Fragment Details (II)
1. Basic concepts
1) What kind of ghost is it and what's its use?
Answer: Fragment can make your app enjoy silky design. If your app wants to improve its performance substantially and reduce its memory usage, the same interface Activity takes up more memory than Fragment, and the response speed of Fragment is much faster than Activty on low-end mobile phones, even several times faster! If your app currently or later has platforms such as transplanting tablets, you can save a lot of time and energy.
The following is a Fragment processing diagram given in the document, which corresponds to the different situations between the mobile phone and the tablet.
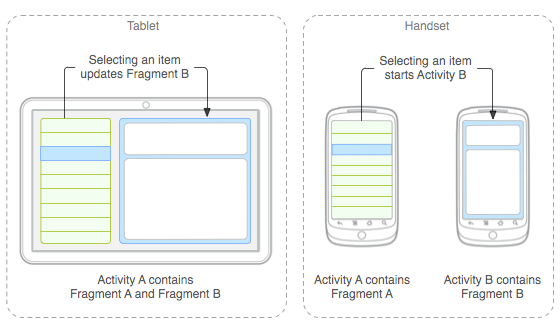
PS: Simple news browsing page, using two fragments to display news lists and news content respectively.
2) Fragment's Life Cycle Diagram
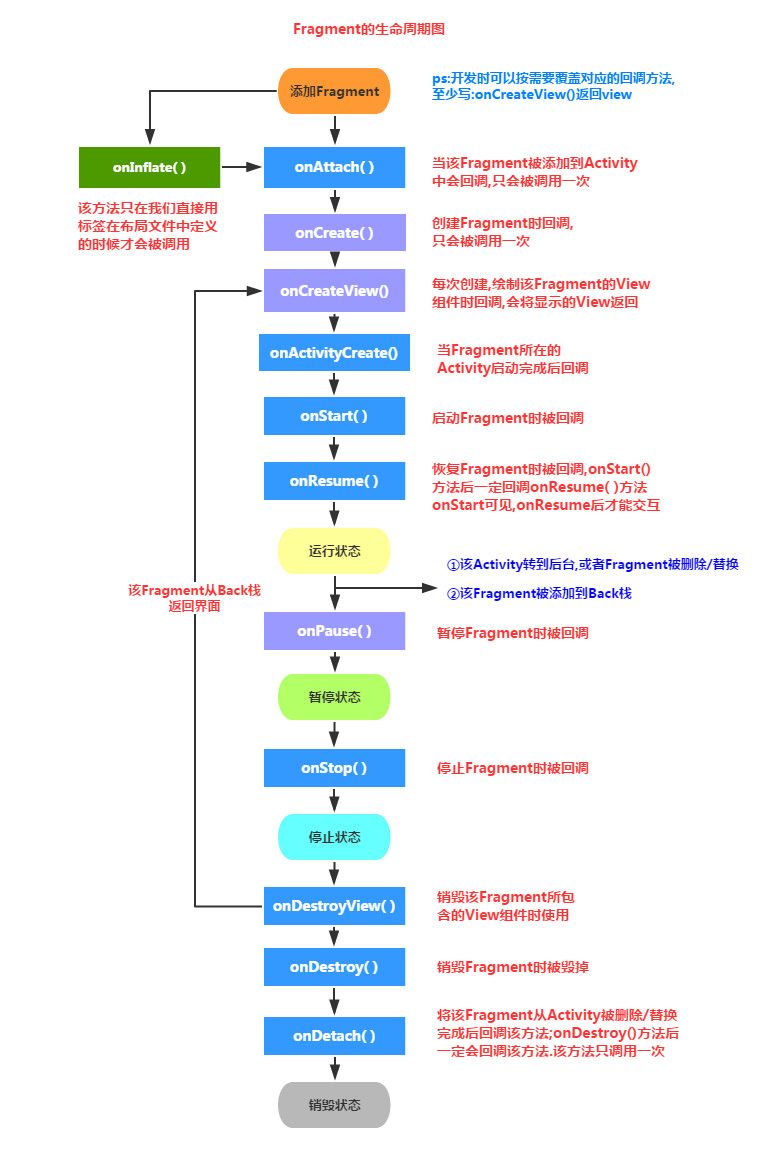
You can see that Fragment has several additional lifecycle callback methods than Activity:
- onAttach(Activity)
Called when Fragment is associated with Activity. - onCreateView(LayoutInflater, ViewGroup,Bundle)
Create a view of the Fragment - onActivityCreated(Bundle)
Called when Activity's onCreate method returns - onDestoryView()
Corresponding to onCreateView, this Fragment is called when the view is removed - onDetach()
Corresponding to onAttach, it is called when the Fragment and Activity association is cancelled
Note: All other methods except onCreateView, if you override them, you must call the parent class's implementation of the method.
2. Create a Fragment
1) Statically loaded Fragment
Implementation process:
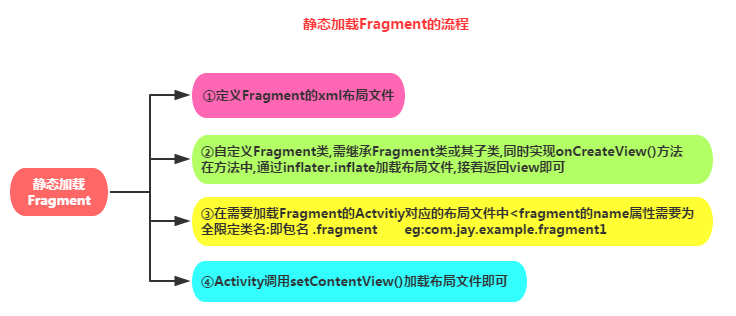
Sample code:
Step 1: Define the layout of fragments, which are what fragments display.
Step 2: To customize a Fragment class, you need to inherit Fragment or its subclasses, override the onCreateView() method, which calls the inflater.inflate() method to load the layout file of Fragment, and then return the loaded view object.
public class Fragmentone extends Fragment { @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { View view = inflater.inflate(R.layout.fragment1, container,false); return view; } }
Step 3: Add a fragment label to the layout file corresponding to Activeness that needs to load Fragments. Remember that the name attribute is a fully qualified class name, which is to include the package name of Fragments, such as:
<fragment android:id="@+id/fragment1" android:name="com.jay.example.fragmentdemo.Fragmentone" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1" />
Step 4: Activity calls setContentView() in onCreate() method to load the layout file.
2) Dynamic Loading Fragment
Implementation process:
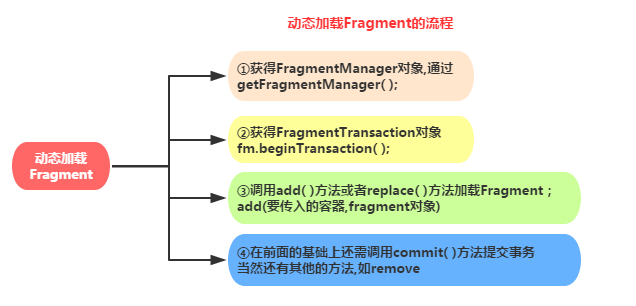
Sample code: This demonstrates switching Fragment when switching between horizontal and vertical screens:
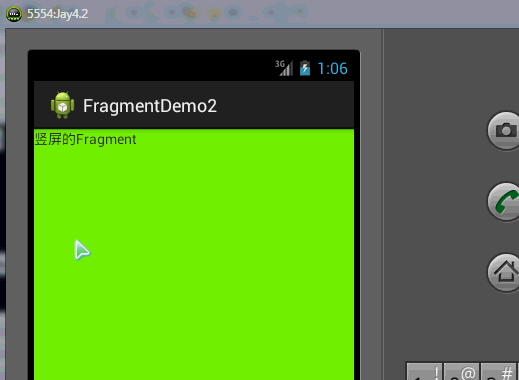
MainActivity
protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //[1] Obtaining the Resolution of Mobile Phone WindowManager wm = (WindowManager) getSystemService(WINDOW_SERVICE); int width = wm.getDefaultDisplay().getWidth(); int height = wm.getDefaultDisplay().getHeight(); //[2] Judgment of horizontal and vertical screens //[3] Managers who obtain Fragment s get them directly from context FragmentManager fragmentManager = getFragmentManager(); FragmentTransaction beginTransaction = fragmentManager.beginTransaction(); //Opening things if(height > width){ //The description is that the vertical screen loads a Fragment android.R.id.content// form representing the current mobile phone. beginTransaction.replace(android.R.id.content, new Fragment1()); }else { //The explanation is that the horizontal screen loads a Fragment. beginTransaction.replace(android.R.id.content, new Fragment2()); } //[4] Last step remember comment beginTransaction.commit(); }
Fragment1 and Fragment2 have the same code except for the different layout, so we paste a Fragment1.
//Defining Fragment as part of Activity public class Fragment1 extends Fragment { //Calling this method when the system first draws the ui allows Fragment to display its layout content @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { //Converting a layout into a View object through an inflator View view = inflater.inflate(R.layout.fragment1, null); return view; } }
3.Fragment Management and Fragment Transaction
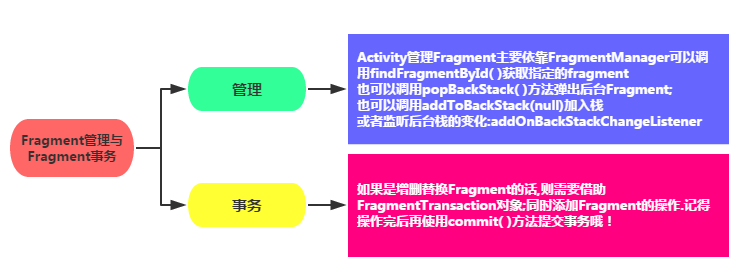
4. Interaction between Fragment and Activity
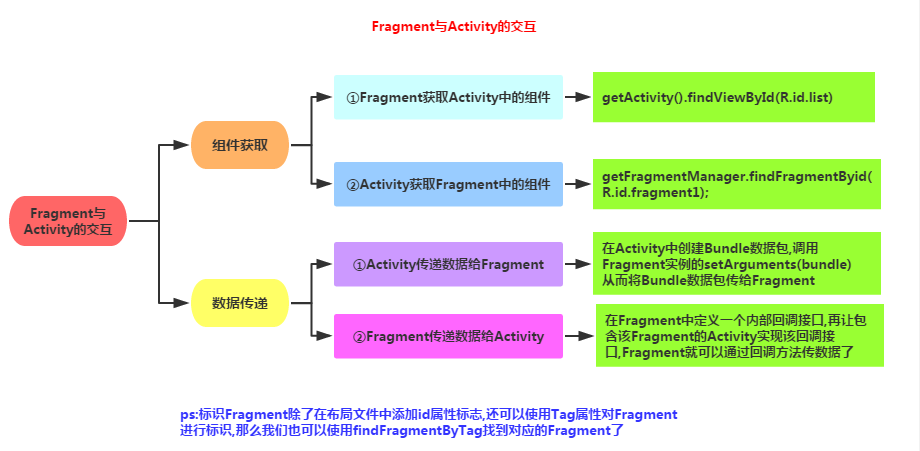
1) Component acquisition
Fragment obtains components in Activity | getActivity().findViewById(R.id.list); |
Activity obtains components in Fragment (based on id and tag) | getFragmentManager.findFragmentByid(R.id.fragment1); |
2) Data transfer
Activit y delivers data to Fragment:
Create a Bundle package in Activity, call the setArguments(bundle) of the Fragment instance to pass the Bundle package to Fragment, then call getArguments in Fragment to get the Bundle object, and parse it.
Fragment delivers data to Activity
Define an internal callback interface in Fragment, and let Activity which contains Fragment implement the callback interface. Fragment can transfer data through the callback interface, callback, I believe many people know what it is, but can not write it out. Baidu "fragment data to Activity" on the Internet is all Mr. Li Gang's code. It's really speechless. Here we write down some generations. Well, I believe the reader can understand it at a glance.
Step 1: Define a callback interface: (in Fragment)
/*Interface*/ public interface CallBack{ /*Define a way to get information*/ public void getResult(String result); }
Step 2: Interface callback (in Fragment)
/*Interface callback*/ public void getData(CallBack callBack){ /*Get the text box information, of course, you can also pass other types of parameters, see the requirements.*/ String msg = editText.getText().toString(); callBack.getResult(msg); }
Step 3: Use interface callback to read data (in Activity)
/* Data acquisition using interface callback */ leftFragment.getData(new CallBack() { @Override public void getResult(String result) { /*Print information*/ Toast.makeText(MainActivity.this, "-->>" + result, 1).show(); } });
(3) Data transmission between Fragments and Fragments
In fact, it is very simple to find the Fragment object to accept the data and call setArguments directly to transfer the data in. Usually, when replace, that is, when Fragment jumps to transfer the data, then you just need to call his setArguments method to transfer the data after initializing the Fragment to jump.
If two fragments need to transfer data in real time, rather than jumping, they need to get data from f1 in Activity first, and then to f2, that is, to use Activity as a medium.~
MainActivity
//[1] Acquisition of fragment managers FragmentManager fragmentManager = getFragmentManager(); //[2] Open a thing FragmentTransaction beginTransaction = fragmentManager.beginTransaction(); //[2.1] Replace fragment beginTransaction.replace(R.id.ll1, new Fragment1(),"f1"); beginTransaction.replace(R.id.ll2, new Fragment2(),"f2"); //[3] Open things beginTransaction.commit();
Like Fragment 2 (), Fragment 1 () only writes Fragment 1 ().
public class Fragment1 extends Fragment { //Calling this method when the system first draws the ui allows Fragment to display its layout content @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { //Converting a layout into a View object through an inflator View view = inflater.inflate(R.layout.fragment1, null); //[1] Find the button to set the click event view.findViewById(R.id.btn_update).setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { //[2] Modify the content of fragment 2 through the public bridge of fragment - > activity Fragment2 fragment2 = (Fragment2) getActivity().getFragmentManager().findFragmentByTag("f2"); fragment2.updateText("Ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha ha"); } }); return view; } }