Definition
- Stack is a kind of linear table with limited operation
- The limitation is that only insert and delete operations are allowed at one end of the table
- One end that allows operations is called the top of the stack, and the other is called the bottom of the stack
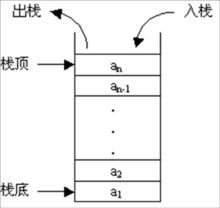
Main operation
- Push / push / push: insert element
- Out / out of stack: delete element
Nature
- First in first out: the element that first enters the stack can only be last out of the stack
Classification of stack
- Static stack: stack implemented by array
- Dynamic stack: a stack implemented by linked list
Implementation of dynamic stack
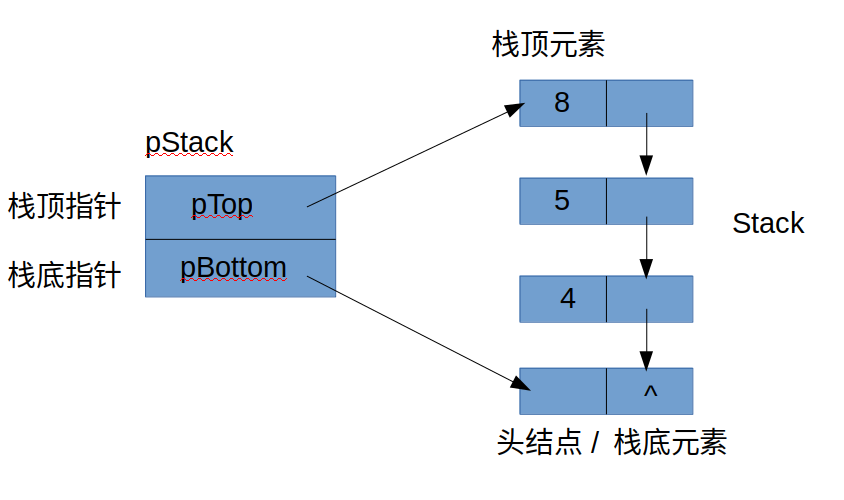
Stack structure
#include <stdio.h> #include <stdlib.h> #include <malloc.h> #include <stdbool.h> /** Define node data type */ typedef struct Node{ int data; struct Node * pNext; }NODE,*PNODE; /** Define stack structure */ typedef struct stack{ PNODE pTop; PNODE pBottom; }STACK,*PSTACK; /** Declaring function */ void init_stack(PSTACK); //Initializing a stack, that is, building a stack structure void push_stack(PSTACK,int); //Stack an element void traverse_stack(PSTACK); //Traversal stack bool isEmpty(PSTACK); //Judge stack space bool pop_stack(PSTACK,int *); //Stack out void clear_stack(PSTACK); //Emptying stack int main() { STACK s; //Define a stack //Test initialization stack init_stack(&s); //Test stack push_stack(&s,2); push_stack(&s,5); push_stack(&s,4); push_stack(&s,8); //Test traversal stack traverse_stack(&s); //Test empty stack clear_stack(&s); //Test out stack int element; //Save deleted elements if(pop_stack(&s,&element)){ printf("Delete succeeded. The elements you deleted are:%d \n",element); }else{ printf("Delete failed!\n"); } traverse_stack(&s); printf("Hello world!\n"); return 0; } /** Initialization stack */ void init_stack(PSTACK pStack){ //Generate a header node for the stack that does not store any data, and point to it with the stack bottom pointer pStack->pBottom = (PNODE)malloc(sizeof(NODE)); if(pStack->pBottom == NULL){ printf("Memory allocation failed, end of program!"); exit(-1); } //The memory allocation is successful. Also point the top of stack pointer to the head node pStack->pTop = pStack->pBottom; //Initialize the header node and set the pointer field of the header node to NULL pStack->pTop->pNext=NULL; } /** Stack an element */ void push_stack(PSTACK pStack,int element){ //Dynamically allocate a storage space for the new node PNODE pNew = (PNODE)malloc(sizeof(NODE)); if(pNew == NULL){ printf("Memory allocation failed, end of program!"); exit(-1); } //Put the value of the newly inserted element into the data field pNew->data = element; //Stack pressing: hang the new node behind the head node pNew->pNext = pStack->pTop; //Point the top of stack pointer to the top of stack element pStack->pTop = pNew; return; } /** Traversal stack */ void traverse_stack(PSTACK pStack){ //Get the location of the top element of the stack PNODE p = pStack->pTop; //Traversal stack while(p != pStack->pBottom){ printf("%d\t",p->data); p = p->pNext; } printf("\n"); return; } /** Judge whether the stack is empty */ bool isEmpty(PSTACK pStack){ if(pStack->pBottom == pStack->pTop) return true; else return false; } /** Stack and save the stack element */ bool pop_stack(PSTACK pStack,int *pElement){ if(isEmpty(pStack)){ printf("Stack out failed! The stack is empty!"); return false; } //Define a node whose value is saved and deleted PNODE p = pStack->pTop; *pElement = p->data; pStack->pTop = p->pNext; free(p); p=NULL; return true; } /** Emptying stack */ void clear_stack(PSTACK pStack){ if(isEmpty(pStack)) return; PNODE p = pStack->pTop; PNODE q = NULL; while(p != pStack->pBottom){ q = p->pNext; free(p); p=q; } pStack->pTop = pStack->pBottom; return; }