angularJs-The factory and service of angularJs
Keywords:
angular
AngularJS
angularAndService
Angular js supports the concept of "separation of concerns" in the architecture using services; services are both js functions and responsible for only one specific task; this also makes them separate entities for maintenance and testing; controllers and filters can call them as the basis of requirements. Services are injected using dependency injection mechanism of angular js.
Angular Js provide many intrinsic services such as: $http,$route,$window,$location, etc. Each service is responsible for a specific task, $http is used to create Ajax calls to obtain server data, $route is used to define routing information,... But built-in services are always prefixed with a $symbol.
Introduce two ways to create services: factories and services
Factory
For services of existing instance objects, Factory takes precedence and returns this object directly. For example: share transfer parameters among multiple controller s, encapsulate the request resources of $resource
Service
For services that need to be created by new, service takes precedence, and angular automatically creates the object instance. Service is easier to organize a set of API s with the same business logic, which makes business logic more cohesive.
Example display
<html ng-app="myApp" >
<head>
<title>The factoryAndService of angularJs</title>
</head>
<body>
<h2>AngularJS`Sample</h2>
<div ng-controller="CalcController">
<p>Number: <input type="number" ng-model="number" />
<button ng-click="square()">x<sup>2</sup></button>
<p>Result: {{result}}</p >
</div>
<script src="angular.min.js"></script>
<script src="angular-route.min.js"></script>
<script src="angular-ui-router.min.js"></script>
<script>
var myApp = angular.module("myApp",[]);
//factory
myApp.factory("mathCalc",function(){
var factory = {};
factory.formula = function(a,b){
return a *b
}
return factory ;
});
//service
myApp.service("myService",function(mathCalc){
this.square = function(a){
return mathCalc.formula(a,a);
}
});
myApp.controller("CalcController",["myService","$scope",function(myService,$scope){
$scope.square = function(){
$scope.result = myService.square($scope.number);
}
}])
</script>
</body>
</html>
Browser Display
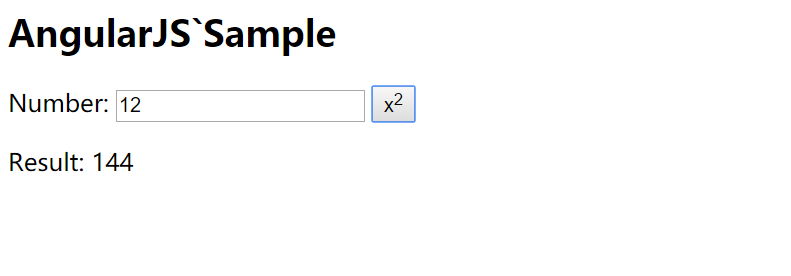
Posted by willchoong on Sun, 10 Feb 2019 06:24:19 -0800