Write in front: This document uses version 7.0, version 8.0 method name has been changed, it is recommended to see the official document, the overall business logic and principles have not changed. Official website
In the process of android programming, we will write a lot of layout and click events. Simple and repetitive operations like initial view and view monitor make people feel troublesome, so we can use annotations to implement them. ButterKnife is a relatively simple and easy-to-understand open source framework in annotations, while online documents and examples are out of date. Version changes after 7.0 Very big, the previous annotations can not be used, so draw on the official documents to summarize, and then introduce how to use. Basically refer to the official documents, plus their own experience.
ButterKnife Advantages:
1. Powerful View Binding and Click Event Processing Function, Simplify Code, Improve Development Efficiency
2. Handling ViewHolder Binding in Adapter Conveniently
3. Runtime will not affect the efficiency of APP, easy to use and configure.
4. Clear code and readability
Use experience:
1.Activity ButterKnife.bind(this); must be set ContentView (); and after the parent bind is bound, the subclass does not need to bind any more.
2.Fragment ButterKnife.bind(this, mRootView);
3. Attribute layout cannot be modified with private or static, otherwise error will be reported.
4.setContentView() cannot be implemented through annotations. (Other annotation frameworks can)
Official websitehttp://jakewharton.github.io/butterknife/
Use steps:
Import the ButterKnife jar package:
1) If you are Eclipse, you can download the jar package from the official website.
2) If you are Android Studio, you can search butterknife directly by File - > Project Structure - > Dependencies - > Library dependency. The first one is
3) Of course, you can also configure it with maven and gradle
-
MAVEN
-
<dependency>
-
<groupId>com.jakewharton</groupId>
-
<artifactId>butterknife</artifactId>
-
<version>(insert latest version)</version>
-
</dependency>
-
-
GRADLE
-
compile 'com.jakewharton:butterknife:(insert latest version)'
-
-
Be sure to suppress this lint warning in your build.gradle.(Close)
-
lintOptions {
-
disable 'InvalidPackage'
-
}
Note that if you use it in a Library project, follow the following steps (described in github) or you cannot find view:
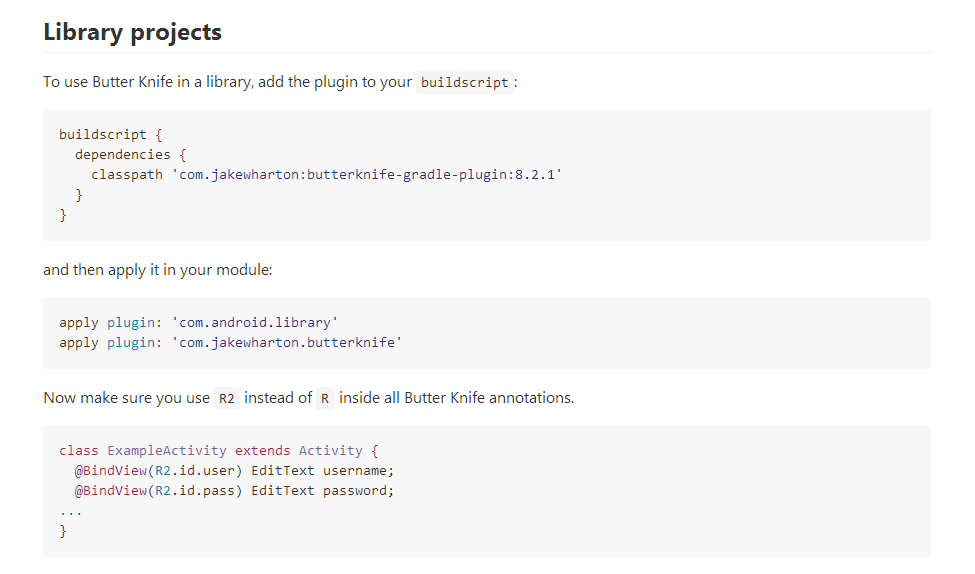
Note: Official website and github There are also corresponding reference steps.
2. Common usage methods:
1) Since onCreate binds Activity in Activity every time, I suggest writing a BaseActivity to complete the binding and inherit subclasses.
Note: ButterKnife.bind(this); binding Activity must be after setContentView:
The implementation is as follows (as in the FragmentActivity implementation):
-
public abstract class BaseActivity extends Activity {
-
public abstract int getContentViewId();
-
-
@Override
-
protected void onCreate(Bundle savedInstanceState) {
-
super.onCreate(savedInstanceState);
-
setContentView(getContentViewId());
-
ButterKnife.bind(this);
-
initAllMembersView(savedInstanceState);
-
}
-
-
protected abstract void initAllMembersView(Bundle savedInstanceState);
-
-
@Override
-
protected void onDestroy() {
-
super.onDestroy();
-
ButterKnife.unbind(this);
-
}
-
}
2) Binding fragment
-
public abstract class BaseFragment extends Fragment {
-
public abstract int getContentViewId();
-
protected Context context;
-
protected View mRootView;
-
-
@Nullable
-
@Override
-
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
-
mRootView =inflater.inflate(getContentViewId(),container,false);
-
ButterKnife.bind(this,mRootView);
-
this.context = getActivity();
-
initAllMembersView(savedInstanceState);
-
return mRootView;
-
}
-
-
protected abstract void initAllMembersView(Bundle savedInstanceState);
-
-
@Override
-
public void onDestroyView() {
-
super.onDestroyView();
-
ButterKnife.unbind(this);
-
}
-
}
3) Binding view
-
@Bind(R.id.hello_world)
-
TextView mHelloWorldTextView;
-
@Bind(R.id.app_name)
-
TextView mAppNameTextView;
4) Binding resources
-
@BindString(R.string.app_name)
-
String appName;
-
@BindColor(R.color.red)
-
int textColor;
-
@BindDrawable(R.mipmap.ic_launcher)
-
Drawable drawable;
-
@Bind(R.id.imageview)
-
ImageView mImageView;
-
@Bind(R.id.checkbox)
-
CheckBox mCheckBox;
-
@BindDrawable(R.drawable.selector_image)
-
Drawable selector;
5) Adapter ViewHolder binding
-
public class TestAdapter extends BaseAdapter {
-
private List<String> list;
-
private Context context;
-
-
public TestAdapter(Context context, List<String> list) {
-
this.list = list;
-
this.context = context;
-
}
-
-
@Override
-
public int getCount() {
-
return list==null ? 0 : list.size();
-
}
-
-
@Override
-
public Object getItem(int position) {
-
return list.get(position);
-
}
-
-
@Override
-
public long getItemId(int position) {
-
return position;
-
}
-
-
@Override
-
public View getView(int position, View convertView, ViewGroup parent) {
-
ViewHolder holder;
-
if (convertView == null) {
-
convertView = LayoutInflater.from(context).inflate(R.layout.layout_list_item, null);
-
holder = new ViewHolder(convertView);
-
convertView.setTag(holder);
-
} else {
-
holder = (ViewHolder) convertView.getTag();
-
}
-
holder.textview.setText("item=====" + position);
-
return convertView;
-
}
-
-
static class ViewHolder {
-
@Bind(R.id.hello_world)
-
TextView textview;
-
-
public ViewHolder(View view) {
-
ButterKnife.bind(this, view);
-
}
-
}
-
}
6) Binding of click events: Click events can be bound without declaring view and without setOnClickLisener ().
A. Direct binding of a method
-
@OnClick(R.id.submit)
-
public void submit(View view) {
-
-
}
b. The parameters of all listening methods are optional
-
@OnClick(R.id.submit)
-
public void submit() {
-
-
}
c. Define a specific type that will be automatically converted
-
@OnClick(R.id.submit)
-
public void sayHi(Button button) {
-
button.setText("Hello!");
-
}
d. Multiple view s handle the same click event in a unified way, which is convenient and avoids the trouble of repeated invocation of extraction methods.
-
@OnClick({ R.id.door1, R.id.door2, R.id.door3 })
-
public void pickDoor(DoorView door) {
-
if (door.hasPrizeBehind()) {
-
Toast.makeText(this, "You win!", LENGTH_SHORT).show();
-
} else {
-
Toast.makeText(this, "Try again", LENGTH_SHORT).show();
-
}
-
}
e. Custom view can bind its own listener without specifying id
-
public class FancyButton extends Button {
-
@OnClick
-
public void onClick() {
-
-
}
-
}
f. Add an addTextChangedListener to EditText (that is, the use of multiple callbacks to listen for) and use the specified callbacks to implement the method you want to call back. Which annotation will be used without peer-in to see the annotations on the source code?
-
@OnTextChanged(value = R.id.mobileEditText, callback = OnTextChanged.Callback.BEFORE_TEXT_CHANGED)
-
void beforeTextChanged(CharSequence s, int start, int count, int after) {
-
-
}
-
@OnTextChanged(value = R.id.mobileEditText, callback = OnTextChanged.Callback.TEXT_CHANGED)
-
void onTextChanged(CharSequence s, int start, int before, int count) {
-
-
}
-
@OnTextChanged(value = R.id.mobileEditText, callback = OnTextChanged.Callback.AFTER_TEXT_CHANGED)
-
void afterTextChanged(Editable s) {
-
-
}
7) Unify a set of View s
A. Load a list
-
@Bind({ R.id.first_name, R.id.middle_name, R.id.last_name })
-
List<EditText> nameViews;
b. Setting up unified processing
-
static final ButterKnife.Action<View> DISABLE = new ButterKnife.Action<View>() {
-
@Override public void apply(View view, int index) {
-
view.setEnabled(false);
-
}
-
};
-
static final ButterKnife.Setter<View, Boolean> ENABLED = new ButterKnife.Setter<View, Boolean>() {
-
@Override public void set(View view, Boolean value, int index) {
-
view.setEnabled(value);
-
}
-
};
c. Unified Operational Processing, e.g. Setting Points, Attributes, etc.
-
ButterKnife.apply(nameViews, DISABLE);
-
ButterKnife.apply(nameViews, ENABLED, false);
8) Optional binding: By default, "binding" and "listening" binding are required. If the target view cannot be found, an exception is thrown. So do short processing
-
@Nullable @Bind(R.id.might_not_be_there) TextView mightNotBeThere;
-
-
@Nullable @OnClick(R.id.maybe_missing) void onMaybeMissingClicked() {
-
-
}
3. Code obfuscation
-
-keep class butterknife.** { *; }
-
-dontwarn butterknife.internal.**
-
-keep class **$$ViewBinder { *; }
-
-
-keepclasseswithmembernames class * {
-
@butterknife.* <fields>;
-
}
-
-
-keepclasseswithmembernames class * {
-
@butterknife.* <methods>;
-
}
IV. The Use of Zelezny Plug-ins
In Android Studio - > File - > Settings - > Plugins - > Search for Zelezny download add line, you can quickly generate the corresponding component instance object, without manual writing. When used, on the layout resource code to import annotated Activity or Fragment or ViewHolder, right-click --> Generate -- Generate ButterKnife Injections, and then a selection box appears as shown in the figure. (This dynamic chart is from the official website)
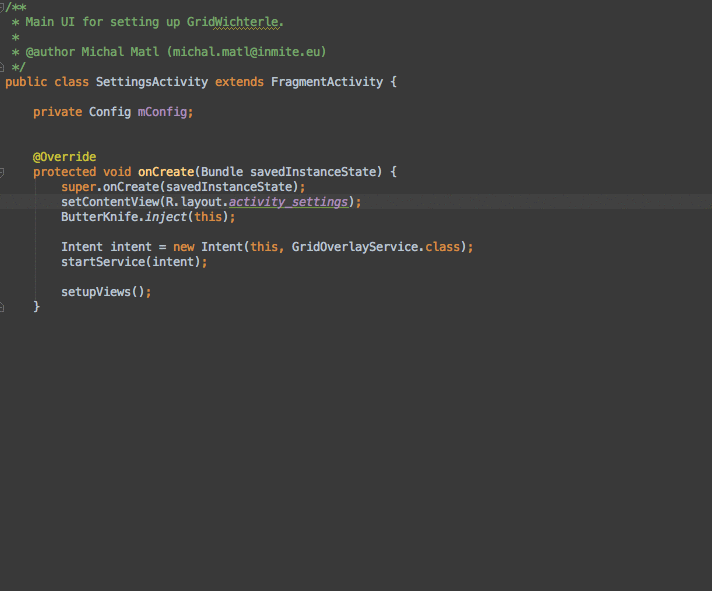