overridden by subclasses
Modifying a field with final prevents it from being reassigned
If you are not sure whether public is required, you will not declare it as public, that is, expose as few external fields and methods as possible.
Defining a method as package permission is helpful for testing, because as long as the test class and the tested class are in the same package, the test code can access the package permission method of the tested class.
A. java file can only contain one public class, but can contain multiple non-public classes. If there is a public class, the file name must be the same as the name of the public class.
Inner class
Inner Class
If a class is defined inside another class, this class is Inner Class
class Outer { class Inner { // An Inner Class is defined } }
The Outer Class defined above is a common class, while the Inner Class is an Inner Class. The biggest difference between the Inner Class and the common class is that the instance of the Inner Class cannot exist alone and must be attached to an instance of the Outer Class
To instantiate a Inner, we must first create an instance of Outer, and then invoke the new of the Outer instance to create the Inner instance:
Outer.Inner inner = outer.new Inner();
Use Outer.this to access this instance. Therefore, instantiating an Inner Class cannot be separated from the Outer instance.
Compared with ordinary classes, Inner Class has an additional "privilege" in addition to referencing Outer instances, that is, it can modify the private field of Outer Class. Because the scope of Inner Class is within Outer Class, it can access the private field and method of Outer Class.
The Outer class is compiled as Outer.class, and the Inner class is compiled as Outer$Inner.class.
Anonymous Class
Define anonymous classes as follows:
Runnable r = new Runnable() {
//Implement the necessary abstract methods...};
void asyncHello() { Runnable r = new Runnable() { @Override public void run() { System.out.println("Hello, " + Outer.this.name); } }; new Thread(r).start(); }
Anonymous classes, like inner classes, can access the private fields and methods of outer classes
The Outer class is compiled as Outer.class, and the anonymous class is compiled as Outer .class. If there are multiple anonymous classes, the Java compiler will name each anonymous class Outer , Outer,, Outer in turn
Static Nested Class
static class StaticNested { void hello() { System.out.println("Hello, " + Outer.NAME); } }
A separate class, but Outer cannot be referenced. this, you can access private methods and fields
It is no longer attached to Outer instances, but a completely independent class, so it cannot reference Outer.this, but it can access Outer's private static fields and static methods. If you move StaticNested outside of Outer, you lose access to private.