Preface
The tool used in this note is Android Studio.
I am a rookie. This is my first study note in Jane. I hope that in the future I can also insist on writing, for nothing else, just to improve my own technology. If you read my notes, which happened to be incorrect, please give me more advice. Thank you very much!
Recently, I want to write a small project in my spare time. The purpose is to exercise myself. The first step encountered the problem of social account login. This article mainly talks about the integration of QQ login, and may write micro-letters and micro-blogs in the future.
QQ Login Video Address: http://www.imooc.com/learn/656
QQ login integration
-
Tencent Open Platform Address: http://open.qq.com/
If the students who have not registered need to register first, they also need to upload their own ID cards and certificates. Tencent can only register successfully after passing the examination. -
Create an application and get the appID. If you create an application, the test will assign you an appid, the rest will not be used; if it is really used in the project, you need to fill in all the requirements of Tencent.
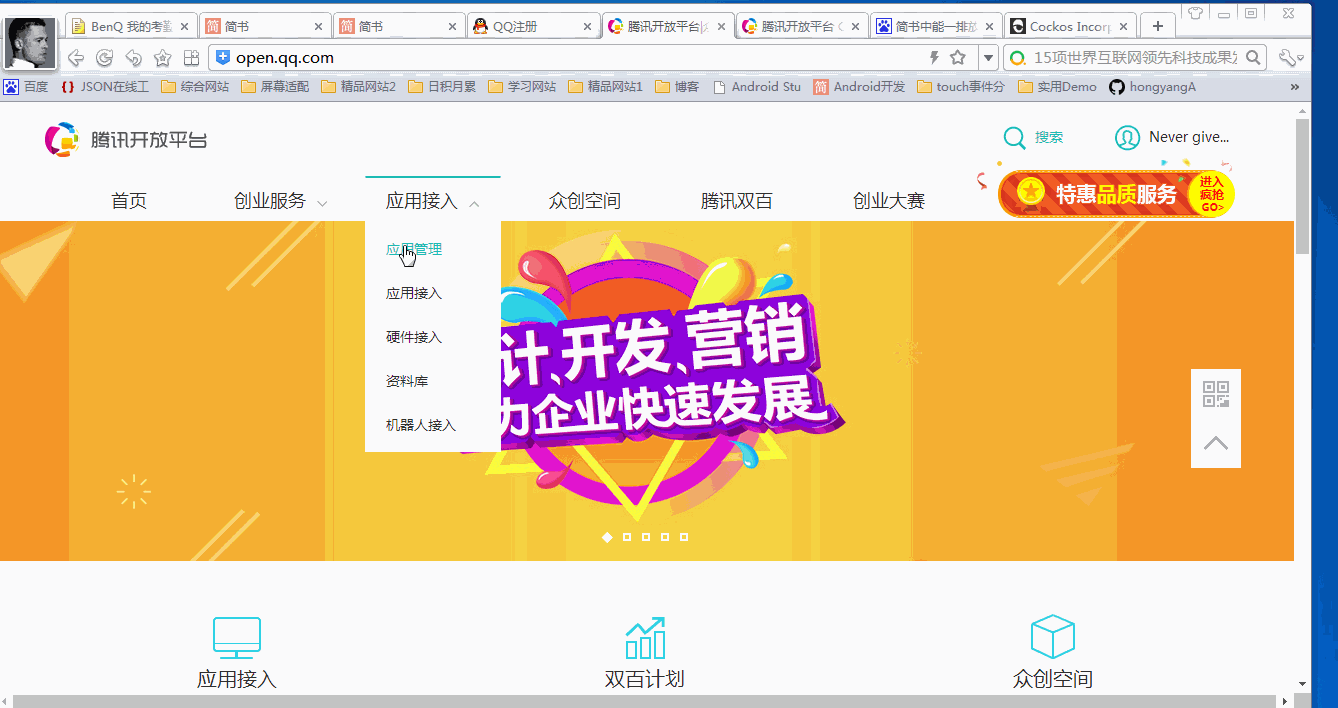
3. Download Tencent SDK, address: http://wiki.open.qq.com/wiki/mobile/SDK%E4%B8%8B%E8%BD%BD
After downloading, decompress as follows:
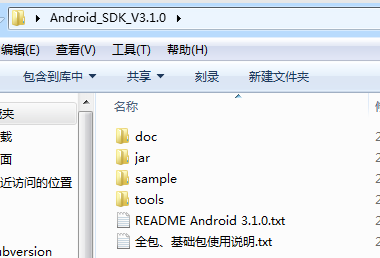
Open the jar package, copy the mta-sdk-1.6.2.jar and mta-sdk-1.6.2.jar into your modle libs, and then add the dependencies.


Preparations completed, code phase
Add appropriate permissions to AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET"></uses-permission>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"></uses-permission>
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE"></uses-permission>
Add these codes to Application
<activity
android:name="com.tencent.tauth.AuthActivity"
android:launchMode="singleTask"
android:noHistory="true">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<!-- This place needs to be applied for on an open platform. appid:tencent+appid -->
<data android:scheme="tencent1105765943" />
</intent-filter>
</activity>
<activity
android:name="com.tencent.connect.common.AssistActivity"
android:configChanges="orientation|keyboardHidden"
android:screenOrientation="behind"
android:theme="@android:style/Theme.Translucent.NoTitleBar" />
Layout code
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center_horizontal">
<ImageView
android:id="@+id/iv_figure"
android:layout_marginTop="20dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/unlogin"/>
<TextView
android:id="@+id/tv_nickname"
android:layout_marginTop="10dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="QQ Nickname?"/>
<Button
android:id="@+id/btn_login"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:text="Sign in"/>
<Button
android:id="@+id/btn_logout"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:text="Sign out"/>
</LinearLayout>
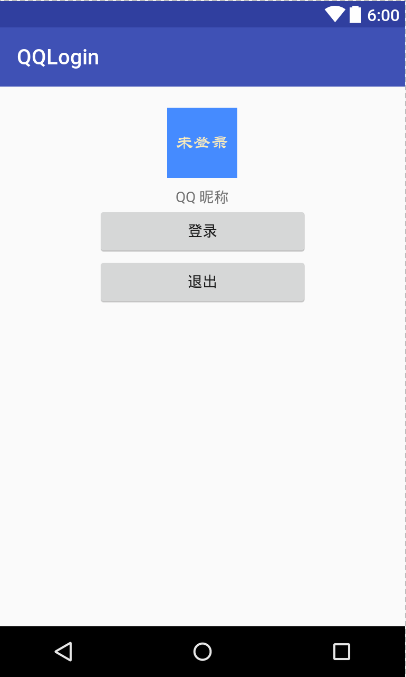
Code in MainActivity
package hexun.qqlogin;
import android.content.Intent;
import android.content.SharedPreferences;
import android.preference.PreferenceManager;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import com.squareup.picasso.Picasso;
import com.tencent.connect.UserInfo;
import com.tencent.connect.auth.QQToken;
import com.tencent.tauth.IUiListener;
import com.tencent.tauth.Tencent;
import com.tencent.tauth.UiError;
import org.json.JSONException;
import org.json.JSONObject;
public class MainActivity extends AppCompatActivity {
private Button login, logout;
private Tencent mTencent;
private static final String APPID = "1105771437";
private QQLoginListener mListener;
private UserInfo mInfo;
private ImageView figure;
private TextView nickName;
private String name, figureurl;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView();
}
private void initView() {
figure = (ImageView) findViewById(R.id.iv_figure);
nickName = (TextView) findViewById(R.id.tv_nickname);
mListener = new QQLoginListener();
// Instantiate Tencent
if (mTencent == null) {
mTencent = Tencent.createInstance(APPID, this);
}
// Click login
login = (Button) findViewById(R.id.btn_login);
login.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
QQLogin();
}
});
// Click Exit
logout = (Button) findViewById(R.id.btn_logout);
logout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (mTencent.isSessionValid()) {
mTencent.logout(MainActivity.this);
figure.setImageResource(R.drawable.unlogin);
nickName.setText("QQ Nickname?");
}
}
});
}
/**
* Login method
*/
private void QQLogin() {
//If session is unavailable, log in, otherwise you are logged in
if (!mTencent.isSessionValid()) {
mTencent.login(this, "all", mListener);
}
}
// Implementing a successful login interface
private class QQLoginListener implements IUiListener {
@Override
public void onComplete(Object object) { //Successful login
//Get openid and token
initOpenIdAndToken(object);
//Getting User Information
getUserInfo();
}
@Override
public void onError(UiError uiError) { //Logon failure
}
@Override
public void onCancel() { //Cancel Logon
}
}
private void initOpenIdAndToken(Object object) {
JSONObject jb = (JSONObject) object;
try {
String openID = jb.getString("openid"); //openid User Unique Identification
String access_token = jb.getString("access_token");
String expires = jb.getString("expires_in");
mTencent.setOpenId(openID);
mTencent.setAccessToken(access_token, expires);
} catch (JSONException e) {
e.printStackTrace();
}
}
private void getUserInfo() {
QQToken token = mTencent.getQQToken();
mInfo = new UserInfo(MainActivity.this, token);
mInfo.getUserInfo(new IUiListener() {
@Override
public void onComplete(Object object) {
JSONObject jb = (JSONObject) object;
try {
name = jb.getString("nickname");
figureurl = jb.getString("figureurl_qq_2"); //url of head picture
nickName.setText(name);
Picasso.with(MainActivity.this).load(figureurl).into(figure);
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void onError(UiError uiError) {
}
@Override
public void onCancel() {
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
mTencent.onActivityResultData(requestCode, resultCode, data, mListener);
}
}
Operation effect

End
If there are any mistakes in this article, please give more advice and sincere thanks. If I feel helpful to you and hope to give you a compliment, I will be more motivated to record my learning...
Link: http://www.jianshu.com/p/e59bc198e88f
Copyright belongs to the author. For commercial reprints, please contact the author for authorization. For non-commercial reprints, please indicate the source.