1. International conventions: Fig. 1 + Github address
Android Multi-State Layout + Global ToolBar-Github Address Give me a star t. Thank you for your support.
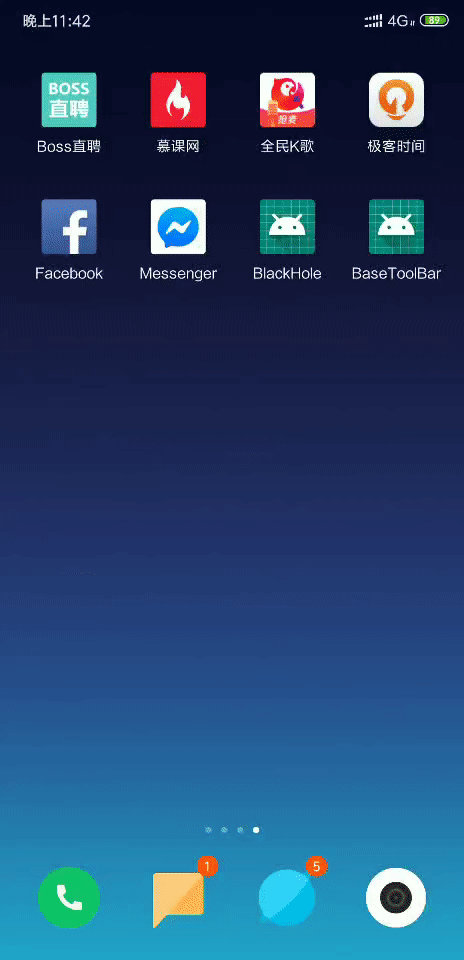
2. Functional overview
2.1 Brief Introduction of Global Title Bar Function
1. Custom XML
2. One line of code controls whether the title bar is added
3. Basic methods can be encapsulated in BaseActivity, such as Title text, color, size, left button or text, etc.
4. Highly custom encapsulation for direct use of commercial projects
2.2 Multi-state Layout
1. Two basic layouts, Loading Layout and Fail Layout, have been added by default, but you need to add your own page and get View directly for other operations.
2. Accessible layout status
3. One line of code to set the layout status
4. If you feel that the basic layout is not enough, you can customize to add layout XML display.
3. Usage
1. add
1.1 Add in global build.gradle
allprojects { repositories { ... maven { url 'https://jitpack.io' } } }
1.2 Add in build.gradle under app
dependencies { implementation 'com.github.luckyfj:BaseToolBar:1.0.0' }
2. The BaseStateLayout method can be directly used in the inheritance system of BaseActivity. Here is my TestBaseActivity, which can be referred to.
package com.hackerfj.basetoolbar; import android.view.View; import android.view.ViewGroup; import android.widget.LinearLayout; import android.widget.TextView; import com.hackerfj.statelayout.BaseStateLayout; public abstract class BaseActivity extends BaseStateLayout { /** * @return Content layout */ protected abstract int contentView(); /** * Initialization method */ protected abstract void initViews(); /** * Set the content layout, because this is BaseActivity, which passes contentView to inheritance page settings * @return */ @Override protected int setContentLayout() { return contentView(); } /** * Set whether to add Toolbar to set boolean, and if set to false, set Toolbar to null. * @return */ @Override protected boolean isAddToolBar() { return true; } /** * Setting the title bar View * @return */ @Override protected int setToolbar() { return R.layout.base_tool_bar; } /** * Setting View in Load * @return */ @Override protected int setLoadingView() { return R.layout.layout_loading; } /** * Setting View for Load Failure * @return */ @Override protected int setFailView() { return R.layout.layout_fail; } /** * Set the initialization operation, because this is BaseActivity, which passes initView to the inheritance page settings * @return */ @Override public void initView() { initViews(); } /** * Example: Setting the title, you can use it to do more things, just to name a few. * @param title */ public void setToolbarTitle(String title){ TextView tvTitle = getBaseToolbar().findViewById(R.id.tv_bar_title); tvTitle.setText(title); } /** * Dynamically set the View I want to display * @param view */ public void addView(int view){ View myView = View.inflate(this, view, null); LinearLayout.LayoutParams params = new LinearLayout.LayoutParams(ViewGroup.LayoutParams.MATCH_PARENT, ViewGroup.LayoutParams.MATCH_PARENT); myView.setLayoutParams(params); setMyView(myView); } }
3. Use the Activity sample code Kotlin
package com.hackerfj.basetoolbar import kotlinx.android.synthetic.main.base_tool_bar.* class TestActivity : BaseActivity() { override fun contentView(): Int { return R.layout.activity_main } override fun initViews() { setToolbarTitle("homepage") tv_bar_title.setOnClickListener { // Layout switch to loading setLayout(com.hackerfj.statelayout.LayoutState.STATE_LOADING) } tv_bar_left.setOnClickListener { // Layout switch to failure setLayout(com.hackerfj.statelayout.LayoutState.STATE_FAIL) } tv_bar_right.setOnClickListener { // Layout switch to content page // setLayout(LayoutState.STATE_SUCCESS) //Dynamically set the View I want to display addView(R.layout.layout_my_view) } } }
4. Use Activity sample code Java
package com.hackerfj.basetoolbar; import android.view.View; public class TestActivity extends BaseActivity { @Override protected int contentView() { return R.layout.activity_main; } @Override protected void initViews() { setToolbarTitle("homepage"); findViewById(R.id.tv_bar_title).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Layout switch to loading setLayout(com.hackerfj.statelayout.LayoutState.STATE_LOADING); } }); findViewById(R.id.tv_bar_left).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Layout switch to failure setLayout(com.hackerfj.statelayout.LayoutState.STATE_FAIL); } }); findViewById(R.id.tv_bar_right).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Layout switch to content page // setLayout(LayoutState.STATE_SUCCESS) //Dynamically set the View I want to display addView(R.layout.layout_my_view); } }); } }