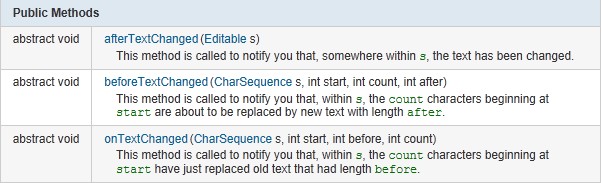
When the content in EditText changes, the TextChangedListener event will be triggered, and the abstract method in TextWatcher will be called.
Layout:
1, Monitor the number of text boxes and prompt how many characters can be input
- <?xml version="1.0" encoding="utf-8"?>
- <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent" >
- <EditText
- android:id="@+id/main_et"
- android:layout_width="fill_parent"
- android:layout_height="wrap_content" />
- <TextView
- android:id="@+id/main_tv"
- android:layout_width="fill_parent"
- android:layout_height="wrap_content" />
- </LinearLayout>
MainActivity:
- package chay.mian;
- import android.app.Activity;
- import android.os.Bundle;
- import android.text.Editable;
- import android.text.TextWatcher;
- import android.view.View;
- import android.widget.EditText;
- import android.widget.TextView;
- public class MainActivity extends Activity {
- private EditText editText;
- private TextView tip;
- private final int charMaxNum = 10; //Number of words allowed
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
- init();
- }
- private void init() {
- editText = (EditText) findViewById(R.id.main_et);
- editText.addTextChangedListener(new EditChangedListener());
- tip = (TextView) findViewById(R.id.main_tv);
- tip.setText("0/"+charMaxNum);
- }
- class EditChangedListener implements TextWatcher {
- private CharSequence temp; //Text before listening
- private int editStart; //Cursor start position
- private int editEnd; //Cursor end position
- //Status before entering text
- @Override
- public void beforeTextChanged(CharSequence s, int start, int count,int after) {
- temp = s;
- }
- //Enter the status in the text, count is the number of characters entered at one time
- @Override
- public void onTextChanged(CharSequence s, int start, int before, int count) {
- // if (charMaxNum - s.length() <= 5) {
- //tip.setText("can also input" + (charMaxNum - s.length()) + "character");
- // }
- tip.setText((s.length()) + "/" + charMaxNum);
- }
- //Status after entering text
- @Override
- public void afterTextChanged(Editable s) {
- /** Get the start and end position of the cursor, record the number index just exceeded after the maximum number for control*/
- editStart = editText.getSelectionStart();
- editEnd = editText.getSelectionEnd();
- if (temp.length() > charMaxNum) {
- //Toast.makeText(getApplicationContext(), "input up to 10 characters", Toast.LENGTH_SHORT).show();
- s.delete(editStart - 1, editEnd);
- editText.setText(s);
- editText.setSelection(s.length());
- }
- }
- };
- }
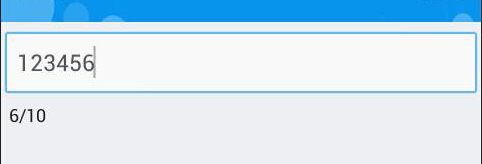
2, For data type inspection, when the input is not an integer number, the input box will pop up immediately and prompt for correction
MainActivity:
- package chay.mian;
- import android.app.Activity;
- import android.app.AlertDialog;
- import android.content.DialogInterface;
- import android.os.Bundle;
- import android.text.Editable;
- import android.text.TextWatcher;
- import android.util.Log;
- import android.widget.EditText;
- import android.widget.TextView;
- public class MainActivity extends Activity {
- private EditText editText;
- private TextView tip;
- String str;
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_mian);
- init();
- }
- private void init() {
- editText = (EditText) findViewById(R.id.mian_et);
- editText.addTextChangedListener(new EditChangedListener());
- tip = (TextView) findViewById(R.id.mian_tv);
- tip.setText("Please enter an integer number");
- }
- class EditChangedListener implements TextWatcher {
- //Status before entering text
- @Override
- public void beforeTextChanged(CharSequence s, int start, int count, int after) {
- Log.d("TAG", "beforeTextChanged--------------->");
- }
- //Enter the status in the text, count is the number of characters entered at one time
- @Override
- public void onTextChanged(CharSequence s, int start, int before, int count) {
- Log.d("TAG", "onTextChanged--------------->");
- }
- //Status after entering text
- @Override
- public void afterTextChanged(Editable s) {
- Log.d("TAG", "afterTextChanged--------------->");
- str = editText.getText().toString();
- try {
- // if ((editText.getText().toString()) != null)
- Integer.parseInt(str);
- } catch (Exception e) {
- showDialog();
- }
- }
- };
- private void showDialog() {
- AlertDialog dialog;
- AlertDialog.Builder builder = new AlertDialog.Builder(this);
- builder.setTitle("news").setIcon(android.R.drawable.stat_notify_error);
- builder.setMessage("The integer number you output is wrong, please correct it");
- builder.setPositiveButton("Determine", new DialogInterface.OnClickListener() {
- @Override
- public void onClick(DialogInterface dialog, int which) {
- // TODO Auto-generated method stub
- }
- });
- dialog = builder.create();
- dialog.show();
- }
- }
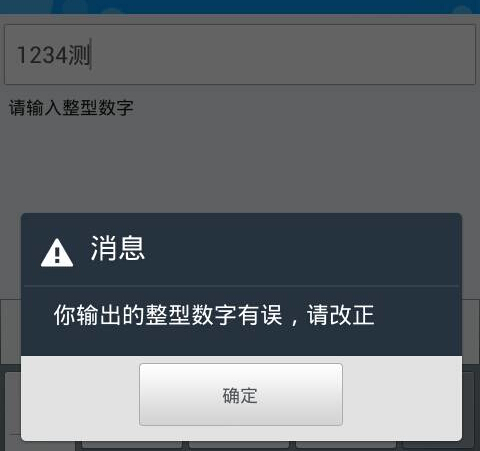
View the order in which these methods are called in LogCat:
beforeTextChanged-->onTextChanged-->onTextChanged
Welcome to http://blog.csdn.net/ycwol/article/details/46594275