How to transmit data over the network in android: HttpURLConnection OKHttp JsonObject Gson
Records of 2017/3/11
Using network technology: using data on the network to let our APP interact with the back-end server
Usage of WebView: Embedding a browser in APP to display our web pages (you know the best way to develop a responsive page after learning the web)
Small example:
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<WebView
android:id="@+id/web_view"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</LinearLayout>
Increase network request privileges:
<uses-permission android:name="android.permission.INTERNET"/>
MainActivity.java
package com.example.ldp.com.webview;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.webkit.WebView;
import android.webkit.WebViewClient;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
WebView webView = (WebView)findViewById(R.id.web_view);
webView.getSettings().setJavaScriptEnabled(true);
webView.setWebViewClient(new WebViewClient());
webView.loadUrl("http://www.baidu.com");
}
}
Design sketch: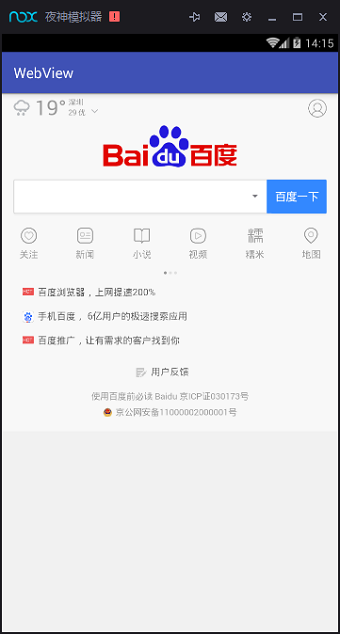
Here we use an HTTP protocol request. There is a lot of knowledge on the Internet. Interested netizens can go to see it.
Learn to use HttpURLConnection
Two ways: HttpURLConnection and HttpClient.
Steps:
This step should be clear at a glance.ERL url = new URL("http://www.baidu.com"); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setRequestMethod("GET"); connection.setConnecTimeout(8000); connection.setReadTimeout(8000); InputStream in = connection.getInputStream(); connection.disconnect();
ScorllView > Control: Mobile phone screen is very small, usually content can not be displayed, with this can be up and down sliding display.
Next, I'll take a screenshot to illustrate:
Finally, remember to open INTERNET access rights
Why use the runOnUiThread() method? Because subthreads are not allowed to perform UI operations in android, this method is used
Subthreads can be converted into main threads.
We do not have to use HttpURLConnection for network access. There are many network communication libraries on the market that can be replaced.
For example, OKHttp, Picasso, Retrofit, etc.
Focus on OKHttp (add library dependencies):
Design sketch:
Focus on learning how to parse JSON data from the network. In the beginning, the network data were transmitted by xml, which is the transmission.
Later, it was found that this kind of data is not only very slow, but also inefficient. The later json data format:
Use JSONObject to parse (which I often use on the web)
Design sketch:
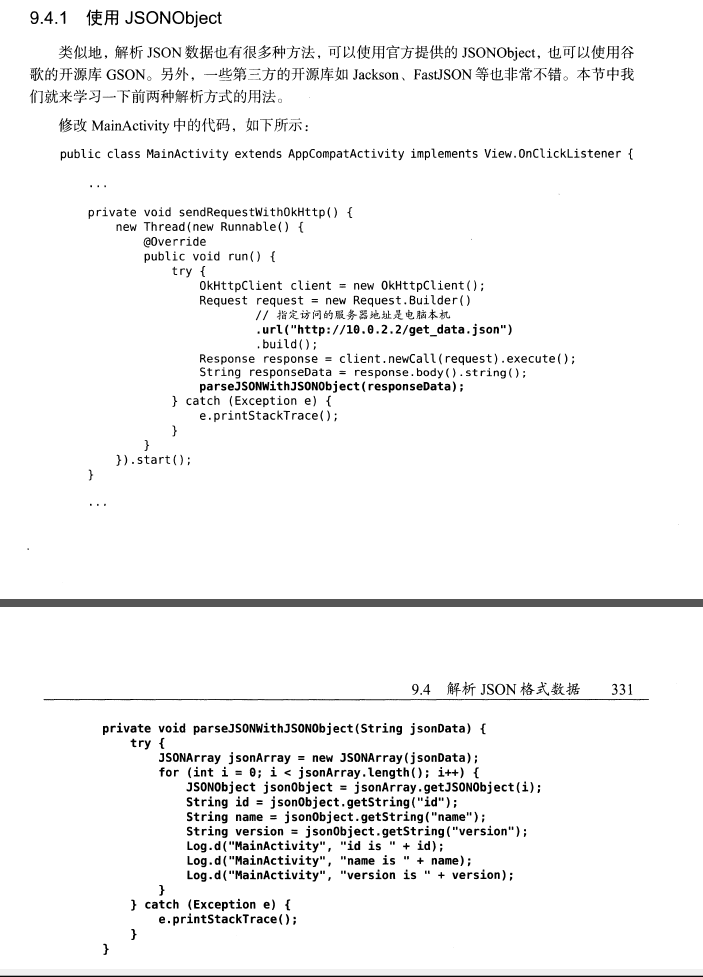
Using GSON parsing:
Add dependency libraries: com.google.code.gson:gson:2.8.0
Where is it simpler than JSONObject in that it converts json data format into objects and learns object mapping?
Is it easy to understand and use it more and more simple?
Two sentences of code are done.
Gson gson = new Gson(); Person person = gson.fromJson(jsonData,Person.class);
If one group is OK, but what if it's multiple groups? Think of List, and with the help of the data type that TypeToken expects to parse, it's passed into fromJson
List<Person> people = gson.fromJson(jsonData,new TypeToken<Lsit<Person>>(){}.getType());
Usage effect chart:
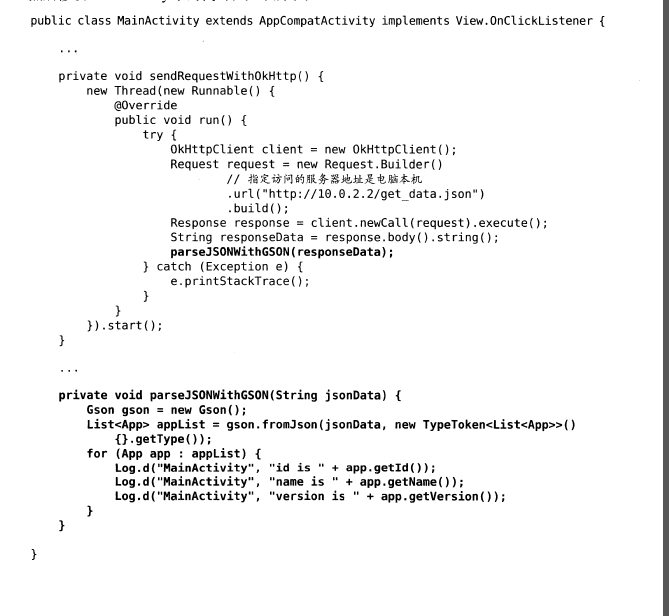
When using network transmission, we all need to make an HTTP request to the network, and there will be a lot of the same and redundant code.
At this point, we want to write this as a tool class:
Design sketch: